Description
Class for a generic ED finite element node, with x,y,z displacement and a 3D rotation.
This is the typical node that can be used for beams, etc.
#include <ChNodeFEAxyzrot.h>
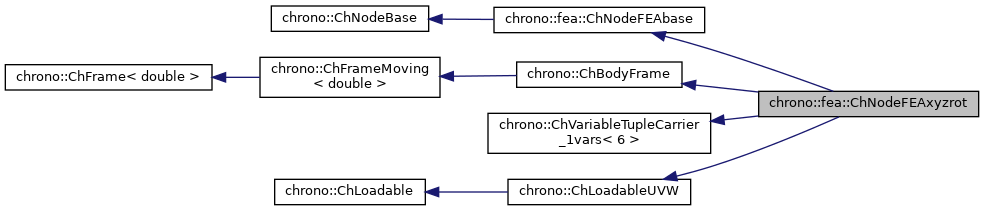
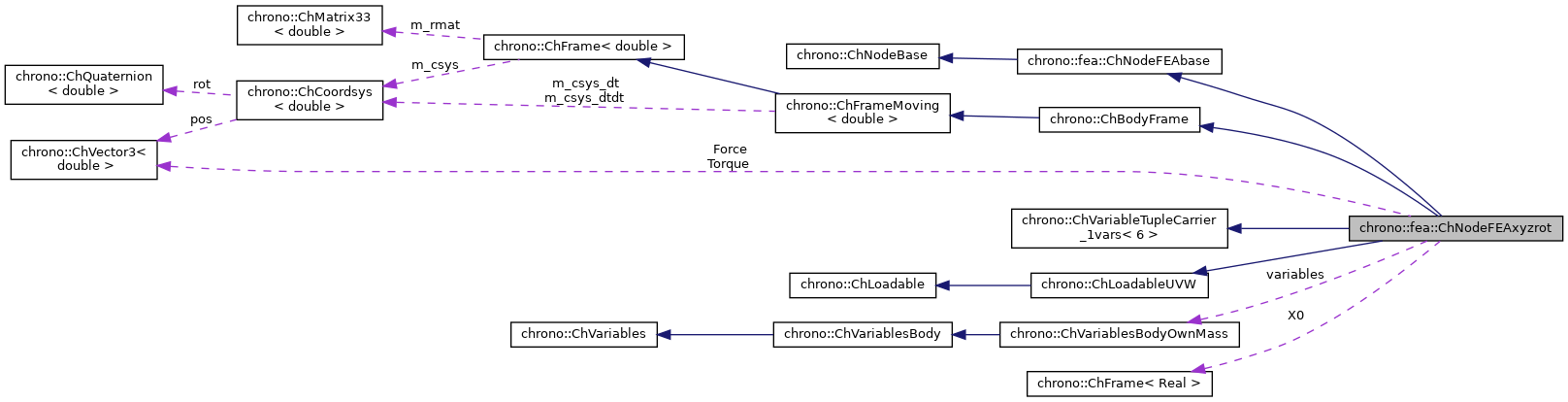
Public Member Functions | |
ChNodeFEAxyzrot (ChFrame<> initialf=ChFrame<>()) | |
ChNodeFEAxyzrot (const ChNodeFEAxyzrot &other) | |
ChNodeFEAxyzrot & | operator= (const ChNodeFEAxyzrot &other) |
virtual ChVariables & | Variables () override |
Return a reference to the encapsulated variables, representing states (pos, speed or accel.) and forces. | |
virtual void | Relax () override |
Set the rest position as the actual position. | |
virtual void | ForceToRest () override |
Reset to no speed and acceleration. | |
virtual void | SetFixed (bool fixed) override |
Fix/release this node. More... | |
virtual bool | IsFixed () const override |
Return true if the node is fixed (i.e., its state variables are not changed by the solver). | |
double | GetMass () const |
Get atomic mass of the node. | |
void | SetMass (double m) |
Set atomic mass of the node. | |
ChMatrix33 & | GetInertia () |
Access atomic inertia of the node. | |
void | SetX0 (const ChFrame<> &mx) |
Set the initial (reference) frame. | |
const ChFrame & | GetX0 () const |
Get the initial (reference) frame. | |
ChFrame & | GetX0ref () |
Access the initial (reference) frame. | |
void | SetForce (const ChVector3d &frc) |
Set the 3d applied force, in absolute reference. | |
const ChVector3d & | GetForce () const |
Get the 3d applied force, in absolute reference. | |
void | SetTorque (const ChVector3d &trq) |
Set the 3d applied torque, in node reference. | |
const ChVector3d & | GetTorque () const |
Get the 3d applied torque, in node reference. | |
ChFrameMoving & | Frame () |
Access the frame of the node - in absolute csys, with infos on actual position, speed, acceleration, etc. | |
virtual unsigned int | GetNumCoordsPosLevel () const override |
Get the number of degrees of freedom (7 because quaternion for rotation). | |
virtual unsigned int | GetNumCoordsVelLevel () const override |
Get the number of degrees of freedom, derivative (6 because angular velocity for rotation derivative). | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow de-serialization of transient data from archives. | |
virtual ChVariables * | GetVariables1 () override |
virtual void | NodeIntStateGather (const unsigned int off_x, ChState &x, const unsigned int off_v, ChStateDelta &v, double &T) override |
virtual void | NodeIntStateScatter (const unsigned int off_x, const ChState &x, const unsigned int off_v, const ChStateDelta &v, const double T) override |
virtual void | NodeIntStateGatherAcceleration (const unsigned int off_a, ChStateDelta &a) override |
virtual void | NodeIntStateScatterAcceleration (const unsigned int off_a, const ChStateDelta &a) override |
virtual void | NodeIntStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) override |
virtual void | NodeIntStateGetIncrement (const unsigned int off_x, const ChState &x_new, const ChState &x, const unsigned int off_v, ChStateDelta &Dv) override |
virtual void | NodeIntLoadResidual_F (const unsigned int off, ChVectorDynamic<> &R, const double c) override |
virtual void | NodeIntLoadResidual_Mv (const unsigned int off, ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) override |
virtual void | NodeIntLoadLumpedMass_Md (const unsigned int off, ChVectorDynamic<> &Md, double &error, const double c) override |
virtual void | NodeIntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R) override |
virtual void | NodeIntFromDescriptor (const unsigned int off_v, ChStateDelta &v) override |
virtual void | InjectVariables (ChSystemDescriptor &descriptor) override |
Register with the given system descriptor any ChVariable objects associated with this item. | |
