Description
Base class for Chrono::Multicore systems.
#include <ChSystemMulticore.h>
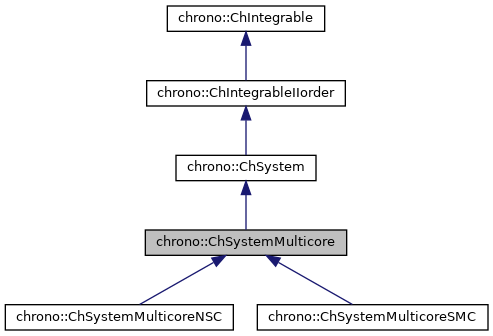
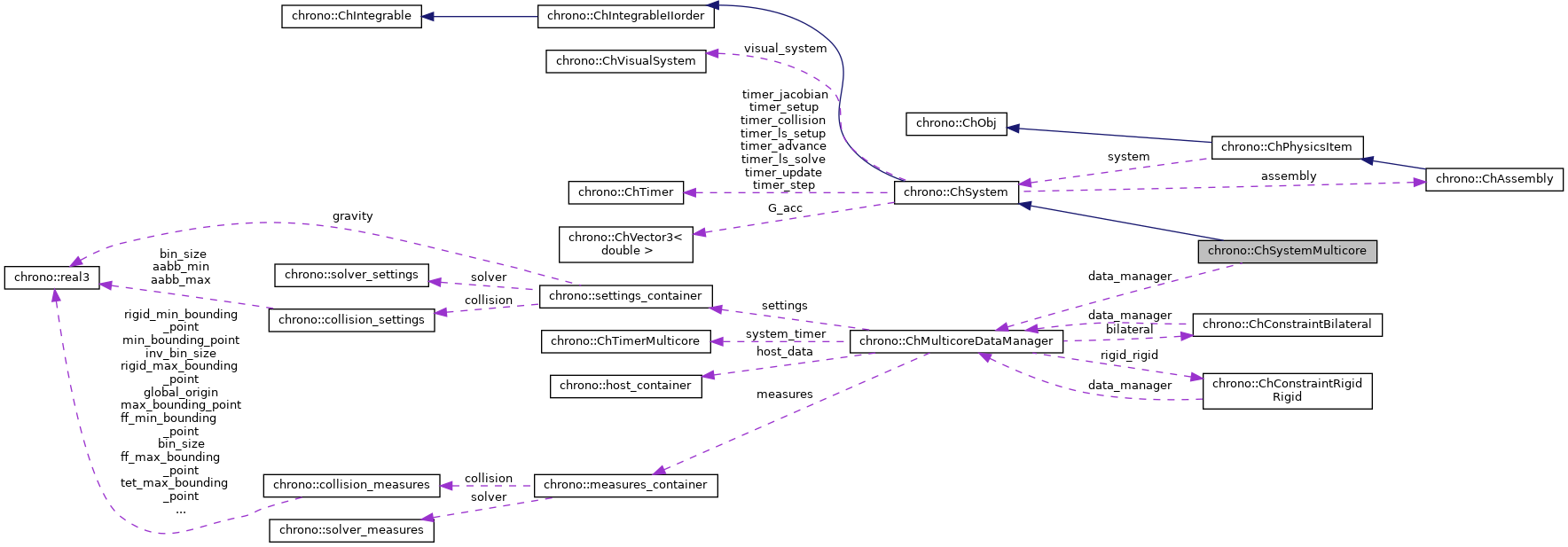
Public Member Functions | |
ChSystemMulticore (const ChSystemMulticore &other) | |
virtual bool | AdvanceDynamics () override |
Performs a single dynamics simulation step, advancing the system state by the current step size. | |
virtual void | AddBody (std::shared_ptr< ChBody > body) override |
Attach a body to the underlying assembly. | |
virtual void | AddShaft (std::shared_ptr< ChShaft > shaft) override |
Attach a shaft to the underlying assembly. | |
virtual void | AddLink (std::shared_ptr< ChLinkBase > link) override |
Attach a link to the underlying assembly. | |
virtual void | AddOtherPhysicsItem (std::shared_ptr< ChPhysicsItem > newitem) override |
Attach a ChPhysicsItem object that is not a body, link, or mesh. | |
void | ClearForceVariables () |
virtual void | Update () |
virtual void | UpdateBilaterals () |
virtual void | UpdateLinks () |
virtual void | UpdateOtherPhysics () |
virtual void | UpdateRigidBodies () |
virtual void | UpdateShafts () |
virtual void | UpdateMotorLinks () |
virtual void | Update3DOFBodies () |
void | RecomputeThreads () |
virtual void | AddMaterialSurfaceData (std::shared_ptr< ChBody > newbody)=0 |
virtual void | UpdateMaterialSurfaceData (int index, ChBody *body)=0 |
virtual void | Setup () override |
Counts the number of bodies and links. More... | |
virtual void | SetCollisionSystemType (ChCollisionSystem::Type type) override |
Set the collision detection system used by this Chrono system to the specified type. | |
virtual void | SetMaterialCompositionStrategy (std::unique_ptr< ChContactMaterialCompositionStrategy > &&strategy) override |
Change the default composition laws for contact surface materials (coefficient of friction, cohesion, compliance, etc.). | |
virtual void | PrintStepStats () |
virtual unsigned int | GetNumBodies () const override |
Get the total number of bodies added to the system, including fixed and sleeping bodies. | |
virtual unsigned int | GetNumShafts () const override |
Get the number of shafts. | |
virtual unsigned int | GetNumContacts () override |
Gets the number of contacts. | |
virtual unsigned int | GetNumConstraints () override |
Get the number of scalar constraints in the system. | |
virtual unsigned int | GetNumConstraintsBilateral () override |
Get the number of bilateral scalar constraints. | |
virtual unsigned int | GetNumConstraintsUnilateral () override |
Get the number of unilateral scalar constraints. | |
virtual double | GetTimerStep () const override |
Return the time (in seconds) spent for computing the time step. | |
virtual double | GetTimerAdvance () const override |
Return the time (in seconds) for time integration, within the time step. | |
virtual double | GetTimerLSsolve () const override |
Return the time (in seconds) for the solver, within the time step. More... | |
virtual double | GetTimerLSsetup () const override |
Return the time (in seconds) for the solver Setup phase, within the time step. | |
virtual double | GetTimerJacobian () const override |
Return the time (in seconds) for calculating/loading Jacobian information, within the time step. | |
virtual double | GetTimerCollision () const override |
Return the time (in seconds) for runnning the collision detection step, within the time step. | |
virtual double | GetTimerSetup () const override |
Return the time (in seconds) for system setup, within the time step. | |
virtual double | GetTimerUpdate () const override |
Return the time (in seconds) for updating auxiliary data, within the time step. | |
virtual void | CalculateContactForces () |
Calculate cummulative contact forces for all bodies in the system. More... | |
virtual ChVector3d | GetBodyAppliedForce (ChBody *body) override |
Return the resultant applied force on the specified body. More... | |
virtual ChVector3d | GetBodyAppliedTorque (ChBody *body) override |
Return the resultant applied torque on the specified body. More... | |
virtual real3 | GetBodyContactForce (std::shared_ptr< ChBody > body) const =0 |
