Description
Definition of the OpenMP granular terrain node (using Chrono::Multicore).
#include <ChVehicleCosimTerrainNodeGranularOMP.h>
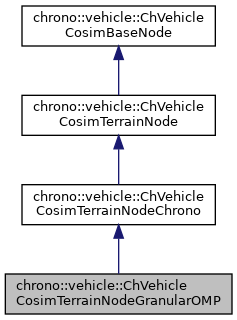
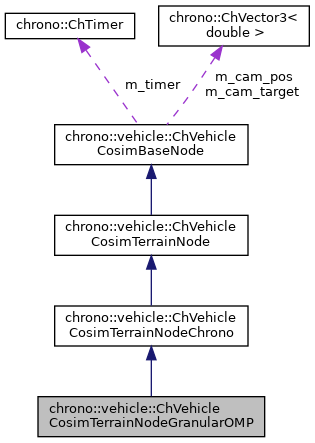
Public Member Functions | |
ChVehicleCosimTerrainNodeGranularOMP (double length, double width, ChContactMethod method) | |
Create a Chrono::Multicore granular terrain node using the specified contact method (SMC or NSC). | |
ChVehicleCosimTerrainNodeGranularOMP (ChContactMethod method, const std::string &specfile) | |
Create a Chrono::Multicore granular terrain node using the specified contact method (SMC or NSC) and set parameters from the provided JSON specfile. | |
virtual ChSystem * | GetSystem () override |
Return a pointer to the underlying Chrono system. | |
void | SetNumThreads (int num_threads) |
Set the number of OpenMP threads Chrono::multicore terrain simulation. | |
void | SetFromSpecfile (const std::string &specfile) |
Set full terrain specification from JSON specfile. | |
void | SetWallThickness (double thickness) |
Set container wall thickness (default: 0.2) | |
void | SetGranularMaterial (double radius, double density) |
Set properties of granular material. More... | |
void | SetMaterialSurface (const std::shared_ptr< ChContactMaterial > &mat) |
Set the material properties for terrain. More... | |
void | UseMaterialProperties (bool flag) |
Specify whether contact coefficients are based on material properties (default: true). More... | |
void | SetContactForceModel (ChSystemSMC::ContactForceModel model) |
Set the normal contact force model (default: Hertz). More... | |
void | SetTangentialDisplacementModel (ChSystemSMC::TangentialDisplacementModel model) |
Set the tangential contact displacement model (default: OneStep). More... | |
void | SetSamplingMethod (utils::SamplingType type, double init_height, double sep_factor=1.001, bool in_layers=false) |
Set sampling method for generation of granular material. More... | |
void | SetProxyContactRadius (double radius) |
Set sweeping sphere radius for proxy bodies (default 5e-3). More... | |
void | SetInputFromCheckpoint (const std::string &filename) |
Initialize granular terrain from the specified checkpoint file (which must exist in the output directory). More... | |
void | SetSettlingTime (double time) |
Set simulation length for settling of granular material (default: 0.4). | |
void | SetSettlingKineticEneryThreshold (double threshold) |
Set total kinetic energy threshold as stopping criteria for settling (default: 1e-3). | |
void | EnableSettlingOutput (bool output, double output_fps=100) |
Enable/disable output during settling (default: false). More... | |
void | Settle () |
Obtain settled terrain configuration. More... | |
virtual void | OnInitialize (unsigned int num_objects) override |
Initialize this Chrono terrain node. More... | |
virtual void | WriteCheckpoint (const std::string &filename) const override |
Write checkpoint to the specified file (which will be created in the output directory). | |
double | CalculatePackingDensity (double &depth) |
Estimate packing density (eta) of granular material in current configuration. More... | |
virtual void | OutputVisualizationData (int frame) override final |
Output post-processing visualization data. | |
![]() | |
Type | GetType () const |
Return the type of this terrain node. | |
void | SetProxyFixed (bool fixed) |
Set the proxy bodies as fixed to ground. | |
virtual double | GetInitHeight () const override final |
Return the terrain initial height. | |
void | AddRigidObstacle (const RigidObstacle &obstacle) |
Add a rigid obstacle. | |
![]() | |
virtual NodeType | GetNodeType () const override |
Return the node type as NodeType::TERRAIN. | |
void | SetDimensions (double length, double width) |
Set the terrain patch dimensions. More... | |
void | GetDimensions (double &length, double &width) |
