Public Member Functions |
Protected Member Functions |
Protected Attributes |
Friends |
List of all members
chrono::vehicle::ChVehicleVisualSystemIrrlicht Class Reference
Description
Customized Chrono Irrlicht visualization for vehicle simulations.
This class implements an Irrlicht-based visualization wrapper for vehicles. This class extends ChVisualSystemIrrlicht and provides the following functionality:
- rendering of the entire Irrlicht scene
- custom chase-camera (which can be controlled with keyboard)
- optional rendering of links, springs, stats, etc.
#include <ChVehicleVisualSystemIrrlicht.h>
Inheritance diagram for chrono::vehicle::ChVehicleVisualSystemIrrlicht:

Collaboration diagram for chrono::vehicle::ChVehicleVisualSystemIrrlicht:
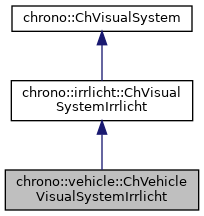
Public Member Functions | |
ChVehicleVisualSystemIrrlicht () | |
Construct a vehicle Irrlicht visualization system. | |
void | SetJoystickConfigFile (const std::string &filename) |
Set joystick JSON configuration file name. | |
void | SetJoystickDebug (bool val) |
Enable/disable joystick debugging output (default: false). | |
void | SetButtonCallback (int button, void(*cbfun)()) |
Feed button number and callback function to implement a custom callback. | |
virtual void | AttachVehicle (vehicle::ChVehicle *vehicle) override |
Attach a vehicle to this Irrlicht vehicle visualization system. | |
void | SetHUDLocation (int HUD_x, int HUD_y) |
Set the upper-left point of HUD elements. | |
void | EnableStats (bool val) |
Turn on/off rendering of stats (HUD). | |
void | EnableSound (bool sound) |
Turn on/off Irrklang sound generation. More... | |
virtual void | Initialize () override |
Initialize the visualization system. | |
virtual void | Render () override |
Render the Irrlicht scene and additional visual elements. | |
virtual void | Advance (double step) override |
Advance the dynamics of the chase camera. More... | |
![]() | |
ChVisualSystemIrrlicht (ChSystem *sys, const ChVector3d &camera_pos=ChVector3d(2, 2, 2), const ChVector3d &camera_targ=ChVector3d(0, 0, 0)) | |
Auto-initialized run-time visualization system, with default settings. | |
virtual void | AttachSystem (ChSystem *sys) override |
Attach another Chrono system to the run-time visualization system. More... | |
void | SetAntialias (bool val) |
Enable/disable antialias (default true). More... | |
void | EnableFullscreen (bool val) |
Enable/disable full-screen mode (default false). More... | |
void | EnableShadows (bool val) |
Enable/disable shadows (default false). More... | |
void | SetDriverType (irr::video::E_DRIVER_TYPE driver_type) |
Set the device driver type (default irr::video::EDT_DIRECT3D9). More... | |
void | SetWindowSize (unsigned int width, unsigned int height) |
Set the window size (default 640x480). More... | |
void | SetWindowTitle (const std::string &win_title) |
Set the windoiw title (default ""). More... | |
void | SetWindowId (void *window_id) |
Set the window ID. More... | |
void | SetCameraVertical (CameraVerticalDir vert) |
Use Y-up camera rendering (default CameraVerticalDir::Y). More... | |
CameraVerticalDir | GetCameraVertical () |
Tells if the current camera vertical mode is Y or Z. | |
void | SetLogLevel (irr::ELOG_LEVEL log_level) |
Set the Irrlicht logging level (default irr::ELL_INFORMATION). More... | |
void | SetSymbolScale (double scale) |
Set the scale for symbol drawing (default: 1). | |
void | AddLogo (const std::string &logo_filename=GetChronoDataFile("logo_chrono_alpha.png")) |
Add a logo in a 3D scene. More... | |
virtual int | AddCamera (const ChVector3d &pos, ChVector3d targ=VNULL) override |
Add a camera in an Irrlicht 3D scene. More... | |
virtual void | AddGrid (double x_step, double y_step, int nx, int ny, ChCoordsys<> pos=CSYSNORM, ChColor col=ChColor(0.1f, 0.1f, 0.1f)) override |
Add a grid with specified parameters in the x-y plane of the given frame. More... | |
void | UpdateGrid (int id, const ChCoordsys<> &csys) |
void | AddGuiColorbar (const std::string &title, const ChVector2d &range, ChColormap::Type type, bool bimodal=false, const ChVector2i &pos=ChVector2i(740, 20), const ChVector2i &size=ChVector2i(30, 300)) |
Add a colorbar widget. More... | |
virtual void | SetCameraPosition (int id, const ChVector3d &pos) override |
