Description
Markers are auxiliary reference frames attached to a rigid body and moving with the body.
Most often, markers are used as references to build ChLink() constraints between two rigid bodies. ChMarker also allows user-defined motion laws of the marker with respect to the parent body, e.g., to represent imposed trajectories.
#include <ChMarker.h>
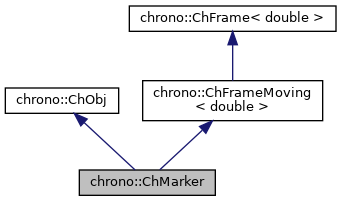
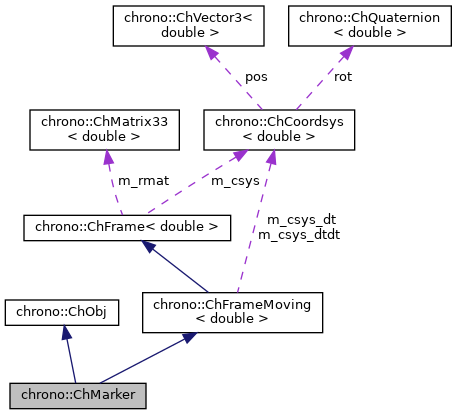
Public Types | |
enum | MotionType { MotionType::FUNCTIONS, MotionType::KEYFRAMED, MotionType::EXTERNAL } |
Public Member Functions | |
ChMarker (const std::string &name, ChBody *body, const ChCoordsys<> &rel_csys, const ChCoordsys<> &rel_csys_dt, const ChCoordsys<> &rel_csys_dtdt) | |
ChMarker (const ChMarker &other) | |
virtual ChMarker * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
ChBody * | GetBody () const |
Gets a pointer to the associated rigid body. | |
void | SetBody (ChBody *body) |
Sets the parent rigid body. | |
void | ImposeRelativeTransform (const ChFrame<> &frame) |
Set body-relative marker frame and update auxiliary variables. More... | |
void | ImposeAbsoluteTransform (const ChFrame<> &frame) |
Set absolute coordinate marker frame and update auxiliary variables. More... | |
const ChCoordsysd & | GetRestCoordsys () const |
Get the 'resting position'. More... | |
const ChFrameMoving< double > & | GetAbsFrame () const |
Get reference to the inner 'absolute frame' auxiliary coordinates. More... | |
const ChCoordsysd & | GetAbsCoordsys () const |
Get the translation and rotation (as a ChCoordsysd) with respect to the absolute coordinates. | |
const ChCoordsysd & | GetAbsCoordsysDt () const |
Get the speed of translation and rotation (as a derived ChCoordsysd) with respect to the absolute coordinates. | |
const ChCoordsysd & | GetAbsCoordsysDt2 () const |
Get the acceleration of translation and rotation (as a derived ChCoordsysd) with respect to the absolute coordinates. | |
void | SetAbsCoordsys (const ChCoordsysd &csys) |
Set the translation and rotation (as a ChCoordsysd) with respect to the absolute coordinates. More... | |
void | SetAbsCoordsysDt (const ChCoordsysd &csys_dt) |
Set the speed of translation and rotation (as a ChCoordsysd) with respect to the absolute coordinates. More... | |
void | SetAbsCoordsysDt2 (const ChCoordsysd &csys_dtdt) |
Set the speed of translation and rotation (as a ChCoordsysd) with respect to the absolute coordinates. More... | |
ChVector3d | GetAbsAngVel () const |
Get the angular velocity with respect to global frame, expressed in absolute coordinates. | |
ChVector3d | GetAbsAngAcc () const |
Get the angular acceleration with respect to global frame, expressed in absolute coordinates. | |
void | SetMotionType (MotionType motion_type) |
Set the imposed motion type (default: MotionType::FUNCTIONS). | |
void | SetMotionX (std::shared_ptr< ChFunction > funct) |
Set the imposed motion law, for translation on X body axis. | |
void | SetMotionY (std::shared_ptr< ChFunction > funct) |
Set the imposed motion law, for translation on Y body axis. | |
void | SetMotionZ (std::shared_ptr< ChFunction > funct) |
Set the imposed motion law, for translation on Z body axis. | |
void | SetMotionAngle (std::shared_ptr< ChFunction > funct) |
Set the imposed motion law, for rotation about an axis. | |
void | SetMotionAxis (ChVector3d axis) |
Set the axis of rotation, if rotation motion law is used. | |
MotionType | GetMotionType () const |
Get the imposed motion type. | |
std::shared_ptr< ChFunction > | GetMotionX () const |
Get imposed motion law, for translation on X body axis. | |
std::shared_ptr< ChFunction > | GetMotionY () const |
Get imposed motion law, for translation on Y body axis. | |
std::shared_ptr< ChFunction > | GetMotionZ () const |
Get imposed motion law, for translation on Z body axis. | |
std::shared_ptr< ChFunction > | GetMotionAngle () const |
Get imposed motion law, for rotation about an axis. | |
ChVector3d | GetMotionAxis () const |
Get the axis of rotation, if rotation motion law is used. | |
void | UpdateTime (double mytime) |
Update the time-dependent variables (e.g., function objects to impose the body-relative motion). | |
void | UpdateState () |
Update auxiliary variables (e.g., the m_abs_frame data) at current state. | |
void | Update (double mytime) |
Call UpdateTime() and UpdateState() at once. | |