virtual void | VariablesFbReset () override |
Set the 'fb' part (the known term) of the encapsulated ChVariables to zero. | |
virtual void | VariablesFbLoadForces (double factor=1) override |
Add the current forces (applied to node) into the encapsulated ChVariables. More... | |
virtual void | VariablesQbLoadSpeed () override |
Initialize the 'qb' part of the ChVariables with the current value of speeds. | |
virtual void | VariablesQbSetSpeed (double step=0) override |
Fetch the item speed (ex. More... | |
virtual void | VariablesFbIncrementMq () override |
Add M*q (masses multiplied current 'qb') to Fb, ex. More... | |
virtual void | VariablesQbIncrementPosition (double step) override |
Increment node positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor. More... | |
virtual unsigned int | GetLoadableNumCoordsPosLevel () override |
Gets the number of DOFs affected by this element (position part) | |
virtual unsigned int | GetLoadableNumCoordsVelLevel () override |
Gets the number of DOFs affected by this element (speed part) | |
virtual void | LoadableGetStateBlockPosLevel (int block_offset, ChState &mD) override |
Gets all the DOFs packed in a single vector (position part) | |
virtual void | LoadableGetStateBlockVelLevel (int block_offset, ChStateDelta &mD) override |
Gets all the DOFs packed in a single vector (speed part) | |
virtual void | LoadableStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) override |
Increment all DOFs using a delta. | |
virtual unsigned int | GetNumFieldCoords () override |
Number of coordinates in the interpolated field, ex=3 for a tetrahedron finite element or a cable, etc. More... | |
virtual unsigned int | GetNumSubBlocks () override |
Get the number of DOFs sub-blocks. | |
virtual unsigned int | GetSubBlockOffset (unsigned int nblock) override |
Get the offset of the specified sub-block of DOFs in global vector. | |
virtual unsigned int | GetSubBlockSize (unsigned int nblock) override |
Get the size of the specified sub-block of DOFs in global vector. | |
virtual bool | IsSubBlockActive (unsigned int nblock) const override |
Check if the specified sub-block of DOFs is active. | |
virtual void | LoadableGetVariables (std::vector< ChVariables * > &mvars) override |
Get the pointers to the contained ChVariables, appending to the mvars vector. | |
virtual void | ComputeNF (const double U, const double V, const double W, ChVectorDynamic<> &Qi, double &detJ, const ChVectorDynamic<> &F, ChVectorDynamic<> *state_x, ChVectorDynamic<> *state_w) override |
Evaluate Q=N'*F, for Q generalized lagrangian load, where N is some type of matrix evaluated at point P(U,V,W) assumed in absolute coordinates, and F is a load assumed in absolute coordinates. More... | |
virtual double | GetDensity () override |
This is not needed because not used in quadrature. | |
![]() | |
virtual void | SetIndex (unsigned int mindex) |
Sets the global index of the node. | |
virtual unsigned int | GetIndex () |
Gets the global index of the node. | |
virtual void | SetupInitial (ChSystem *system) |
Initial setup. | |
![]() | |
ChNodeBase (const ChNodeBase &other) | |
ChNodeBase & | operator= (const ChNodeBase &other) |
virtual unsigned int | GetNumCoordsPosLevelActive () const |
Get the actual number of active degrees of freedom. More... | |
virtual unsigned int | GetNumCoordsVelLevelActive () const |
Get the actual number of active degrees of freedom, derivative. More... | |
virtual bool | IsAllCoordsActive () const |
Return true if all node DOFs are active (no node variable is fixed). | |
unsigned int | NodeGetOffsetPosLevel () |
Get offset in the state vector (position part). | |
unsigned int | NodeGetOffsetVelLevel () |
Get offset in the state vector (speed part). | |
void | NodeSetOffsetPosLevel (const unsigned int moff) |
