Description
Base class for a coil-spring or air-spring solid axle suspension.
The suspension subsystem is modeled with respect to a right-handed frame, with X pointing towards the front, Y to the left, and Z up (ISO standard). The suspension reference frame is assumed to be always aligned with that of the vehicle. When attached to a chassis, only an offset is provided.
All point locations are assumed to be given for the left half of the suspension and will be mirrored (reflecting the y coordinates) to construct the right side.
#include <ChSolidThreeLinkAxle.h>
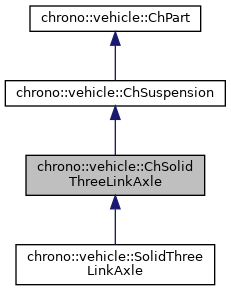
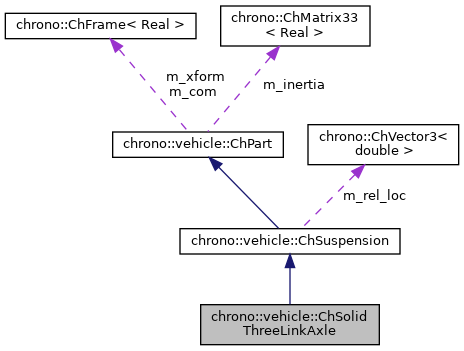
Public Member Functions | |
ChSolidThreeLinkAxle (const std::string &name) | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual bool | IsSteerable () const final override |
Specify whether or not this suspension can be steered. | |
virtual bool | IsIndependent () const final override |
Specify whether or not this is an independent suspension. | |
virtual void | Construct (std::shared_ptr< ChChassis > chassis, std::shared_ptr< ChSubchassis > subchassis, std::shared_ptr< ChSteering > steering, const ChVector3d &location, double left_ang_vel, double right_ang_vel) override |
Construct this suspension subsystem. More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the suspension subsystem. More... | |
virtual void | RemoveVisualizationAssets () override |
Remove visualization assets for the suspension subsystem. | |
virtual double | GetTrack () override |
Get the wheel track for the suspension subsystem. | |
std::shared_ptr< ChLinkTSDA > | GetSpring (VehicleSide side) const |
Get a handle to the specified spring element. | |
std::shared_ptr< ChLinkTSDA > | GetShock (VehicleSide side) const |
Get a handle to the specified shock (damper) element. | |
virtual std::vector< ForceTSDA > | ReportSuspensionForce (VehicleSide side) const override |
Return current suspension TSDA force information on the specified side. | |
double | GetSpringForce (VehicleSide side) const |
Get the force in the spring element. | |
double | GetSpringLength (VehicleSide side) const |
Get the current length of the spring element. | |
double | GetSpringDeformation (VehicleSide side) const |
Get the current deformation of the spring element. | |
double | GetShockForce (VehicleSide side) const |
Get the force in the shock (damper) element. | |
double | GetShockLength (VehicleSide side) const |
Get the current length of the shock (damper) element. | |
double | GetShockVelocity (VehicleSide side) const |
Get the current deformation velocity of the shock (damper) element. | |
virtual void | LogConstraintViolations (VehicleSide side) override |
Log current constraint violations. | |
void | LogHardpointLocations (const ChVector3d &ref, bool inches=false) |
![]() | |
const ChVector3d & | GetRelPosition () const |
Get the location of the suspension subsystem relative to the associated chassis reference frame. More... | |
std::shared_ptr< ChSpindle > | GetSpindle (VehicleSide side) const |
Get a handle to the spindle body on the specified side. | |
std::shared_ptr< ChShaft > | GetAxle (VehicleSide side) const |
Get a handle to the axle shaft on the specified side. | |
std::shared_ptr< ChLinkLockRevolute > | GetRevolute (VehicleSide side) const |
Get a handle to the revolute joint on the specified side. | |
const ChVector3d & | GetSpindlePos (VehicleSide side) const |
Get the global location of the spindle on the specified side. | |
ChQuaternion | GetSpindleRot (VehicleSide side) const |
Get the orientation of the spindle body on the specified side. More... | |
const ChVector3d & | GetSpindleLinVel (VehicleSide side) const |
Get the linear velocity of the spindle body on the specified side. More... | |
ChVector3d | GetSpindleAngVel (VehicleSide side) const |
Get the angular velocity of the spindle body on the specified side. More... | |
double | GetAxleSpeed (VehicleSide side) const |
Get the angular speed of the axle on the specified side. | |
virtual void | Synchronize (double time) |
Synchronize this suspension subsystem. | |
