chrono::vehicle::marder::Marder_Vehicle Class Reference
Description
Definition of an Marder tracked vehicle with segmented tracks.
Both single-pin and double-pin track assemblies can be used with this vehicle model.
#include <Marder_Vehicle.h>
Inheritance diagram for chrono::vehicle::marder::Marder_Vehicle:
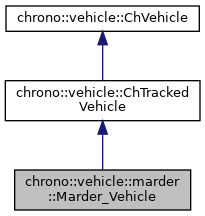
Collaboration diagram for chrono::vehicle::marder::Marder_Vehicle:
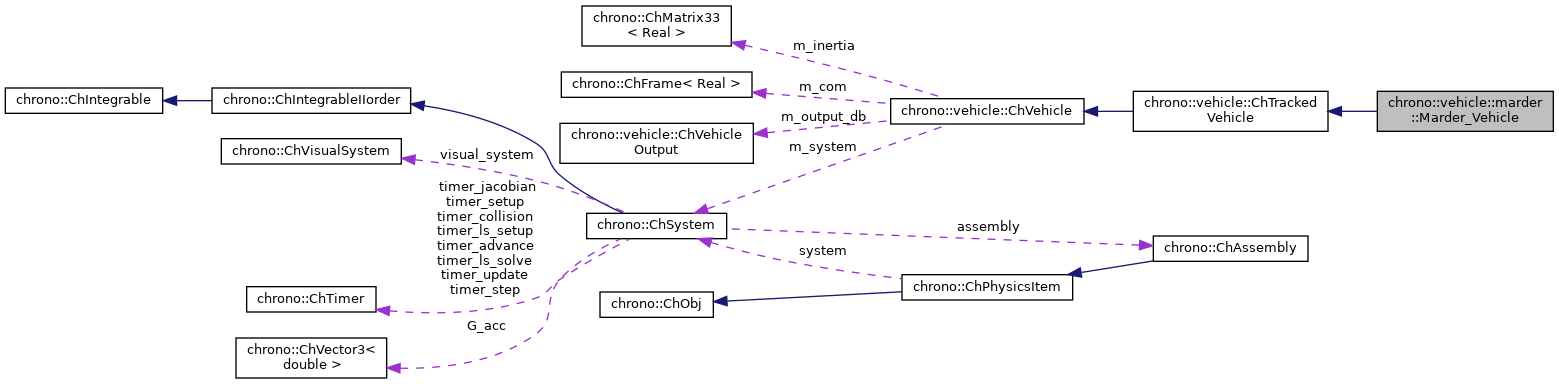
Public Member Functions | |
Marder_Vehicle (bool fixed, TrackShoeType shoe_type, DrivelineTypeTV driveline_type, BrakeType brake_type, ChContactMethod contact_method=ChContactMethod::NSC, CollisionType chassis_collision_type=CollisionType::NONE) | |
Construct the M113 vehicle within an automatically created Chrono system. | |
Marder_Vehicle (bool fixed, TrackShoeType shoe_type, DrivelineTypeTV driveline_type, BrakeType brake_type, ChSystem *system, CollisionType chassis_collision_type=CollisionType::NONE) | |
Construct the M113 vehicle within the specified Chrono system. | |
void | CreateTrack (bool val) |
Create the track shoes (default: true). | |
virtual void | Initialize (const ChCoordsys<> &chassisPos, double chassisFwdVel=0) override |
Initialize the M113 vehicle at the specified location and orientation. | |
![]() | |
virtual | ~ChTrackedVehicle () |
Destructor. | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle system template. | |
std::shared_ptr< ChTrackAssembly > | GetTrackAssembly (VehicleSide side) const |
Get the specified suspension subsystem. | |
std::shared_ptr< ChDrivelineTV > | GetDriveline () const |
Get a handle to the vehicle's driveline subsystem. | |
size_t | GetNumTrackSuspensions (VehicleSide side) const |
Get the number of suspensions in the specified track assembly. | |
size_t | GetNumTrackShoes (VehicleSide side) const |
Get the number of shoes in the specified track assembly. | |
std::shared_ptr< ChTrackShoe > | GetTrackShoe (VehicleSide side, size_t id) const |
Get a handle to the specified track shoe. | |
BodyState | GetTrackShoeState (VehicleSide side, size_t shoe_id) const |
Get the complete state for the specified track shoe. More... | |
void | GetTrackShoeStates (VehicleSide side, BodyStates &states) const |
Get the complete states for all track shoes of the specified track assembly. More... | |
void | SetSprocketVisualizationType (VisualizationType vis) |
Set visualization type for the sprocket subsystem. | |
void | SetIdlerVisualizationType (VisualizationType vis) |
Set visualization type for the idler subsystem. | |
void | SetSuspensionVisualizationType (VisualizationType vis) |
Set visualization type for the suspension subsystems. | |
void | SetIdlerWheelVisualizationType (VisualizationType vis) |
Set visualization type for the idler wheel subsystem. | |
void | SetRoadWheelVisualizationType (VisualizationType vis) |
Set visualization type for the road-wheel subsystems. | |
void | SetRollerVisualizationType (VisualizationType vis) |
Set visualization type for the roller subsystems. | |
void | SetTrackShoeVisualizationType (VisualizationType vis) |
Set visualization type for the track shoe subsystems. | |
void | SetSprocketCollide (bool state) |
Enable/disable collision for the sprocket subsystem. | |
void | SetIdlerCollide (bool state) |
Enable/disable collision for the idler subsystem. | |
void | SetRoadWheelCollide (bool state) |
Enable/disable collision for the road-wheel subsystems. | |
