Description
Base class for a wheeled vehicle driveline subsystem.
#include <ChDrivelineWV.h>
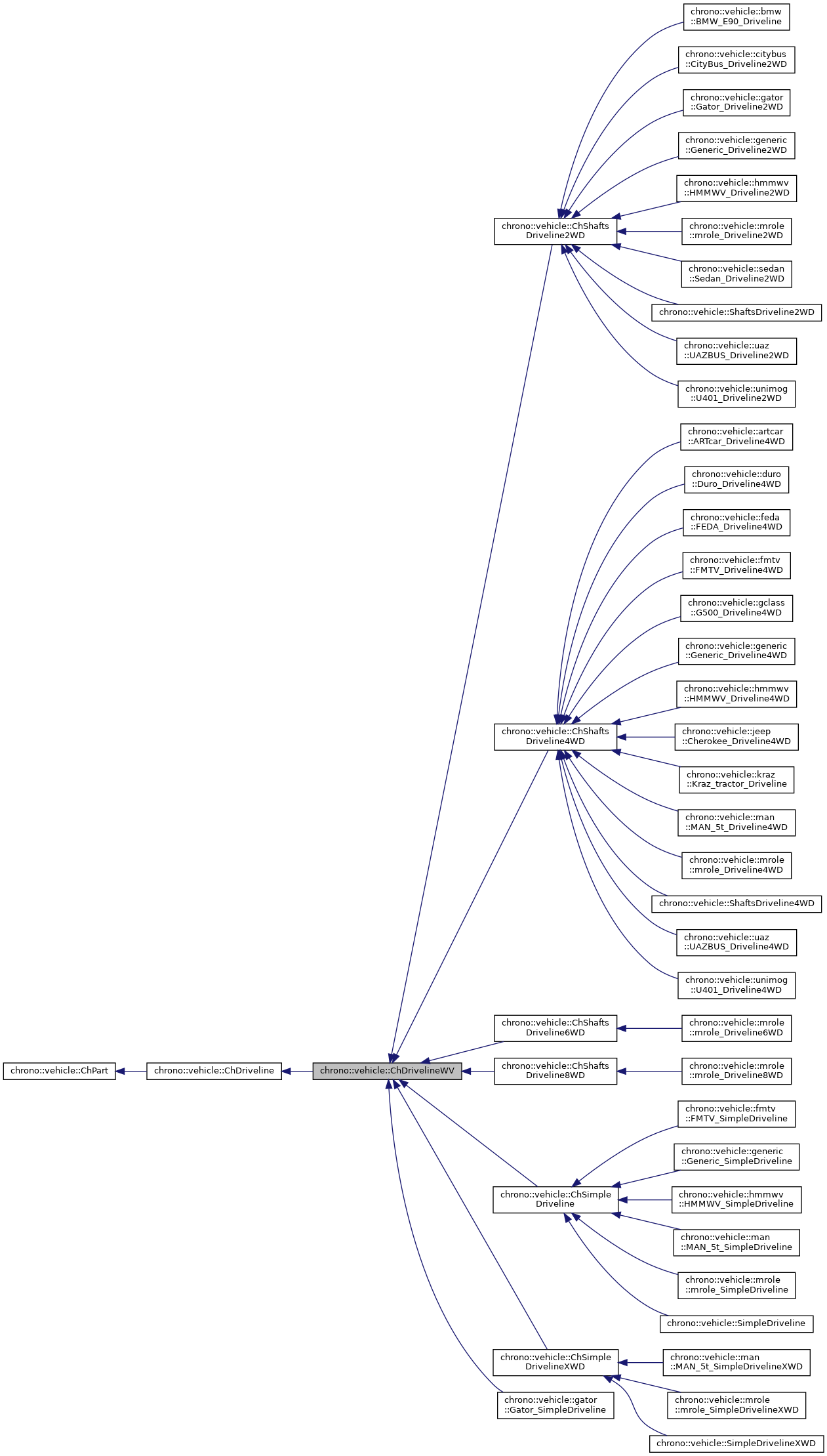
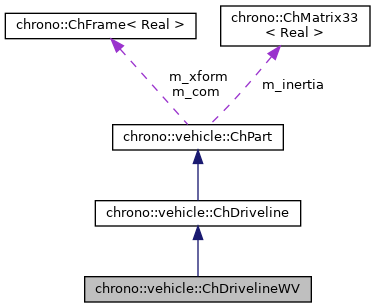
Public Member Functions | |
virtual unsigned int | GetNumDrivenAxles () const =0 |
Return the number of driven axles. | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis, const ChAxleList &axles, const std::vector< int > &driven_axles)=0 |
Initialize the driveline subsystem. More... | |
virtual void | LockAxleDifferential (int axle, bool lock) |
Lock/unlock the differential on the specified axle. More... | |
virtual void | LockCentralDifferential (int which, bool lock) |
Lock/unlock the specified central differential. More... | |
const std::vector< int > & | GetDrivenAxleIndexes () const |
Get the indexes of the vehicle's axles driven by this driveline subsystem. | |
virtual double | GetSpindleTorque (int axle, VehicleSide side) const =0 |
Get the motor torque to be applied to the specified spindle. | |
![]() | |
void | Initialize (std::shared_ptr< ChChassis > chassis) |
Initialize the driveline. | |
virtual void | Synchronize (double time, const DriverInputs &driver_inputs, double driveshaft_torque)=0 |
Update the driveline subsystem. More... | |
virtual void | Disconnect ()=0 |
Disconnect driveline. | |
virtual double | GetOutputDriveshaftSpeed () const =0 |
Return the output driveline speed of the driveshaft. More... | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
bool | IsInitialized () const |
Return flag indicating whether or not the part is fully constructed. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Protected Member Functions | |
ChDrivelineWV (const std::string &name) | |
![]() | |
ChDriveline (const std::string &name) | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector3d &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) const |
Export the list of bodies to the specified JSON document. | |
void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) const |
Export the list of shafts to the specified JSON document. | |
void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) const |
Export the list of joints to the specified JSON document. | |
void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) const |
Export the list of shaft couples to the specified JSON document. | |
