Description
Base class for all tire nodes.
#include <ChVehicleCosimTireNode.h>
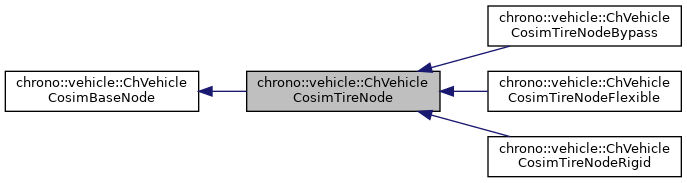
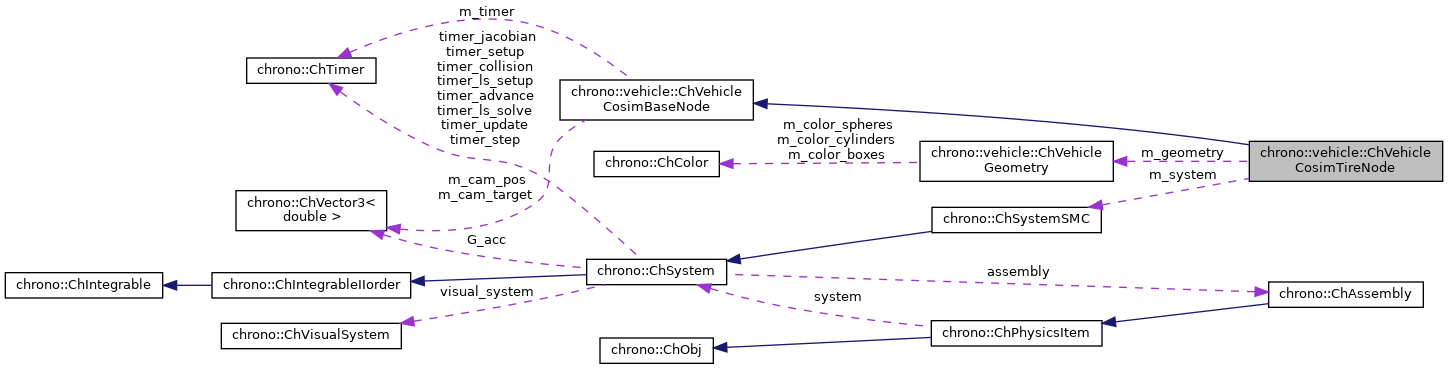
Public Types | |
enum | TireType { TireType::RIGID, TireType::FLEXIBLE, TireType::BYPASS, TireType::UNKNOWN } |
Tire type. More... | |
![]() | |
enum | NodeType { NodeType::MBS_WHEELED, NodeType::MBS_TRACKED, NodeType::TERRAIN, NodeType::TIRE } |
Type of node participating in co-simulation. More... | |
enum | InterfaceType { InterfaceType::BODY, InterfaceType::MESH } |
Type of the vehicle-terrain communication interface. More... | |
Public Member Functions | |
virtual NodeType | GetNodeType () const override final |
Return the node type as NodeType::TIRE. | |
virtual TireType | GetTireType () const =0 |
Return the tire type. | |
void | EnableTirePressure (bool val) |
Enable/disable tire pressure (default: true). | |
ChSystem & | GetSystem () |
Get a reference to the underlying Chrono system. More... | |
virtual void | Initialize () override final |
Initialize this node. More... | |
virtual void | Synchronize (int step_number, double time) override final |
Synchronize this node. More... | |
virtual void | OutputData (int frame) override final |
Output logging and debugging data. | |
![]() | |
std::string | GetNodeTypeString () const |
Return the node type as a string. | |
bool | IsCosimNode () const |
Return true if this node is part of the co-simulation infrastructure. | |
void | SetStepSize (double step) |
Set the integration step size (default: 1e-4). | |
double | GetStepSize () const |
Get the integration step size. | |
void | SetOutDir (const std::string &dir_name, const std::string &suffix="") |
Set the name of the output directory and an identifying suffix. More... | |
void | SetVerbose (bool verbose) |
Enable/disable verbose messages during simulation (default: true). | |
void | EnableRuntimeVisualization (double render_fps=100, bool save_img=false) |
Enable run-time visualization (default: false). More... | |
void | SetCameraPosition (const ChVector3d &cam_pos, const ChVector3d &cam_target=VNULL) |
Set camera location and target point. | |
void | SetCameraTracking (bool track) |
Enable/disable tracking of objects (default: true). | |
void | EnablePostprocessVisualization (double render_fps=100) |
Enable Blender postprocessing (default: false). More... | |
const std::string & | GetOutDirName () const |
Get the output directory name for this node. | |
double | GetStepExecutionTime () const |
Get the simulation execution time for the current step on this node. More... | |
double | GetTotalExecutionTime () const |
Get the cumulative simulation execution time on this node. | |
virtual void | OnRender () |
Render the current simulation frame. More... | |
virtual void | Advance (double step_size)=0 |
Advance simulation. More... | |
void | Render (double step_size) |
Render simulation frame. More... | |
virtual void | OutputVisualizationData (int frame)=0 |
Output post-processing visualization data. More... | |
virtual void | WriteCheckpoint (const std::string &filename) const |
Write checkpoint to the specified file (which will be created in the output directory). | |
Static Public Member Functions | |
static std::string | GetTireTypeAsString (TireType type) |
Return a string describing the tire type. | |
static TireType | GetTireTypeFromString (const std::string &type) |
