Public Types |
Public Member Functions |
Static Public Attributes |
Protected Attributes |
List of all members
chrono::ChFile_ps Class Reference
Description
Class for postScript(TM) output.
Defines special file class ChFile_ps for EPS or PS output, i.e. Encapsulated PostScript or PostScript (TM) files including vectorial plots, graphs, lines, fonts, etc.
#include <ChFilePS.h>
Inheritance diagram for chrono::ChFile_ps:
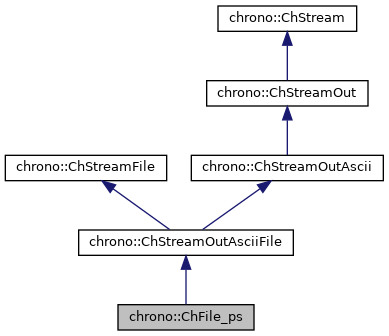
Collaboration diagram for chrono::ChFile_ps:
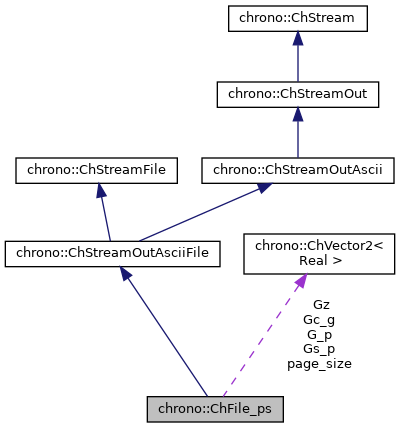
Public Types | |
enum | Justification { LEFT, RIGHT, CENTER } |
enum | Space { PAGE, GRAPH } |
![]() | |
enum | eChMode { CHFILE_NORMAL = 0, CHFILE_NOWRITE, CHFILE_SAFEWRITE, CHFILE_OPENLATER } |
[Obsolete] Modes for chrono files (the ch-modes). More... | |
enum | eChStreamError { CHSTREAM_OK = 0, CHSTREAM_EOF, CHSTREAM_FAIL } |
Errors for chrono files (the ch-modes). More... | |
Public Member Functions | |
ChFile_ps (const std::string &filename, double x=1, double y=1, double w=20, double h=29, const std::string &prolog_filename="prolog.ps") | |
Constructor, with optional position and size of bounding box. | |
~ChFile_ps () | |
Destructor. Write trailer to PS file and close it. | |
const ChVector2 & | Get_G_p () const |
Get viewport position of left-lower graph corner, in page space. | |
const ChVector2 & | Get_Gs_p () const |
Get viewport width/height of graph, in page space. | |
const ChVector2 & | Get_Gc_g () const |
Get viewport center, in 2d graph space;. | |
const ChVector2 & | Get_Gz () const |
Get viewport graph zoom factor: | |
void | Set_G_p (ChVector2<> mv) |
Set viewport position of left-lower graph corner, in page space. | |
void | Set_Gs_p (ChVector2<> mv) |
Set viewport width/height of graph, in page space. | |
void | Set_Gc_g (ChVector2<> mv) |
Set viewport center, in 2d graph space;. | |
void | Set_Gz (double mz) |
Set viewport graph zoom factor: | |
void | Set_Gz (ChVector2<> mz) |
Set viewport graph zoom factor: | |
void | Set_ZoomPan_by_fit (double Xgmin, double Xgmax, double Ygmin, double Ygmax) |
Set viewport zoom and pan (center) given the max/min extension in graph space. | |
ChVector2 | To_page_from_graph (ChVector2<> mv_g) const |
Transform position from 'page space' to 'graph viewport space'. | |
ChVector2 | To_graph_from_page (ChVector2<> mv_p) const |
Transform position from 'graph viewport space' to 'page space',. | |
ChVector2 | TransPt (ChVector2<> mfrom, Space space) const |
void | SetGray (double mf) |
Sets the gray level of next drawn item, 0..1. | |
void | SetWidth (double mf) |
Sets the width of next drawn line. | |
void | SetLinecap (double mf) |
Sets the linecap. | |
void | SetRGB (double r, double g, double b) |
Sets the RGB color of next drawn item. | |
void | SetRGB (ChFile_ps_color mc) |
Sets the color of next drawn item. | |
void | SetFont (char *name, double size) |
Sets the font of next drawn text (note: must use PS embedded fonts like "Times-Roman", etc.) | |
void | CustomPsCommand (char *command) |
void | MoveTo (ChVector2<> mp) |
void | StartLine () |
void | AddLinePoint (ChVector2<> mp) |
void | CloseLine () |
void | PaintStroke () |
void | PaintFill () |
void | Clip () |
void | GrSave () |
void | GrRestore () |
void | DrawPoint (ChVector2<> mfrom, Space space) |
Draws a single "dot" point,. | |
void | DrawLine (ChVector2<> mfrom, ChVector2<> mto, Space space) |
Draws line from point to point,. | |
void | DrawRectangle (ChVector2<> mfrom, ChVector2<> mwh, Space space, bool filled) |
Draws rectangle from point to point. | |
void | ClipRectangle (ChVector2<> mfrom, ChVector2<> mwh, Space space) |
Sets clip rectangle draw region, from point to point (remember GrSave() and GrRestore() before and later..) | |
void | ClipToGraph () |
Sets clip rectangle as graph region (remember GrSave() and GrRestore() before and later..) | |
void | DrawText (ChVector2<> mfrom, char *string, Space space, Justification justified=Justification::LEFT) |
Draw text at given position. | |
void | DrawText (ChVector2<> mfrom, double number, Space space, Justification justified=Justification::LEFT) |
Draw number at given position. | |
void | DrawGraphAxes (ChFile_ps_graph_setting *msetting) |