void | SetRollerCollide (bool state) |
Enable/disable collision for the roller subsystems. | |
void | SetTrackShoeCollide (bool state) |
Enable/disable collision for the track shoe subsystems. | |
void | EnableCollision (int flags) |
Set collision flags for the various subsystems. More... | |
virtual void | SetChassisVehicleCollide (bool state) override |
Enable/disable collision between the chassis and all other vehicle subsystems. More... | |
void | EnableCustomContact (std::shared_ptr< ChTrackCustomContact > callback) |
Enable user-defined contact forces between track shoes and idlers and/or road-wheels and/or ground. More... | |
void | MonitorContacts (int flags) |
Set contacts to be monitored. More... | |
void | SetRenderContactNormals (bool val) |
Render normals of all monitored contacts. | |
void | SetRenderContactForces (bool val, double scale) |
Render forces of all monitored contacts. | |
void | SetContactCollection (bool val) |
Turn on/off contact data collection. More... | |
bool | IsPartInContact (TrackedCollisionFlag::Enum part) const |
Return true if the specified vehicle part is currently experiencing a collision. | |
ChVector3d | GetSprocketResistiveTorque (VehicleSide side) const |
Return estimated resistive torque on the specified sprocket. More... | |
void | WriteContacts (const std::string &filename) |
Write contact information to file. More... | |
void | SetTrackAssemblyOutput (VehicleSide side, bool state) |
Enable/disable output for the track assemblies. More... | |
virtual void | InitializeInertiaProperties () override final |
Calculate total vehicle mass. More... | |
void | Synchronize (double time, const DriverInputs &driver_inputs) |
Update the state of this vehicle at the current time. More... | |
void | Synchronize (double time, const DriverInputs &driver_inputs, const TerrainForces &shoe_forces_left, const TerrainForces &shoe_forces_right) |
Update the state of this vehicle at the current time. More... | |
virtual void | Advance (double step) override final |
Advance the state of this vehicle by the specified time step. More... | |
void | LockDifferential (bool lock) |
Lock/unlock the differential (if available). | |
void | DisconnectDriveline () |
Disconnect driveline. More... | |
virtual void | LogConstraintViolations () override |
Log current constraint violations. | |
void | LogSubsystemTypes () |
Log the types (template names) of current vehicle subsystems. | |
virtual std::string | ExportComponentList () const override |
Return a JSON string with information on all modeling components in the vehicle system. More... | |
virtual void | ExportComponentList (const std::string &filename) const override |
Write a JSON-format file with information on all modeling components in the vehicle system. More... | |
![]() | |
virtual | ~ChVehicle () |
Destructor. | |
const std::string & | GetName () const |
Get the name identifier for this vehicle. | |
void | SetName (const std::string &name) |
Set the name identifier for this vehicle. | |
uint16_t | GetVehicleTag () const |
Get vehicle tag. More... | |
ChSystem * | GetSystem () |
Get a pointer to the Chrono ChSystem. | |
double | GetChTime () const |
Get the current simulation time of the underlying ChSystem. | |
std::shared_ptr< ChChassis > | GetChassis () const |
Get a handle to the vehicle's main chassis subsystem. | |
std::shared_ptr< ChChassisRear > | GetChassisRear (int id) const |
Get the specified specified rear chassis subsystem. | |