Set the location of the specified camera. | |
virtual void | SetCameraTarget (int id, const ChVector3d &target) override |
Set the target (look-at) point of the specified camera. | |
virtual void | SetCameraPosition (const ChVector3d &pos) override |
Set the location of the current (active) camera. | |
virtual void | SetCameraTarget (const ChVector3d &target) override |
Set the target (look-at) point of the current (active) camera. | |
void | AddSkyBox (const std::string &texture_dir=GetChronoDataFile("skybox/")) |
Add a sky box in a 3D scene. More... | |
irr::scene::ILightSceneNode * | AddLightDirectional (double elevation=60, double azimuth=60, ChColor ambient=ChColor(0.5f, 0.5f, 0.5f), ChColor specular=ChColor(0.2f, 0.2f, 0.2f), ChColor diffuse=ChColor(1.0f, 1.0f, 1.0f)) |
Add a directional light to the scene. More... | |
irr::scene::ILightSceneNode * | AddLight (const ChVector3d &pos, double radius, ChColor color=ChColor(0.7f, 0.7f, 0.7f)) |
Add a point light to the scene. More... | |
irr::scene::ILightSceneNode * | AddLightWithShadow (const ChVector3d &pos, const ChVector3d &aim, double radius, double near_value, double far_value, double angle, unsigned int resolution=512, ChColor color=ChColor(1, 1, 1), bool directional=false, bool clipborder=true) |
Add a point light that cast shadow (using soft shadows/shadow maps) Note that the quality of the shadow strictly depends on how close 'near_value' and 'far_value' are to the bounding box of the scene. More... | |
void | AddTypicalLights () |
Simple shortcut to set two point lights in the scene. More... | |
void | AddUserEventReceiver (irr::IEventReceiver *receiver) |
Attach a custom event receiver to the application. | |
void | EnableShadows (std::shared_ptr< ChPhysicsItem > item=nullptr) |
Enable shadow maps for all visual models in a scene or only for a single physics item. More... | |
void | EnableContactDrawing (ContactsDrawMode mode) |
Enable contact rendering (default: none). More... | |
void | EnableLinkDrawing (LinkDrawMode mode) |
Enable rendering of link (joint) frames (default: none). More... | |
void | EnableBodyFrameDrawing (bool val) |
Enable rendering of body frames (default: false). More... | |
void | EnableLinkFrameDrawing (bool val) |
Enable rendering of link frames (default: false). More... | |
void | EnableCollisionShapeDrawing (bool val) |
Enable rendering of collision shapes (default: false). More... | |
void | EnableAbsCoordsysDrawing (bool val) |
Enable rendering of the absolute coordinate system (default: none). More... | |
void | ShowProfiler (bool val) |
Show the realtime profiler in the 3D view. | |
void | ShowExplorer (bool val) |
Show the object explorer. | |
void | ShowInfoPanel (bool val) |
Show the info panel in the 3D view. | |
void | ShowConvergencePlot (bool val) |
Show the convergence plot (available only for iterative solvers). | |
void | SetInfoTab (int ntab) |
Set the active tab on the info panel. More... | |
irr::IrrlichtDevice * | GetDevice () |
irr::video::IVideoDriver * | GetVideoDriver () |
irr::scene::ISceneManager * | GetSceneManager () |
irr::scene::ICameraSceneNode * | GetActiveCamera () |
irr::gui::IGUIEnvironment * | GetGUIEnvironment () |
void * | GetWindowId () const |
Get the window ID. | |
ChIrrGUI * | GetGUI () |
Return the Irrlicht ChIrrGUI object. | |
virtual void | BindAll () override |
Process all visual assets in the associated ChSystem. More... | |
virtual void | BindItem (std::shared_ptr< ChPhysicsItem > item) override |
Process the visual assets for the spcified physics item. More... | |
virtual void | UnbindItem (std::shared_ptr< ChPhysicsItem > item) override |
Remove the visual assets for the specified physics item from this visualization system. | |
virtual int | AddVisualModel (std::shared_ptr< ChVisualModel > model, const ChFrame<> &frame) override |
Add a visual model not associated with a physical item. More... | |
virtual int | AddVisualModel (std::shared_ptr< ChVisualShape > shape, const ChFrame<> &frame) override |
Add a visual model not associated with a physical item. More... | |
virtual void | UpdateVisualModel (int id, const ChFrame<> &frame) override |
Update the position of the specified visualization-only model. | |
