Description
Load representing a XYZ bushing between a ChNodeXYZ and a ChBody application point, with given with spring stiffness as a ChFunction of displacement, for each X,Y,Z direction along the auxiliary frame at the attachment point.
You can set the attachment point via SetApplicationFrameB().
#include <ChLoadsNodeXYZ.h>
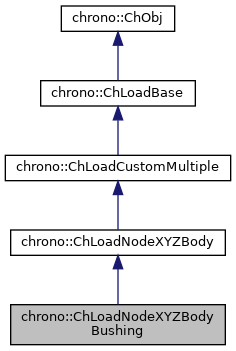
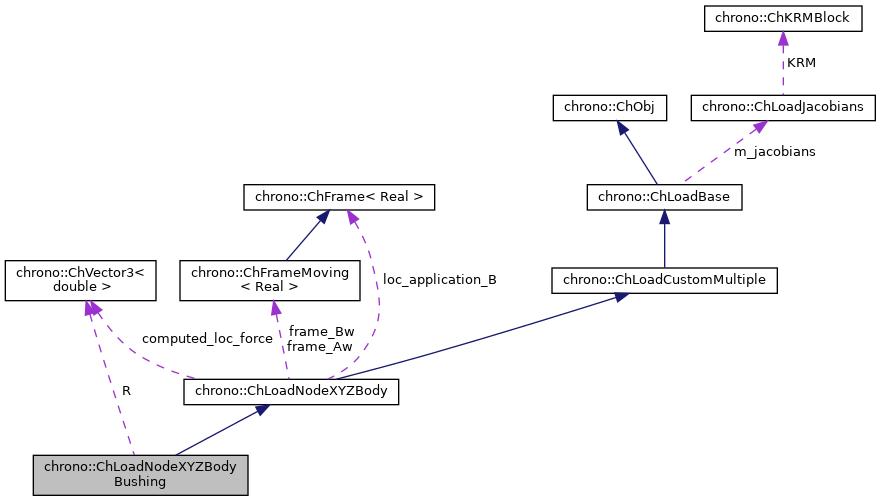
Public Member Functions | |
ChLoadNodeXYZBodyBushing (std::shared_ptr< ChNodeXYZ > nodeA, std::shared_ptr< ChBody > bodyB) | |
virtual ChLoadNodeXYZBodyBushing * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual void | ComputeForce (const ChFrameMoving<> &rel_AB, ChVector3d &loc_force) override |
Compute the force on the nodeA, in local coord system, given relative position of nodeA respect to B. More... | |
void | SetFunctionForceX (std::shared_ptr< ChFunction > fx) |
Set force as a function of displacement on X (default: constant 0 function). | |
void | SetFunctionForceY (std::shared_ptr< ChFunction > fy) |
Set force as a function of displacement on X (default: constant 0 function). | |
void | SetFunctionForceZ (std::shared_ptr< ChFunction > fz) |
Set force as a function of displacement on X (default: constant 0 function). | |
void | SetDamping (const ChVector3d damping) |
Set xyz constant damping coefficients along the three directions xyz. More... | |
ChVector3d | GetDamping () const |
Return the vector of damping coefficients. | |
void | SetStiff (bool ms) |
Declare this load as stiff or non-stiff (default: false). More... | |
![]() | |
ChLoadNodeXYZBody (std::shared_ptr< ChNodeXYZ > node, std::shared_ptr< ChBody > body) | |
ChVector3d | GetForce () const |
Return the last computed value of the applied force. More... | |
void | SetApplicationFrameB (const ChFrame<> &application_frame) |
Set the application frame of load on body. | |
ChFrame | GetApplicationFrameB () const |
Get the application frame of load on body. | |
ChFrameMoving | GetAbsoluteFrameB () const |
Get absolute coordinate of body frame (last computed). | |
std::shared_ptr< ChNodeXYZ > | GetNode () const |
std::shared_ptr< ChBody > | GetBody () const |
![]() | |
ChLoadCustomMultiple (std::vector< std::shared_ptr< ChLoadable >> &loadable_objects) | |
ChLoadCustomMultiple (std::shared_ptr< ChLoadable > loadableA, std::shared_ptr< ChLoadable > loadableB) | |
ChLoadCustomMultiple (std::shared_ptr< ChLoadable > loadableA, std::shared_ptr< ChLoadable > loadableB, std::shared_ptr< ChLoadable > loadableC) | |
virtual int | LoadGetNumCoordsPosLevel () override |
Gets the number of DOFs affected by this load (position part). | |
virtual int | LoadGetNumCoordsVelLevel () override |
Gets the number of DOFs affected by this load (speed part). | |
virtual void | LoadGetStateBlock_x (ChState &mD) override |
Gets all the current DOFs packed in a single vector (position part). | |
virtual void | LoadGetStateBlock_w (ChStateDelta &mD) override |
Gets all the current DOFs packed in a single vector (speed part). | |
virtual void | LoadStateIncrement (const ChState &x, const ChStateDelta &dw, ChState &x_new) override |
Increment a packed state (e.g., as obtained by LoadGetStateBlock_x()) by a given packed state-delta. More... | |
