Description
template<class Real = double>
class chrono::ChFrameMoving< Real >
Representation of a moving 3D.
A ChFrameMoving is a ChFrame that also keeps track of the frame velocity and acceleration.
See Coordinate Systems manual page.
#include <ChFrameMoving.h>
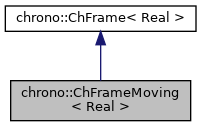
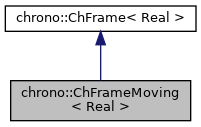
Public Member Functions | |
ChFrameMoving () | |
Default constructor (identity frame). | |
ChFrameMoving (const ChVector3< Real > &v, const ChQuaternion< Real > &q=ChQuaternion< Real >(1, 0, 0, 0)) | |
Construct from pos and rot (as a quaternion). | |
ChFrameMoving (const ChVector3< Real > &v, const ChMatrix33< Real > &R) | |
Construct from pos and rotation (as a 3x3 matrix). | |
ChFrameMoving (const ChCoordsys< Real > &C) | |
Construct from a coordsys. | |
ChFrameMoving (const ChFrame< Real > &F) | |
Construct from a frame. | |
ChFrameMoving (const ChFrameMoving< Real > &other) | |
Copy constructor, build from another moving frame. | |
virtual | ~ChFrameMoving () |
Destructor. | |
ChFrameMoving< Real > & | operator= (const ChFrameMoving< Real > &other) |
Assignment operator: copy from another moving frame. | |
ChFrameMoving< Real > & | operator= (const ChFrame< Real > &other) |
Assignment operator: copy from another frame. | |
bool | operator== (const ChFrameMoving< Real > &other) const |
Returns true for identical frames. | |
bool | operator!= (const ChFrameMoving< Real > &other) const |
Returns true for different frames. | |
ChFrameMoving< Real > | operator>> (const ChFrameMoving< Real > &F) const |
Transform another frame through this frame. More... | |
ChFrameMoving< Real > | operator* (const ChFrameMoving< Real > &F) const |
Transform another frame through this frame. More... | |
ChFrameMoving< Real > & | operator>>= (const ChFrameMoving< Real > &F) |
Transform this frame by pre-multiplication with another frame. More... | |
ChFrameMoving< Real > & | operator*= (const ChFrameMoving< Real > &F) |
Transform this frame by post-multiplication with another frame. More... | |
ChFrameMoving< Real > & | operator>>= (const ChVector3< Real > &v) |
Transform this frame by pre-multiplication with a given vector (translate frame). | |
ChFrameMoving< Real > & | operator>>= (const ChQuaternion< Real > &q) |
Transform this frame by pre-multiplication with a given quaternion (rotate frame). | |
ChFrameMoving< Real > & | operator>>= (const ChCoordsys< Real > &C) |
Transform this frame by pre-multiplication with a given coordinate system. | |
ChFrameMoving< Real > & | operator>>= (const ChFrame< Real > &F) |
Transform this frame by pre-multiplication with another frame. | |
const ChCoordsys< Real > & | GetCoordsysDt () const |
Return both rotation and translation velocities as a ChCoordsys object. | |
const ChCoordsys< Real > & | GetCoordsysDt2 () const |
Return both rotation and translation accelerations as a ChCoordsys object. | |
const ChVector3< Real > & | GetPosDt () const |
Return the linear velocity. | |
const ChVector3< Real > & | GetLinVel () const |
Return the linear velocity. | |
const ChVector3< Real > & | GetPosDt2 () const |
Return the linear acceleration. | |
const ChVector3< Real > & | GetLinAcc () const |
Return the linear acceleration. | |
const ChQuaternion< Real > & | GetRotDt () const |
Return the rotation velocity as a quaternion. | |
const ChQuaternion< Real > & | GetRotDt2 () const |
Return the rotation acceleration as a quaternion. | |
ChVector3< Real > | GetAngVelLocal () const |
Compute the angular velocity (expressed in local coords). | |
ChVector3< Real > | GetAngVelParent () const |
Compute the actual angular velocity (expressed in parent coords). | |
ChVector3< Real > | GetAngAccLocal () const |
Compute the actual angular acceleration (expressed in local coords). | |
ChVector3< Real > | GetAngAccParent () const |
Compute the actual angular acceleration (expressed in parent coords). | |
virtual void | SetCoordsysDt (const ChCoordsys< Real > &csys_dt) |
Set both linear and rotation velocities as a single ChCoordsys derivative. | |
virtual void | SetPosDt (const ChVector3< Real > &vel) |
Set the linear velocity. | |
virtual void | SetLinVel (const ChVector3< Real > &vel) |
Set the linear velocity. | |
virtual void | SetRotDt (const ChQuaternion< Real > &q_dt) |
Set the rotation velocity as a quaternion derivative. More... | |
virtual void | SetAngVelLocal (const ChVector3< Real > &w) |
Set the rotation velocity from the given angular velocity (expressed in local coordinates). | |
virtual void | SetAngVelParent (const ChVector3< Real > &w) |