Set offset in the state vector (position part). | |
void | NodeSetOffsetVelLevel (const unsigned int moff) |
Set offset in the state vector (speed part). | |
![]() | |
ChBodyFrame (const ChBodyFrame &other) | |
ChWrenchd | AppliedForceLocalToWrenchParent (const ChVector3d &force, const ChVector3d &appl_point) |
Transform a force applied to a point on the body to a force and moment at the frame origin. More... | |
ChWrenchd | AppliedForceParentToWrenchParent (const ChVector3d &force, const ChVector3d &appl_point) |
Transform a force applied to a point on the body to a force and moment at the frame origin. More... | |
![]() | |
ChFrameMoving () | |
Default constructor (identity frame). | |
ChFrameMoving (const ChVector3< double > &v, const ChQuaternion< double > &q=ChQuaternion< double >(1, 0, 0, 0)) | |
Construct from pos and rot (as a quaternion). | |
ChFrameMoving (const ChVector3< double > &v, const ChMatrix33< double > &R) | |
Construct from pos and rotation (as a 3x3 matrix). | |
ChFrameMoving (const ChCoordsys< double > &C) | |
Construct from a coordsys. | |
ChFrameMoving (const ChFrame< double > &F) | |
Construct from a frame. | |
ChFrameMoving (const ChFrameMoving< double > &other) | |
Copy constructor, build from another moving frame. | |
virtual | ~ChFrameMoving () |
Destructor. | |
ChFrameMoving< double > & | operator= (const ChFrameMoving< double > &other) |
Assignment operator: copy from another moving frame. | |
ChFrameMoving< double > & | operator= (const ChFrame< double > &other) |
Assignment operator: copy from another frame. | |
bool | operator== (const ChFrameMoving< double > &other) const |
Returns true for identical frames. | |
bool | operator!= (const ChFrameMoving< double > &other) const |
Returns true for different frames. | |
ChFrameMoving< double > | operator>> (const ChFrameMoving< double > &F) const |
Transform another frame through this frame. More... | |
ChFrameMoving< double > | operator* (const ChFrameMoving< double > &F) const |
Transform another frame through this frame. More... | |
ChFrameMoving< double > & | operator>>= (const ChFrameMoving< double > &F) |
Transform this frame by pre-multiplication with another frame. More... | |
ChFrameMoving< double > & | operator>>= (const ChVector3< double > &v) |
Transform this frame by pre-multiplication with a given vector (translate frame). | |
ChFrameMoving< double > & | operator>>= (const ChQuaternion< double > &q) |
Transform this frame by pre-multiplication with a given quaternion (rotate frame). | |
ChFrameMoving< double > & | operator>>= (const ChCoordsys< double > &C) |
Transform this frame by pre-multiplication with a given coordinate system. | |
ChFrameMoving< double > & | operator>>= (const ChFrame< double > &F) |
Transform this frame by pre-multiplication with another frame. | |
ChFrameMoving< double > & | operator*= (const ChFrameMoving< double > &F) |
Transform this frame by post-multiplication with another frame. More... | |
const ChCoordsys< double > & | GetCoordsysDt () const |
Return both rotation and translation velocities as a ChCoordsys object. | |
const ChCoordsys< double > & | GetCoordsysDt2 () const |
Return both rotation and translation accelerations as a ChCoordsys object. | |
const ChVector3< double > & | GetPosDt () const |
Return the linear velocity. | |
const ChVector3< double > & | GetLinVel () const |
Return the linear velocity. | |
const ChVector3< double > & | GetPosDt2 () const |
Return the linear acceleration. | |
const ChVector3< double > & | GetLinAcc () const |
Return the linear acceleration. | |
const ChQuaternion< double > & | GetRotDt () const |
Return the rotation velocity as a quaternion. | |
const ChQuaternion< double > & | GetRotDt2 () const |
Return the rotation acceleration as a quaternion. | |
ChVector3< double > | GetAngVelLocal () const |
Compute the angular velocity (expressed in local coords). | |