Get the contact force on the specified body. More... | |
virtual real3 | GetBodyContactTorque (std::shared_ptr< ChBody > body) const =0 |
Get the contact torque on the specified body. More... | |
settings_container * | GetSettings () |
virtual void | SetNumThreads (int num_threads_chrono, int num_threads_collision=0, int num_threads_eigen=0) override |
Set the number of OpenMP threads used by Chrono itself, Eigen, and the collision detection system. More... | |
void | EnableThreadTuning (int min_threads, int max_threads) |
Enable dynamic adjustment of number of threads between the specified limits. More... | |
double | CalculateConstraintViolation (std::vector< double > &cvec) |
Calculate the (linearized) bilateral constraint violations. More... | |
![]() | |
ChSystem () | |
Create a physical system. | |
ChSystem (const ChSystem &other) | |
Copy constructor. | |
virtual | ~ChSystem () |
Destructor. | |
virtual ChSystem * | Clone () const =0 |
"Virtual" copy constructor. More... | |
void | SetTimestepperType (ChTimestepper::Type type) |
Set the method for time integration (time stepper type). More... | |
ChTimestepper::Type | GetTimestepperType () const |
Get the current method for time integration (time stepper type). | |
void | SetTimestepper (std::shared_ptr< ChTimestepper > stepper) |
Set the timestepper object to be used for time integration. | |
std::shared_ptr< ChTimestepper > | GetTimestepper () const |
Get the timestepper currently used for time integration. | |
virtual void | SetCollisionSystem (std::shared_ptr< ChCollisionSystem > coll_system) |
Set the collision detection system used by this Chrono system. | |
std::shared_ptr< ChCollisionSystem > | GetCollisionSystem () const |
Access the underlying collision system. More... | |
const ChContactMaterialCompositionStrategy & | GetMaterialCompositionStrategy () const |
Accessor for the current composition laws for contact surface material. | |
void | SetMaxPenetrationRecoverySpeed (double value) |
Set the speed limit of exiting from penetration situations (default: 0.6). More... | |
virtual void | SetSolver (std::shared_ptr< ChSolver > newsolver) |
Attach a solver (derived from ChSolver) for use by this system. More... | |
virtual std::shared_ptr< ChSolver > | GetSolver () |
Access the solver currently associated with this system. | |
void | SetSolverType (ChSolver::Type type) |
Choose the solver type, to be used for the simultaneous solution of the constraints in dynamical simulations (as well as in kinematics, statics, etc.) Notes: More... | |
ChSolver::Type | GetSolverType () const |
Gets the current solver type. | |
void | SetSystemDescriptor (std::shared_ptr< ChSystemDescriptor > newdescriptor) |
Instead of using the default 'system descriptor', you can create your own custom descriptor (inherited from ChSystemDescriptor) and plug it into the system using this function. | |
std::shared_ptr< ChSystemDescriptor > | GetSystemDescriptor () |
Access directly the 'system descriptor'. | |
void | SetGravitationalAcceleration (const ChVector3d &gacc) |
Set the gravitational acceleration vector. | |
const ChVector3d & | GetGravitationalAcceleration () const |
Get the gravitatoinal acceleration vector. | |
double | GetChTime () const |
Get the simulation time of this system. | |
void | SetChTime (double time) |
Set (overwrite) the simulation time of this system. | |
double | GetStep () const |
Gets the current time step used for integration (dynamic and kinematic simulation). More... | |
size_t | GetNumSteps () const |
Return the total number of time steps taken so far. | |
void | ResetNumSteps () |
Reset to 0 the total number of time steps. | |
int | DoStepDynamics (double step_size) |
Advance the dynamics simulation by a single time step of given length. More... | |
bool | DoFrameDynamics (double frame_time, double step_size) |
Advance the dynamics simulation until the specified frame end time is reached. More... | |
AssemblyAnalysis::ExitFlag | DoStepKinematics (double step_size) |
Advance the kinematics simulation for a single step of given length. | |
AssemblyAnalysis::ExitFlag | DoFrameKinematics (double frame_time, double step_size) |