Get the terrain patch dimensions. | |
virtual void | Initialize () override final |
Initialize this node. More... | |
virtual void | Synchronize (int step_number, double time) override final |
Synchronize this node. More... | |
virtual void | Advance (double step_size) override final |
Advance simulation. More... | |
virtual void | OutputData (int frame) override final |
Output logging and debugging data. | |
![]() | |
std::string | GetNodeTypeString () const |
Return the node type as a string. | |
bool | IsCosimNode () const |
Return true if this node is part of the co-simulation infrastructure. | |
void | SetStepSize (double step) |
Set the integration step size (default: 1e-4). | |
double | GetStepSize () const |
Get the integration step size. | |
void | SetOutDir (const std::string &dir_name, const std::string &suffix="") |
Set the name of the output directory and an identifying suffix. More... | |
void | SetVerbose (bool verbose) |
Enable/disable verbose messages during simulation (default: true). | |
void | EnableRuntimeVisualization (double render_fps=100, bool save_img=false) |
Enable run-time visualization (default: false). More... | |
void | SetCameraPosition (const ChVector3d &cam_pos, const ChVector3d &cam_target=VNULL) |
Set camera location and target point. | |
void | SetCameraTracking (bool track) |
Enable/disable tracking of objects (default: true). | |
void | EnablePostprocessVisualization (double render_fps=100) |
Enable Blender postprocessing (default: false). More... | |
const std::string & | GetOutDirName () const |
Get the output directory name for this node. | |
double | GetStepExecutionTime () const |
Get the simulation execution time for the current step on this node. More... | |
double | GetTotalExecutionTime () const |
Get the cumulative simulation execution time on this node. | |
void | Render (double step_size) |
Render simulation frame. More... | |
Additional Inherited Members | |
![]() | |
enum | Type { Type::RIGID, Type::SCM, Type::GRANULAR_OMP, Type::GRANULAR_GPU, Type::GRANULAR_SPH, Type::UNKNOWN } |
Type of Chrono terrain. More... | |
![]() | |
enum | NodeType { NodeType::MBS_WHEELED, NodeType::MBS_TRACKED, NodeType::TERRAIN, NodeType::TIRE } |
Type of node participating in co-simulation. More... | |
enum | InterfaceType { InterfaceType::BODY, InterfaceType::MESH } |
Type of the vehicle-terrain communication interface. More... | |
![]() | |
static std::string | GetTypeAsString (Type type) |
Return a string describing the type of this terrain node. | |
static Type | GetTypeFromString (const std::string &type) |
Infer the terrain node type from the given string. | |
static bool | ReadSpecfile (const std::string &specfile, rapidjson::Document &d) |
Read a JSON specification file for a Chrono terrain node. | |
static Type | GetTypeFromSpecfile (const std::string &specfile) |
Get the terrain type from the given JSON specification file. | |
static ChVector2d | GetSizeFromSpecfile (const std::string &specfile) |
Get the terrain dimensions (length and width) from the given JSON specification file. | |
![]() | |
static std::string | OutputFilename (const std::string &dir, const std::string &root, const std::string &ext, int frame, int frame_digits) |
Utility function for creating an output file name. More... | |
![]() | |
ChVehicleCosimTerrainNodeChrono (Type type, double length, double width, ChContactMethod method) | |
Construct a base class terrain node. More... | |
![]() | |
ChVehicleCosimTerrainNode (double length, double width) | |
Construct a terrain node to wrap a terrain patch of given length and width. | |
virtual void | OnSynchronize (int step_number, double time) |
Perform any additional operations after the data exchange and synchronization with the MBS node. More... | |
virtual void | UpdateMeshProxy (unsigned int i, MeshState &mesh_state) |