virtual void | Advance (double step) |
Advance the state of the suspension subsystem by the specified time step. | |
void | ApplyAxleTorque (VehicleSide side, double torque) |
Apply the provided motor torque. More... | |
void | Initialize (std::shared_ptr< ChChassis > chassis, std::shared_ptr< ChSubchassis > subchassis, std::shared_ptr< ChSteering > steering, const ChVector3d &location, double left_ang_vel=0, double right_ang_vel=0) |
Initialize this suspension subsystem. More... | |
virtual double | getSpindleRadius () const =0 |
Return the radius of the spindle body (visualization only). | |
virtual double | getSpindleWidth () const =0 |
Return the width of the spindle body (visualization only). | |
virtual std::shared_ptr< ChBody > | GetAntirollBody (VehicleSide side) const |
Specify the suspension body on the specified side to attach a possible antirollbar subsystem. More... | |
virtual std::shared_ptr< ChBody > | GetBrakeBody (VehicleSide side) const |
Specify the body on the specified side for a possible connection to brake subsystem. More... | |
virtual std::vector< ForceRSDA > | ReportSuspensionTorque (VehicleSide side) const |
Return current RSDA torque information on the specified side. | |
void | ApplyParkingBrake (bool brake) |
Simple model of a parking brake. | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
virtual uint16_t | GetVehicleTag () const |
Get the tag of the associated vehicle. More... | |
int | GetBodyTag () const |
Get the tag for component bodies. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Protected Types | |
enum | PointId { SHOCK_A, SHOCK_C, SPRING_A, SPRING_C, SPINDLE, TRIANGLE_A, TRIANGLE_C, LINK_A, LINK_C, NUM_POINTS } |
Identifiers for the various hardpoints. More... | |
Protected Member Functions | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
virtual const ChVector3d | getLocation (PointId which)=0 |
Return the location of the specified hardpoint. More... | |
virtual double | getCamberAngle () const |
Return the camber angle, in radians (default: 0). | |
virtual double | getToeAngle () const |
Return the toe angle, in radians (default: 0). More... | |
virtual const ChVector3d | getAxleTubeCOM () const =0 |
Return the center of mass of the axle tube. | |
virtual double | getAxleTubeMass () const =0 |
Return the mass of the axle tube body. | |
virtual double | getSpindleMass () const =0 |
Return the mass of the spindle body. | |
virtual double | getTriangleMass () const =0 |
Return the mass of the triangle body. | |
virtual double | getLinkMass () const =0 |
Return the mass of the triangle body. | |
virtual double | getAxleTubeRadius () const =0 |
Return the radius of the axle tube body (visualization only). | |
virtual const ChVector3d & | getAxleTubeInertia () const =0 |
Return the moments of inertia of the axle tube body. | |
virtual const ChVector3d & | getSpindleInertia () const =0 |
Return the moments of inertia of the spindle body. | |
virtual const ChVector3d & | getTriangleInertia () const =0 |
Return the moments of inertia of the triangle body. | |
virtual const ChVector3d & | getLinkInertia () const =0 |
Return the moments of inertia of the triangle body. | |
virtual double | getAxleInertia () const =0 |
Return the inertia of the axle shaft. | |
virtual double | getSpringRestLength () const =0 |
Return the free (rest) length of the spring element. | |
virtual double | getShockRestLength () const |
Return the free (rest) length of the shock element. | |
virtual std::shared_ptr< ChLinkTSDA::ForceFunctor > | getSpringForceFunctor () const =0 |
Return the functor object for spring force. | |
virtual std::shared_ptr< ChLinkTSDA::ForceFunctor > | getShockForceFunctor () const =0 |
Return the functor object for shock force. | |
![]() | |
ChSuspension (const std::string &name) | |
Construct a suspension subsystem with given name. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Protected Attributes | |
std::shared_ptr< ChBody > | m_axleTube |
handles to the axle tube body /std::shared_ptr<ChBody> m_tierod; ///< handles to the tierod body /std::shared_ptr<ChLinkLockFree> m_axleTubeGuide; ///< allows all translations and rotations | |
