Description
Full double wishbone front suspension for the FEDA vehicle.
The control arms are modeled using rigid bodies.
#include <FEDA_DoubleWishbone.h>
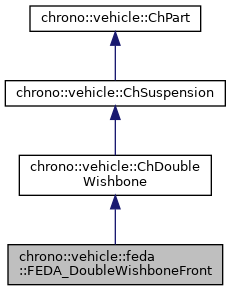
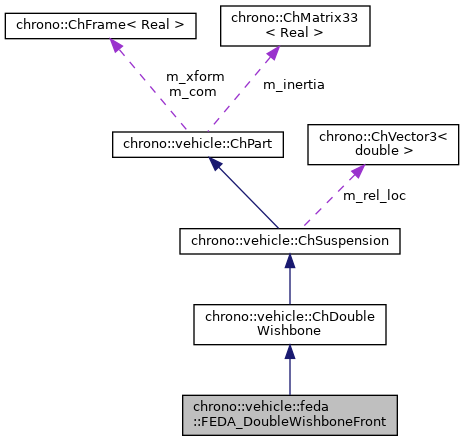
Public Member Functions | |
FEDA_DoubleWishboneFront (const std::string &name, int rideHeightMode=2, int damperMode=1) | |
virtual bool | UseTierodBodies () const override |
Indicate whether or not tirod bodies are modelled (default: false). More... | |
virtual double | getCamberAngle () const override |
Return the camber angle, in radians (default: 0). | |
virtual double | getToeAngle () const override |
Return the toe angle, in radians (default: 0). More... | |
virtual double | getSpindleMass () const override |
Return the mass of the spindle body. | |
virtual double | getUCAMass () const override |
Return the mass of the upper control arm body. | |
virtual double | getLCAMass () const override |
Return the mass of the lower control body. | |
virtual double | getUprightMass () const override |
Return the mass of the upright body. | |
virtual double | getTierodMass () const override |
Return the mass of the tierod body. | |
virtual double | getSpindleRadius () const override |
Return the radius of the spindle body (visualization only). | |
virtual double | getSpindleWidth () const override |
Return the width of the spindle body (visualization only). | |
virtual double | getUCARadius () const override |
Return the radius of the upper control arm body (visualization only). | |
virtual double | getLCARadius () const override |
Return the radius of the lower control arm body (visualization only). | |
virtual double | getUprightRadius () const override |
Return the radius of the upright body (visualization only). | |
virtual double | getTierodRadius () const override |
Return the radius of the tierod body (visualization only). | |
virtual const ChVector3d & | getSpindleInertia () const override |
Return the moments of inertia of the spindle body. | |
virtual const ChVector3d & | getUCAInertiaMoments () const override |
Return the moments of inertia of the upper control arm body. | |
virtual const ChVector3d & | getUCAInertiaProducts () const override |
Return the products of inertia of the upper control arm body. | |
virtual const ChVector3d & | getLCAInertiaMoments () const override |
Return the moments of inertia of the lower control arm body. | |
virtual const ChVector3d & | getLCAInertiaProducts () const override |
Return the products of inertia of the lower control arm body. | |
virtual const ChVector3d & | getUprightInertiaMoments () const override |
Return the moments of inertia of the upright body. | |
virtual const ChVector3d & | getUprightInertiaProducts () const override |
Return the products of inertia of the upright body. | |
virtual const ChVector3d | getTierodInertia () const override |
Return the moments of inertia of the tierod body. | |
virtual double | getAxleInertia () const override |
Return the inertia of the axle shaft. | |
virtual double | getSpringRestLength () const override |
Return the free (rest) length of the spring element. | |
virtual std::shared_ptr< ChLinkTSDA::ForceFunctor > | getSpringForceFunctor () const override |
Return the functor object for spring force. | |
virtual std::shared_ptr< ChLinkTSDA::ForceFunctor > | getShockForceFunctor () const override |
Return the functor object for shock force. | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis, std::shared_ptr< ChSubchassis > subchassis, std::shared_ptr< ChSteering > steering, const ChVector3d &location, double left_ang_vel=0, double right_ang_vel=0) override |
Initialize this suspension subsystem. More... | |
![]() | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual bool | IsSteerable () const final override |
Specify whether or not this suspension can be steered. | |
virtual bool | IsIndependent () const final override |
Specify whether or not this is an independent suspension. | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the suspension subsystem. More... | |
virtual void | RemoveVisualizationAssets () override |
Remove visualization assets for the suspension subsystem. | |
virtual double | GetTrack () override |
Get the wheel track for the suspension subsystem. | |