void | UpdatedExternalTime (double prevtime, double mtime) |
Update time from external signal. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow deserialization of transient data from archives. | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Get the unique integer identifier of this object. More... | |
void | SetTag (int tag) |
Set an object integer tag (default: -1). More... | |
int | GetTag () const |
Get the tag of this object. | |
void | SetName (const std::string &myname) |
Set the name of this object. | |
const std::string & | GetName () const |
Get the name of this object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
virtual std::string & | ArchiveContainerName () |
![]() | |
ChFrameMoving () | |
Default constructor (identity frame). | |
ChFrameMoving (const ChVector3< double > &v, const ChQuaternion< double > &q=ChQuaternion< double >(1, 0, 0, 0)) | |
Construct from pos and rot (as a quaternion). | |
ChFrameMoving (const ChVector3< double > &v, const ChMatrix33< double > &R) | |
Construct from pos and rotation (as a 3x3 matrix). | |
ChFrameMoving (const ChCoordsys< double > &C) | |
Construct from a coordsys. | |
ChFrameMoving (const ChFrame< double > &F) | |
Construct from a frame. | |
ChFrameMoving (const ChFrameMoving< double > &other) | |
Copy constructor, build from another moving frame. | |
virtual | ~ChFrameMoving () |
Destructor. | |
ChFrameMoving< double > & | operator= (const ChFrameMoving< double > &other) |
Assignment operator: copy from another moving frame. | |
ChFrameMoving< double > & | operator= (const ChFrame< double > &other) |
Assignment operator: copy from another frame. | |
bool | operator== (const ChFrameMoving< double > &other) const |
Returns true for identical frames. | |
bool | operator!= (const ChFrameMoving< double > &other) const |
Returns true for different frames. | |
ChFrameMoving< double > | operator>> (const ChFrameMoving< double > &F) const |
Transform another frame through this frame. More... | |
ChFrameMoving< double > | operator* (const ChFrameMoving< double > &F) const |
Transform another frame through this frame. More... | |
ChFrameMoving< double > & | operator>>= (const ChFrameMoving< double > &F) |
Transform this frame by pre-multiplication with another frame. More... | |
ChFrameMoving< double > & | operator>>= (const ChVector3< double > &v) |
Transform this frame by pre-multiplication with a given vector (translate frame). | |
ChFrameMoving< double > & | operator>>= (const ChQuaternion< double > &q) |
Transform this frame by pre-multiplication with a given quaternion (rotate frame). | |
ChFrameMoving< double > & | operator>>= (const ChCoordsys< double > &C) |
Transform this frame by pre-multiplication with a given coordinate system. | |
ChFrameMoving< double > & | operator>>= (const ChFrame< double > &F) |
Transform this frame by pre-multiplication with another frame. | |
ChFrameMoving< double > & | operator*= (const ChFrameMoving< double > &F) |
Transform this frame by post-multiplication with another frame. More... | |
const ChCoordsys< double > & | GetCoordsysDt () const |
Return both rotation and translation velocities as a ChCoordsys object. | |
const ChCoordsys< double > & | GetCoordsysDt2 () const |
Return both rotation and translation accelerations as a ChCoordsys object. | |
const ChVector3< double > & | GetPosDt () const |
Return the linear velocity. | |
const ChVector3< double > & | GetLinVel () const |
Return the linear velocity. | |
const ChVector3< double > & | GetPosDt2 () const |
Return the linear acceleration. | |
const ChVector3< double > & | GetLinAcc () const |
Return the linear acceleration. | |
const ChQuaternion< double > & | GetRotDt () const |
Return the rotation velocity as a quaternion. | |
const ChQuaternion< double > & | GetRotDt2 () const |
Return the rotation acceleration as a quaternion. | |
ChVector3< double > | GetAngVelLocal () const |
Compute the angular velocity (expressed in local coords). | |
ChVector3< double > | GetAngVelParent () const |
Compute the actual angular velocity (expressed in parent coords). | |
ChVector3< double > | GetAngAccLocal () const |
Compute the actual angular acceleration (expressed in local coords). | |
ChVector3< double > | GetAngAccParent () const |
Compute the actual angular acceleration (expressed in parent coords). | |
virtual void | SetCoordsysDt (const ChCoordsys< double > &csys_dt) |
