Description
Base class for all Chrono solvers (for linear problems or complementarity problems).
See ChSystemDescriptor for more information about the problem formulation and the data structures passed to the solver.
#include <ChSolver.h>
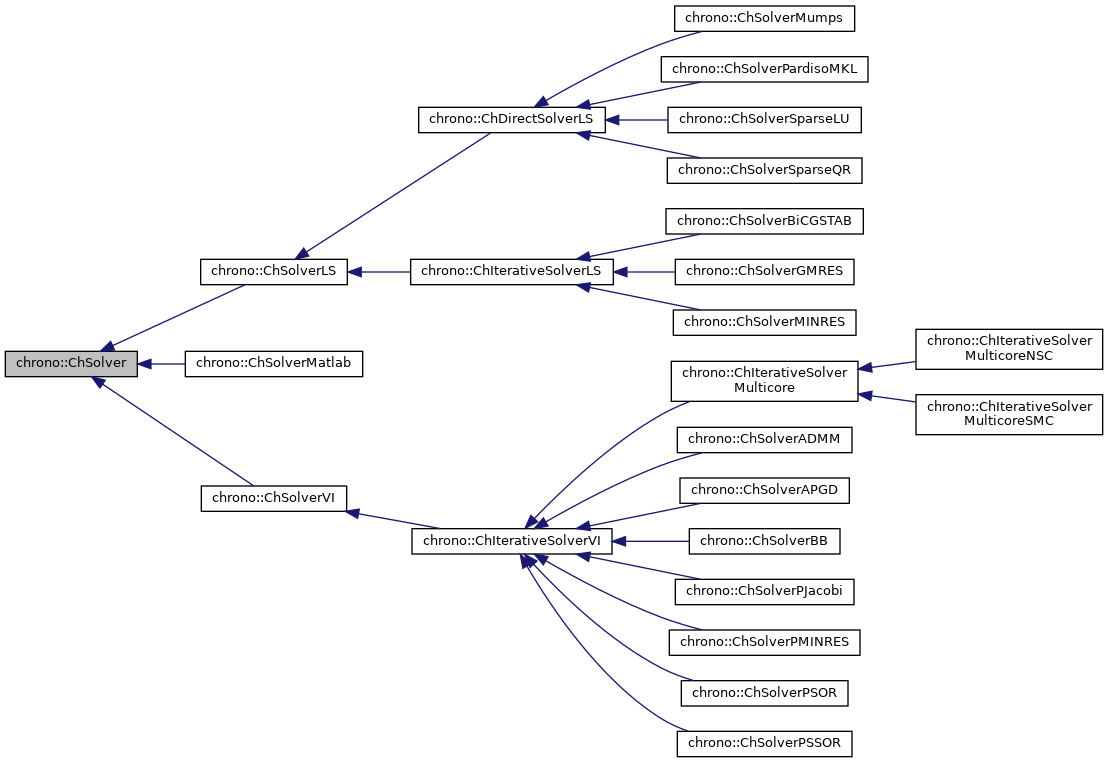
Public Types | |
enum | Type { Type::PSOR, Type::PSSOR, Type::PJACOBI, Type::PMINRES, Type::BARZILAIBORWEIN, Type::APGD, Type::ADMM, Type::SPARSE_LU, Type::SPARSE_QR, Type::PARDISO_MKL, Type::MUMPS, Type::GMRES, Type::MINRES, Type::BICGSTAB, CUSTOM } |
Available types of solvers. More... | |
Public Member Functions | |
virtual Type | GetType () const |
Return type of the solver. | |
virtual bool | IsIterative () const =0 |
Return true if iterative solver. | |
virtual bool | IsDirect () const =0 |
Return true if direct solver. | |
virtual ChIterativeSolver * | AsIterative () |
Downcast to ChIterativeSolver. | |
virtual ChDirectSolverLS * | AsDirect () |
Downcast to ChDirectSolver. | |
virtual bool | SolveRequiresMatrix () const =0 |
Indicate whether or not the Solve() phase requires an up-to-date problem matrix. More... | |
virtual double | Solve (ChSystemDescriptor &sysd)=0 |
Performs the solution of the problem. More... | |
virtual bool | Setup (ChSystemDescriptor &sysd) |
This function does the setup operations for the solver. More... | |
void | SetVerbose (bool mv) |
Set verbose output from solver. | |
void | EnableWrite (bool val, const std::string &frame, const std::string &out_dir=".") |
Enable/disable debug output of matrix, RHS, and solution vector. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) |
Method to allow de-serialization of transient data from archives. | |
Static Public Member Functions | |
static std::string | GetTypeAsString (Type type) |
Return the solver type as a string. | |
Protected Attributes | |
bool | verbose |
bool | write_matrix |
std::string | output_dir |
std::string | frame_id |
Member Enumeration Documentation
◆ Type
|
strong |
Available types of solvers.
Member Function Documentation
◆ Setup()
|
inlinevirtual |
This function does the setup operations for the solver.
The purpose of this function is to prepare the solver for subsequent calls to the solve function. The system descriptor contains the constraints and variables. This function is called only as frequently it is determined that it is appropriate to perform the setup phase.
Reimplemented in chrono::ChDirectSolverLS, and chrono::ChIterativeSolverLS.
◆ Solve()
|
pure virtual |
Performs the solution of the problem.
This function MUST be implemented in children classes, with specialized methods such as iterative or direct solvers. The system descriptor contains the constraints and variables. Returns true if it successfully solves the problem and false otherwise.
Implemented in chrono::ChDirectSolverLS, chrono::ChIterativeSolverLS, chrono::ChSolverPMINRES, chrono::ChSolverADMM, chrono::ChSolverPSSOR, chrono::ChSolverMatlab, chrono::ChIterativeSolverMulticore, chrono::ChSolverBB, chrono::ChSolverPJacobi, chrono::ChSolverPSOR, and chrono::ChSolverAPGD.
◆ SolveRequiresMatrix()
|
pure virtual |
Indicate whether or not the Solve() phase requires an up-to-date problem matrix.
Typically, direct solvers only need the matrix for the Setup() phase. However, iterative solvers likely require the matrix to perform the necessary matrix-vector operations.
Implemented in chrono::ChIterativeSolverLS, chrono::ChDirectSolverLS, chrono::ChIterativeSolverVI, and chrono::ChSolverMatlab.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChSolver.h
- /builds/uwsbel/chrono/src/chrono/solver/ChSolver.cpp