Advance the kinematics simulation to the specified frame end time. More... | |
bool | DoStaticAnalysis (ChStaticAnalysis &analysis) |
Perform a generic static analysis. | |
bool | DoStaticLinear () |
Solve the position of static equilibrium (and the reactions). More... | |
bool | DoStaticNonlinear (int nsteps=10, bool verbose=false) |
Solve the position of static equilibrium (and the reactions). More... | |
bool | DoStaticNonlinearRheonomic (int max_num_iterations=10, bool verbose=false, std::shared_ptr< ChStaticNonLinearRheonomicAnalysis::IterationCallback > callback=nullptr) |
Solve the position of static equilibrium (and the reactions). More... | |
bool | DoStaticRelaxing (double step_size, int num_iterations=10) |
Find the static equilibrium configuration (and the reactions) starting from the current position. More... | |
unsigned int | GetSolverSolveCount () const |
Return the number of calls to the solver's Solve() function. More... | |
unsigned int | GetSolverSetupCount () const |
Return the number of calls to the solver's Setup() function. More... | |
AssemblyAnalysis::ExitFlag | DoAssembly (int action, int max_num_iterationsNR=6, double abstol_residualNR=1e-10, double reltol_updateNR=1e-6, double abstol_updateNR=1e-6) |
Assemble the system. More... | |
unsigned int | RemoveRedundantConstraints (bool remove_links=false, double qr_tol=1e-6, bool verbose=false) |
Remove redundant constraints through QR decomposition of the constraints Jacobian matrix. More... | |
unsigned int | GetNumThreadsChrono () const |
unsigned int | GetNumThreadsCollision () const |
unsigned int | GetNumThreadsEigen () const |
const ChAssembly & | GetAssembly () const |
Get the underlying assembly containing all physics items. | |
virtual void | AddMesh (std::shared_ptr< fea::ChMesh > mesh) |
Attach a mesh to the underlying assembly. | |
void | Add (std::shared_ptr< ChPhysicsItem > item) |
Attach an arbitrary ChPhysicsItem (e.g. More... | |
void | AddBatch (std::shared_ptr< ChPhysicsItem > item) |
Items added in this way are added like in the Add() method, but not instantly, they are simply queued in a batch of 'to add' items, that are added automatically at the first Setup() call. More... | |
void | FlushBatch () |
If some items are queued for addition in the assembly, using AddBatch(), this will effectively add them and clean the batch. More... | |
virtual void | RemoveBody (std::shared_ptr< ChBody > body) |
Remove a body from this assembly. | |
virtual void | RemoveShaft (std::shared_ptr< ChShaft > shaft) |
Remove a shaft from this assembly. | |
virtual void | RemoveLink (std::shared_ptr< ChLinkBase > link) |
Remove a link from this assembly. | |
virtual void | RemoveMesh (std::shared_ptr< fea::ChMesh > mesh) |
Remove a mesh from the assembly. | |
virtual void | RemoveOtherPhysicsItem (std::shared_ptr< ChPhysicsItem > item) |
Remove a ChPhysicsItem object that is not a body or a link. | |
void | Remove (std::shared_ptr< ChPhysicsItem > item) |
Remove arbitrary ChPhysicsItem that was added to the underlying assembly. | |
void | RemoveAllBodies () |
Remove all bodies from the underlying assembly. | |
void | RemoveAllShafts () |
Remove all shafts from the underlying assembly. | |
void | RemoveAllLinks () |
Remove all links from the underlying assembly. | |
void | RemoveAllMeshes () |
Remove all meshes from the underlying assembly. | |
void | RemoveAllOtherPhysicsItems () |
Remove all physics items not in the body, link, or mesh lists. | |
const std::vector< std::shared_ptr< ChBody > > & | GetBodies () const |
Get the list of bodies. | |
const std::vector< std::shared_ptr< ChShaft > > & | GetShafts () const |
Get the list of shafts. | |
const std::vector< std::shared_ptr< ChLinkBase > > & | GetLinks () const |
Get the list of links. | |
const std::vector< std::shared_ptr< fea::ChMesh > > & | GetMeshes () const |
Get the list of meshes. | |
const std::vector< std::shared_ptr< ChPhysicsItem > > & | GetOtherPhysicsItems () const |
Get the list of physics items that are not in the body or link lists. | |
std::shared_ptr< ChBody > | SearchBody (const std::string &name) const |
Search a body by its name. | |