Set both linear and rotation velocities as a single ChCoordsys derivative. | |
virtual void | SetPosDt (const ChVector3< double > &vel) |
Set the linear velocity. | |
virtual void | SetLinVel (const ChVector3< double > &vel) |
Set the linear velocity. | |
virtual void | SetRotDt (const ChQuaternion< double > &q_dt) |
Set the rotation velocity as a quaternion derivative. More... | |
virtual void | SetAngVelLocal (const ChVector3< double > &w) |
Set the rotation velocity from the given angular velocity (expressed in local coordinates). | |
virtual void | SetAngVelParent (const ChVector3< double > &w) |
Set the rotation velocity from given angular velocity (expressed in parent coordinates). | |
virtual void | SetCoordsysDt2 (const ChCoordsys< double > &csys_dtdt) |
Set the linear and rotation accelerations as a single ChCoordsys derivative. | |
virtual void | SetPosDt2 (const ChVector3< double > &acc) |
Set the linear acceleration. | |
virtual void | SetLinAcc (const ChVector3< double > &acc) |
Set the linear acceleration. | |
virtual void | SetRotDt2 (const ChQuaternion< double > &q_dtdt) |
Set the rotation acceleration as a quaternion derivative. More... | |
virtual void | SetAngAccLocal (const ChVector3< double > &a) |
Set the rotation acceleration from given angular acceleration (expressed in local coordinates). More... | |
virtual void | SetAngAccParent (const ChVector3< double > &a) |
Set the rotation acceleration from given angular acceleration (expressed in parent coordinates). | |
void | ComputeRotMatDt (ChMatrix33< double > &R_dt) const |
Compute the time derivative of the rotation matrix. | |
void | ComputeRotMatDt2 (ChMatrix33< double > &R_dtdt) |
Compute the second time derivative of the rotation matrix. | |
ChMatrix33< double > | GetRotMatDt () |
Return the time derivative of the rotation matrix. | |
ChMatrix33< double > | GetRotMatDt2 () |
Return the second time derivative of the rotation matrix. | |
void | ConcatenatePreTransformation (const ChFrameMoving< double > &F) |
Apply a transformation (rotation and translation) represented by another frame. More... | |
void | ConcatenatePostTransformation (const ChFrameMoving< double > &F) |
Apply a transformation (rotation and translation) represented by another frame F in local coordinate. More... | |
ChVector3< double > | PointSpeedLocalToParent (const ChVector3< double > &localpos) const |
Return the velocity in the parent frame of a point fixed to this frame and expressed in local coordinates. | |
ChVector3< double > | PointSpeedLocalToParent (const ChVector3< double > &localpos, const ChVector3< double > &localspeed) const |
Return the velocity in the parent frame of a moving point, given the point location and velocity expressed in local coordinates. | |
ChVector3< double > | PointAccelerationLocalToParent (const ChVector3< double > &localpos) const |
Return the acceleration in the parent frame of a point fixed to this frame and expressed in local coordinates. More... | |
ChVector3< double > | PointAccelerationLocalToParent (const ChVector3< double > &localpos, const ChVector3< double > &localspeed, const ChVector3< double > &localacc) const |
Return the acceleration in the parent frame of a moving point, given the point location, velocity, and acceleration expressed in local coordinates. | |
ChVector3< double > | PointSpeedParentToLocal (const ChVector3< double > &parentpos, const ChVector3< double > &parentspeed) const |
Return the velocity of a point expressed in this frame, given the point location and velocity in the parent frame. | |
ChVector3< double > | PointAccelerationParentToLocal (const ChVector3< double > &parentpos, const ChVector3< double > &parentspeed, const ChVector3< double > &parentacc) const |
Return the acceleration of a point expressed in this frame, given the point location, velocity, and acceleration in the parent frame. | |
ChFrameMoving< double > | TransformLocalToParent (const ChFrameMoving< double > &F) const |
Transform a moving frame from 'this' local coordinate system to parent frame coordinate system. | |
ChFrameMoving< double > | TransformParentToLocal (const ChFrameMoving< double > &F) const |
Transform a moving frame from the parent coordinate system to 'this' local frame coordinate system. | |
bool | Equals (const ChFrameMoving< double > &other) const |
Returns true if this transform is identical to the other transform. | |