std::shared_ptr< ChBody > | m_triangleBody |
axle guide body with spherical link and rotary link | |
std::shared_ptr< ChLinkLockRevolute > | m_triangleRev |
triangle to chassis revolute joint | |
std::shared_ptr< ChLinkLockSpherical > | m_triangleSph |
triangle to axle tube spherical joint | |
std::shared_ptr< ChBody > | m_linkBody [2] |
axle guide body with spherical link and universal link | |
std::shared_ptr< ChLinkUniversal > | m_linkBodyToChassis [2] |
std::shared_ptr< ChLinkLockSpherical > | m_linkBodyToAxleTube [2] |
std::shared_ptr< ChLinkTSDA > | m_shock [2] |
handles to the spring links (L/R) | |
std::shared_ptr< ChLinkTSDA > | m_spring [2] |
handles to the shock links (L/R) | |
![]() | |
ChVector3d | m_rel_loc |
location relative to chassis | |
std::shared_ptr< ChSpindle > | m_spindle [2] |
handles to spindle bodies | |
std::shared_ptr< ChShaft > | m_axle [2] |
handles to axle shafts | |
std::shared_ptr< ChShaftBodyRotation > | m_axle_to_spindle [2] |
handles to spindle-shaft connectors | |
std::shared_ptr< ChLinkLockRevolute > | m_revolute [2] |
handles to spindle revolute joints | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
int | m_obj_tag |
tag for part objects | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Member Enumeration Documentation
◆ PointId
|
protected |
Identifiers for the various hardpoints.
Constructor & Destructor Documentation
◆ ChSolidThreeLinkAxle()
chrono::vehicle::ChSolidThreeLinkAxle::ChSolidThreeLinkAxle | ( | const std::string & | name | ) |
- Parameters
-
[in] name name of the subsystem
Member Function Documentation
◆ AddVisualizationAssets()
|
overridevirtual |
Add visualization assets for the suspension subsystem.
This default implementation uses primitives.
Reimplemented from chrono::vehicle::ChSuspension.
◆ Construct()
|
overridevirtual |
Construct this suspension subsystem.
The suspension subsystem is initialized by attaching it to the specified chassis and (if provided) to the specified subchassis, at the specified location (with respect to and expressed in the reference frame of the chassis). It is assumed that the suspension reference frame is always aligned with the chassis reference frame. Since this suspension is non-steerable, the steering subsystem is always ignored.
- Parameters
-
[in] chassis associated chassis subsystem [in] subchassis associated subchassis subsystem (may be null) [in] steering associated steering subsystem (may be null) [in] location location relative to the chassis frame [in] left_ang_vel initial angular velocity of left wheel [in] right_ang_vel initial angular velocity of right wheel
Implements chrono::vehicle::ChSuspension.
◆ getLocation()
|
protectedpure virtual |
Return the location of the specified hardpoint.
The returned location must be expressed in the suspension reference frame.
◆ getToeAngle()
|
inlineprotectedvirtual |
Return the toe angle, in radians (default: 0).
A positive value indicates toe-in, a negative value indicates toe-out.
Reimplemented in chrono::vehicle::SolidThreeLinkAxle.
◆ InitializeInertiaProperties()
|
overrideprotectedvirtual |
Initialize subsystem inertia properties.
Derived classes must override this function and set the subsystem mass (m_mass) and, if constant, the subsystem COM frame and its inertia tensor. This function is called during initialization of the vehicle system.
Implements chrono::vehicle::ChPart.
◆ UpdateInertiaProperties()
|
overrideprotectedvirtual |
Update subsystem inertia properties.
Derived classes must override this function and set the global subsystem transform (m_xform) and, unless constant, the subsystem COM frame (m_com) and its inertia tensor (m_inertia). Calculate the current inertia properties and global frame of this subsystem. This function is called every time the state of the vehicle system is advanced in time.
Implements chrono::vehicle::ChPart.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/suspension/ChSolidThreeLinkAxle.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/suspension/ChSolidThreeLinkAxle.cpp