Description
Class for feeders like vibrating bowls, conveyor belts.
Compared to ChConveyor, this class provides more functionality, where the vibrating feeder can have any shape. The trick is that the feeder part needs not to vibrate: this ChFeeder simply modifies the contact data between colliding parts and the part marked as feeder (which can be simply static) so that a tangential velocity is imposed: the overall effect is like what happens for very high frequency of vibration.
#include <ChFeeder.h>
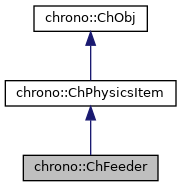
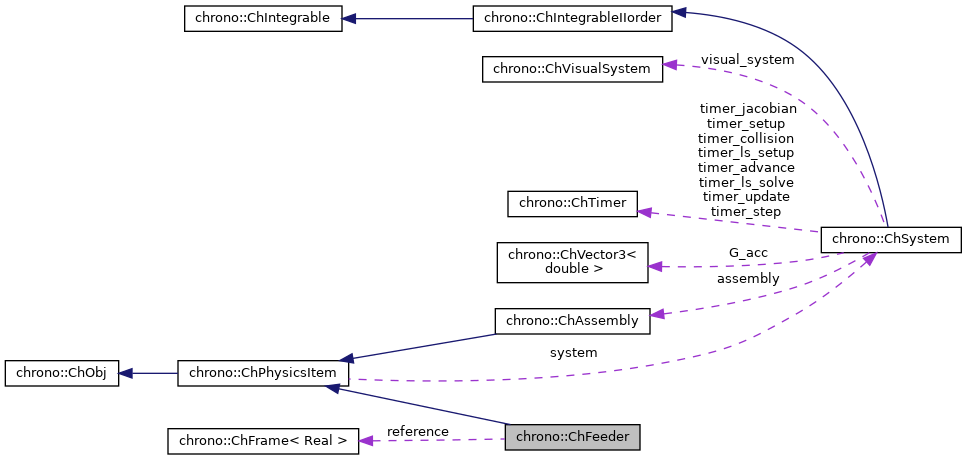
Public Member Functions | |
ChFeeder (const ChFeeder &other) | |
virtual ChFeeder * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
void | SetFeederObject (std::shared_ptr< ChContactable > mfeeder) |
Set the feeder object, defining the surface of the vibrating feeder. | |
std::shared_ptr< ChContactable > | GetFeederObject () |
Get the feeder object, defining the surface of the vibrating feeder. | |
void | SetFeederVibration (ChFrame<> ref, double vx, double vy, double vz, double wx, double wy, double wz) |
Set the reference for the (virtual, not simulated) vibration of the feeder, in abs space. More... | |
void | GetFeederVibration (ChFrame<> &ref, double &vx, double &vy, double &vz, double &wx, double &wy, double &wz) |
Get the reference for the (virtual, not simulated) vibration of the feeder, in abs space. | |
virtual unsigned int | GetNumCoordsPosLevel () override |
Number of coordinates. | |
virtual unsigned int | GetNumCoordsVelLevel () override |
Number of speed coordinates. | |
virtual unsigned int | GetNumConstraintsBilateral () override |
Get the number of scalar constraints. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
Method to allow deserialization of transient data from archives. | |
![]() | |
ChPhysicsItem (const ChPhysicsItem &other) | |
ChSystem * | GetSystem () const |
Get the pointer to the parent ChSystem(). | |
virtual void | SetSystem (ChSystem *m_system) |
Set the pointer to the parent ChSystem(). More... | |
virtual bool | IsActive () const |
Return true if the object is active and included in dynamics. | |
virtual bool | IsCollisionEnabled () const |
Tell if the object is subject to collision. More... | |
virtual void | AddCollisionModelsToSystem (ChCollisionSystem *coll_sys) const |
Add to the provided collision system any collision models managed by this physics item. More... | |
virtual void | RemoveCollisionModelsFromSystem (ChCollisionSystem *coll_sys) const |
Remove from the provided collision system any collision models managed by this physics item. More... | |
virtual void | SyncCollisionModels () |
Synchronize the position and bounding box of any collsion models managed by this physics item. | |
virtual ChAABB | GetTotalAABB () const |
Get the axis-aligned bounding box (AABB) of this object. More... | |
virtual ChVector3d | GetCenter () const |
Get a symbolic 'center' of the object. More... | |
virtual void | Setup () |
Perform setup operations. More... | |
virtual void | ForceToRest () |
Set zero speed (and zero accelerations) in state, without changing the position. More... | |
virtual unsigned int | GetNumConstraints () |
Get the number of scalar constraints. | |
virtual unsigned int | GetNumConstraintsUnilateral () |
Get the number of unilateral scalar constraints. | |
unsigned int | GetOffset_x () |
Get offset in the state vector (position part) | |
unsigned int | GetOffset_w () |
Get offset in the state vector (speed part) | |
unsigned int | GetOffset_L () |
Get offset in the lagrangian multipliers. | |
void | SetOffset_x (const unsigned int moff) |
Set offset in the state vector (position part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_w (const unsigned int moff) |
Set offset in the state vector (speed part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_L (const unsigned int moff) |
Set offset in the lagrangian multipliers Note: only the ChSystem::Setup function should use this. | |
virtual void | IntStateGather (const unsigned int off_x, ChState &x, const unsigned int off_v, ChStateDelta &v, double &T) |
From item's state to global state vectors y={x,v} pasting the states at the specified offsets. More... | |
virtual void | IntStateScatter (const unsigned int off_x, const ChState &x, const unsigned int off_v, const ChStateDelta &v, const double T, bool full_update) |