Update the state of the i-th proxy mesh. More... | |
![]() | |
ChVehicleCosimBaseNode (const std::string &name) | |
void | SendGeometry (const utils::ChBodyGeometry &geom, int dest) const |
Utility function to pack and send a struct with geometry information. | |
void | RecvGeometry (utils::ChBodyGeometry &geom, int source) const |
Utility function to receive and unpack a struct with geometry information. | |
void | ProgressBar (unsigned int x, unsigned int n, unsigned int w=50) |
Utility function to display a progress bar to the terminal. More... | |
![]() | |
Type | m_type |
terrain type | |
ChContactMethod | m_method |
contact method (SMC or NSC) | |
std::shared_ptr< ChContactMaterial > | m_material_terrain |
material properties for terrain bodies | |
double | m_init_height |
terrain initial height | |
std::vector< std::shared_ptr< Proxy > > | m_proxies |
proxies for solid objects | |
bool | m_fixed_proxies |
are proxies fixed to ground? | |
std::vector< RigidObstacle > | m_obstacles |
list of rigid obstacles | |
![]() | |
double | m_dimX |
patch length (X direction) | |
double | m_dimY |
patch width (Y direction) | |
std::vector< ChVector3d > | m_path_points |
vehicle path (optional) | |
bool | m_wheeled |
comm node (true: TIRE nodes, false: tracked MBS node) | |
InterfaceType | m_interface_type |
communication interface (body or mesh) | |
int | m_num_objects |
number of interacting objects | |
std::vector< ChVector3d > | m_init_loc |
initial locations of interacting objects | |
std::vector< ChAABB > | m_aabb |
AABB of collision models for interacting objects. | |
std::vector< utils::ChBodyGeometry > | m_geometry |
contact geometry and materials for interacting objects | |
std::vector< double > | m_load_mass |
vertical load on interacting objects | |
std::vector< int > | m_obj_map |
mapping from interacting object to shape | |
std::vector< MeshState > | m_mesh_state |
mesh state (used for MESH communication) | |
std::vector< BodyState > | m_rigid_state |
rigid state (used for BODY communication interface) | |
std::vector< MeshContact > | m_mesh_contact |
mesh contact forces (used for MESH communication interface) | |
std::vector< TerrainForce > | m_rigid_contact |
rigid contact force (used for BODY communication interface) | |
ChVector3d | m_chassis_loc |
current chassis location | |
![]() | |
int | m_rank |
MPI rank of this node (in MPI_COMM_WORLD) | |
double | m_step_size |
integration step size | |
std::string | m_name |
name of the node | |
std::string | m_out_dir |
top-level output directory | |
std::string | m_node_out_dir |
node-specific output directory | |
std::ofstream | m_outf |
output file stream | |
bool | m_renderRT |
if true, perform run-time rendering | |
bool | m_renderRT_all |
if true, render all frames | |
double | m_renderRT_step |
time step between rendered frames | |
bool | m_writeRT |
if true, write images to file | |
bool | m_renderPP |
if true, save data for post-processing | |
bool | m_renderPP_all |
if true, save data at all frames | |
double | m_renderPP_step |
time step between post-processing save frames | |
bool | m_track |
track objects | |
ChVector3d | m_cam_pos |
camera location | |
ChVector3d | m_cam_target |
camera target (lookat) point | |
unsigned int | m_num_wheeled_mbs_nodes |
unsigned int | m_num_tracked_mbs_nodes |
unsigned int | m_num_terrain_nodes |
unsigned int | m_num_tire_nodes |
ChTimer | m_timer |
timer for integration cost | |
double | m_cum_sim_time |
cumulative integration cost | |
bool | m_verbose |
verbose messages during simulation? | |
![]() | |
static const double | m_gacc = -9.81 |
Member Function Documentation
◆ CalculatePackingDensity()
double chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP::CalculatePackingDensity | ( | double & | depth | ) |
Estimate packing density (eta) of granular material in current configuration.