ChVector3< double > | GetAngVelParent () const |
Compute the actual angular velocity (expressed in parent coords). | |
ChVector3< double > | GetAngAccLocal () const |
Compute the actual angular acceleration (expressed in local coords). | |
ChVector3< double > | GetAngAccParent () const |
Compute the actual angular acceleration (expressed in parent coords). | |
virtual void | SetCoordsysDt (const ChCoordsys< double > &csys_dt) |
Set both linear and rotation velocities as a single ChCoordsys derivative. | |
virtual void | SetPosDt (const ChVector3< double > &vel) |
Set the linear velocity. | |
virtual void | SetLinVel (const ChVector3< double > &vel) |
Set the linear velocity. | |
virtual void | SetRotDt (const ChQuaternion< double > &q_dt) |
Set the rotation velocity as a quaternion derivative. More... | |
virtual void | SetAngVelLocal (const ChVector3< double > &w) |
Set the rotation velocity from the given angular velocity (expressed in local coordinates). | |
virtual void | SetAngVelParent (const ChVector3< double > &w) |
Set the rotation velocity from given angular velocity (expressed in parent coordinates). | |
virtual void | SetCoordsysDt2 (const ChCoordsys< double > &csys_dtdt) |
Set the linear and rotation accelerations as a single ChCoordsys derivative. | |
virtual void | SetPosDt2 (const ChVector3< double > &acc) |
Set the linear acceleration. | |
virtual void | SetLinAcc (const ChVector3< double > &acc) |
Set the linear acceleration. | |
virtual void | SetRotDt2 (const ChQuaternion< double > &q_dtdt) |
Set the rotation acceleration as a quaternion derivative. More... | |
virtual void | SetAngAccLocal (const ChVector3< double > &a) |
Set the rotation acceleration from given angular acceleration (expressed in local coordinates). More... | |
virtual void | SetAngAccParent (const ChVector3< double > &a) |
Set the rotation acceleration from given angular acceleration (expressed in parent coordinates). | |
void | ComputeRotMatDt (ChMatrix33< double > &R_dt) const |
Compute the time derivative of the rotation matrix. | |
void | ComputeRotMatDt2 (ChMatrix33< double > &R_dtdt) |
Compute the second time derivative of the rotation matrix. | |
ChMatrix33< double > | GetRotMatDt () |
Return the time derivative of the rotation matrix. | |
ChMatrix33< double > | GetRotMatDt2 () |
Return the second time derivative of the rotation matrix. | |
void | ConcatenatePreTransformation (const ChFrameMoving< double > &F) |
Apply a transformation (rotation and translation) represented by another frame. More... | |
void | ConcatenatePostTransformation (const ChFrameMoving< double > &F) |
Apply a transformation (rotation and translation) represented by another frame F in local coordinate. More... | |
ChVector3< double > | PointSpeedLocalToParent (const ChVector3< double > &localpos) const |
Return the velocity in the parent frame of a point fixed to this frame and expressed in local coordinates. | |
ChVector3< double > | PointSpeedLocalToParent (const ChVector3< double > &localpos, const ChVector3< double > &localspeed) const |
Return the velocity in the parent frame of a moving point, given the point location and velocity expressed in local coordinates. | |
ChVector3< double > | PointAccelerationLocalToParent (const ChVector3< double > &localpos) const |
Return the acceleration in the parent frame of a point fixed to this frame and expressed in local coordinates. More... | |
ChVector3< double > | PointAccelerationLocalToParent (const ChVector3< double > &localpos, const ChVector3< double > &localspeed, const ChVector3< double > &localacc) const |
Return the acceleration in the parent frame of a moving point, given the point location, velocity, and acceleration expressed in local coordinates. | |
ChVector3< double > | PointSpeedParentToLocal (const ChVector3< double > &parentpos, const ChVector3< double > &parentspeed) const |