bool | Equals (const ChFrameMoving< double > &other, double tol) const |
Returns true if this transform is equal to the other transform, within a tolerance 'tol'. | |
virtual void | Invert () override |
Invert in place. More... | |
ChFrameMoving< double > | GetInverse () const |
Return the inverse transform. | |
![]() | |
ChFrame () | |
Default constructor (identity frame). | |
ChFrame (const ChVector3< double > &v, const ChQuaternion< double > &q=ChQuaternion< double >(1, 0, 0, 0)) | |
Construct from position and rotation (as quaternion). | |
ChFrame (const ChVector3< double > &v, const ChMatrix33< double > &R) | |
Construct from pos and rotation (as a 3x3 matrix). | |
ChFrame (const ChVector3< double > &v, const double angle, const ChVector3< double > &u) | |
Construct from position mv and rotation of angle alpha around unit vector mu. | |
ChFrame (const ChCoordsys< double > &C) | |
Construct from a coordsys. | |
ChFrame (const ChFrame< double > &other) | |
Copy constructor, build from another frame. | |
ChFrame< double > & | operator= (const ChFrame< double > &other) |
Assignment operator: copy from another frame. | |
bool | operator== (const ChFrame< double > &other) const |
Returns true for identical frames. | |
bool | operator!= (const ChFrame< double > &other) const |
Returns true for different frames. | |
ChFrame< double > | operator* (const ChFrame< double > &F) const |
Transform another frame through this frame. More... | |
ChVector3< double > | operator* (const ChVector3< double > &v) const |
Transform a vector through this frame (express in parent frame). More... | |
ChFrame< double > | operator>> (const ChFrame< double > &F) const |
Transform another frame through this frame. More... | |
ChVector3< double > | operator/ (const ChVector3< double > &v) const |
Transform a vector through this frame (express from parent frame). More... | |
ChFrame< double > & | operator>>= (const ChFrame< double > &F) |
Transform this frame by pre-multiplication with another frame. More... | |
ChFrame< double > & | operator>>= (const ChVector3< double > &v) |
Transform this frame by pre-multiplication with a given vector (translate frame). | |
ChFrame< double > & | operator>>= (const ChQuaternion< double > &q) |
Transform this frame by pre-multiplication with a given quaternion (rotate frame). | |
ChFrame< double > & | operator>>= (const ChCoordsys< double > &C) |
Transform this frame by pre-multiplication with a given coordinate system. | |
ChFrame< double > & | operator*= (const ChFrame< double > &F) |
Transform this frame by post-multiplication with another frame. More... | |
const ChCoordsys< double > & | GetCoordsys () const |
Return both current rotation and translation as a ChCoordsys object. | |
const ChVector3< double > & | GetPos () const |
Return the current translation vector. | |
const ChQuaternion< double > & | GetRot () const |
Return the current rotation quaternion. | |
const ChMatrix33< double > & | GetRotMat () const |
Return the current 3x3 rotation matrix. | |
ChVector3< double > | GetRotAxis () const |
Get axis of finite rotation, in parent space. | |
double | GetRotAngle () const |
Get angle of rotation about axis of finite rotation. | |
void | SetCoordsys (const ChCoordsys< double > &C) |
Impose both translation and rotation as a single ChCoordsys. More... | |
void | SetCoordsys (const ChVector3< double > &v, const ChQuaternion< double > &q) |
Impose both translation and rotation. More... | |
void | SetRot (const ChQuaternion< double > &q) |
Impose the rotation as a quaternion. More... | |
void | SetRot (const ChMatrix33< double > &R) |
Impose the rotation as a 3x3 matrix. More... | |
void | SetPos (const ChVector3< double > &pos) |
Impose the translation vector. | |
void | ConcatenatePreTransformation (const ChFrame< double > &F) |
Apply a transformation (rotation and translation) represented by another frame. More... | |
void | ConcatenatePostTransformation (const ChFrame< double > &F) |
Apply a transformation (rotation and translation) represented by another frame F in local coordinate. More... | |
void | Move (const ChVector3< double > &v) |
An easy way to move the frame by the amount specified by vector v, (assuming v expressed in parent coordinates) | |
void | Move (const ChCoordsys< double > &C) |