std::shared_ptr< ChChassisConnector > | GetChassisConnector (int id) const |
Get a handle to the specified chassis connector. | |
std::shared_ptr< ChBodyAuxRef > | GetChassisBody () const |
Get a handle to the vehicle's chassis body. | |
std::shared_ptr< ChBodyAuxRef > | GetChassisRearBody (int id) const |
Get a handle to the specified rear chassis body. | |
std::shared_ptr< ChPowertrainAssembly > | GetPowertrainAssembly () const |
Get the powertrain attached to this vehicle. | |
std::shared_ptr< ChEngine > | GetEngine () const |
Get the engine in the powertrain assembly (if a powertrain is attached). | |
std::shared_ptr< ChTransmission > | GetTransmission () const |
Get the transmission in the powertrain assembly (if a powertrain is attached). | |
double | GetMass () const |
Get the vehicle total mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current vehicle COM frame (relative to and expressed in the vehicle reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current vehicle inertia (relative to the vehicle COM frame). | |
const ChFrameMoving & | GetRefFrame () const |
Get the current vehicle reference frame. More... | |
const ChFrame & | GetTransform () const |
Get the current vehicle transform relative to the global frame. More... | |
const ChVector3d & | GetPos () const |
Get the vehicle global location. More... | |
ChQuaternion | GetRot () const |
Get the vehicle orientation. More... | |
double | GetRoll () const |
Get vehicle roll angle. More... | |
double | GetPitch () const |
Get vehicle pitch angle. More... | |
double | GetRoll (const ChTerrain &terrain) const |
Get vehicle roll angle (relative to local terrain). More... | |
double | GetPitch (const ChTerrain &terrain) const |
Get vehicle pitch angle (relative to local terrain). More... | |
double | GetSpeed () const |
Get the vehicle speed (velocity component in the vehicle forward direction). More... | |
double | GetSlipAngle () const |
Get the vehicle slip angle. More... | |
double | GetRollRate () const |
Get the vehicle roll rate. More... | |
double | GetPitchRate () const |
Get the vehicle pitch rate. More... | |
double | GetYawRate () const |
Get the vehicle yaw rate. More... | |
double | GetTurnRate () const |
Get the vehicle turn rate. More... | |
ChVector3d | GetPointLocation (const ChVector3d &locpos) const |
Get the global position of the specified point. More... | |
ChVector3d | GetPointVelocity (const ChVector3d &locpos) const |
Get the global velocity of the specified point. More... | |
ChVector3d | GetPointAcceleration (const ChVector3d &locpos) const |
Get the acceleration at the specified point. More... | |
ChVector3d | GetDriverPos () const |
Get the global location of the driver. | |
void | EnableRealtime (bool val) |
Enable/disable soft real-time (default: false). More... | |
double | GetRTF () const |
Get current estimated RTF (real time factor). More... | |
double | GetStepRTF () const |
Get current estimated step RTF (real time factor). More... | |
void | SetCollisionSystemType (ChCollisionSystem::Type collsys_type) |
Change the default collision detection system. More... | |
void | SetOutput (ChVehicleOutput::Type type, const std::string &out_dir, const std::string &out_name, double output_step) |
Enable output for this vehicle system. More... | |
void | SetOutput (ChVehicleOutput::Type type, std::ostream &out_stream, double output_step) |
Enable output for this vehicle system using an existing output stream. More... | |