From global state vectors y={x,v} to item's state (and update) fetching the states at the specified offsets. More... | |
virtual void | IntStateGatherAcceleration (const unsigned int off_a, ChStateDelta &a) |
From item's state acceleration to global acceleration vector. More... | |
virtual void | IntStateScatterAcceleration (const unsigned int off_a, const ChStateDelta &a) |
From global acceleration vector to item's state acceleration. More... | |
virtual void | IntStateGatherReactions (const unsigned int off_L, ChVectorDynamic<> &L) |
From item's reaction forces to global reaction vector. More... | |
virtual void | IntStateScatterReactions (const unsigned int off_L, const ChVectorDynamic<> &L) |
From global reaction vector to item's reaction forces. More... | |
virtual void | IntStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) |
Computes x_new = x + Dt , using vectors at specified offsets. More... | |
virtual void | IntStateGetIncrement (const unsigned int off_x, const ChState &x_new, const ChState &x, const unsigned int off_v, ChStateDelta &Dv) |
Computes Dt = x_new - x, using vectors at specified offsets. More... | |
virtual void | IntLoadResidual_F (const unsigned int off, ChVectorDynamic<> &R, const double c) |
Takes the F force term, scale and adds to R at given offset: R += c*F. More... | |
virtual void | IntLoadResidual_Mv (const unsigned int off, ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) |
Takes the M*v term, multiplying mass by a vector, scale and adds to R at given offset: R += c*M*w. More... | |
virtual void | IntLoadLumpedMass_Md (const unsigned int off, ChVectorDynamic<> &Md, double &err, const double c) |
Adds the lumped mass to a Md vector, representing a mass diagonal matrix. More... | |
virtual void | IntLoadResidual_CqL (const unsigned int off_L, ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) |
Takes the term Cq'*L, scale and adds to R at given offset: R += c*Cq'*L. More... | |
virtual void | IntLoadConstraint_C (const unsigned int off, ChVectorDynamic<> &Qc, const double c, bool do_clamp, double recovery_clamp) |
Takes the term C, scale and adds to Qc at given offset: Qc += c*C. More... | |
virtual void | IntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R, const unsigned int off_L, const ChVectorDynamic<> &L, const ChVectorDynamic<> &Qc) |
Prepare variables and constraints to accommodate a solution: More... | |
virtual void | IntFromDescriptor (const unsigned int off_v, ChStateDelta &v, const unsigned int off_L, ChVectorDynamic<> &L) |
After a solver solution, fetch values from variables and constraints into vectors: More... | |
virtual void | InjectVariables (ChSystemDescriptor &descriptor) |
Register with the given system descriptor any ChVariable objects associated with this item. | |
virtual void | InjectConstraints (ChSystemDescriptor &descriptor) |
Register with the given system descriptor any ChConstraint objects associated with this item. | |
virtual void | LoadConstraintJacobians () |
Compute and load current Jacobians in encapsulated ChConstraint objects. | |
virtual void | InjectKRMMatrices (ChSystemDescriptor &descriptor) |
Register with the given system descriptor any ChKRMBlock objects associated with this item. | |
virtual void | LoadKRMMatrices (double Kfactor, double Rfactor, double Mfactor) |
Compute and load current stiffnes (K), damping (R), and mass (M) matrices in encapsulated ChKRMBlock objects. More... | |
virtual void | VariablesFbReset () |
Sets the 'fb' part (the known term) of the encapsulated ChVariables to zero. | |
virtual void | VariablesFbLoadForces (double factor=1) |
Adds the current forces (applied to item) into the encapsulated ChVariables, in the 'fb' part: qf+=forces*factor. | |
virtual void | VariablesQbLoadSpeed () |
Initialize the 'qb' part of the ChVariables with the current value of speeds. More... | |
virtual void | VariablesFbIncrementMq () |
Adds M*q (masses multiplied current 'qb') to Fb, ex. More... | |
virtual void | VariablesQbSetSpeed (double step=0) |
Fetches the item speed (ex. More... | |
virtual void | VariablesQbIncrementPosition (double step) |
Increment item positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor. More... | |
virtual void | ConstraintsBiReset () |