virtual int | LoadGetNumFieldCoords () override |
Number of coordinates in the interpolated field. More... | |
virtual void | ComputeJacobian (ChState *state_x, ChStateDelta *state_w) override |
Compute Jacobian matrices K=-dQ/dx, R=-dQ/dv, and M=-dQ/da. More... | |
virtual void | LoadIntLoadResidual_F (ChVectorDynamic<> &R, double c) override |
Add the internal loads Q (pasted at global offsets) into a global vector R, multiplied by a scaling factor c. More... | |
virtual void | LoadIntLoadResidual_Mv (ChVectorDynamic<> &R, const ChVectorDynamic<> &w, double c) override |
Increment a vector R with the matrix-vector product M*w, scaled by the factor c. More... | |
virtual void | LoadIntLoadLumpedMass_Md (ChVectorDynamic<> &Md, double &err, const double c) override |
Add the lumped mass to an Md vector, representing a mass diagonal matrix. More... | |
virtual void | CreateJacobianMatrices () override |
Create the Jacobian loads if needed and set the ChVariables referenced by the sparse KRM block. | |
virtual ChVectorDynamic & | GetQ () |
Access the generalized load vector Q. | |
![]() | |
ChLoadJacobians * | GetJacobians () |
Access the Jacobians (if any, i.e. if this is a stiff load). | |
virtual void | Update (double time, bool update_assets) override |
Update, called at least at each time step. More... | |
virtual void | InjectKRMMatrices (ChSystemDescriptor &descriptor) |
Register with the given system descriptor any ChKRMBlock objects associated with this item. | |
virtual void | LoadKRMMatrices (double Kfactor, double Rfactor, double Mfactor) |
Compute and load current stiffnes (K), damping (R), and mass (M) matrices in encapsulated ChKRMBlock objects. More... | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Get the unique integer identifier of this object. More... | |
void | SetTag (int tag) |
Set an object integer tag (default: -1). More... | |
int | GetTag () const |
Get the tag of this object. | |
void | SetName (const std::string &myname) |
Set the name of this object. | |
const std::string & | GetName () const |
Get the name of this object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
void | AddVisualModel (std::shared_ptr< ChVisualModel > model) |
Add an (optional) visualization model. More... | |
std::shared_ptr< ChVisualModel > | GetVisualModel () const |
Access the visualization model (if any). More... | |
void | AddVisualShape (std::shared_ptr< ChVisualShape > shape, const ChFrame<> &frame=ChFrame<>()) |
Add the specified visual shape to the visualization model. More... | |
std::shared_ptr< ChVisualShape > | GetVisualShape (unsigned int i) const |
Access the specified visualization shape in the visualization model (if any). More... | |
void | AddVisualShapeFEA (std::shared_ptr< ChVisualShapeFEA > shapeFEA) |
Add the specified FEA visualization object to the visualization model. More... | |
std::shared_ptr< ChVisualShapeFEA > | GetVisualShapeFEA (unsigned int i) const |
Access the specified FEA visualization object in the visualization model (if any). More... | |
virtual ChFrame | GetVisualModelFrame (unsigned int nclone=0) const |
Get the reference frame (expressed in and relative to the absolute frame) of the visual model. More... | |
