Description
Base class for continuous band track shoes using rigid treads.
Derived classes specify actual template definitions, using different models for the track web.
#include <ChTrackShoeBand.h>
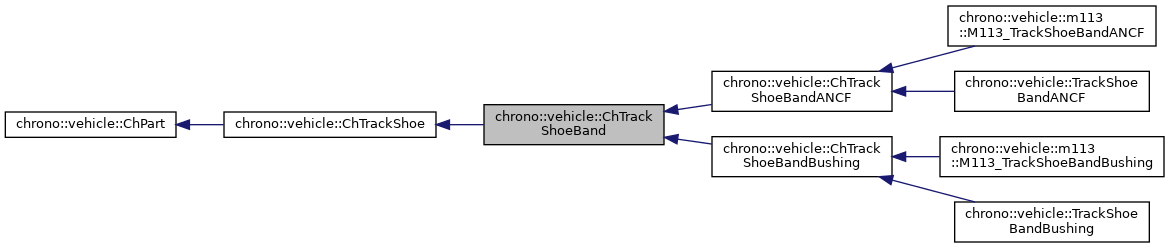
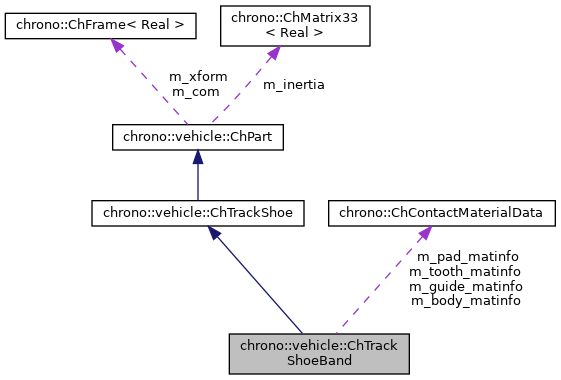
Public Member Functions | |
ChTrackShoeBand (const std::string &name) | |
virtual double | GetPitch () const override |
Return the pitch length of the track shoe. More... | |
virtual void | Initialize (std::shared_ptr< ChBodyAuxRef > chassis, const ChVector3d &location, const ChQuaternion<> &rotation) override |
Initialize this track shoe subsystem. More... | |
void | WriteTreadVisualizationMesh (const std::string &out_dir) |
Write the procedurally-generated tread body visualization mesh to a Wavefront OBJ file. | |
void | ExportTreadVisualizationMeshPovray (const std::string &out_dir) |
Export the procedurally-generated tread body visualization mesh as a macro in a PovRay include file. | |
![]() | |
virtual GuidePinType | GetType () const =0 |
Return the type of track shoe (guiding pin). More... | |
size_t | GetIndex () const |
Get the index of this track shoe within its containing track assembly. | |
std::shared_ptr< ChBody > | GetShoeBody () const |
Get the shoe body. | |
virtual ChVector3d | GetTension () const =0 |
Get track tension at this track shoe. More... | |
virtual double | GetHeight () const =0 |
Return the height of the track shoe. | |
virtual ChVehicleGeometry | GetGroundContactGeometry () const |
Return contact geometry and material for interaction with terrain. | |
void | EnableCollision (bool val) |
Turn on/off collision flag for the shoe body. | |
void | SetIndex (size_t index) |
Set the index of this track shoe within its containing track assembly. | |
virtual void | Connect (std::shared_ptr< ChTrackShoe > next, ChTrackAssembly *assembly, ChChassis *chassis, bool ccw)=0 |
Connect this track shoe to the specified neighbor. More... | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Protected Member Functions | |
virtual double | GetTreadMass () const =0 |
Return the mass of the tread body. | |
virtual double | GetWebMass () const =0 |
Return the mass of the web. More... | |
virtual const ChVector3d & | GetTreadInertia () const =0 |
Return the moments of inertia of the tread body. | |
virtual const ChVector3d & | GetWebInertia () const =0 |
Return the moments of inertia of the web. More... | |
virtual const ChVector3d & | GetGuideBoxDimensions () const =0 |
Return the dimensions of the contact box for the guiding pin. More... | |
virtual double | GetGuideBoxOffsetX () const =0 |
Return the offset (in X direction) of the guiding pin. | |
virtual ChVector3d | GetLateralContactPoint () const override |
Return the location of the guiding pin center, expressed in the shoe reference frame. | |
virtual double | GetBeltWidth () const =0 |
Return the width of the CB track belt (in the Y direction) | |
virtual double | GetToothTipLength () const =0 |
Return the length of the flat tip of the tread tooth tip (in the X direction) | |
virtual double | GetToothBaseLength () const =0 |
Return the length of the base of the tread tooth (in the X direction) where the tooth circular profile ends. | |
virtual double | GetToothWidth () const =0 |
Return the width of the one of the tooth profile sections of the tread tooth (in the Y direction) | |
virtual double | GetToothHeight () const =0 |
Return the height from the base to the tip of the tread tooth profile (in the Z direction) | |
virtual double | GetToothArcRadius () const =0 |
Return the radius of the tooth profile arc that connects the tooth tip and base lines. | |
virtual double | GetWebLength () const =0 |
Return the combined length of all of the web sections (in the X direction) | |
virtual double | GetWebThickness () const =0 |
Return the thickness of the web section (in the Z direction) | |
virtual double | GetTreadLength () const =0 |
Return the length of the tread below the web area (in the X direction, tread pad for ground contact) | |
virtual double | GetTreadThickness () const =0 |
Return the thickness of the tread below the web area (tread pad for ground contact) | |
virtual const std::string & | GetTreadVisualizationMeshName () const =0 |
Specify the name assigned to the procedurally-generated tread body visualization mesh. | |
