Description
Motor to enforce the relative speed w(t) between two shafts, using a rheonomic constraint.
The relative speed represents an anglular velocity for rotational motor and a linear speed for linear motors. Note: no compliance is allowed, so if the actuator hits an undeformable obstacle it hits a pathological situation and the solver result can be unstable/unpredictable. Think at it as a servo drive with "infinitely stiff" control. This type of motor is very easy to use, stable and efficient, and should be used if the 'infinitely stiff' control assumption is a good approximation of what you simulate (e.g., very good and reactive controllers). By default it is initialized with constant angular speed: df/dt = 1. Use SetSpeedFunction() to change to other speed functions.
#include <ChShaftsMotorSpeed.h>
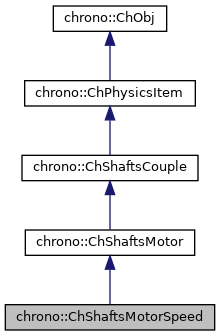
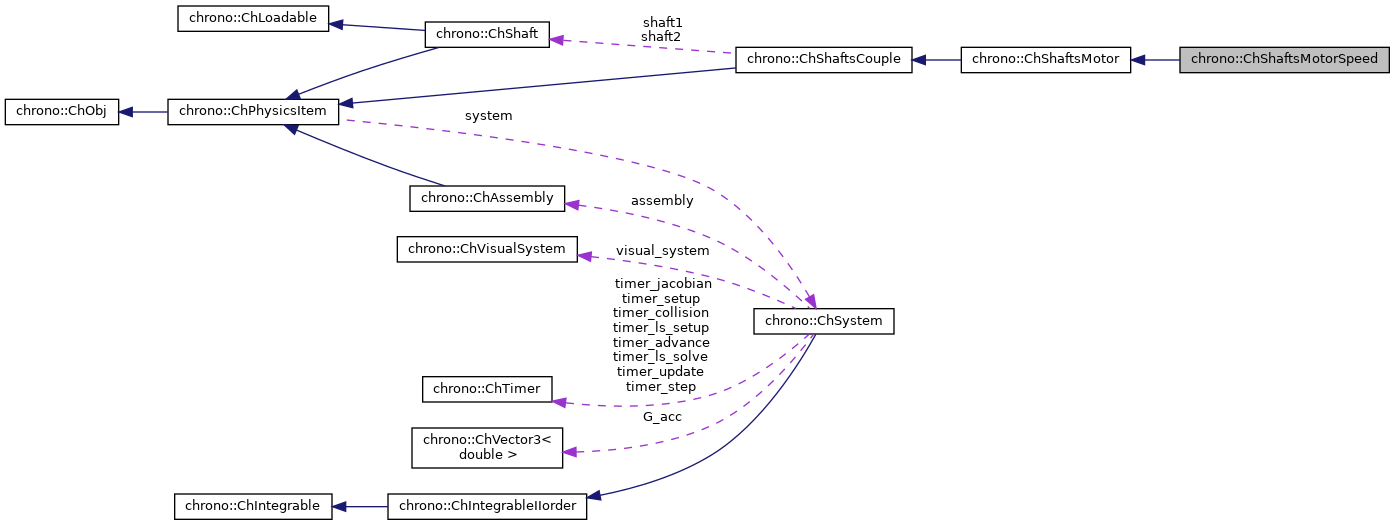
Public Member Functions | |
ChShaftsMotorSpeed (const ChShaftsMotorSpeed &other) | |
virtual ChShaftsMotorSpeed * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
void | SetSpeedFunction (const std::shared_ptr< ChFunction > function) |
Sets the angular speed function s(t). More... | |
std::shared_ptr< ChFunction > | GetSpeedFunction () const |
Gets the speed function s(t). | |
void | SetOffset (double offset) |
Set initial offset (default: 0). | |
double | GetOffset () const |
Get initial offset. | |
void | AvoidDrift (bool avoid) |
Enable relative position drift avoidance (default: true). More... | |
bool | Initialize (std::shared_ptr< ChShaft > shaft_1, std::shared_ptr< ChShaft > shaft_2) override |
Initialize this motor, given two shafts to join. More... | |
virtual double | GetMotorLoad () const override |
Get the current motor torque between shaft2 and shaft1, expressed as applied to shaft1. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow deserialization of transient data from archives. More... | |
![]() | |
ChShaftsMotor (const ChShaftsMotor &other) | |
virtual double | GetMotorPos () const |
Get the position of the motor, as position of shaft 1 with respect to 2. More... | |
virtual double | GetMotorPosDt () const |
Get the motor speed, as speed of shaft 1 with respect to 2. | |
virtual double | GetMotorPosDt2 () const |
Get the motor acceleration, as accelerations of shaft 1 with respect to 2. | |
virtual int | GetMotorNumTurns () const |
Get number of full rotations for this shafts rotational motor. More... | |
virtual double | GetMotorAngleWrapped () const |
Get the motor angle in the range [-pi, +pi]. More... | |
virtual double | GetReaction1 () const override |
Get the reaction torque exchanged between the two shafts, considered as applied to the 1st axis. | |
virtual double | GetReaction2 () const override |
Get the reaction torque exchanged between the two shafts, considered as applied to the 2nd axis. | |
![]() | |
ChShaftsCouple (const ChShaftsCouple &other) | |
virtual unsigned int | GetNumAffectedCoords () |
Get the number of scalar variables affected by constraints in this couple. | |
ChShaft * | GetShaft1 () const |
Get the first (input) shaft. | |
ChShaft * | GetShaft2 () const |
Get the second (output) shaft. | |
double | GetRelativePos () const |
Get the actual relative position (angle or displacement) in terms of phase of shaft 1 with respect to 2. | |
double | GetRelativePosDt () const |
Get the actual relative speed (angle or displacement) of shaft 1 with respect to 2. | |
double | GetRelativePosDt2 () const |
Get the actual relative acceleration (angle or displacement) of shaft 1 with respect to 2. | |
![]() | |
ChPhysicsItem (const ChPhysicsItem &other) | |
ChSystem * | GetSystem () const |
Get the pointer to the parent ChSystem(). | |