std::shared_ptr< ChBody > | SearchBodyID (int id) const |
Search a body by its ID. | |
std::shared_ptr< ChShaft > | SearchShaft (const std::string &name) const |
Search a shaft by its name. | |
std::shared_ptr< ChLinkBase > | SearchLink (const std::string &name) const |
Search a link by its name. | |
std::shared_ptr< fea::ChMesh > | SearchMesh (const std::string &name) const |
Search a mesh by its name. | |
std::shared_ptr< ChPhysicsItem > | SearchOtherPhysicsItem (const std::string &name) const |
Search from other ChPhysics items (not bodies, links, or meshes) by name. | |
std::shared_ptr< ChMarker > | SearchMarker (const std::string &name) const |
Search a marker by its name. | |
std::shared_ptr< ChMarker > | SearchMarker (int id) const |
Search a marker by its unique ID. | |
std::shared_ptr< ChPhysicsItem > | Search (const std::string &name) const |
Search an item (body, link or other ChPhysics items) by name. | |
virtual unsigned int | GetNumBodiesActive () const |
Get the number of active bodies, excluding sleeping or fixed. | |
virtual unsigned int | GetNumBodiesSleeping () const |
Get the number of sleeping bodies. | |
virtual unsigned int | GetNumBodiesFixed () const |
Get the number of bodies fixed to ground. | |
virtual unsigned int | GetNumShaftsSleeping () const |
Get the number of shafts that are in sleeping mode (excluding fixed shafts). | |
virtual unsigned int | GetNumShaftsFixed () const |
Get the number of shafts that are fixed to ground. | |
virtual unsigned int | GetNumShaftsTotal () const |
Get the total number of shafts added to the assembly, including the grounded and sleeping shafts. | |
virtual unsigned int | GetNumLinks () const |
Get the number of links (including non active). | |
virtual unsigned int | GetNumLinksActive () const |
Get the number of active links. | |
virtual unsigned int | GetNumMeshes () const |
Get the number of meshes. | |
virtual unsigned int | GetNumOtherPhysicsItems () const |
Get the number of other physics items (including non active). | |
virtual unsigned int | GetNumOtherPhysicsItemsActive () const |
Get the number of other active physics items. | |
void | ShowHierarchy (std::ostream &m_file, int level=0) const |
Write the hierarchy of contained bodies, markers, etc. More... | |
void | Clear () |
Removes all bodies/marker/forces/links/contacts, also resets timers and events. | |
virtual ChContactMethod | GetContactMethod () const =0 |
Return the contact method supported by this system. More... | |
virtual void | CustomEndOfStep () |
Executes custom processing at the end of step. More... | |
double | ComputeCollisions () |
Perform the collision detection. More... | |
void | RegisterCustomCollisionCallback (std::shared_ptr< CustomCollisionCallback > callback) |
Specify a callback object to be invoked at each collision detection step. More... | |
void | UnregisterCustomCollisionCallback (std::shared_ptr< CustomCollisionCallback > callback) |
Remove the given collision callback from this system. | |
virtual void | SetContactContainer (std::shared_ptr< ChContactContainer > container) |
Change the underlying contact container. More... | |
std::shared_ptr< ChContactContainer > | GetContactContainer () const |
Access the underlying contact container. More... | |
void | SetSleepingAllowed (bool ms) |
Turn on this feature to let the system put to sleep the bodies whose motion has almost come to a rest. More... | |
bool | IsSleepingAllowed () const |
Tell if the system will put to sleep the bodies whose motion has almost come to a rest. | |
ChVisualSystem * | GetVisualSystem () const |
Get the visual system to which this ChSystem is attached (if any). | |
double | GetTimerCollisionBroad () const |
Return the time (in seconds) for broadphase collision detection, within the time step. | |
double | GetTimerCollisionNarrow () const |
Return the time (in seconds) for narrowphase collision detection, within the time step. | |
double | GetRTF () const |
Get current estimated RTF (real time factor). More... | |
void | SetRTF (double rtf) |
Set (overwrite) the RTF value for this system (if calculated externally). | |
void | ResetTimers () |
Resets the timers. | |