Return the velocity of a point expressed in this frame, given the point location and velocity in the parent frame. | |
ChVector3< double > | PointAccelerationParentToLocal (const ChVector3< double > &parentpos, const ChVector3< double > &parentspeed, const ChVector3< double > &parentacc) const |
Return the acceleration of a point expressed in this frame, given the point location, velocity, and acceleration in the parent frame. | |
ChFrameMoving< double > | TransformLocalToParent (const ChFrameMoving< double > &F) const |
Transform a moving frame from 'this' local coordinate system to parent frame coordinate system. | |
ChFrameMoving< double > | TransformParentToLocal (const ChFrameMoving< double > &F) const |
Transform a moving frame from the parent coordinate system to 'this' local frame coordinate system. | |
bool | Equals (const ChFrameMoving< double > &other) const |
Returns true if this transform is identical to the other transform. | |
bool | Equals (const ChFrameMoving< double > &other, double tol) const |
Returns true if this transform is equal to the other transform, within a tolerance 'tol'. | |
virtual void | Invert () override |
Invert in place. More... | |
ChFrameMoving< double > | GetInverse () const |
Return the inverse transform. | |
![]() | |
ChFrame () | |
Default constructor (identity frame). | |
ChFrame (const ChVector3< double > &v, const ChQuaternion< double > &q=ChQuaternion< double >(1, 0, 0, 0)) | |
Construct from position and rotation (as quaternion). | |
ChFrame (const ChVector3< double > &v, const ChMatrix33< double > &R) | |
Construct from pos and rotation (as a 3x3 matrix). | |
ChFrame (const ChVector3< double > &v, const double angle, const ChVector3< double > &u) | |
Construct from position mv and rotation of angle alpha around unit vector mu. | |
ChFrame (const ChCoordsys< double > &C) | |
Construct from a coordsys. | |
ChFrame (const ChFrame< double > &other) | |
Copy constructor, build from another frame. | |
ChFrame< double > & | operator= (const ChFrame< double > &other) |
Assignment operator: copy from another frame. | |
bool | operator== (const ChFrame< double > &other) const |
Returns true for identical frames. | |
bool | operator!= (const ChFrame< double > &other) const |
Returns true for different frames. | |
ChFrame< double > | operator* (const ChFrame< double > &F) const |
Transform another frame through this frame. More... | |
ChVector3< double > | operator* (const ChVector3< double > &v) const |
Transform a vector through this frame (express in parent frame). More... | |
ChFrame< double > | operator>> (const ChFrame< double > &F) const |
Transform another frame through this frame. More... | |
ChVector3< double > | operator/ (const ChVector3< double > &v) const |
Transform a vector through this frame (express from parent frame). More... | |
ChFrame< double > & | operator>>= (const ChFrame< double > &F) |
Transform this frame by pre-multiplication with another frame. More... | |
ChFrame< double > & | operator>>= (const ChVector3< double > &v) |
Transform this frame by pre-multiplication with a given vector (translate frame). | |
ChFrame< double > & | operator>>= (const ChQuaternion< double > &q) |
Transform this frame by pre-multiplication with a given quaternion (rotate frame). | |
ChFrame< double > & | operator>>= (const ChCoordsys< double > &C) |
Transform this frame by pre-multiplication with a given coordinate system. | |
ChFrame< double > & | operator*= (const ChFrame< double > &F) |
Transform this frame by post-multiplication with another frame. More... | |
const ChCoordsys< double > & | GetCoordsys () const |
Return both current rotation and translation as a ChCoordsys object. | |
const ChVector3< double > & | GetPos () const |
Return the current translation vector. | |
