Description
Base class for a Hendrickson PRIMAXX EX suspension.
Derived from ChSuspension, but still an abstract base class.
The suspension subsystem is modeled with respect to a right-handed frame, with X pointing towards the front, Y to the left, and Z up (ISO standard). The suspension reference frame is assumed to be always aligned with that of the vehicle. When attached to a chassis, only an offset is provided.
All point locations are assumed to be given for the left half of the suspension and will be mirrored (reflecting the y coordinates) to construct the right side.
#include <ChHendricksonPRIMAXX.h>

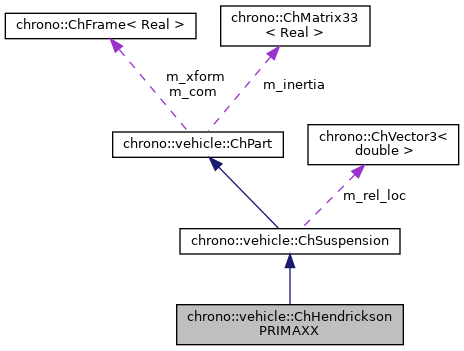
Public Member Functions | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual bool | IsSteerable () const final override |
Specify whether or not this suspension can be steered. | |
virtual bool | IsIndependent () const final override |
Specify whether or not this is an independent suspension. | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis, std::shared_ptr< ChSubchassis > subchassis, std::shared_ptr< ChSteering > steering, const ChVector3d &location, double left_ang_vel=0, double right_ang_vel=0) override |
Initialize this suspension subsystem. More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the suspension subsystem. More... | |
virtual void | RemoveVisualizationAssets () override |
Remove visualization assets for the suspension subsystem. | |
virtual double | GetTrack () override |
Get the wheel track for the suspension subsystem. | |
virtual std::vector< ForceTSDA > | ReportSuspensionForce (VehicleSide side) const override |
Return current suspension TSDA force information on the specified side. | |
double | GetShockLBForce (VehicleSide side) const |
There could be a spring (coil or air) and damper element between chassis and lower beam and a second spring and damper element between chassis and housing. More... | |
double | GetShockLBLength (VehicleSide side) const |
Get the current length of the spring-damper element. | |
double | GetShockLBVelocity (VehicleSide side) const |
Get the current deformation velocity of the spring-damper element. | |
double | GetShockAHForce (VehicleSide side) const |
Spring (coil or air) and damper element between chassis and axle housing (AH) Get the force in the air spring (coil or spring) and a damper element. More... | |
double | GetShockAHLength (VehicleSide side) const |
Get the current length of the spring-damper element. | |
double | GetShockAHVelocity (VehicleSide side) const |
Get the current deformation velocity of the spring-damper element. | |
virtual void | LogConstraintViolations (VehicleSide side) override |
Log current constraint violations. | |
void | LogHardpointLocations (const ChVector3d &ref, bool inches=false) |
Log the locations of all hardpoints. More... | |
![]() | |
const ChVector3d & | GetRelPosition () const |
Get the location of the suspension subsystem relative to the associated chassis reference frame. More... | |
std::shared_ptr< ChBody > | GetSpindle (VehicleSide side) const |
Get a handle to the spindle body on the specified side. | |
std::shared_ptr< ChShaft > | GetAxle (VehicleSide side) const |
Get a handle to the axle shaft on the specified side. | |
std::shared_ptr< ChLinkLockRevolute > | GetRevolute (VehicleSide side) const |
Get a handle to the revolute joint on the specified side. | |
const ChVector3d & | GetSpindlePos (VehicleSide side) const |
Get the global location of the spindle on the specified side. | |
ChQuaternion | GetSpindleRot (VehicleSide side) const |
Get the orientation of the spindle body on the specified side. More... | |
const ChVector3d & | GetSpindleLinVel (VehicleSide side) const |
Get the linear velocity of the spindle body on the specified side. More... | |
ChVector3d | GetSpindleAngVel (VehicleSide side) const |