void | EnableSolverMatrixWrite (bool val, const std::string &out_dir=".") |
DEBUGGING. More... | |
bool | IsSolverMatrixWriteEnabled () const |
Return true if solver matrices are being written to disk. | |
void | WriteSystemMatrices (bool save_M, bool save_K, bool save_R, bool save_Cq, const std::string &path, bool one_indexed=true) |
Write the mass (M), damping (R), stiffness (K), and constraint Jacobian (Cq) matrices at current configuration. More... | |
void | GetMassMatrix (ChSparseMatrix &M) |
Compute the system-level mass matrix and load in the provided sparse matrix. | |
void | GetStiffnessMatrix (ChSparseMatrix &K) |
Compute the system-level stiffness matrix and load in the provided sparse matrix. More... | |
void | GetDampingMatrix (ChSparseMatrix &R) |
Compute the system-level damping matrix and load in the provided sparse matrix. More... | |
void | GetConstraintJacobianMatrix (ChSparseMatrix &Cq) |
Compute the system-level constraint Jacobian matrix and load in the provided sparse matrix. More... | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) |
Method to allow deserialization of transient data from archives. | |
void | Update (double mytime, bool update_assets=true) |
Updates all the auxiliary data and children of bodies, forces, links, given their current state. | |
void | Update (bool update_assets=true) |
Updates all the auxiliary data and children of bodies, forces, links, given their current state. | |
void | ForceUpdate () |
In normal usage, no system update is necessary at the beginning of a new dynamics step (since an update is performed at the end of a step). More... | |
void | IntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R, const unsigned int off_L, const ChVectorDynamic<> &L, const ChVectorDynamic<> &Qc) |
void | IntFromDescriptor (const unsigned int off_v, ChStateDelta &v, const unsigned int off_L, ChVectorDynamic<> &L) |
void | InjectVariables (ChSystemDescriptor &sys_descriptor) |
Register with the given system descriptor all ChVariable objects associated with items in the system. | |
void | InjectConstraints (ChSystemDescriptor &sys_descriptor) |
Register with the given system descriptor any ChConstraint objects associated with items in the system. | |
void | LoadConstraintJacobians () |
Compute and load current Jacobians in encapsulated ChConstraint objects. | |
void | InjectKRMMatrices (ChSystemDescriptor &sys_descriptor) |
Register with the given system descriptor any ChKRMBlock objects associated with items in the system. | |
void | LoadKRMMatrices (double Kfactor, double Rfactor, double Mfactor) |
Compute and load current stiffnes (K), damping (R), and mass (M) matrices in encapsulated ChKRMBlock objects. More... | |
void | VariablesFbReset () |
void | VariablesFbLoadForces (double factor=1) |
void | VariablesQbLoadSpeed () |
void | VariablesFbIncrementMq () |
void | VariablesQbSetSpeed (double step=0) |
void | VariablesQbIncrementPosition (double step) |
void | ConstraintsBiReset () |
void | ConstraintsBiLoad_C (double factor=1, double recovery_clamp=0.1, bool do_clamp=false) |
void | ConstraintsBiLoad_Ct (double factor=1) |
void | ConstraintsBiLoad_Qc (double factor=1) |
void | ConstraintsFbLoadForces (double factor=1) |
void | ConstraintsFetch_react (double factor=1) |
virtual unsigned int | GetNumCoordsPosLevel () override |
Get the number of coordinates at the position level. More... | |
virtual unsigned int | GetNumCoordsVelLevel () override |
Get the number of coordinates at the velocity level. More... | |
virtual void | StateGather (ChState &x, ChStateDelta &v, double &T) override |
From system to state y={x,v}. | |
virtual void | StateScatter (const ChState &x, const ChStateDelta &v, const double T, bool full_update) override |
From state Y={x,v} to system. This also triggers an update operation. | |
virtual void | StateGatherAcceleration (ChStateDelta &a) override |
From system to state derivative (acceleration), some timesteppers might need last computed accel. | |
virtual void | StateScatterAcceleration (const ChStateDelta &a) override |
From state derivative (acceleration) to system, sometimes might be needed. | |
virtual void | StateGatherReactions (ChVectorDynamic<> &L) override |