const ChQuaternion< double > & | GetRot () const |
Return the current rotation quaternion. | |
const ChMatrix33< double > & | GetRotMat () const |
Return the current 3x3 rotation matrix. | |
ChVector3< double > | GetRotAxis () const |
Get axis of finite rotation, in parent space. | |
double | GetRotAngle () const |
Get angle of rotation about axis of finite rotation. | |
virtual void | SetCoordsys (const ChCoordsys< double > &C) |
Impose both translation and rotation as a single ChCoordsys. More... | |
virtual void | SetCoordsys (const ChVector3< double > &v, const ChQuaternion< double > &q) |
Impose both translation and rotation. More... | |
virtual void | SetRot (const ChQuaternion< double > &q) |
Impose the rotation as a quaternion. More... | |
virtual void | SetRot (const ChMatrix33< double > &R) |
Impose the rotation as a 3x3 matrix. More... | |
virtual void | SetPos (const ChVector3< double > &pos) |
Impose the translation vector. | |
void | ConcatenatePreTransformation (const ChFrame< double > &F) |
Apply a transformation (rotation and translation) represented by another frame. More... | |
void | ConcatenatePostTransformation (const ChFrame< double > &F) |
Apply a transformation (rotation and translation) represented by another frame F in local coordinate. More... | |
void | Move (const ChVector3< double > &v) |
An easy way to move the frame by the amount specified by vector v, (assuming v expressed in parent coordinates) | |
void | Move (const ChCoordsys< double > &C) |
Apply both translation and rotation, assuming both expressed in parent coordinates, as a vector for translation and quaternion for rotation,. | |
ChVector3< double > | TransformPointLocalToParent (const ChVector3< double > &v) const |
Transform a point from the local frame coordinate system to the parent coordinate system. | |
ChVector3< double > | TransformPointParentToLocal (const ChVector3< double > &v) const |
Transforms a point from the parent coordinate system to local frame coordinate system. | |
ChVector3< double > | TransformDirectionLocalToParent (const ChVector3< double > &d) const |
Transform a direction from the parent frame coordinate system to 'this' local coordinate system. | |
ChVector3< double > | TransformDirectionParentToLocal (const ChVector3< double > &d) const |
Transforms a direction from 'this' local coordinate system to parent frame coordinate system. | |
ChWrench< double > | TransformWrenchLocalToParent (const ChWrench< double > &w) const |
Transform a wrench from the local coordinate system to the parent coordinate system. | |
ChWrench< double > | TransformWrenchParentToLocal (const ChWrench< double > &w) const |
Transform a wrench from the parent coordinate system to the local coordinate system. | |
ChFrame< double > | TransformLocalToParent (const ChFrame< double > &F) const |
Transform a frame from 'this' local coordinate system to parent frame coordinate system. | |
ChFrame< double > | TransformParentToLocal (const ChFrame< double > &F) const |
Transform a frame from the parent coordinate system to 'this' local frame coordinate system. | |
bool | Equals (const ChFrame< double > &other) const |
Returns true if this transform is identical to the other transform. | |
bool | Equals (const ChFrame< double > &other, double tol) const |
Returns true if this transform is equal to the other transform, within a tolerance 'tol'. | |
void | Normalize () |
Normalize the rotation, so that quaternion has unit length. | |
virtual void | SetIdentity () |
Sets to no translation and no rotation. | |
ChFrame< double > | GetInverse () const |
Return the inverse transform. | |
![]() | |
virtual bool | IsTetrahedronIntegrationNeeded () |
If true, use quadrature over u,v,w in [0..1] range as tetrahedron volumetric coords (with z=1-u-v-w) otherwise use default quadrature over u,v,w in [-1..+1] as box isoparametric coords. | |