Get the angular velocity of the spindle body on the specified side. More... | |
double | GetAxleSpeed (VehicleSide side) const |
Get the angular speed of the axle on the specified side. | |
virtual void | Synchronize (double time) |
Synchronize this suspension subsystem. | |
virtual void | Advance (double step) |
Advance the state of the suspension subsystem by the specified time step. | |
void | ApplyAxleTorque (VehicleSide side, double torque) |
Apply the provided motor torque. More... | |
virtual double | getSpindleRadius () const =0 |
Return the radius of the spindle body (visualization only). | |
virtual double | getSpindleWidth () const =0 |
Return the width of the spindle body (visualization only). | |
virtual std::shared_ptr< ChBody > | GetAntirollBody (VehicleSide side) const |
Specify the suspension body on the specified side to attach a possible antirollbar subsystem. More... | |
virtual std::shared_ptr< ChBody > | GetBrakeBody (VehicleSide side) const |
Specify the body on the specified side for a possible connection to brake subsystem. More... | |
virtual std::vector< ForceRSDA > | ReportSuspensionTorque (VehicleSide side) const |
Return current RSDA torque information on the specified side. | |
void | ApplyParkingBrake (bool brake) |
Simple model of a parking brake. | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Protected Types | |
enum | PointId { SPINDLE, KNUCKLE_L, KNUCKLE_U, TIEROD_C, TIEROD_K, TORQUEROD_C, TORQUEROD_AH, LOWERBEAM_C, LOWERBEAM_AH, LOWERBEAM_TB, SHOCKAH_C, SHOCKAH_AH, SHOCKLB_C, SHOCKLB_LB, KNUCKLE_CM, TORQUEROD_CM, LOWERBEAM_CM, NUM_POINTS } |
Identifiers for the various hardpoints. More... | |
enum | DirectionId { UNIV_AXIS_LOWERBEAM_BEAM, UNIV_AXIS_LOWERBEAM_CHASSIS, UNIV_AXIS_TORQUEROD_ROD, UNIV_AXIS_TORQUEROD_CHASSIS, NUM_DIRS } |
Identifiers for the various vectors. More... | |
Protected Member Functions | |
ChHendricksonPRIMAXX (const std::string &name) | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
virtual bool | UseTierodBodies () const |
Indicate whether or not tirod bodies are modelled (default: false). More... | |
virtual const ChVector3d | getLocation (PointId which)=0 |
Return the location of the specified hardpoint. More... | |
virtual const ChVector3d | getDirection (DirectionId which)=0 |
virtual const ChVector3d | getAxlehousingCOM () const =0 |
Return the center of mass of the axle tube. | |
virtual const ChVector3d | getTransversebeamCOM () const =0 |
Return the center of mass of the transverse beam. | |
virtual double | getSpindleMass () const =0 |
Return the mass of the spindle body. | |
virtual double | getKnuckleMass () const =0 |
Return the mass of the knuckle body. | |
virtual double | getTorquerodMass () const =0 |
Return the mass of the torque rod body. | |
virtual double | getLowerbeamMass () const =0 |
Return the mass of the lower beam body. | |
virtual double | getTransversebeamMass () const =0 |
Return the mass of the transverse beam body. | |
virtual double | getAxlehousingMass () const =0 |
Return the mass of the axle housing body. | |
virtual double | getTierodMass () const |
Return the mass of the tierod body. | |
virtual const ChVector3d & | getSpindleInertia () const =0 |
Return the moments of inertia of the spindle body. | |
virtual const ChVector3d & | getKnuckleInertia () const =0 |
Return the moments of inertia of the knuckle body. | |
virtual const ChVector3d & | getTorquerodInertia () const =0 |
Return the moments of inertia of the torque rod body. | |
virtual const ChVector3d & | getLowerbeamInertia () const =0 |
Return the moments of inertia of the lower beam body. | |
virtual const ChVector3d & | getTransversebeamInertia () const =0 |
Return the moments of inertia of the transverse beam body. | |
virtual const ChVector3d & | getAxlehousingInertia () const =0 |
Return the moments of inertia of the axle housing body. | |