Set the rotation velocity from given angular velocity (expressed in parent coordinates). | |
virtual void | SetCoordsysDt2 (const ChCoordsys< Real > &csys_dtdt) |
Set the linear and rotation accelerations as a single ChCoordsys derivative. | |
virtual void | SetPosDt2 (const ChVector3< Real > &acc) |
Set the linear acceleration. | |
virtual void | SetLinAcc (const ChVector3< Real > &acc) |
Set the linear acceleration. | |
virtual void | SetRotDt2 (const ChQuaternion< Real > &q_dtdt) |
Set the rotation acceleration as a quaternion derivative. More... | |
virtual void | SetAngAccLocal (const ChVector3< Real > &a) |
Set the rotation acceleration from given angular acceleration (expressed in local coordinates). More... | |
virtual void | SetAngAccParent (const ChVector3< Real > &a) |
Set the rotation acceleration from given angular acceleration (expressed in parent coordinates). | |
void | ComputeRotMatDt (ChMatrix33< Real > &R_dt) const |
Compute the time derivative of the rotation matrix. | |
void | ComputeRotMatDt2 (ChMatrix33< Real > &R_dtdt) |
Compute the second time derivative of the rotation matrix. | |
ChMatrix33< Real > | GetRotMatDt () |
Return the time derivative of the rotation matrix. | |
ChMatrix33< Real > | GetRotMatDt2 () |
Return the second time derivative of the rotation matrix. | |
void | ConcatenatePreTransformation (const ChFrameMoving< Real > &F) |
Apply a transformation (rotation and translation) represented by another frame. More... | |
void | ConcatenatePostTransformation (const ChFrameMoving< Real > &F) |
Apply a transformation (rotation and translation) represented by another frame F in local coordinate. More... | |
ChVector3< Real > | PointSpeedLocalToParent (const ChVector3< Real > &localpos) const |
Return the velocity in the parent frame of a point fixed to this frame and expressed in local coordinates. | |
ChVector3< Real > | PointSpeedLocalToParent (const ChVector3< Real > &localpos, const ChVector3< Real > &localspeed) const |
Return the velocity in the parent frame of a moving point, given the point location and velocity expressed in local coordinates. | |
ChVector3< Real > | PointAccelerationLocalToParent (const ChVector3< Real > &localpos) const |
Return the acceleration in the parent frame of a point fixed to this frame and expressed in local coordinates. More... | |
ChVector3< Real > | PointAccelerationLocalToParent (const ChVector3< Real > &localpos, const ChVector3< Real > &localspeed, const ChVector3< Real > &localacc) const |
Return the acceleration in the parent frame of a moving point, given the point location, velocity, and acceleration expressed in local coordinates. | |
ChVector3< Real > | PointSpeedParentToLocal (const ChVector3< Real > &parentpos, const ChVector3< Real > &parentspeed) const |
Return the velocity of a point expressed in this frame, given the point location and velocity in the parent frame. | |
ChVector3< Real > | PointAccelerationParentToLocal (const ChVector3< Real > &parentpos, const ChVector3< Real > &parentspeed, const ChVector3< Real > &parentacc) const |
Return the acceleration of a point expressed in this frame, given the point location, velocity, and acceleration in the parent frame. | |
ChFrameMoving< Real > | TransformLocalToParent (const ChFrameMoving< Real > &F) const |
Transform a moving frame from 'this' local coordinate system to parent frame coordinate system. | |
ChFrameMoving< Real > | TransformParentToLocal (const ChFrameMoving< Real > &F) const |
Transform a moving frame from the parent coordinate system to 'this' local frame coordinate system. | |
bool | Equals (const ChFrameMoving< Real > &other) const |
Returns true if this transform is identical to the other transform. | |
bool | Equals (const ChFrameMoving< Real > &other, Real tol) const |
Returns true if this transform is equal to the other transform, within a tolerance 'tol'. | |
virtual void | Invert () override |
Invert in place. More... | |
ChFrameMoving< Real > | GetInverse () const |
Return the inverse transform. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChFrame () | |
Default constructor (identity frame). | |
ChFrame (const ChVector3< Real > &v, const ChQuaternion< Real > &q=ChQuaternion< Real >(1, 0, 0, 0)) | |
Construct from position and rotation (as quaternion). | |
ChFrame (const ChVector3< Real > &v, const ChMatrix33< Real > &R) | |
Construct from pos and rotation (as a 3x3 matrix). | |
ChFrame (const ChVector3< Real > &v, const Real angle, const ChVector3< Real > &u) | |