Apply both translation and rotation, assuming both expressed in parent coordinates, as a vector for translation and quaternion for rotation,. | |
ChVector3< double > | TransformPointLocalToParent (const ChVector3< double > &v) const |
Transform a point from the local frame coordinate system to the parent coordinate system. | |
ChVector3< double > | TransformPointParentToLocal (const ChVector3< double > &v) const |
Transforms a point from the parent coordinate system to local frame coordinate system. | |
ChVector3< double > | TransformDirectionLocalToParent (const ChVector3< double > &d) const |
Transform a direction from the parent frame coordinate system to 'this' local coordinate system. | |
ChVector3< double > | TransformDirectionParentToLocal (const ChVector3< double > &d) const |
Transforms a direction from 'this' local coordinate system to parent frame coordinate system. | |
ChWrench< double > | TransformWrenchLocalToParent (const ChWrench< double > &w) const |
Transform a wrench from the local coordinate system to the parent coordinate system. | |
ChWrench< double > | TransformWrenchParentToLocal (const ChWrench< double > &w) const |
Transform a wrench from the parent coordinate system to the local coordinate system. | |
ChFrame< double > | TransformLocalToParent (const ChFrame< double > &F) const |
Transform a frame from 'this' local coordinate system to parent frame coordinate system. | |
ChFrame< double > | TransformParentToLocal (const ChFrame< double > &F) const |
Transform a frame from the parent coordinate system to 'this' local frame coordinate system. | |
bool | Equals (const ChFrame< double > &other) const |
Returns true if this transform is identical to the other transform. | |
bool | Equals (const ChFrame< double > &other, double tol) const |
Returns true if this transform is equal to the other transform, within a tolerance 'tol'. | |
void | Normalize () |
Normalize the rotation, so that quaternion has unit length. | |
virtual void | SetIdentity () |
Sets to no translation and no rotation. | |
ChFrame< double > | GetInverse () const |
Return the inverse transform. | |
Additional Inherited Members | |
![]() | |
int | GenerateUniqueIdentifier () |
![]() | |
double | ChTime |
object simulation time | |
std::string | m_name |
object name | |
int | m_identifier |
object unique identifier | |
int | m_tag |
user-supplied tag | |
![]() | |
ChCoordsys< double > | m_csys_dt |
rotation and position velocity, as vector + quaternion | |
ChCoordsys< double > | m_csys_dtdt |
rotation and position acceleration, as vector + quaternion | |
![]() | |
ChCoordsys< double > | m_csys |
position and rotation, as vector + quaternion | |
ChMatrix33< double > | m_rmat |
3x3 orthogonal rotation matrix | |
Member Enumeration Documentation
◆ MotionType
|
strong |
Member Function Documentation
◆ GetAbsFrame()
|
inline |
Get reference to the inner 'absolute frame' auxiliary coordinates.
This object (coordinates/speeds/accel. of marker expressed in absolute coordinates) is useful for performance reasons. Note! it is updated only after each Update() function.
◆ GetRestCoordsys()
|
inline |
Get the 'resting position'.
This is the position which the marker should have when the x,y,z motion laws are at time=0.
◆ ImposeAbsoluteTransform()
void chrono::ChMarker::ImposeAbsoluteTransform | ( | const ChFrame<> & | frame | ) |
Set absolute coordinate marker frame and update auxiliary variables.
The current position becomes the 'resting position' coordinates for the current time.
◆ ImposeRelativeTransform()
void chrono::ChMarker::ImposeRelativeTransform | ( | const ChFrame<> & | frame | ) |
Set body-relative marker frame and update auxiliary variables.
The current position becomes the 'resting position' coordinates for the current time.
◆ SetAbsCoordsys()
|
inline |
Set the translation and rotation (as a ChCoordsysd) with respect to the absolute coordinates.
NOTE! internal use only, for the moment. Use ImposeAbsoluteTransform() if needed.
◆ SetAbsCoordsysDt()
|
inline |
Set the speed of translation and rotation (as a ChCoordsysd) with respect to the absolute coordinates.
NOTE! internal use only, for the moment.
◆ SetAbsCoordsysDt2()
|
inline |
Set the speed of translation and rotation (as a ChCoordsysd) with respect to the absolute coordinates.
NOTE! internal use only, for the moment.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChMarker.h
- /builds/uwsbel/chrono/src/chrono/physics/ChMarker.cpp