Description
Base class for a Toe Bar steering subsystem.
The steering subsystem is modeled with respect to a right-handed frame with X pointing towards the front, Y to the left, and Z up (ISO standard).
When attached to a chassis, both an offset and a rotation (as a quaternion) are provided.
#include <ChRotaryArm.h>
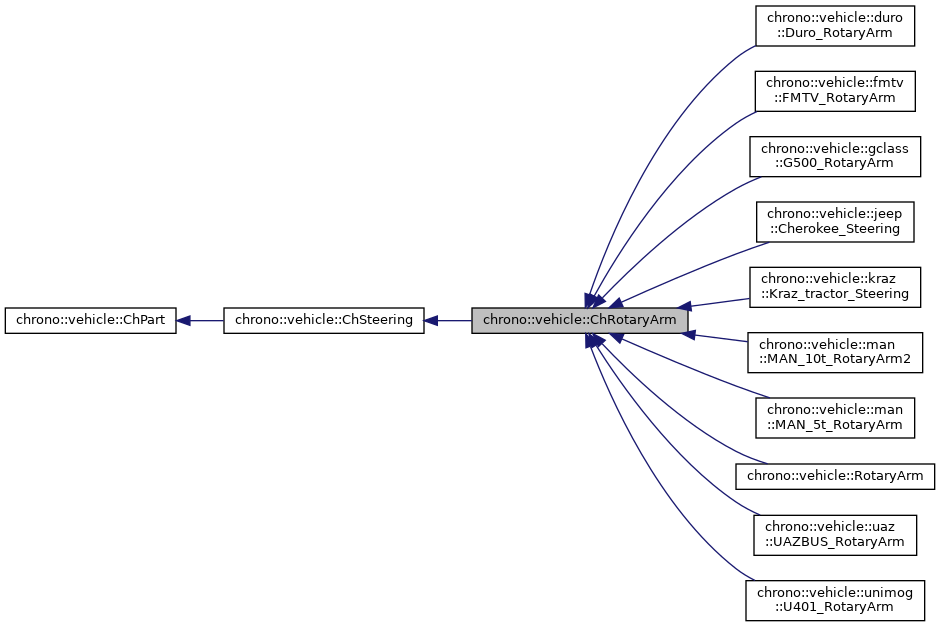
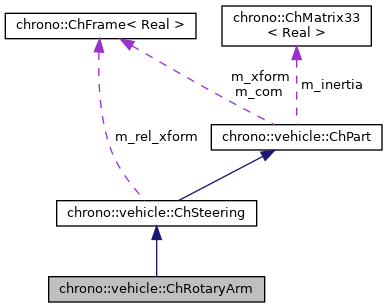
Public Member Functions | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis, const ChVector3d &location, const ChQuaternion<> &rotation) override |
Initialize this steering subsystem. More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the steering subsystem. More... | |
virtual void | RemoveVisualizationAssets () override |
Remove visualization assets for the steering subsystem. | |
virtual void | Synchronize (double time, const DriverInputs &driver_inputs) override |
Update the state of this steering subsystem at the current time. More... | |
virtual void | LogConstraintViolations () override |
Log current constraint violations. | |
![]() | |
const ChFrame & | GetRelTransform () const |
Get the frame of the steering subsystem relative to the associated chassis reference frame. | |
std::shared_ptr< ChBody > | GetSteeringLink () const |
Get a handle to the main link of the steering subsystems. More... | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Protected Types | |
enum | PointId { ARM_L, ARM_C, NUM_POINTS } |
Identifiers for the various hardpoints. More... | |
enum | DirectionId { REV_AXIS, NUM_DIRS } |
Identifiers for the various direction unit vectors. More... | |
Protected Member Functions | |
ChRotaryArm (const std::string &name, bool vehicle_frame_inertia=false) | |
Protected constructor. More... | |
void | SetVehicleFrameInertiaFlag (bool val) |
Indicate whether or not inertia matrices are specified with respect to a vehicle-aligned centroidal frame (flag=true) or with respect to the body centroidal frame (flag=false). More... | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
virtual const ChVector3d | getLocation (PointId which)=0 |
Return the location of the specified hardpoint. More... | |
virtual const ChVector3d | getDirection (DirectionId which)=0 |
Return the unit vector for the specified direction. More... | |
virtual double | getPitmanArmMass () const =0 |
Return the mass of the Pitman arm body. | |
virtual const ChVector3d & | getPitmanArmInertiaMoments () const =0 |
Return the moments of inertia of the Pitman arm body. | |
virtual const ChVector3d & | getPitmanArmInertiaProducts () const =0 |
Return the products of inertia of the Pitman arm body. | |
virtual double | getPitmanArmRadius () const =0 |
Return the radius of the Pitman arm body (visualization only). | |
virtual double | getMaxAngle () const =0 |
Return the maximum rotation angle of the revolute joint. | |
![]() | |
ChSteering (const std::string &name) | |
Construct a steering subsystem with given name. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Protected Attributes | |
std::shared_ptr< ChLinkMotorRotationAngle > | m_revolute |
handle to the chassis-arm revolute joint | |
![]() | |
ChFrame | m_rel_xform |
location and orientation relative to associated chassis | |
std::shared_ptr< ChBody > | m_link |
handle to the main steering link | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Member Enumeration Documentation
◆ DirectionId
|
protected |
◆ PointId
|
protected |
Constructor & Destructor Documentation
◆ ChRotaryArm()
|
protected |
Protected constructor.
