Description
Base class for a sub-chassis system for wheeled vehicles.
#include <ChSubchassis.h>
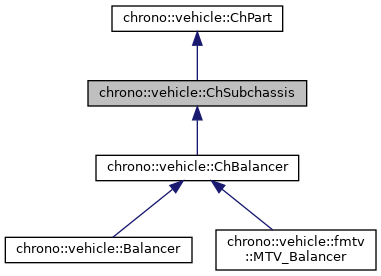
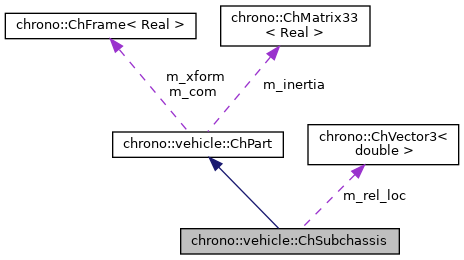
Public Member Functions | |
const ChVector3d & | GetLocation () const |
Get the location of the subchassis relative to the chassis reference frame. More... | |
std::shared_ptr< ChBody > | GetBeam (VehicleSide side) const |
Get a handle to the beam body on the specified side. | |
void | Initialize (std::shared_ptr< ChChassis > chassis, const ChVector3d &location) |
Initialize this subchassis subsystem. More... | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
virtual uint16_t | GetVehicleTag () const |
Get the tag of the associated vehicle. More... | |
int | GetBodyTag () const |
Get the tag for component bodies. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Protected Member Functions | |
ChSubchassis (const std::string &name) | |
Construct a subchassis subsystem with given name. | |
virtual void | Construct (std::shared_ptr< ChChassis > chassis, const ChVector3d &location)=0 |
Construct the concrete subchassis subsystem. More... | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
virtual void | InitializeInertiaProperties ()=0 |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties ()=0 |
Update subsystem inertia properties. More... | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Protected Attributes | |
ChVector3d | m_rel_loc |
location relative to chassis | |
std::shared_ptr< ChBody > | m_beam [2] |
handles to beam bodies | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
int | m_obj_tag |
tag for part objects | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Member Function Documentation
◆ Construct()
|
protectedpure virtual |
Construct the concrete subchassis subsystem.
The subchassis is initialized by attaching it to the specified chassis at the specified location (with respect to and expressed in the reference frame of the chassis). It is assumed that the subchassis reference frame is always aligned with the chassis reference frame.
- Parameters
-
[in] chassis associated chassis [in] location location relative to the chassis frame
Implemented in chrono::vehicle::ChBalancer.
◆ GetLocation()
|
inline |
Get the location of the subchassis relative to the chassis reference frame.
The subchassis reference frame is always aligned with the chassis reference frame.
◆ Initialize()
void chrono::vehicle::ChSubchassis::Initialize | ( | std::shared_ptr< ChChassis > | chassis, |
const ChVector3d & | location | ||
) |
Initialize this subchassis subsystem.
The subchassis is initialized by attaching it to the specified chassis at the specified location (with respect to and expressed in the reference frame of the chassis). It is assumed that the subchassis reference frame is always aligned with the chassis reference frame.
- Parameters
-
[in] chassis associated chassis [in] location location relative to the chassis frame
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/ChSubchassis.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/ChSubchassis.cpp