Description
Base class for all geometric objects representing lines in 3D space.
This is the base for all U-parametric object, implementing Evaluate() that returns a point as a function of the U parameter.
#include <ChLine.h>
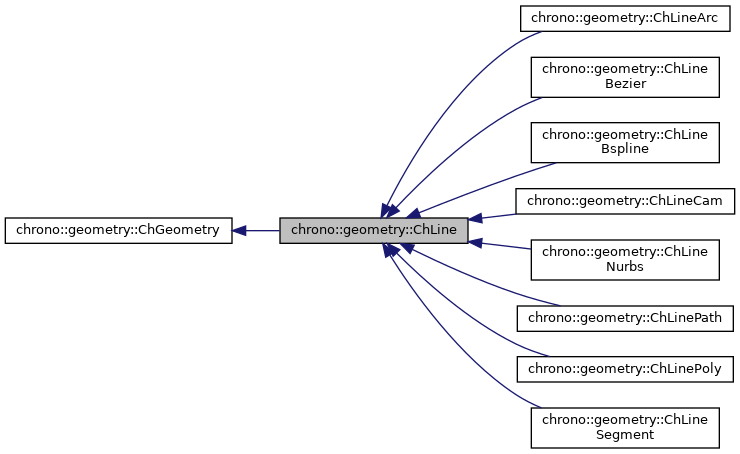
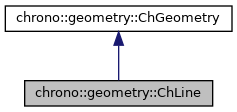
Public Member Functions | |
ChLine (const ChLine &source) | |
virtual Type | GetClassType () const override |
"Virtual" copy constructor (covariant return type). More... | |
virtual ChVector | Evaluate (double U) const =0 |
Return a point on the line, given parametric coordinate U. More... | |
virtual ChVector | GetTangent (double parU) const |
Return the tangent unit vector at the parametric coordinate U (in [0,1]). More... | |
virtual bool | Get_closed () const |
Tell if the curve is closed. | |
virtual void | Set_closed (bool mc) |
virtual int | Get_complexity () const |
Tell the complexity. | |
virtual void | Set_complexity (int mc) |
virtual int | GetManifoldDimension () const override |
This is a line. | |
bool | FindNearestLinePoint (ChVector<> &point, double &resU, double approxU, double tol) const |
Find the parameter resU for the nearest point on curve to "point". | |
virtual double | Length (int sampling) const |
Returns curve length. Typical sampling 1..5 (1 already gives correct result with degree1 curves) | |
virtual ChVector | GetEndA () const |
Return the start point of the line. More... | |
virtual ChVector | GetEndB () const |
Return the end point of the line. More... | |
double | CurveCurveDist (ChLine *compline, int samples) const |
Returns adimensional information on "how much" this curve is similar to another in its overall shape (does not matter parametrization or start point). More... | |
double | CurveSegmentDist (ChLine *complinesegm, int samples) const |
Same as before, but returns "how near" is complinesegm to whatever segment of this line (does not matter the percentual of line). More... | |
double | CurveCurveDistMax (ChLine *compline, int samples) const |
Same as above, but instead of making average of the distances, these functions return the maximum of the distances... | |
double | CurveSegmentDistMax (ChLine *complinesegm, int samples) const |
virtual bool | DrawPostscript (ChFile_ps *mfle, int markpoints, int bezier_interpolate) |
Draw into the current graph viewport of a ChFile_ps file. | |
virtual void | ArchiveOut (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
ChGeometry (const ChGeometry &source) | |
virtual ChGeometry * | Clone () const =0 |
"Virtual" copy constructor. | |
virtual ChAABB | GetBoundingBox () const |
Compute bounding box along the directions of the shape definition frame. More... | |
void | InflateBoundingBox (ChAABB &bbox) const |
Enlarge the given existing bounding box with the bounding box of this object. | |
virtual double | GetBoundingSphereRadius () const |
Returns the radius of a bounding sphere for this geometry. More... | |
virtual ChVector | Baricenter () const |
Compute center of mass. | |
virtual void | Update () |
Generic update of internal data. | |
Protected Attributes | |
bool | closed |
int | complexityU |
Additional Inherited Members | |
![]() | |
enum | Type { NONE, SPHERE, ELLIPSOID, BOX, CYLINDER, TRIANGLE, CAPSULE, CONE, LINE, LINE_ARC, LINE_BEZIER, LINE_CAM, LINE_PATH, LINE_POLY, LINE_SEGMENT, ROUNDED_BOX, ROUNDED_CYLINDER, TRIANGLEMESH, TRIANGLEMESH_CONNECTED, TRIANGLEMESH_SOUP } |
Enumeration of geometric object types. | |
Member Function Documentation
◆ CurveCurveDist()
double chrono::geometry::ChLine::CurveCurveDist | ( | ChLine * | compline, |
int | samples | ||
) | const |
Returns adimensional information on "how much" this curve is similar to another in its overall shape (does not matter parametrization or start point).
Try with 20 samples. The return value is somewhat the "average distance between the two curves". Note that the result is affected by "weight" of curves. If it chnges from default 1.0, the distance estimation is higher/lower (ex: if a curve defines low 'weight' in its central segment, its CurveCurveDistance from another segment is not much affected by errors near the central segment).
◆ CurveSegmentDist()
double chrono::geometry::ChLine::CurveSegmentDist | ( | ChLine * | complinesegm, |
int | samples | ||
) | const |
Same as before, but returns "how near" is complinesegm to whatever segment of this line (does not matter the percentual of line).
Again, this is affected by "weight" of curves. If weight changes along curves ->'weighted' distance
◆ Evaluate()
|
pure virtual |
Return a point on the line, given parametric coordinate U.
Parameter U always work in 0..1 range. The default implementation always returns the origin of the surface frame.
Implemented in chrono::geometry::ChLineCam, chrono::geometry::ChLineNurbs, chrono::geometry::ChLineBspline, chrono::geometry::ChLineArc, chrono::geometry::ChLineBezier, chrono::geometry::ChLinePath, chrono::geometry::ChLinePoly, and chrono::geometry::ChLineSegment.
◆ GetClassType()
|
inlineoverridevirtual |
"Virtual" copy constructor (covariant return type).
Get the class type as an enum.
Reimplemented from chrono::geometry::ChGeometry.
Reimplemented in chrono::geometry::ChLineCam, chrono::geometry::ChLineArc, chrono::geometry::ChLineBezier, chrono::geometry::ChLinePoly, chrono::geometry::ChLinePath, and chrono::geometry::ChLineSegment.
◆ GetEndA()
|
inlinevirtual |
Return the start point of the line.
By default, evaluates line at U=0.
Reimplemented in chrono::geometry::ChLinePath.
◆ GetEndB()
|
inlinevirtual |
Return the end point of the line.
By default, evaluates line at U=1.
Reimplemented in chrono::geometry::ChLinePath.
◆ GetTangent()
|
virtual |
Return the tangent unit vector at the parametric coordinate U (in [0,1]).
This default implementation uses finite differences.
Reimplemented in chrono::geometry::ChLineNurbs, and chrono::geometry::ChLineBspline.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/geometry/ChLine.h
- /builds/uwsbel/chrono/src/chrono/geometry/ChLine.cpp