chrono::ChCollisionModelDistributed Class Reference
Description
Specialization of ChCollisionModelChrono to track the model AABB.
The model AABB is used in testing intersections with sub-domains.
#include <ChCollisionModelDistributed.h>
Inheritance diagram for chrono::ChCollisionModelDistributed:
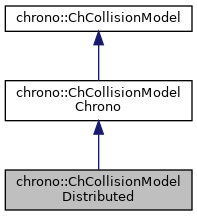
Collaboration diagram for chrono::ChCollisionModelDistributed:
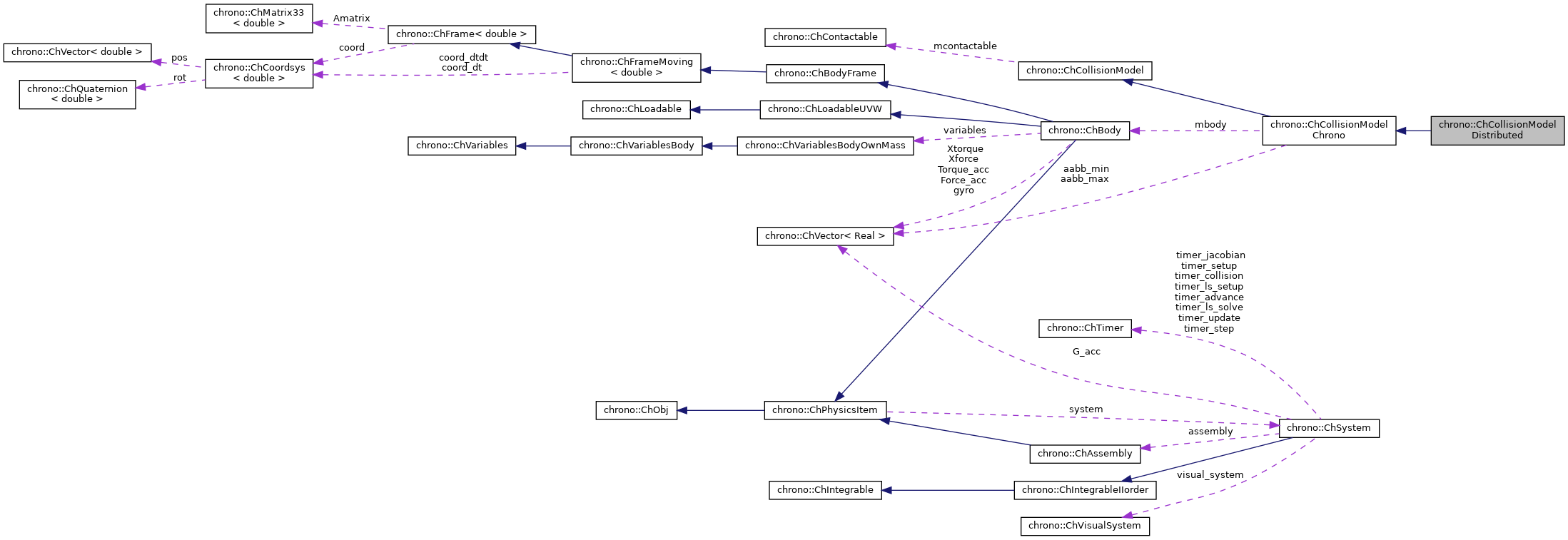
Public Member Functions | |
virtual geometry::ChAABB | GetBoundingBox () const override |
Return the current axis aligned bounding box (AABB) of the collision model. More... | |
![]() | |
virtual ChCollisionSystemType | GetType () const override |
Return the type of this collision model. | |
virtual bool | AddCopyOfAnotherModel (ChCollisionModel *another) override |
Add all shapes already contained in another model. | |
virtual void | SyncPosition () override |
Sets the position and orientation of the collision model as the rigid body current position. | |
ChBody * | GetBody () const |
Return a pointer to the associated body. | |
void | SetBody (ChBody *body) |
Set the pointer to the owner rigid body. | |
virtual void | SetContactable (ChContactable *mc) override |
Sets the pointer to the contactable object. | |
virtual ChCoordsys | GetShapePos (int index) const override |
Return the position and orientation of the collision shape with specified index, relative to the model frame. | |
virtual void | ArchiveOut (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) override |
Method to allow deserialization of transient data from archives. | |
![]() | |
void | Clear () |
Delete all inserted collision shapes. More... | |
void | Build () |
Complete the construction of the collision model. More... | |
void | AddShape (std::shared_ptr< ChCollisionShape > shape, const ChFrame<> &frame=ChFrame<>()) |
Add a collision shape with specified position within the model. More... | |
void | AddCylinder (std::shared_ptr< ChMaterialSurface > material, double radius, const ChVector<> &p1, const ChVector<> &p2) |
Convenience function to add a cylinder specified through a radius and end points. More... | |
ChContactable * | GetContactable () |
Get the pointer to the contactable object. | |
virtual ChPhysicsItem * | GetPhysicsItem () |
Get the pointer to the client owner ChPhysicsItem. More... | |
virtual void | SetFamily (int mfamily) |
By default, all collision objects belong to family n.0, but you can set family in range 0..15. More... | |
virtual int | GetFamily () |
virtual void | SetFamilyMaskNoCollisionWithFamily (int mfamily) |
By default, family mask is all turned on, so all families can collide with this object, but you can turn on-off some bytes of this mask so that some families do not collide. More... | |
virtual void | SetFamilyMaskDoCollisionWithFamily (int mfamily) |
virtual bool | GetFamilyMaskDoesCollisionWithFamily (int mfamily) |
Tells if the family mask of this collision object allows for the collision with another collision object belonging to a given family. More... | |
virtual short int | GetFamilyGroup () const |
Return the collision family group of this model. More... | |
virtual void | SetFamilyGroup (short int group) |
Set the collision family group of this model. More... | |
virtual short int | GetFamilyMask () const |
Return the collision mask for this model. More... | |
virtual void | SetFamilyMask (short int mask) |
Set the collision mask for this model. More... | |
virtual void | SetSafeMargin (double amargin) |
