chrono::vehicle::feda::FEDA Class Reference
Description
Definition of the FED-alpha assembly.
This class encapsulates a concrete wheeled vehicle model with parameters corresponding to a FED-alpha, the powertrain model, and the 4 tires. It provides wrappers to access the different systems and subsystems, functions for specifying the driveline, powertrain, and tire types, as well as functions for controlling the visualization mode of each component.
#include <FEDA.h>
Collaboration diagram for chrono::vehicle::feda::FEDA:
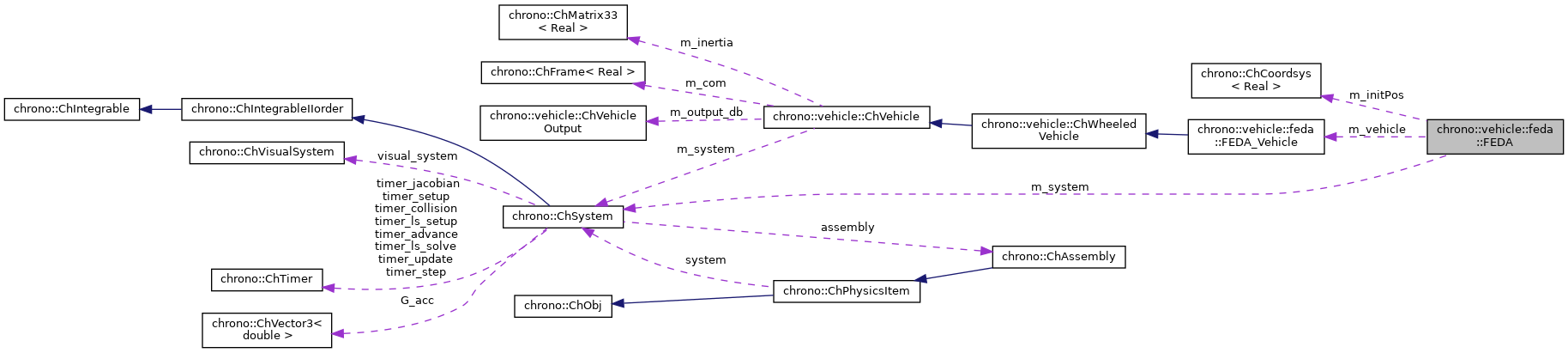
Public Types | |
enum | DamperMode { FSD, PASSIVE_LOW, PASSIVE_HIGH } |
Public Member Functions | |
FEDA (ChSystem *system) | |
void | SetContactMethod (ChContactMethod val) |
void | SetCollisionSystemType (ChCollisionSystem::Type collsys_type) |
void | SetChassisFixed (bool val) |
void | SetChassisCollisionType (CollisionType val) |
void | SetBrakeType (BrakeType brake_type) |
void | SetEngineType (EngineModelType val) |
void | SetTransmissionType (TransmissionModelType val) |
void | SetTireType (TireModelType val) |
void | SetTireCollisionType (ChTire::CollisionType collType) |
void | SetTirePressure (double pressure) |
void | SetInitPosition (const ChCoordsys<> &pos) |
void | SetInitFwdVel (double fwdVel) |
void | SetInitWheelAngVel (const std::vector< double > &omega) |
void | SetTireStepSize (double step_size) |
void | SetRideHeight_Low () |
void | SetRideHeight_OnRoad () |
void | SetRideHeight_ObstacleCrossing () |
void | SetDamperMode (DamperMode theDamperMode=DamperMode::FSD) |
ChSystem * | GetSystem () const |
ChWheeledVehicle & | GetVehicle () const |
std::shared_ptr< ChChassis > | GetChassis () const |
std::shared_ptr< ChBodyAuxRef > | GetChassisBody () const |
std::shared_ptr< ChTransmission > | GetTransmission () const |
void | Initialize () |
void | LockAxleDifferential (int axle, bool lock) |
void | SetAerodynamicDrag (double Cd, double area, double air_density) |
void | SetChassisVisualizationType (VisualizationType vis) |
void | SetSuspensionVisualizationType (VisualizationType vis) |
void | SetSteeringVisualizationType (VisualizationType vis) |
void | SetWheelVisualizationType (VisualizationType vis) |
void | SetTireVisualizationType (VisualizationType vis) |
void | Synchronize (double time, const DriverInputs &driver_inputs, const ChTerrain &terrain) |
void | Advance (double step) |
void | LogHardpointLocations () |
void | DebugLog (int what) |
Protected Attributes | |
ChContactMethod | m_contactMethod |
ChCollisionSystem::Type | m_collsysType |
CollisionType | m_chassisCollisionType |
bool | m_fixed |
TireModelType | m_tireType |
BrakeType | m_brake_type |
EngineModelType | m_engineType |
TransmissionModelType | m_transmissionType |
double | m_tire_step_size |
ChCoordsys | m_initPos |
double | m_initFwdVel |
std::vector< double > | m_initOmega |
bool | m_apply_drag |
double | m_Cd |
double | m_area |
double | m_air_density |
ChSystem * | m_system |
FEDA_Vehicle * | m_vehicle |
double | m_tire_mass |
double | m_tire_pressure |
int | m_ride_height_config |
int | m_damper_mode |
ChTire::CollisionType | m_tire_collision_type |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_models/vehicle/feda/FEDA.h
- /builds/uwsbel/chrono/src/chrono_models/vehicle/feda/FEDA.cpp