- Parameters
-
[in] name name of the subsystem [in] vehicle_frame_inertia inertia specified in vehicle-aligned centroidal frames?
Member Function Documentation
◆ AddVisualizationAssets()
|
overridevirtual |
Add visualization assets for the steering subsystem.
This default implementation uses primitives.
Reimplemented from chrono::vehicle::ChPart.
◆ getDirection()
|
protectedpure virtual |
Return the unit vector for the specified direction.
The returned vector must be expressed in the suspension reference frame.
Implemented in chrono::vehicle::jeep::Cherokee_Steering, chrono::vehicle::duro::Duro_RotaryArm, chrono::vehicle::gclass::G500_RotaryArm, chrono::vehicle::kraz::Kraz_tractor_Steering, chrono::vehicle::man::MAN_10t_RotaryArm2, chrono::vehicle::man::MAN_5t_RotaryArm, chrono::vehicle::fmtv::FMTV_RotaryArm, chrono::vehicle::uaz::UAZBUS_RotaryArm, chrono::vehicle::unimog::U401_RotaryArm, and chrono::vehicle::RotaryArm.
◆ getLocation()
|
protectedpure virtual |
Return the location of the specified hardpoint.
The returned location must be expressed in the suspension reference frame.
Implemented in chrono::vehicle::jeep::Cherokee_Steering, chrono::vehicle::duro::Duro_RotaryArm, chrono::vehicle::gclass::G500_RotaryArm, chrono::vehicle::kraz::Kraz_tractor_Steering, chrono::vehicle::man::MAN_10t_RotaryArm2, chrono::vehicle::man::MAN_5t_RotaryArm, chrono::vehicle::fmtv::FMTV_RotaryArm, chrono::vehicle::uaz::UAZBUS_RotaryArm, chrono::vehicle::unimog::U401_RotaryArm, and chrono::vehicle::RotaryArm.
◆ Initialize()
|
overridevirtual |
Initialize this steering subsystem.
The steering subsystem is initialized by attaching it to the specified chassis at the specified location (with respect to and expressed in the reference frame of the chassis) and with specified orientation (with respect to the chassis reference frame).
- Parameters
-
[in] chassis associated chassis subsystem [in] location location relative to the chassis frame [in] rotation orientation relative to the chassis frame
Reimplemented from chrono::vehicle::ChSteering.
◆ InitializeInertiaProperties()
|
overrideprotectedvirtual |
Initialize subsystem inertia properties.
Derived classes must override this function and set the subsystem mass (m_mass) and, if constant, the subsystem COM frame and its inertia tensor. This function is called during initialization of the vehicle system.
Implements chrono::vehicle::ChPart.
◆ SetVehicleFrameInertiaFlag()
|
inlineprotected |
Indicate whether or not inertia matrices are specified with respect to a vehicle-aligned centroidal frame (flag=true) or with respect to the body centroidal frame (flag=false).
Note that this function must be called before Initialize().
◆ Synchronize()
|
overridevirtual |
Update the state of this steering subsystem at the current time.
The steering subsystem is provided the current steering driver input (a value between -1 and +1). Positive steering input indicates steering to the left. This function is called during the vehicle update.
- Parameters
-
[in] time current time [in] driver_inputs current driver inputs
Implements chrono::vehicle::ChSteering.
◆ UpdateInertiaProperties()
|
overrideprotectedvirtual |
Update subsystem inertia properties.
Derived classes must override this function and set the global subsystem transform (m_xform) and, unless constant, the subsystem COM frame (m_com) and its inertia tensor (m_inertia). Calculate the current inertia properties and global frame of this subsystem. This function is called every time the state of the vehicle system is advanced in time.
Implements chrono::vehicle::ChPart.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/steering/ChRotaryArm.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/steering/ChRotaryArm.cpp