virtual void | SetSystem (ChSystem *m_system) |
Set the pointer to the parent ChSystem(). More... | |
virtual bool | IsActive () const |
Return true if the object is active and included in dynamics. | |
virtual bool | IsCollisionEnabled () const |
Tell if the object is subject to collision. More... | |
virtual void | AddCollisionModelsToSystem (ChCollisionSystem *coll_sys) const |
Add to the provided collision system any collision models managed by this physics item. More... | |
virtual void | RemoveCollisionModelsFromSystem (ChCollisionSystem *coll_sys) const |
Remove from the provided collision system any collision models managed by this physics item. More... | |
virtual void | SyncCollisionModels () |
Synchronize the position and bounding box of any collsion models managed by this physics item. | |
virtual ChAABB | GetTotalAABB () const |
Get the axis-aligned bounding box (AABB) of this object. More... | |
virtual ChVector3d | GetCenter () const |
Get a symbolic 'center' of the object. More... | |
virtual void | Setup () |
Perform setup operations. More... | |
virtual void | ForceToRest () |
Set zero speed (and zero accelerations) in state, without changing the position. More... | |
virtual unsigned int | GetNumCoordsVelLevel () |
Get the number of coordinates at the velocity level. More... | |
virtual unsigned int | GetNumConstraints () |
Get the number of scalar constraints. | |
virtual unsigned int | GetNumConstraintsUnilateral () |
Get the number of unilateral scalar constraints. | |
unsigned int | GetOffset_x () |
Get offset in the state vector (position part) | |
unsigned int | GetOffset_w () |
Get offset in the state vector (speed part) | |
unsigned int | GetOffset_L () |
Get offset in the lagrangian multipliers. | |
void | SetOffset_x (const unsigned int moff) |
Set offset in the state vector (position part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_w (const unsigned int moff) |
Set offset in the state vector (speed part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_L (const unsigned int moff) |
Set offset in the lagrangian multipliers Note: only the ChSystem::Setup function should use this. | |
virtual void | IntStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) |
Computes x_new = x + Dt , using vectors at specified offsets. More... | |
virtual void | IntStateGetIncrement (const unsigned int off_x, const ChState &x_new, const ChState &x, const unsigned int off_v, ChStateDelta &Dv) |
Computes Dt = x_new - x, using vectors at specified offsets. More... | |
virtual void | InjectKRMMatrices (ChSystemDescriptor &descriptor) |
Register with the given system descriptor any ChKRMBlock objects associated with this item. | |
virtual void | LoadKRMMatrices (double Kfactor, double Rfactor, double Mfactor) |
Compute and load current stiffnes (K), damping (R), and mass (M) matrices in encapsulated ChKRMBlock objects. More... | |
virtual void | VariablesQbIncrementPosition (double step) |
Increment item positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor. More... | |
virtual void | ConstraintsBiLoad_Qc (double factor=1) |
Adds the current Qc (the vector of C_dtdt=0 -> [Cq]*q_dtdt=Qc ) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsFbLoadForces (double factor=1) |
Adds the current link-forces, if any, (caused by springs, etc.) to the 'fb' vectors of the ChVariables referenced by encapsulated ChConstraints. | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Get the unique integer identifier of this object. More... | |
void | SetTag (int tag) |
Set an object integer tag (default: -1). More... | |
int | GetTag () const |
Get the tag of this object. | |
void | SetName (const std::string &myname) |
Set the name of this object. | |