Set the suggested collision 'inward safe margin' for the shapes to be added from now on. More... | |
virtual float | GetSafeMargin () |
Returns the inward safe margin (see SetSafeMargin() ) | |
virtual void | SetEnvelope (double amargin) |
Set the suggested collision outward 'envelope' used from shapes added from now on. More... | |
virtual float | GetEnvelope () |
Return the outward safe margin (see SetEnvelope() ) | |
int | GetNumShapes () const |
Return the number of collision shapes in this model. | |
const std::vector< std::shared_ptr< ChCollisionShape > > & | GetShapes () const |
Get the list of collision shapes in this model. | |
std::shared_ptr< ChCollisionShape > | GetShape (int index) |
Get the collision shape with specified index. | |
std::vector< double > | GetShapeDimensions (int index) const |
Return shape characteristic dimensions. More... | |
void | SetShapeMaterial (int index, std::shared_ptr< ChMaterialSurface > mat) |
Set the contact material for the collision shape with specified index. | |
void | SetAllShapesMaterial (std::shared_ptr< ChMaterialSurface > mat) |
Set the contact material for all collision shapes in the model (all shapes will share the material). More... | |
Friends | |
class | ChCollisionBoundaryDistributed |
class | ChCollisionSystemDistributed |
Additional Inherited Members | |
![]() | |
typedef std::pair< std::shared_ptr< ChCollisionShape >, ChFrame<> > | ShapeInstance |
A ShapeInstance is a pair of a collision shape and its position in the model. | |
![]() | |
static void | SetDefaultSuggestedEnvelope (double menv) |
Using this function BEFORE you start creating collision shapes, it will make all following collision shapes to take this collision envelope (safe outward layer) as default. More... | |
static void | SetDefaultSuggestedMargin (double mmargin) |
Using this function BEFORE you start creating collision shapes, it will make all following collision shapes to take this collision margin (inward penetration layer) as default. More... | |
static double | GetDefaultSuggestedEnvelope () |
static double | GetDefaultSuggestedMargin () |
![]() | |
std::vector< real3 > | local_convex_data |
ChVector | aabb_min |
ChVector | aabb_max |
![]() | |
void | CopyShapes (ChCollisionModel *other) |
Copy the collision shapes from another model. | |
virtual float | GetSuggestedFullMargin () |
![]() | |
ChBody * | mbody |
associated contactable (rigid body only) | |
std::vector< std::shared_ptr< ctCollisionShape > > | m_ct_shapes |
list of Chrono collision shapes in model | |
![]() | |
float | model_envelope |
Maximum envelope: surrounding volume from surface to the exterior. | |
float | model_safe_margin |
Maximum margin value to be used for fast penetration contact detection. | |
ChContactable * | mcontactable |
Pointer to the contactable object. | |
short int | family_group |
Collision family group. | |
short int | family_mask |
Collision family mask. | |
std::vector< ShapeInstance > | m_shape_instances |
list of collision shapes and positions in model | |
std::vector< std::shared_ptr< ChCollisionShape > > | m_shapes |
extended list of collision shapes | |
Member Function Documentation
◆ GetBoundingBox()
|
overridevirtual |
Return the current axis aligned bounding box (AABB) of the collision model.
Only valid at beginning of simulation.
Reimplemented from chrono::ChCollisionModelChrono.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_distributed/collision/ChCollisionModelDistributed.h
- /builds/uwsbel/chrono/src/chrono_distributed/collision/ChCollisionModelDistributed.cpp