Note that porosity is phi=1-eta and void ratio is e=(1-eta)/eta=phi/(1-phi). The function also returns the current depth of granular material.
◆ EnableSettlingOutput()
void chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP::EnableSettlingOutput | ( | bool | output, |
double | output_fps = 100 |
||
) |
Enable/disable output during settling (default: false).
If enabled, output files are generated with the specified frequency.
◆ OnInitialize()
|
overridevirtual |
Initialize this Chrono terrain node.
Construct the terrain system and the proxy bodies, then finalize the underlying system.
Reimplemented from chrono::vehicle::ChVehicleCosimTerrainNodeChrono.
◆ SetContactForceModel()
void chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP::SetContactForceModel | ( | ChSystemSMC::ContactForceModel | model | ) |
Set the normal contact force model (default: Hertz).
Note that this setting is only relevant when using the SMC method.
◆ SetGranularMaterial()
void chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP::SetGranularMaterial | ( | double | radius, |
double | density | ||
) |
Set properties of granular material.
- Parameters
-
radius particle radius (default: 0.01) density particle material density (default: 2000)
◆ SetInputFromCheckpoint()
void chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP::SetInputFromCheckpoint | ( | const std::string & | filename | ) |
Initialize granular terrain from the specified checkpoint file (which must exist in the output directory).
By default, particles are created uniformly distributed in the specified domain such that they are initially not in contact.
◆ SetMaterialSurface()
void chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP::SetMaterialSurface | ( | const std::shared_ptr< ChContactMaterial > & | mat | ) |
Set the material properties for terrain.
The type of material must be consistent with the contact method (SMC or NSC) specified at construction. These parameters characterize the material for the container and (if applicable) the granular material. Object contact material is received from the rig node.
◆ SetProxyContactRadius()
|
inline |
Set sweeping sphere radius for proxy bodies (default 5e-3).
This value is used as a "thickness" for collision meshes (a non-zero value can improve robustness of the collision detection algorithm).
◆ SetSamplingMethod()
void chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP::SetSamplingMethod | ( | utils::SamplingType | type, |
double | init_height, | ||
double | sep_factor = 1.001 , |
||
bool | in_layers = false |
||
) |
Set sampling method for generation of granular material.
The granular material is created in the volume defined by the x-y dimensions of the terrain patch and the specified initial height, using the specified sampling type, layer by layer or all at once. Note: for correct HCP, do not initialize in layers!
- Parameters
-
type volume sampling type (default POISSON_DISK) init_height height of granular material at initialization (default 0.2) sep_factor radius inflation factor for initial separation in_layers initialize material in layers
◆ SetTangentialDisplacementModel()
void chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP::SetTangentialDisplacementModel | ( | ChSystemSMC::TangentialDisplacementModel | model | ) |
Set the tangential contact displacement model (default: OneStep).
Note that this setting is only relevant when using the SMC method.
◆ Settle()
void chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP::Settle | ( | ) |
Obtain settled terrain configuration.
This is an optional operation that can be performed for granular terrain before initiating communictation with the rig node. For granular terrain, a settled configuration can be obtained either through simulation or by initializing particles from a previously generated checkpointing file.
◆ UseMaterialProperties()
void chrono::vehicle::ChVehicleCosimTerrainNodeGranularOMP::UseMaterialProperties | ( | bool | flag | ) |
Specify whether contact coefficients are based on material properties (default: true).
Note that this setting is only relevant when using the SMC method.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/cosim/terrain/ChVehicleCosimTerrainNodeGranularOMP.h
- /builds/uwsbel/chrono/src/chrono_vehicle/cosim/terrain/ChVehicleCosimTerrainNodeGranularOMP.cpp