void | AddShoeContact (ChContactMethod contact_method) |
Add contact geometry for the tread body. More... | |
void | AddShoeVisualization () |
Add visualization of the tread body, based on primitives corresponding to the contact shapes. More... | |
![]() | |
ChTrackShoe (const std::string &name) | |
Construct a track shoe subsystem with given name. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
virtual void | InitializeInertiaProperties ()=0 |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties ()=0 |
Update subsystem inertia properties. More... | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Static Protected Member Functions | |
static ChColor | GetColor (size_t index) |
Get index-specific color (for visualization) | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Protected Attributes | |
ChContactMaterialData | m_tooth_matinfo |
data for contact material for teeth (sprocket interaction) | |
ChContactMaterialData | m_body_matinfo |
date for contact material for main body (wheel interaction) | |
ChContactMaterialData | m_pad_matinfo |
data for contact material for pad (ground interaction) | |
ChContactMaterialData | m_guide_matinfo |
date for contact material for guide pin (wheel interaction) | |
![]() | |
size_t | m_index |
index of this track shoe within its containing track assembly | |
std::shared_ptr< ChBody > | m_shoe |
handle to the shoe body | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
Friends | |
class | ChSprocketBand |
class | SprocketBandContactCB |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
Constructor & Destructor Documentation
◆ ChTrackShoeBand()
chrono::vehicle::ChTrackShoeBand::ChTrackShoeBand | ( | const std::string & | name | ) |
- Parameters
-
[in] name name of the subsystem
Member Function Documentation
◆ AddShoeContact()
|
protected |
Add contact geometry for the tread body.
Note that this is for contact with wheels, idler, and ground only. This contact geometry does not affect contact with the sprocket.
◆ AddShoeVisualization()
|
protected |
Add visualization of the tread body, based on primitives corresponding to the contact shapes.
Note that the "primitive" shape for the tread body is a procedurally-generated mesh.
◆ GetGuideBoxDimensions()
|
protectedpure virtual |
Return the dimensions of the contact box for the guiding pin.
Note that this is for contact with wheels, idler, and ground only. This contact geometry does not affect contact with the sprocket.
Implemented in chrono::vehicle::m113::M113_TrackShoeBandANCF, chrono::vehicle::m113::M113_TrackShoeBandBushing, chrono::vehicle::TrackShoeBandANCF, and chrono::vehicle::TrackShoeBandBushing.
◆ GetPitch()
|
overridevirtual |
Return the pitch length of the track shoe.
This quantity must agree with the pitch of the sprocket gear.
Implements chrono::vehicle::ChTrackShoe.
◆ GetWebInertia()
|
protectedpure virtual |
Return the moments of inertia of the web.
These will be distributed over the specified number of web segments.
Implemented in chrono::vehicle::m113::M113_TrackShoeBandANCF, chrono::vehicle::m113::M113_TrackShoeBandBushing, chrono::vehicle::TrackShoeBandANCF, and chrono::vehicle::TrackShoeBandBushing.
◆ GetWebMass()
|
protectedpure virtual |
Return the mass of the web.
This will be equally distributed over the specified number of web segments.
Implemented in chrono::vehicle::m113::M113_TrackShoeBandANCF, chrono::vehicle::m113::M113_TrackShoeBandBushing, chrono::vehicle::TrackShoeBandANCF, and chrono::vehicle::TrackShoeBandBushing.
◆ Initialize()
|
overridevirtual |
Initialize this track shoe subsystem.
The track shoe is created within the specified system and initialized at the specified location and orientation (expressed in the global frame). This version initializes the bodies of a CB rigid-link track shoe such that the center of the track shoe subsystem is at the specified location and all bodies have the specified orientation.
- Parameters
-
[in] chassis handle to the chassis body [in] location location relative to the chassis frame [in] rotation orientation relative to the chassis frame
Reimplemented from chrono::vehicle::ChTrackShoe.
Reimplemented in chrono::vehicle::ChTrackShoeBandANCF, and chrono::vehicle::ChTrackShoeBandBushing.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/track_shoe/ChTrackShoeBand.h
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/track_shoe/ChTrackShoeBand.cpp