virtual const ChVector3d | getTierodInertia () const |
Return the moments of inertia of the tierod body. | |
virtual double | getAxleInertia () const =0 |
Return the inertia of the axle shaft. | |
virtual double | getKnuckleRadius () const =0 |
Return the radius of the knuckle body (visualization only). | |
virtual double | getTorquerodRadius () const =0 |
Return the radius of the torque rod body (visualization only). | |
virtual double | getLowerbeamRadius () const =0 |
Return the radius of the lower beam body (visualization only). | |
virtual double | getTransversebeamRadius () const =0 |
Return the radius of the transverse beam body (visualization only). | |
virtual double | getAxlehousingRadius () const =0 |
Return the radius of the axle housing body (visualization only). | |
virtual double | getTierodRadius () const |
Return the radius of the tierod body (visualization only). | |
virtual double | getShockLBRestLength () const =0 |
Return the free (rest) length of the spring element. | |
virtual std::shared_ptr< ChLinkTSDA::ForceFunctor > | getShockLBForceCallback () const =0 |
Return the functor object for shock force. | |
virtual double | getShockAHRestLength () const =0 |
Return the free (rest) length of the spring element. | |
virtual std::shared_ptr< ChLinkTSDA::ForceFunctor > | getShockAHForceCallback () const =0 |
Return the functor object for shock force. | |
virtual std::shared_ptr< ChVehicleBushingData > | getTierodBushingData () const |
Return stiffness and damping data for the tierod bushings. More... | |
![]() | |
ChSuspension (const std::string &name) | |
Construct a suspension subsystem with given name. | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Protected Attributes | |
std::shared_ptr< ChBody > | m_knuckle [2] |
the knuckle bodies (left/right) | |
std::shared_ptr< ChBody > | m_torquerod [2] |
torquerod bodies (left/right) | |
std::shared_ptr< ChBody > | m_lowerbeam [2] |
lowerbeam bodies (left/right) | |
std::shared_ptr< ChBody > | m_transversebeam |
transversebeam body | |
std::shared_ptr< ChBody > | m_axlehousing |
axlehousing body | |
std::shared_ptr< ChBody > | m_tierod [2] |
tierod bodies, if used (left/right) | |
std::shared_ptr< ChLinkLockRevolute > | m_revoluteKingpin [2] |
knuckle-axle housing joints (left/right) | |
std::shared_ptr< ChLinkLockSpherical > | m_sphericalTorquerod [2] |
torquerod-axle housing joints (left/right) | |
std::shared_ptr< ChLinkLockRevolute > | m_revoluteTorquerod [2] |
torquerod-chasis joints (left/right) | |
std::shared_ptr< ChLinkLockSpherical > | m_sphericalLowerbeam [2] |
lowerbeam-axle housing joints (left/right) | |
std::shared_ptr< ChLinkLockRevolute > | m_revoluteLowerbeam [2] |
lowerbeam chasis joints (left/right) | |
std::shared_ptr< ChLinkLockSpherical > | m_sphericalTB [2] |
transversebeam-lower beam joints (left/right) | |
std::shared_ptr< ChLinkDistance > | m_distTierod [2] |
tierod distance constraints (left/right) | |
std::shared_ptr< ChVehicleJoint > | m_sphericalTierod [2] |
tierod-upright spherical joints (left/right) | |
std::shared_ptr< ChVehicleJoint > | m_universalTierod [2] |
tierod-chassis universal joints (left/right) | |
std::shared_ptr< ChLinkTSDA > | m_shockLB [2] |
spring links (left/right) | |
std::shared_ptr< ChLinkTSDA > | m_shockAH [2] |
spring links (left/right) | |
![]() | |
ChVector3d | m_rel_loc |
location relative to chassis | |
std::shared_ptr< ChBody > | m_spindle [2] |
handles to spindle bodies | |
std::shared_ptr< ChShaft > | m_axle [2] |
handles to axle shafts | |
std::shared_ptr< ChShaftBodyRotation > | m_axle_to_spindle [2] |
handles to spindle-shaft connectors | |
std::shared_ptr< ChLinkLockRevolute > | m_revolute [2] |
handles to spindle revolute joints | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Member Enumeration Documentation
◆ DirectionId
|
protected |
Identifiers for the various vectors.