Infer the tire type from the given string. | |
static bool | ReadSpecfile (const std::string &specfile, rapidjson::Document &d) |
Read a JSON specification file for a tire. | |
static TireType | GetTireTypeFromSpecfile (const std::string &specfile) |
Get the tire type from the given JSON specification file. | |
![]() | |
static std::string | OutputFilename (const std::string &dir, const std::string &root, const std::string &ext, int frame, int frame_digits) |
Utility function for creating an output file name. More... | |
Protected Member Functions | |
ChVehicleCosimTireNode (int index, const std::string &tire_json="") | |
Construct a base class tire co-simulation node. More... | |
virtual InterfaceType | GetInterfaceType () const =0 |
Specify the type of communication interface (BODY or MESH) required by this the tire node. More... | |
virtual double | GetTireMass () const |
Return the tire mass. | |
virtual double | GetTireRadius () const |
Return the tire radius. | |
virtual double | GetTireWidth () const |
Return the tire width. | |
virtual void | InitializeTire (std::shared_ptr< ChWheel >, const ChVector3d &init_loc)=0 |
Initialize the tire by attaching it to the provided ChWheel. More... | |
virtual void | ApplySpindleState (const BodyState &spindle_state) |
Apply the spindle state. More... | |
virtual void | LoadSpindleForce (TerrainForce &spindle_force) |
Load current spindle force. More... | |
virtual void | ApplySpindleForce (const TerrainForce &spindle_force) |
Apply the spindle force (BODY communication interface). More... | |
virtual void | LoadMeshState (MeshState &mesh_state) |
Load current tire mesh state. More... | |
virtual void | ApplyMeshForces (const MeshContact &mesh_contact) |
Apply the mesh contact forces. More... | |
virtual void | OnOutputData (int frame) |
Perform additional output at the specified frame (called once per integration step). | |
![]() | |
ChVehicleCosimBaseNode (const std::string &name) | |
void | SendGeometry (const utils::ChBodyGeometry &geom, int dest) const |
Utility function to pack and send a struct with geometry information. | |
void | RecvGeometry (utils::ChBodyGeometry &geom, int source) const |
Utility function to receive and unpack a struct with geometry information. | |
void | ProgressBar (unsigned int x, unsigned int n, unsigned int w=50) |
Utility function to display a progress bar to the terminal. More... | |
Protected Attributes | |
ChSystemSMC * | m_system |
containing system | |
bool | m_tire_pressure |
tire pressure enabled? | |
int | m_index |
index of the tire | |
std::shared_ptr< ChBody > | m_spindle |
spindle body | |
std::shared_ptr< ChWheel > | m_wheel |
wheel subsystem (to which a tire is attached) | |
std::shared_ptr< ChTire > | m_tire |
tire subsystem | |
utils::ChBodyGeometry | m_geometry |
tire geometry and contact material | |
![]() | |
int | m_rank |
MPI rank of this node (in MPI_COMM_WORLD) | |
double | m_step_size |
integration step size | |
std::string | m_name |
name of the node | |
std::string | m_out_dir |
top-level output directory | |
std::string | m_node_out_dir |
node-specific output directory | |
std::ofstream | m_outf |
output file stream | |
bool | m_renderRT |
if true, perform run-time rendering | |
bool | m_renderRT_all |
if true, render all frames | |
double | m_renderRT_step |
time step between rendered frames | |
bool | m_writeRT |