Sets to zero the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_C (double factor=1, double recovery_clamp=0.1, bool do_clamp=false) |
Adds the current C (constraint violation) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Ct (double factor=1) |
Adds the current Ct (partial t-derivative, as in C_dt=0-> [Cq]*q_dt=-Ct) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Qc (double factor=1) |
Adds the current Qc (the vector of C_dtdt=0 -> [Cq]*q_dtdt=Qc ) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsFbLoadForces (double factor=1) |
Adds the current link-forces, if any, (caused by springs, etc.) to the 'fb' vectors of the ChVariables referenced by encapsulated ChConstraints. | |
virtual void | ConstraintsFetch_react (double factor=1) |
Fetches the reactions from the lagrangian multiplier (l_i) of encapsulated ChConstraints. More... | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Get the unique integer identifier of this object. More... | |
void | SetTag (int tag) |
Set an object integer tag (default: -1). More... | |
int | GetTag () const |
Get the tag of this object. | |
void | SetName (const std::string &myname) |
Set the name of this object. | |
const std::string & | GetName () const |
Get the name of this object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
void | AddVisualModel (std::shared_ptr< ChVisualModel > model) |
Add an (optional) visualization model. More... | |
std::shared_ptr< ChVisualModel > | GetVisualModel () const |
Access the visualization model (if any). More... | |
void | AddVisualShape (std::shared_ptr< ChVisualShape > shape, const ChFrame<> &frame=ChFrame<>()) |
Add the specified visual shape to the visualization model. More... | |
std::shared_ptr< ChVisualShape > | GetVisualShape (unsigned int i) const |
Access the specified visualization shape in the visualization model (if any). More... | |
void | AddVisualShapeFEA (std::shared_ptr< ChVisualShapeFEA > shapeFEA) |
Add the specified FEA visualization object to the visualization model. More... | |
std::shared_ptr< ChVisualShapeFEA > | GetVisualShapeFEA (unsigned int i) const |
Access the specified FEA visualization object in the visualization model (if any). More... | |
virtual ChFrame | GetVisualModelFrame (unsigned int nclone=0) const |
Get the reference frame (expressed in and relative to the absolute frame) of the visual model. More... | |
virtual unsigned int | GetNumVisualModelClones () const |
Return the number of clones of the visual model associated with this object. More... | |
void | AddCamera (std::shared_ptr< ChCamera > camera) |
Attach a camera to this object. More... | |
std::vector< std::shared_ptr< ChCamera > > | GetCameras () const |
Get the set of cameras attached to this object. | |
void | UpdateVisualModel () |
Utility function to update only the associated visual assets (if any). | |
virtual std::string & | ArchiveContainerName () |
Public Attributes | |
ChFrame | reference |
double | v_x |
double | v_y |
double | v_z |
double | w_x |
double | w_y |
double | w_z |
Additional Inherited Members | |
![]() | |
int | GenerateUniqueIdentifier () |
![]() | |
ChSystem * | system |
parent system | |
unsigned int | offset_x |
offset in vector of state (position part) | |
unsigned int | offset_w |
offset in vector of state (speed part) | |
unsigned int | offset_L |
offset in vector of lagrangian multipliers | |
![]() | |
double | ChTime |
object simulation time | |
std::string | m_name |
object name | |
int | m_identifier |
object unique identifier | |
int | m_tag |
user-supplied tag | |
std::shared_ptr< ChVisualModelInstance > | vis_model_instance |
instantiated visualization model | |
std::vector< std::shared_ptr< ChCamera > > | cameras |
set of cameras | |
Member Function Documentation
◆ SetFeederVibration()
void chrono::ChFeeder::SetFeederVibration | ( | ChFrame<> | ref, |
double | vx, | ||
double | vy, | ||
double | vz, | ||
double | wx, | ||
double | wy, | ||
double | wz | ||
) |
Set the reference for the (virtual, not simulated) vibration of the feeder, in abs space.
Also set the eigenvector of the vibration mode as x,y,z,rx,ry,rz about that frame. The six values also define the max speed on that axis.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChFeeder.h
- /builds/uwsbel/chrono/src/chrono/physics/ChFeeder.cpp