Construct from position mv and rotation of angle alpha around unit vector mu. | |
ChFrame (const ChCoordsys< Real > &C) | |
Construct from a coordsys. | |
ChFrame (const ChFrame< Real > &other) | |
Copy constructor, build from another frame. | |
ChFrame< Real > & | operator= (const ChFrame< Real > &other) |
Assignment operator: copy from another frame. | |
bool | operator== (const ChFrame< Real > &other) const |
Returns true for identical frames. | |
bool | operator!= (const ChFrame< Real > &other) const |
Returns true for different frames. | |
ChFrame< Real > | operator* (const ChFrame< Real > &F) const |
Transform another frame through this frame. More... | |
ChFrame< Real > | operator>> (const ChFrame< Real > &F) const |
Transform another frame through this frame. More... | |
ChVector3< Real > | operator* (const ChVector3< Real > &v) const |
Transform a vector through this frame (express in parent frame). More... | |
ChVector3< Real > | operator/ (const ChVector3< Real > &v) const |
Transform a vector through this frame (express from parent frame). More... | |
ChFrame< Real > & | operator>>= (const ChFrame< Real > &F) |
Transform this frame by pre-multiplication with another frame. More... | |
ChFrame< Real > & | operator*= (const ChFrame< Real > &F) |
Transform this frame by post-multiplication with another frame. More... | |
ChFrame< Real > & | operator>>= (const ChVector3< Real > &v) |
Transform this frame by pre-multiplication with a given vector (translate frame). | |
ChFrame< Real > & | operator>>= (const ChQuaternion< Real > &q) |
Transform this frame by pre-multiplication with a given quaternion (rotate frame). | |
ChFrame< Real > & | operator>>= (const ChCoordsys< Real > &C) |
Transform this frame by pre-multiplication with a given coordinate system. | |
const ChCoordsys< Real > & | GetCoordsys () const |
Return both current rotation and translation as a ChCoordsys object. | |
const ChVector3< Real > & | GetPos () const |
Return the current translation vector. | |
const ChQuaternion< Real > & | GetRot () const |
Return the current rotation quaternion. | |
const ChMatrix33< Real > & | GetRotMat () const |
Return the current 3x3 rotation matrix. | |
ChVector3< Real > | GetRotAxis () const |
Get axis of finite rotation, in parent space. | |
Real | GetRotAngle () const |
Get angle of rotation about axis of finite rotation. | |
void | SetCoordsys (const ChCoordsys< Real > &C) |
Impose both translation and rotation as a single ChCoordsys. More... | |
void | SetCoordsys (const ChVector3< Real > &v, const ChQuaternion< Real > &q) |
Impose both translation and rotation. More... | |
void | SetRot (const ChQuaternion< Real > &q) |
Impose the rotation as a quaternion. More... | |
void | SetRot (const ChMatrix33< Real > &R) |
Impose the rotation as a 3x3 matrix. More... | |
void | SetPos (const ChVector3< Real > &pos) |
Impose the translation vector. | |
void | ConcatenatePreTransformation (const ChFrame< Real > &F) |
Apply a transformation (rotation and translation) represented by another frame. More... | |
void | ConcatenatePostTransformation (const ChFrame< Real > &F) |
Apply a transformation (rotation and translation) represented by another frame F in local coordinate. More... | |
void | Move (const ChVector3< Real > &v) |
An easy way to move the frame by the amount specified by vector v, (assuming v expressed in parent coordinates) | |
void | Move (const ChCoordsys< Real > &C) |
Apply both translation and rotation, assuming both expressed in parent coordinates, as a vector for translation and quaternion for rotation,. | |
ChVector3< Real > | TransformPointLocalToParent (const ChVector3< Real > &v) const |
Transform a point from the local frame coordinate system to the parent coordinate system. | |
ChVector3< Real > | TransformPointParentToLocal (const ChVector3< Real > &v) const |
Transforms a point from the parent coordinate system to local frame coordinate system. | |
ChVector3< Real > | TransformDirectionLocalToParent (const ChVector3< Real > &d) const |
Transform a direction from the parent frame coordinate system to 'this' local coordinate system. | |
ChVector3< Real > | TransformDirectionParentToLocal (const ChVector3< Real > &d) const |
Transforms a direction from 'this' local coordinate system to parent frame coordinate system. | |
ChWrench< Real > | TransformWrenchLocalToParent (const ChWrench< Real > &w) const |
Transform a wrench from the local coordinate system to the parent coordinate system. | |
ChWrench< Real > | TransformWrenchParentToLocal (const ChWrench< Real > &w) const |