const std::string & | GetName () const |
Get the name of this object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
void | AddVisualModel (std::shared_ptr< ChVisualModel > model) |
Add an (optional) visualization model. More... | |
std::shared_ptr< ChVisualModel > | GetVisualModel () const |
Access the visualization model (if any). More... | |
void | AddVisualShape (std::shared_ptr< ChVisualShape > shape, const ChFrame<> &frame=ChFrame<>()) |
Add the specified visual shape to the visualization model. More... | |
std::shared_ptr< ChVisualShape > | GetVisualShape (unsigned int i) const |
Access the specified visualization shape in the visualization model (if any). More... | |
void | AddVisualShapeFEA (std::shared_ptr< ChVisualShapeFEA > shapeFEA) |
Add the specified FEA visualization object to the visualization model. More... | |
std::shared_ptr< ChVisualShapeFEA > | GetVisualShapeFEA (unsigned int i) const |
Access the specified FEA visualization object in the visualization model (if any). More... | |
virtual ChFrame | GetVisualModelFrame (unsigned int nclone=0) const |
Get the reference frame (expressed in and relative to the absolute frame) of the visual model. More... | |
virtual unsigned int | GetNumVisualModelClones () const |
Return the number of clones of the visual model associated with this object. More... | |
void | AddCamera (std::shared_ptr< ChCamera > camera) |
Attach a camera to this object. More... | |
std::vector< std::shared_ptr< ChCamera > > | GetCameras () const |
Get the set of cameras attached to this object. | |
void | UpdateVisualModel () |
Utility function to update only the associated visual assets (if any). | |
virtual std::string & | ArchiveContainerName () |
Additional Inherited Members | |
![]() | |
int | GenerateUniqueIdentifier () |
![]() | |
ChShaft * | shaft1 |
first shaft | |
ChShaft * | shaft2 |
second shaft | |
![]() | |
ChSystem * | system |
parent system | |
unsigned int | offset_x |
offset in vector of state (position part) | |
unsigned int | offset_w |
offset in vector of state (speed part) | |
unsigned int | offset_L |
offset in vector of lagrangian multipliers | |
![]() | |
double | ChTime |
object simulation time | |
std::string | m_name |
object name | |
int | m_identifier |
object unique identifier | |
int | m_tag |
user-supplied tag | |
std::shared_ptr< ChVisualModelInstance > | vis_model_instance |
instantiated visualization model | |
std::vector< std::shared_ptr< ChCamera > > | cameras |
set of cameras | |
Member Function Documentation
◆ ArchiveIn()
|
overridevirtual |
Method to allow deserialization of transient data from archives.
Method to allow de serialization of transient data from archives.
Reimplemented from chrono::ChShaftsMotor.
◆ AvoidDrift()
|
inline |
Enable relative position drift avoidance (default: true).
If true, the constraint is satisfied also at the position level, by integrating the velocity in a separate auxiliary state.
◆ Initialize()
|
overridevirtual |
Initialize this motor, given two shafts to join.
The first shaft is the 'output' shaft of the motor, the second is the 'truss', often fixed and not rotating. The torque is applied to the output shaft, while the truss shafts gets the same torque but with opposite sign. Both shafts must belong to the same ChSystem.
- Parameters
-
shaft_1 first shaft to join (motor output shaft) shaft_2 second shaft to join (motor truss)
Reimplemented from chrono::ChShaftsCouple.
◆ SetSpeedFunction()
|
inline |
Sets the angular speed function s(t).
Best if C0 continuous, otherwise it gives spikes in accelerations.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChShaftsMotorSpeed.h
- /builds/uwsbel/chrono/src/chrono/physics/ChShaftsMotorSpeed.cpp