From system to reaction forces (last computed) - some timestepper might need this. | |
virtual void | StateScatterReactions (const ChVectorDynamic<> &L) override |
From reaction forces to system, ex. store last computed reactions in ChLink objects for plotting etc. | |
virtual void | StateIncrementX (ChState &x_new, const ChState &x, const ChStateDelta &Dx) override |
Perform x_new = x + dx, for x in Y = {x, dx/dt}. More... | |
virtual bool | StateSolveCorrection (ChStateDelta &Dv, ChVectorDynamic<> &DL, const ChVectorDynamic<> &R, const ChVectorDynamic<> &Qc, const double c_a, const double c_v, const double c_x, const ChState &x, const ChStateDelta &v, const double T, bool force_state_scatter, bool full_update, bool force_setup) override |
Assuming a DAE of the form. More... | |
virtual void | LoadResidual_F (ChVectorDynamic<> &R, const double c) override |
Increment a vector R with the term c*F: R += c*F. More... | |
virtual void | LoadResidual_Mv (ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) override |
Increment a vector R with a term that has M multiplied a given vector w: R += c*M*w. More... | |
virtual void | LoadLumpedMass_Md (ChVectorDynamic<> &Md, double &err, const double c) override |
Adds the lumped mass to a Md vector, representing a mass diagonal matrix. More... | |
virtual void | LoadResidual_CqL (ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) override |
Increment a vectorR with the term Cq'*L: R += c*Cq'*L. More... | |
virtual void | LoadConstraint_C (ChVectorDynamic<> &Qc, const double c, const bool do_clamp=false, const double clamp=1e30) override |
Increment a vector Qc with the term C: Qc += c*C. More... | |
virtual void | LoadConstraint_Ct (ChVectorDynamic<> &Qc, const double c) override |
Increment a vector Qc with the term Ct = partial derivative dC/dt: Qc += c*Ct. More... | |
![]() | |
virtual void | StateSetup (ChState &x, ChStateDelta &v, ChStateDelta &a) |
Set up the system state with separate II order components x, v, a for y = {x, v} and dy/dt={v, a}. | |
virtual bool | StateSolveA (ChStateDelta &Dvdt, ChVectorDynamic<> &L, const ChState &x, const ChStateDelta &v, const double T, const double dt, bool force_state_scatter, bool full_update, ChLumpingParms *lumping=nullptr) |
Solve for accelerations: a = f(x,v,t) Given current state y={x,v} , computes acceleration a in the state derivative dy/dt={v,a} and lagrangian multipliers L (if any). More... | |
virtual void | StateGather (ChState &y, double &T) override |
Gather system state in specified array. More... | |
virtual void | StateScatter (const ChState &y, const double T, bool full_update) override |
Scatter the states from the provided array to the system. More... | |
virtual void | StateGatherDerivative (ChStateDelta &Dydt) override |
Gather from the system the state derivatives in specified array. More... | |
virtual void | StateScatterDerivative (const ChStateDelta &Dydt) override |
Scatter the state derivatives from the provided array to the system. More... | |
virtual void | StateIncrement (ChState &y_new, const ChState &y, const ChStateDelta &Dy) override |
Increment state array: y_new = y + Dy. More... | |
virtual bool | StateSolve (ChStateDelta &dydt, ChVectorDynamic<> &L, const ChState &y, const double T, const double dt, bool force_state_scatter, bool full_update, ChLumpingParms *lumping=nullptr) override |
Solve for state derivatives: dy/dt = f(y,t). More... | |
virtual bool | StateSolveCorrection (ChStateDelta &Dy, ChVectorDynamic<> &L, const ChVectorDynamic<> &R, const ChVectorDynamic<> &Qc, const double a, const double b, const ChState &y, const double T, const double dt, bool force_state_scatter, bool full_update, bool force_setup) override final |
Override of method for Ist order implicit integrators. More... | |
![]() | |
virtual unsigned int | GetNumCoordsAccLevel () |
Return the number of coordinates at the acceleration level. | |
virtual void | StateSetup (ChState &y, ChStateDelta &dy) |
Set up the system state. | |
virtual void | LoadResidual_Hv (ChVectorDynamic<> &R, const ChVectorDynamic<> &v, const double c) |