void | InitializePowertrain (std::shared_ptr< ChPowertrainAssembly > powertrain) |
Initialize the given powertrain assembly and associate it to this vehicle. More... | |
void | SetChassisVisualizationType (VisualizationType vis) |
Set visualization mode for the chassis subsystem. | |
void | SetChassisRearVisualizationType (VisualizationType vis) |
Set visualization mode for the rear chassis subsystems. | |
void | SetChassisCollide (bool state) |
Enable/disable collision for the chassis subsystem. More... | |
void | SetChassisOutput (bool state) |
Enable/disable output from the chassis subsystem. | |
bool | HasBushings () const |
Return true if the vehicle model contains bushings. | |
Additional Inherited Members | |
![]() | |
ChTrackedVehicle (const std::string &name, ChContactMethod contact_method=ChContactMethod::NSC) | |
Construct a vehicle system with a default ChSystem. More... | |
ChTrackedVehicle (const std::string &name, ChSystem *system) | |
Construct a vehicle system using the specified ChSystem. More... | |
virtual void | UpdateInertiaProperties () override final |
Calculate current vehicle inertia properties. More... | |
virtual void | Output (int frame, ChVehicleOutput &database) const override |
Output data for all modeling components in the vehicle system. | |
![]() | |
ChVehicle (const std::string &name, ChContactMethod contact_method=ChContactMethod::NSC) | |
Construct a vehicle system with an underlying ChSystem. More... | |
ChVehicle (const std::string &name, ChSystem *system) | |
Construct a vehicle system using the specified ChSystem. More... | |
void | SetSystem (ChSystem *sys) |
Set the associated Chrono system. | |
![]() | |
template<typename T > | |
static bool | AnyOutput (const std::vector< std::shared_ptr< T >> &list) |
Utility function for testing if any subsystem in a list generates output. | |
![]() | |
std::shared_ptr< ChTrackAssembly > | m_tracks [2] |
track assemblies (left/right) | |
std::shared_ptr< ChDrivelineTV > | m_driveline |
driveline subsystem | |
std::shared_ptr< ChTrackCollisionManager > | m_collision_manager |
manager for internal collisions | |
std::shared_ptr< ChTrackContactManager > | m_contact_manager |
manager for internal contacts | |
![]() | |
std::string | m_name |
vehicle name | |
ChSystem * | m_system |
pointer to the Chrono system | |
bool | m_ownsSystem |
true if system created at construction | |
double | m_mass |
total vehicle mass | |
ChFrame | m_com |
current vehicle COM (relative to the vehicle reference frame) | |
ChMatrix33 | m_inertia |
current total vehicle inertia (Relative to the vehicle COM frame) | |
bool | m_output |
generate ouput for this vehicle system | |
ChVehicleOutput * | m_output_db |
vehicle output database | |
double | m_output_step |
output time step | |
double | m_next_output_time |
time for next output | |
int | m_output_frame |
current output frame | |
std::shared_ptr< ChChassis > | m_chassis |
handle to the main chassis subsystem | |
ChChassisRearList | m_chassis_rear |
list of rear chassis subsystems (can be empty) | |
ChChassisConnectorList | m_chassis_connectors |
list of chassis connector (must match m_chassis_rear) | |
std::shared_ptr< ChPowertrainAssembly > | m_powertrain_assembly |
associated powertrain system | |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_models/vehicle/marder/Marder_Vehicle.h
- /builds/uwsbel/chrono/src/chrono_models/vehicle/marder/Marder_Vehicle.cpp