if true, write images to file | |
bool | m_renderPP |
if true, save data for post-processing | |
bool | m_renderPP_all |
if true, save data at all frames | |
double | m_renderPP_step |
time step between post-processing save frames | |
bool | m_track |
track objects | |
ChVector3d | m_cam_pos |
camera location | |
ChVector3d | m_cam_target |
camera target (lookat) point | |
unsigned int | m_num_wheeled_mbs_nodes |
unsigned int | m_num_tracked_mbs_nodes |
unsigned int | m_num_terrain_nodes |
unsigned int | m_num_tire_nodes |
ChTimer | m_timer |
timer for integration cost | |
double | m_cum_sim_time |
cumulative integration cost | |
bool | m_verbose |
verbose messages during simulation? | |
Additional Inherited Members | |
![]() | |
static const double | m_gacc = -9.81 |
Member Enumeration Documentation
◆ TireType
Constructor & Destructor Documentation
◆ ChVehicleCosimTireNode()
|
protected |
Construct a base class tire co-simulation node.
By default, the underlying Chrono system is set yo use the Barzilai-Borwein solver and the Euler implicit linearized integrator. All OpenMP thread numbers are set to 1.
Member Function Documentation
◆ ApplyMeshForces()
|
inlineprotectedvirtual |
Apply the mesh contact forces.
A derived class which implements the MESH communication interface must override this function and must use the MeshContact struct received from the TERRAIN node.
◆ ApplySpindleForce()
|
inlineprotectedvirtual |
Apply the spindle force (BODY communication interface).
The TerrainForce struct contains the terrain forces applied to the spindle as received from the TERRAIN node.
◆ ApplySpindleState()
|
inlineprotectedvirtual |
Apply the spindle state.
The BodyState struct contains the spindle body state as received from the MBS node.
◆ GetInterfaceType()
|
protectedpure virtual |
Specify the type of communication interface (BODY or MESH) required by this the tire node.
◆ GetSystem()
|
inline |
Get a reference to the underlying Chrono system.
This can be used to change simulation settings (solver, integrator, number of threads, etc.).
◆ Initialize()
|
finaloverridevirtual |
Initialize this node.
This function allows the node to initialize itself and, optionally, perform an initial data exchange with any other node.
Reimplemented from chrono::vehicle::ChVehicleCosimBaseNode.
◆ InitializeTire()
|
protectedpure virtual |
Initialize the tire by attaching it to the provided ChWheel.
A derived class must load m_geometry (collision shape and contact material).
◆ LoadMeshState()
|
inlineprotectedvirtual |
Load current tire mesh state.
A derived class which implements the MESH communication interface must override this function and must load the provided MeshState struct to be sent to the TERRAIN node.
◆ LoadSpindleForce()
|
inlineprotectedvirtual |
Load current spindle force.
A derived class which implements the MESH communication interface must override this function and must load the provided TerrainForce struct to be sent to the MBS node.
◆ Synchronize()
|
finaloverridevirtual |
Synchronize this node.
This function is called at every co-simulation synchronization time to allow the node to exchange information with any other node.
Implements chrono::vehicle::ChVehicleCosimBaseNode.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/cosim/ChVehicleCosimTireNode.h
- /builds/uwsbel/chrono/src/chrono_vehicle/cosim/ChVehicleCosimTireNode.cpp