Increment a vector R (usually the residual in a Newton Raphson iteration for solving an implicit integration step) with a term that has H multiplied a given vector w: R += c*H*w. More... | |
Public Attributes | |
ChMulticoreDataManager * | data_manager |
int | current_threads |
Protected Attributes | |
double | old_timer |
double | old_timer_cd |
bool | detect_optimal_threads |
int | detect_optimal_bins |
std::vector< double > | timer_accumulator |
std::vector< double > | cd_accumulator |
uint | frame_threads |
uint | frame_bins |
uint | counter |
std::vector< ChLink * >::iterator | it |
![]() | |
ChAssembly | assembly |
underlying mechanical assembly | |
std::shared_ptr< ChContactContainer > | contact_container |
the container of contacts | |
ChVector3d | G_acc |
gravitational acceleration | |
bool | is_initialized |
if false, an initial setup is required (i.e. a call to Initialize) | |
bool | is_updated |
if false, a new update is required (i.e. a call to Update) | |
unsigned int | m_num_coords_pos |
num of scalar coordinates at position level for all active bodies | |
unsigned int | m_num_coords_vel |
num of scalar coordinates at velocity level for all active bodies | |
unsigned int | m_num_constr |
num of scalar constraints (at velocity level) for all active constraints | |
unsigned int | m_num_constr_bil |
num of scalar bilateral active constraints (velocity level) | |
unsigned int | m_num_constr_uni |
num of scalar unilateral active constraints (velocity level) | |
double | ch_time |
simulation time of the system | |
double | step |
time step | |
bool | use_sleeping |
if true, put to sleep objects that come to rest | |
std::shared_ptr< ChSystemDescriptor > | descriptor |
system descriptor | |
std::shared_ptr< ChSolver > | solver |
solver for DVI or DAE problem | |
double | max_penetration_recovery_speed |
limit for speed of penetration recovery (positive) | |
size_t | stepcount |
internal counter for steps | |
unsigned int | setupcount |
number of calls to the solver's Setup() | |
unsigned int | solvecount |
number of StateSolveCorrection (reset to 0 at each timestep of static analysis) | |
bool | write_matrix |
write current system matrix to file(s); for debugging | |
std::string | output_dir |
output directory for writing system matrices | |
unsigned int | ncontacts |
total number of contacts | |
std::shared_ptr< ChCollisionSystem > | collision_system |
collision engine | |
std::vector< std::shared_ptr< CustomCollisionCallback > > | collision_callbacks |
user-defined collision callbacks | |
std::unique_ptr< ChContactMaterialCompositionStrategy > | composition_strategy |
ChVisualSystem * | visual_system |
material composition strategy More... | |
int | nthreads_chrono |
int | nthreads_eigen |
int | nthreads_collision |
ChTimer | timer_step |
timer for integration step | |
ChTimer | timer_advance |
timer for time integration | |
ChTimer | timer_ls_solve |
timer for solver (excluding setup phase) | |
ChTimer | timer_ls_setup |
timer for solver setup | |
ChTimer | timer_jacobian |
timer for computing/loading Jacobian information | |
ChTimer | timer_collision |
timer for collision detection | |
ChTimer | timer_setup |
timer for system setup | |
ChTimer | timer_update |
timer for system update | |
double | m_RTF |
real-time factor (simulation time / simulated time) | |
std::shared_ptr< ChTimestepper > | timestepper |
time-stepper object | |
ChVectorDynamic | applied_forces |
system-wide vector of applied forces (lazy evaluation) | |
bool | applied_forces_current |
indicates if system-wide vector of forces is up-to-date | |
Additional Inherited Members | |
![]() | |
virtual void | DescriptorPrepareInject (ChSystemDescriptor &sys_descriptor) |
Pushes all ChConstraints and ChVariables contained in links, bodies, etc. into the system descriptor. | |
void | Initialize () |
Initial system setup before analysis. More... | |
bool | ManageSleepingBodies () |
Put bodies to sleep if possible. More... | |
Member Function Documentation
◆ CalculateConstraintViolation()
double chrono::ChSystemMulticore::CalculateConstraintViolation | ( | std::vector< double > & | cvec | ) |
Calculate the (linearized) bilateral constraint violations.