◆ PointId
|
protected |
Identifiers for the various hardpoints.
Constructor & Destructor Documentation
◆ ChHendricksonPRIMAXX()
|
protected |
- Parameters
-
[in] name name of the subsystem
Member Function Documentation
◆ AddVisualizationAssets()
|
overridevirtual |
Add visualization assets for the suspension subsystem.
This default implementation uses primitives.
Reimplemented from chrono::vehicle::ChSuspension.
◆ getLocation()
|
protectedpure virtual |
Return the location of the specified hardpoint.
The returned location must be expressed in the suspension reference frame.
◆ GetShockAHForce()
|
inline |
Spring (coil or air) and damper element between chassis and axle housing (AH) Get the force in the air spring (coil or spring) and a damper element.
Get the force in the spring-damper element.
◆ GetShockLBForce()
|
inline |
There could be a spring (coil or air) and damper element between chassis and lower beam and a second spring and damper element between chassis and housing.
Spring (coil or air) and damper element between chassis and lower beam (LB) Get the force in the air spring (coil or spring) and a damper element Get the force in the spring-damper element.
◆ getTierodBushingData()
|
inlineprotectedvirtual |
Return stiffness and damping data for the tierod bushings.
Used only if tierod bodies are defined (see UseTierodBody). Returning nullptr (default) results in using kinematic joints (spherical + universal).
Reimplemented in chrono::vehicle::HendricksonPRIMAXX.
◆ Initialize()
|
overridevirtual |
Initialize this suspension subsystem.
The suspension subsystem is initialized by attaching it to the specified chassis and (if provided) to the specified subchassis, at the specified location (with respect to and expressed in the reference frame of the chassis). It is assumed that the suspension reference frame is always aligned with the chassis reference frame. If a steering subsystem is provided, the suspension tierods are to be attached to the steering's central link body (steered suspension); otherwise they are to be attached to the chassis (non-steered suspension).
- Parameters
-
[in] chassis associated chassis subsystem [in] subchassis associated subchassis subsystem (may be null) [in] steering associated steering subsystem (may be null) [in] location location relative to the chassis frame [in] left_ang_vel initial angular velocity of left wheel [in] right_ang_vel initial angular velocity of right wheel
Reimplemented from chrono::vehicle::ChSuspension.
◆ InitializeInertiaProperties()
|
overrideprotectedvirtual |
Initialize subsystem inertia properties.
Derived classes must override this function and set the subsystem mass (m_mass) and, if constant, the subsystem COM frame and its inertia tensor. This function is called during initialization of the vehicle system.
Implements chrono::vehicle::ChPart.
◆ LogHardpointLocations()
void chrono::vehicle::ChHendricksonPRIMAXX::LogHardpointLocations | ( | const ChVector3d & | ref, |
bool | inches = false |
||
) |
Log the locations of all hardpoints.
The reported locations are expressed in the suspension reference frame. By default, these values are reported in SI units (meters), but can be optionally reported in inches.
◆ UpdateInertiaProperties()
|
overrideprotectedvirtual |
Update subsystem inertia properties.
Derived classes must override this function and set the global subsystem transform (m_xform) and, unless constant, the subsystem COM frame (m_com) and its inertia tensor (m_inertia). Calculate the current inertia properties and global frame of this subsystem. This function is called every time the state of the vehicle system is advanced in time.
Implements chrono::vehicle::ChPart.
◆ UseTierodBodies()
|
inlineprotectedvirtual |
Indicate whether or not tirod bodies are modelled (default: false).
If false, tierods are modelled using distance constraints. If true, rigid tierod bodies are created (in which case a derived class must provide the mass and inertia) and connected either with kinematic joints or bushings (depending on whether or not bushing data is defined).
Reimplemented in chrono::vehicle::HendricksonPRIMAXX.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/suspension/ChHendricksonPRIMAXX.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/suspension/ChHendricksonPRIMAXX.cpp