virtual unsigned int | GetNumVisualModelClones () const |
Return the number of clones of the visual model associated with this object. More... | |
void | AddCamera (std::shared_ptr< ChCamera > camera) |
Attach a camera to this object. More... | |
std::vector< std::shared_ptr< ChCamera > > | GetCameras () const |
Get the set of cameras attached to this object. | |
void | UpdateVisualModel () |
Utility function to update only the associated visual assets (if any). | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) |
Method to allow de-serialization of transient data from archives. | |
virtual std::string & | ArchiveContainerName () |
Protected Member Functions | |
virtual bool | IsStiff () override |
Report if this is load is stiff. More... | |
![]() | |
virtual void | ComputeQ (ChState *state_x, ChStateDelta *state_w) override |
Compute Q, the generalized load. More... | |
![]() | |
int | GenerateUniqueIdentifier () |
Protected Attributes | |
std::shared_ptr< ChFunction > | force_dX |
std::shared_ptr< ChFunction > | force_dY |
std::shared_ptr< ChFunction > | force_dZ |
ChVector3d | R |
damping coefficients along xyz directions | |
bool | is_stiff |
flag indicating a stiff/non-stiff load | |
![]() | |
ChFrame | loc_application_B |
application point on body B (local) | |
ChVector3d | computed_loc_force |
store computed values here | |
ChFrameMoving | frame_Aw |
for results | |
ChFrameMoving | frame_Bw |
for results | |
![]() | |
ChLoadJacobians * | m_jacobians |
![]() | |
double | ChTime |
object simulation time | |
std::string | m_name |
object name | |
int | m_identifier |
object unique identifier | |
int | m_tag |
user-supplied tag | |
std::shared_ptr< ChVisualModelInstance > | vis_model_instance |
instantiated visualization model | |
std::vector< std::shared_ptr< ChCamera > > | cameras |
set of cameras | |
Additional Inherited Members | |
![]() | |
std::vector< std::shared_ptr< ChLoadable > > | loadables |
ChVectorDynamic | load_Q |
Constructor & Destructor Documentation
◆ ChLoadNodeXYZBodyBushing()
chrono::ChLoadNodeXYZBodyBushing::ChLoadNodeXYZBodyBushing | ( | std::shared_ptr< ChNodeXYZ > | nodeA, |
std::shared_ptr< ChBody > | bodyB | ||
) |
- Parameters
-
nodeA node to apply load bodyB body to apply load
Member Function Documentation
◆ ComputeForce()
|
overridevirtual |
Compute the force on the nodeA, in local coord system, given relative position of nodeA respect to B.
The local coordinate system is that specified with SetApplicationFrameB (the auxiliary frame attached to body).
Implements chrono::ChLoadNodeXYZBody.
◆ IsStiff()
|
inlineoverrideprotectedvirtual |
Report if this is load is stiff.
If so, InjectKRMMatrices will provide the Jacobians of the load.
Implements chrono::ChLoadBase.
◆ SetDamping()
|
inline |
Set xyz constant damping coefficients along the three directions xyz.
This assumes local xyz directions of the frame attached to body via SetApplicationFrameB.
◆ SetStiff()
|
inline |
Declare this load as stiff or non-stiff (default: false).
If set as a stiff load, this enables the automatic computation of the Jacobian through finite differences.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChLoadsNodeXYZ.h
- /builds/uwsbel/chrono/src/chrono/physics/ChLoadsNodeXYZ.cpp