Return the maximum constraint violation.
◆ CalculateContactForces()
|
inlinevirtual |
Calculate cummulative contact forces for all bodies in the system.
Note that this function must be explicitly called by the user at each time where calls to GetContactableForce or ContactableTorque are made.
Reimplemented in chrono::ChSystemMulticoreNSC.
◆ EnableThreadTuning()
void chrono::ChSystemMulticore::EnableThreadTuning | ( | int | min_threads, |
int | max_threads | ||
) |
Enable dynamic adjustment of number of threads between the specified limits.
The initial number of threads is set to min_threads.
◆ GetBodyAppliedForce()
|
overridevirtual |
Return the resultant applied force on the specified body.
This resultant force includes all external applied loads acting on the body (from gravity, loads, springs, etc). However, this does not include any constraint forces. In particular, contact forces are not included if using the NSC formulation, but are included when using the SMC formulation.
Reimplemented from chrono::ChSystem.
◆ GetBodyAppliedTorque()
|
overridevirtual |
Return the resultant applied torque on the specified body.
This resultant torque includes all external applied loads acting on the body (from gravity, loads, springs, etc). However, this does not include any constraint forces. In particular, contact torques are not included if using the NSC formulation, but are included when using the SMC formulation.
Reimplemented from chrono::ChSystem.
◆ GetBodyContactForce()
|
pure virtual |
Get the contact force on the specified body.
Note that ComputeContactForces must be called prior to calling this function at any time where reporting of contact forces is desired.
Implemented in chrono::ChSystemMulticoreSMC, and chrono::ChSystemMulticoreNSC.
◆ GetBodyContactTorque()
|
pure virtual |
Get the contact torque on the specified body.
Note that ComputeContactForces must be called prior to calling this function at any time where reporting of contact torques is desired.
Implemented in chrono::ChSystemMulticoreSMC, and chrono::ChSystemMulticoreNSC.
◆ GetTimerLSsolve()
|
overridevirtual |
Return the time (in seconds) for the solver, within the time step.
Note that this time excludes any calls to the solver's Setup function.
Reimplemented from chrono::ChSystem.
◆ SetNumThreads()
|
overridevirtual |
Set the number of OpenMP threads used by Chrono itself, Eigen, and the collision detection system.
num_threads_chrono - used for all OpenMP constructs and thrust algorithms in Chrono::Multicore. num_threads_collision - Ignored. Chrono::Multicore sets num_threads_collision = num_threads_chrono. num_threads_eigen - used in the Eigen sparse direct solvers and a few linear algebra operations. Note that Eigen enables multi-threaded execution only under certain size conditions. See the Eigen documentation. If passing 0, then num_threads_eigen = num_threads_chrono.
By default, num_threads_chrono is set to omp_get_num_procs() and num_threads_eigen is set to 1.
Reimplemented from chrono::ChSystem.
◆ Setup()
|
overridevirtual |
Counts the number of bodies and links.
Computes the offsets of object states in the global state. Assumes that offset_x, offset_w, and offset_L are already set as starting point for offsetting all the contained sub objects.
Reimplemented from chrono::ChSystem.
Reimplemented in chrono::ChSystemMulticoreSMC.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_multicore/physics/ChSystemMulticore.h
- /builds/uwsbel/chrono/src/chrono_multicore/physics/ChSystemMulticore.cpp