std::shared_ptr< ChLinkTSDA > | GetSpring (VehicleSide side) const |
Get a handle to the specified spring element. | |
std::shared_ptr< ChLinkTSDA > | GetShock (VehicleSide side) const |
Get a handle to the specified shock (damper) element. | |
virtual std::vector< ForceTSDA > | ReportSuspensionForce (VehicleSide side) const override |
Return current suspension TSDA force information on the specified side. | |
double | GetSpringForce (VehicleSide side) const |
Get the force in the spring element. | |
double | GetSpringLength (VehicleSide side) const |
Get the current length of the spring element. | |
double | GetSpringDeformation (VehicleSide side) const |
Get the current deformation of the spring element. | |
double | GetShockForce (VehicleSide side) const |
Get the force in the shock (damper) element. | |
double | GetShockLength (VehicleSide side) const |
Get the current length of the shock (damper) element. | |
double | GetShockVelocity (VehicleSide side) const |
Get the current deformation velocity of the shock (damper) element. | |
ChVector3d | GetBallJointPosLCA (VehicleSide side) |
Get position of the LCA ball joint (expressed in the global frame). | |
ChVector3d | GetBallJointPosUCA (VehicleSide side) |
Get position of the UCA ball joint (expressed in the global frame). | |
virtual void | LogConstraintViolations (VehicleSide side) override |
Log current constraint violations. | |
virtual std::shared_ptr< ChBody > | GetAntirollBody (VehicleSide side) const override |
Specify the suspension body on the specified side to attach a possible antirollbar subsystem. More... | |
void | LogHardpointLocations (const ChVector3d &ref, bool inches=false) |
Log the locations of all hardpoints. More... | |
![]() | |
const ChVector3d & | GetRelPosition () const |
Get the location of the suspension subsystem relative to the associated chassis reference frame. More... | |
std::shared_ptr< ChBody > | GetSpindle (VehicleSide side) const |
Get a handle to the spindle body on the specified side. | |
std::shared_ptr< ChShaft > | GetAxle (VehicleSide side) const |
Get a handle to the axle shaft on the specified side. | |
std::shared_ptr< ChLinkLockRevolute > | GetRevolute (VehicleSide side) const |
Get a handle to the revolute joint on the specified side. | |
const ChVector3d & | GetSpindlePos (VehicleSide side) const |
Get the global location of the spindle on the specified side. | |
ChQuaternion | GetSpindleRot (VehicleSide side) const |
Get the orientation of the spindle body on the specified side. More... | |
const ChVector3d & | GetSpindleLinVel (VehicleSide side) const |
Get the linear velocity of the spindle body on the specified side. More... | |
ChVector3d | GetSpindleAngVel (VehicleSide side) const |
Get the angular velocity of the spindle body on the specified side. More... | |
double | GetAxleSpeed (VehicleSide side) const |
Get the angular speed of the axle on the specified side. | |
virtual void | Synchronize (double time) |
Synchronize this suspension subsystem. | |
virtual void | Advance (double step) |
Advance the state of the suspension subsystem by the specified time step. | |
void | ApplyAxleTorque (VehicleSide side, double torque) |
Apply the provided motor torque. More... | |
virtual std::shared_ptr< ChBody > | GetBrakeBody (VehicleSide side) const |
Specify the body on the specified side for a possible connection to brake subsystem. More... | |
virtual std::vector< ForceRSDA > | ReportSuspensionTorque (VehicleSide side) const |
Return current RSDA torque information on the specified side. | |
void | ApplyParkingBrake (bool brake) |
Simple model of a parking brake. | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
enum | PointId { SPINDLE, UPRIGHT, UCA_F, UCA_B, UCA_U, UCA_CM, LCA_F, LCA_B, LCA_U, LCA_CM, SHOCK_C, SHOCK_A, SPRING_C, SPRING_A, TIEROD_C, TIEROD_U, NUM_POINTS } |
Identifiers for the various hardpoints. More... | |
![]() | |
ChDoubleWishbone (const std::string &name, bool vehicle_frame_inertia=false) | |
Protected constructor. More... | |
void | SetVehicleFrameInertiaFlag (bool val) |
Indicate whether or not inertia matrices are specified with respect to a vehicle-aligned centroidal frame (flag=true) or with respect to the body centroidal frame (flag=false). More... | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