Draw the x/y axes for the graph viewport. | |
void | DrawGraphXY (const ChArray<> &Yvalues, const ChArray<> &Xvalues) |
Draw a line into graph viewport, where the xy coords are stored in two separate arrays: | |
void | DrawGraphXY (const ChArray<> &Yvalues, double Xfrom, double Xstep) |
Draw a line into graph viewport, where the y coords are into an array and x coords run form Xfrom... More... | |
void | DrawGraphLabel (double dx, double dy, double fontsize, char *label, int dolinesample, bool background=true, double backwidth=3.0, ChFile_ps_color bkgndcolor=ChFile_ps_color::WHITE) |
Draws a text label (with "fontsize" size) with potion relative to low-left corner of graph. More... | |
![]() | |
ChStreamOutAsciiFile (const std::string &filename, std::ios::openmode mode=std::ios::trunc) | |
![]() | |
ChStreamFile (const std::string &filename, std::ios::openmode mode) | |
Creates and open a file, given the filename on disk and the opening mode. More... | |
virtual | ~ChStreamFile () |
Destruction means that the file stream is also closed. | |
virtual void | Flush () |
Synchronizes the associated stream buffer with its controlled output sequence. | |
virtual void | Write (const char *data, size_t n) |
Writes to file, up to n chars. More... | |
virtual void | Read (char *data, size_t n) |
Reads from file, up to n chars. More... | |
virtual bool | End_of_stream () const |
Returns true if end of stream reached. | |
std::fstream & | GetFstream () |
Reference to fstream encapsulated here. | |
![]() | |
ChStreamOutAscii & | operator<< (bool bVal) |
Generic << operator for all classes which implement serialization by means of a method named 'void StreamOut(ChStreamOutAscii&)'. | |
ChStreamOutAscii & | operator<< (char tch) |
ChStreamOutAscii & | operator<< (const int nVal) |
ChStreamOutAscii & | operator<< (const double dVal) |
ChStreamOutAscii & | operator<< (const float fVal) |
ChStreamOutAscii & | operator<< (unsigned int unVal) |
ChStreamOutAscii & | operator<< (char *str) |
ChStreamOutAscii & | operator<< (const char *str) |
ChStreamOutAscii & | operator<< (const std::string &str) |
ChStreamOutAscii & | operator<< (unsigned long unVal) |
ChStreamOutAscii & | operator<< (unsigned long long unVal) |
template<class T > | |
ChStreamOutAscii & | operator< (T obj) |
OBSOLETE use ChArchive << operator and ArchiveOut() | |
virtual void | Format (char *formatString,...) |
Output the log message in form of a formatted string, like in printf(). | |
void | SetNumFormat (const char *mf) |
Set the formatting string (ex "%f" or "%g" etc.) for float->text conversion. | |
char * | GetNumFormat () |
void | SetCommentTrailer (char *mt) |
Set the trailer symbol before each comment (example: "#" , or "//"). | |
void | CR () |
Outputs carriage return. | |
void | TAB () |
Output tab. | |
void | Comment (char m_string[]) |
Output comment with preceding trailer (e.g., #). | |
void | Bar () |
Output a separation bar in ASCII file. | |
Static Public Attributes | |
static const double | PS_SCALE_CENTIMETERS = 28.3476 |
static const double | PS_SCALE_INCHES = 72.0 |
Protected Attributes | |
double | unit_scale |
ChVector2 | page_size |
ChVector2 | G_p |
ChVector2 | Gs_p |
ChVector2 | Gc_g |
ChVector2 | Gz |
std::string | prolog_file |
![]() | |
char | number_format [10] |
char | comment_trailer [10] |
Additional Inherited Members |
Member Function Documentation
◆ DrawGraphLabel()
void chrono::ChFile_ps::DrawGraphLabel | ( | double | dx, |
double | dy, | ||
double | fontsize, | ||
char * | label, | ||
int | dolinesample, | ||
bool | background = true , |
||
double | backwidth = 3.0 , |
||
ChFile_ps_color | bkgndcolor = ChFile_ps_color::WHITE |
||
) |
Draws a text label (with "fontsize" size) with potion relative to low-left corner of graph.
If "dolinesample= true", also draws a little horizontal sample of line with current width/color on the left of the label.
◆ DrawGraphXY()
void chrono::ChFile_ps::DrawGraphXY | ( | const ChArray<> & | Yvalues, |
double | Xfrom, | ||
double | Xstep | ||
) |
Draw a line into graph viewport, where the y coords are into an array and x coords run form Xfrom...
with steps Xsteps-
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/core/ChFilePS.h
- /builds/uwsbel/chrono/src/chrono/core/ChFilePS.cpp