void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) const |
Export the list of markers to the specified JSON document. | |
void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) const |
Export the list of translational springs to the specified JSON document. | |
void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) const |
Export the list of rotational springs to the specified JSON document. | |
void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) const |
Export the list of body-body loads to the specified JSON document. | |
Protected Attributes | |
std::vector< int > | m_driven_axles |
indexes of the driven vehicle axles | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_initialized |
specifies whether ot not the part is fully constructed | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector3d &moments, const ChVector3d &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Member Function Documentation
◆ Initialize()
|
pure virtual |
Initialize the driveline subsystem.
This function connects this driveline subsystem to the specified axle subsystems.
- Parameters
-
chassis associated chassis subsystem axles list of all vehicle axle subsystems driven_axles indexes of the driven vehicle axles
Implemented in chrono::vehicle::ChShaftsDriveline8WD, chrono::vehicle::ChShaftsDriveline6WD, chrono::vehicle::ChShaftsDriveline4WD, chrono::vehicle::ChShaftsDriveline2WD, chrono::vehicle::ChSimpleDrivelineXWD, chrono::vehicle::ChSimpleDriveline, and chrono::vehicle::gator::Gator_SimpleDriveline.
◆ LockAxleDifferential()
|
virtual |
Lock/unlock the differential on the specified axle.
By convention, axles are counted front to back, starting with index 0 for the front-most axle.
Reimplemented in chrono::vehicle::ChShaftsDriveline8WD, chrono::vehicle::ChShaftsDriveline6WD, chrono::vehicle::ChShaftsDriveline2WD, and chrono::vehicle::ChShaftsDriveline4WD.
◆ LockCentralDifferential()
|
virtual |
Lock/unlock the specified central differential.
By convention, central differentials are counted from front to back, starting with index 0.
Reimplemented in chrono::vehicle::ChShaftsDriveline8WD, chrono::vehicle::ChShaftsDriveline6WD, chrono::vehicle::ChShaftsDriveline4WD, and chrono::vehicle::ChShaftsDriveline2WD.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/ChDrivelineWV.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/ChDrivelineWV.cpp