chrono::geometry::ChLineBezier Class Reference
Description
Geometric object representing a piecewise cubic Bezier curve in 3D.
#include <ChLineBezier.h>
Inheritance diagram for chrono::geometry::ChLineBezier:
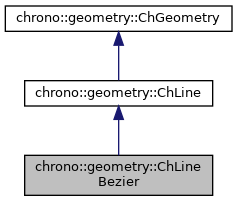
Collaboration diagram for chrono::geometry::ChLineBezier:
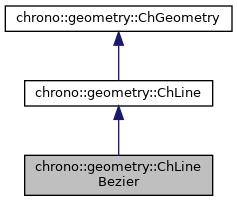
Public Member Functions | |
ChLineBezier (std::shared_ptr< ChBezierCurve > path) | |
ChLineBezier (const std::string &filename) | |
ChLineBezier (const ChLineBezier &source) | |
virtual ChLineBezier * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual Type | GetClassType () const override |
Get the class type as an enum. | |
virtual void | Set_closed (bool mc) override |
virtual void | Set_complexity (int mc) override |
virtual ChAABB | GetBoundingBox () const override |
Compute bounding in the frame of the Bezier curve knots. | |
virtual ChVector | Evaluate (double U) const override |
Return a point on the line, given parametric coordinate U (in [0,1]). | |
virtual void | ArchiveOut (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChLine (const ChLine &source) | |
virtual ChVector | GetTangent (double parU) const |
Return the tangent unit vector at the parametric coordinate U (in [0,1]). More... | |
virtual bool | Get_closed () const |
Tell if the curve is closed. | |
virtual int | Get_complexity () const |
Tell the complexity. | |
virtual int | GetManifoldDimension () const override |
This is a line. | |
bool | FindNearestLinePoint (ChVector<> &point, double &resU, double approxU, double tol) const |
Find the parameter resU for the nearest point on curve to "point". | |
virtual double | Length (int sampling) const |
Returns curve length. Typical sampling 1..5 (1 already gives correct result with degree1 curves) | |
virtual ChVector | GetEndA () const |
Return the start point of the line. More... | |
virtual ChVector | GetEndB () const |
Return the end point of the line. More... | |
double | CurveCurveDist (ChLine *compline, int samples) const |
Returns adimensional information on "how much" this curve is similar to another in its overall shape (does not matter parametrization or start point). More... | |
double | CurveSegmentDist (ChLine *complinesegm, int samples) const |
Same as before, but returns "how near" is complinesegm to whatever segment of this line (does not matter the percentual of line). More... | |
double | CurveCurveDistMax (ChLine *compline, int samples) const |
Same as above, but instead of making average of the distances, these functions return the maximum of the distances... | |
double | CurveSegmentDistMax (ChLine *complinesegm, int samples) const |
virtual bool | DrawPostscript (ChFile_ps *mfle, int markpoints, int bezier_interpolate) |
Draw into the current graph viewport of a ChFile_ps file. | |
![]() | |
ChGeometry (const ChGeometry &source) | |
void | InflateBoundingBox (ChAABB &bbox) const |
Enlarge the given existing bounding box with the bounding box of this object. | |
virtual double | GetBoundingSphereRadius () const |
Returns the radius of a bounding sphere for this geometry. More... | |
virtual ChVector | Baricenter () const |
Compute center of mass. | |
virtual void | Update () |
Generic update of internal data. | |
Additional Inherited Members | |
![]() | |
enum | Type { NONE, SPHERE, ELLIPSOID, BOX, CYLINDER, TRIANGLE, CAPSULE, CONE, LINE, LINE_ARC, LINE_BEZIER, LINE_CAM, LINE_PATH, LINE_POLY, LINE_SEGMENT, ROUNDED_BOX, ROUNDED_CYLINDER, TRIANGLEMESH, TRIANGLEMESH_CONNECTED, TRIANGLEMESH_SOUP } |
Enumeration of geometric object types. | |
![]() | |
bool | closed |
int | complexityU |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/geometry/ChLineBezier.h
- /builds/uwsbel/chrono/src/chrono/geometry/ChLineBezier.cpp