Transform a wrench from the parent coordinate system to the local coordinate system. | |
ChFrame< Real > | TransformLocalToParent (const ChFrame< Real > &F) const |
Transform a frame from 'this' local coordinate system to parent frame coordinate system. | |
ChFrame< Real > | TransformParentToLocal (const ChFrame< Real > &F) const |
Transform a frame from the parent coordinate system to 'this' local frame coordinate system. | |
bool | Equals (const ChFrame< Real > &other) const |
Returns true if this transform is identical to the other transform. | |
bool | Equals (const ChFrame< Real > &other, Real tol) const |
Returns true if this transform is equal to the other transform, within a tolerance 'tol'. | |
void | Normalize () |
Normalize the rotation, so that quaternion has unit length. | |
virtual void | SetIdentity () |
Sets to no translation and no rotation. | |
ChFrame< Real > | GetInverse () const |
Return the inverse transform. | |
Protected Attributes | |
ChCoordsys< Real > | m_csys_dt |
rotation and position velocity, as vector + quaternion | |
ChCoordsys< Real > | m_csys_dtdt |
rotation and position acceleration, as vector + quaternion | |
![]() | |
ChCoordsys< Real > | m_csys |
position and rotation, as vector + quaternion | |
ChMatrix33< Real > | m_rmat |
3x3 orthogonal rotation matrix | |
Friends | |
class | chrono::fmi2::FmuChronoComponentBase |
class | chrono::fmi3::FmuChronoComponentBase |
Member Function Documentation
◆ ConcatenatePostTransformation()
|
inline |
Apply a transformation (rotation and translation) represented by another frame F in local coordinate.
This is equivalent to post-multiply this frame by the other frame F: this'= this * F or this'= F >> this
◆ ConcatenatePreTransformation()
|
inline |
Apply a transformation (rotation and translation) represented by another frame.
This is equivalent to pre-multiply this frame by the other frame F: this'= F * this or this' = this >> F
◆ Invert()
|
inlineoverridevirtual |
Invert in place.
If w=A*v, after A.Invert() we have v=A*w;
Reimplemented from chrono::ChFrame< Real >.
◆ operator*()
|
inline |
Transform another frame through this frame.
If A is this frame and F another frame expressed in A, then G = A * F is the frame F expresssed in the parent frame of A. For a sequence of transformations, i.e. a chain of coordinate systems, one can also write: G = F_1to0 * F_2to1 * F_3to2 * F; i.e., just like done with a sequence of Denavitt-Hartemberg matrix multiplications. This operation is not commutative. Velocities and accelerations are also transformed.
◆ operator*=()
|
inline |
Transform this frame by post-multiplication with another frame.
If A is this frame, then A *= F means A' = A * F or A' = F >> A.
◆ operator>>()
|
inline |
Transform another frame through this frame.
If A is this frame and F another frame expressed in A, then G = F >> A is the frame F expresssed in the parent frame of A. For a sequence of transformations, i.e. a chain of coordinate systems, one can also write: G = F >> F_3to2 >> F_2to1 >> F_1to0; i.e., just like done with a sequence of Denavitt-Hartemberg matrix multiplications (but reverting order). This operation is not commutative. Velocities and accelerations are also transformed.
◆ operator>>=()
|
inline |
Transform this frame by pre-multiplication with another frame.
If A is this frame, then A >>= F means A' = F * A or A' = A >> F.
◆ PointAccelerationLocalToParent()
|
inline |
Return the acceleration in the parent frame of a point fixed to this frame and expressed in local coordinates.
Note:
- the first and second derivatives of pos and rot are assumed to have been assigned.
- when the local angular acceleration is zero, it's still necessary to call SetAngAccLocal(VNULL) because q_dtdt may be nonzero due to nonzero q_dt in case of rotational motion.
◆ SetAngAccLocal()
|
inlinevirtual |
Set the rotation acceleration from given angular acceleration (expressed in local coordinates).
Note: even when the local angular acceleration is zero, this function should still be called because q_dtdt might be nonzero due to nonzero q_dt (in case of rotational motion).
◆ SetRotDt()
|
inlinevirtual |
Set the rotation velocity as a quaternion derivative.
Note: the quaternion must satisfy: dot(q,q_dt)=0.
◆ SetRotDt2()
|
inlinevirtual |
Set the rotation acceleration as a quaternion derivative.
Note: the quaternion must satisfy: dot(q,q_dtdt)+dot(q_dt,q_dt)=0.
The documentation for this class was generated from the following file:
- /builds/uwsbel/chrono/src/chrono/core/ChFrameMoving.h