virtual bool | IsTrianglePrismIntegrationNeeded () |
If true, use quadrature over u,v in [0..1] range as triangle natural coords (with z=1-u-v), and use linear quadrature over w in [-1..+1], otherwise use default quadrature over u,v,w in [-1..+1] as box isoparametric coords. | |
Protected Attributes | |
ChVariablesBodyOwnMass | variables |
3D node variables, with x,y,z displ. and 3D rot. | |
ChFrame | X0 |
reference frame | |
ChVector3d | Force |
applied force | |
ChVector3d | Torque |
applied torque | |
![]() | |
unsigned int | g_index |
global node index | |
![]() | |
unsigned int | offset_x |
offset in vector of state (position part) | |
unsigned int | offset_w |
offset in vector of state (speed part) | |
![]() | |
ChCoordsys< double > | m_csys_dt |
rotation and position velocity, as vector + quaternion | |
ChCoordsys< double > | m_csys_dtdt |
rotation and position acceleration, as vector + quaternion | |
![]() | |
ChCoordsys< double > | m_csys |
position and rotation, as vector + quaternion | |
ChMatrix33< double > | m_rmat |
3x3 orthogonal rotation matrix | |
Additional Inherited Members | |
![]() | |
typedef ChConstraintTuple_1vars< ChVariableTupleCarrier_1vars< N1 > > | type_constraint_tuple |
![]() | |
double | m_TotalMass |
Nodal mass obtained from element mass matrix. | |
![]() | |
static const int | nvars1 |
Member Function Documentation
◆ ComputeNF()
|
overridevirtual |
Evaluate Q=N'*F, for Q generalized lagrangian load, where N is some type of matrix evaluated at point P(U,V,W) assumed in absolute coordinates, and F is a load assumed in absolute coordinates.
det[J] is unused.
- Parameters
-
U x coordinate of application point in absolute space V y coordinate of application point in absolute space W z coordinate of application point in absolute space Qi Return result of N'*F here, maybe with offset block_offset detJ Return det[J] here F Input F vector, size is 6, it is {Force,Torque} both in absolute coords. state_x if != 0, update state (pos. part) to this, then evaluate Q state_w if != 0, update state (speed part) to this, then evaluate Q
Implements chrono::ChLoadableUVW.
◆ GetNumFieldCoords()
|
inlineoverridevirtual |
Number of coordinates in the interpolated field, ex=3 for a tetrahedron finite element or a cable, etc.
Here is 6: xyz displ + xyz rots
Implements chrono::ChLoadable.
◆ SetFixed()
|
overridevirtual |
Fix/release this node.
If fixed, its state variables are not changed by the solver.
Implements chrono::fea::ChNodeFEAbase.
◆ VariablesFbIncrementMq()
|
overridevirtual |
Add M*q (masses multiplied current 'qb') to Fb, ex.
if qb is initialized with v_old using VariablesQbLoadSpeed, this method can be used in timestepping schemes that do: M*v_new = M*v_old + forces*dt
Reimplemented from chrono::ChNodeBase.
◆ VariablesFbLoadForces()
|
overridevirtual |
Add the current forces (applied to node) into the encapsulated ChVariables.
Include in the 'fb' part: qf+=forces*factor
Reimplemented from chrono::ChNodeBase.
◆ VariablesQbIncrementPosition()
|
overridevirtual |
Increment node positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor.
pos+=qb*step If qb is a speed, this behaves like a single step of 1-st order numerical integration (Euler integration).
Reimplemented from chrono::ChNodeBase.
◆ VariablesQbSetSpeed()
|
overridevirtual |
Fetch the item speed (ex.
linear velocity, in xyz nodes) from the 'qb' part of the ChVariables and sets it as the current item speed. If 'step' is not 0, also should compute the approximate acceleration of the item using backward differences, that is accel=(new_speed-old_speed)/step. Mostly used after the solver provided the solution in ChVariables.
Reimplemented from chrono::ChNodeBase.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChNodeFEAxyzrot.h
- /builds/uwsbel/chrono/src/chrono/fea/ChNodeFEAxyzrot.cpp