virtual double | getShockRestLength () const |
Return the free (rest) length of the shock element. | |
virtual std::shared_ptr< ChVehicleBushingData > | getUCABushingData () const |
Return stiffness and damping data for the UCA bushing. More... | |
virtual std::shared_ptr< ChVehicleBushingData > | getLCABushingData () const |
Return stiffness and damping data for the LCA bushing. More... | |
virtual std::shared_ptr< ChVehicleBushingData > | getTierodBushingData () const |
Return stiffness and damping data for the tierod bushings. More... | |
![]() | |
ChSuspension (const std::string &name) | |
Construct a suspension subsystem with given name. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
![]() | |
std::shared_ptr< ChBody > | m_upright [2] |
upright bodies (left/right) | |
std::shared_ptr< ChBody > | m_UCA [2] |
upper control arm bodies (left/right) | |
std::shared_ptr< ChBody > | m_LCA [2] |
lower control arm bodies (left/right) | |
std::shared_ptr< ChBody > | m_tierod [2] |
tierod bodies, if used (left/right) | |
std::shared_ptr< ChVehicleJoint > | m_revoluteUCA [2] |
chassis-UCA revolute joints (left/right) | |
std::shared_ptr< ChVehicleJoint > | m_sphericalUCA [2] |
upright-UCA spherical joints (left/right) | |
std::shared_ptr< ChVehicleJoint > | m_revoluteLCA [2] |
chassis-LCA revolute joints (left/right) | |
std::shared_ptr< ChVehicleJoint > | m_sphericalLCA [2] |
upright-LCA spherical joints (left/right) | |
std::shared_ptr< ChLinkDistance > | m_distTierod [2] |
tierod distance constraints (left/right) | |
std::shared_ptr< ChVehicleJoint > | m_sphericalTierod [2] |
tierod-upright spherical joints (left/right) | |
std::shared_ptr< ChVehicleJoint > | m_universalTierod [2] |
tierod-chassis universal joints (left/right) | |
std::shared_ptr< ChLinkTSDA > | m_shock [2] |
spring links (left/right) | |
std::shared_ptr< ChLinkTSDA > | m_spring [2] |
shock links (left/right) | |
![]() | |
ChVector3d | m_rel_loc |
location relative to chassis | |
std::shared_ptr< ChBody > | m_spindle [2] |
handles to spindle bodies | |
std::shared_ptr< ChShaft > | m_axle [2] |
handles to axle shafts | |
std::shared_ptr< ChShaftBodyRotation > | m_axle_to_spindle [2] |
handles to spindle-shaft connectors | |
std::shared_ptr< ChLinkLockRevolute > | m_revolute [2] |
handles to spindle revolute joints | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
Member Function Documentation
◆ getToeAngle()
|
inlineoverridevirtual |
Return the toe angle, in radians (default: 0).
A positive value indicates toe-in, a negative value indicates toe-out.
Reimplemented from chrono::vehicle::ChDoubleWishbone.
◆ Initialize()
|
overridevirtual |
Initialize this suspension subsystem.
The suspension subsystem is initialized by attaching it to the specified chassis and (if provided) to the specified subchassis, at the specified location (with respect to and expressed in the reference frame of the chassis). It is assumed that the suspension reference frame is always aligned with the chassis reference frame. If a steering subsystem is provided, the suspension tierods are to be attached to the steering's central link body (steered suspension); otherwise they are to be attached to the chassis (non-steered suspension).
- Parameters
-
[in] chassis associated chassis subsystem [in] subchassis associated subchassis subsystem (may be null) [in] steering associated steering subsystem (may be null) [in] location location relative to the chassis frame [in] left_ang_vel initial angular velocity of left wheel [in] right_ang_vel initial angular velocity of right wheel
Reimplemented from chrono::vehicle::ChDoubleWishbone.
◆ UseTierodBodies()
|
inlineoverridevirtual |
Indicate whether or not tirod bodies are modelled (default: false).
If false, tierods are modelled using distance constraints. If true, rigid tierod bodies are created (in which case a derived class must provide the mass and inertia) and connected either with kinematic joints or bushings (depending on whether or not bushing data is defined).
Reimplemented from chrono::vehicle::ChDoubleWishbone.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_models/vehicle/feda/FEDA_DoubleWishbone.h
- /builds/uwsbel/chrono/src/chrono_models/vehicle/feda/FEDA_DoubleWishbone.cpp