virtual bool | Run () override |
Run the Irrlicht device. More... | |
virtual void | Quit () override |
Terminate the visualization system. | |
virtual void | BeginScene () override |
Perform any necessary operations at the beginning of each rendering frame. | |
virtual void | BeginScene (bool backBuffer, bool zBuffer) |
Clean the canvas at the beginning of each rendering frame. | |
virtual void | RenderFrame (const ChFrame<> &frame, double axis_length=1) override |
Render the specified reference frame. | |
virtual void | RenderCOGFrames (double axis_length=1) override |
Render COG frames for all bodies in the system. | |
virtual void | EndScene () override |
End the scene draw at the end of each animation frame. | |
irr::gui::IGUIFont * | GetMonospaceFont () const |
Return a fixed-size font for rendering GUI. | |
void | SetJPEGQuality (unsigned int quality) |
Set the JPEG quality level (between 0 and 100) for saved snapshots (default: 0). More... | |
virtual void | WriteImageToFile (const std::string &filename) override |
Create a snapshot of the last rendered frame and save it to the provided file. More... | |
bool | GetUtilityFlag () const |
Get internal utility flag value. | |
void | SetUtilityFlag (bool flag) |
Set internal utility flag value. | |
irr::SIrrlichtCreationParameters | GetCreationParameters () const |
Get device creation parameters. | |
void | SetCreationParameters (const irr::SIrrlichtCreationParameters &device_params) |
Set device creation parameters. | |
std::vector< std::shared_ptr< RTSCamera > > | GetCameras () const |
Get list of cameras defined for the scene. | |
![]() | |
void | SetVerbose (bool verbose) |
Enable/disable information terminal output during initialization (default: false). | |
bool | IsInitialized () const |
Indicate whether the visual system was initialized or not. | |
void | SetBackgroundColor (const ChColor &color) |
Set window background color. | |
virtual ChVector3d | GetCameraPosition () const |
Get the location of the current (active) camera. | |
virtual ChVector3d | GetCameraTarget () const |
Get the target (look-at) point of the current (active) camera. | |
void | UpdateCamera (int id, const ChVector3d &pos, ChVector3d target) |
Update the location and/or target points of the specified camera. | |
void | UpdateCamera (const ChVector3d &pos, ChVector3d target) |
std::vector< ChSystem * > & | GetSystems () |
Get the list of associated Chrono systems. | |
ChSystem & | GetSystem (int i) const |
Get the specified associated Chrono system. | |
double | GetSimulationTime () const |
Return the current simulated time. More... | |
double | GetRTF () const |
Return the overall real time factor. More... | |
double | GetSimulationRTF (unsigned int i) const |
Return the simulation real-time factor (simulation time / simulated time) for the specified associated system. More... | |
std::vector< double > | GetSimulationRTFs () const |
Return the simulation real-time factor (simulation time / simulated time) for all associated system. More... | |
unsigned int | GetNumBodies () const |
Get the number of bodies (across all visualized systems). More... | |
unsigned int | GetNumLinks () const |
Get the number of links (across all visualized systems). More... | |
unsigned int | GetNumMeshes () const |
Get the number of meshes (across all visualized systems). | |
unsigned int | GetNumShafts () const |
Get the number of shafts (across all visualized systems). | |
unsigned int | GetNumStates () const |
Get the number of coordinates at the velocity level (across all visualized systems). | |
unsigned int | GetNumConstraints () const |
Get the number of scalar constraints (across all visualized systems). | |
unsigned int | GetNumContacts () const |
Gets the number of contacts. | |
void | SetImageOutputDirectory (const std::string &dir) |
Set output directory for saving frame snapshots (default: "."). | |
void | SetImageOutput (bool val) |
Enable/disable writing of frame snapshots to file. | |
Protected Member Functions | |
virtual void | renderOtherGraphics () |
Render additional graphics. | |
virtual void | renderOtherStats (int left, int top) |
Render additional vehicle information. | |
void | renderLinGauge (const std::string &msg, double factor, bool sym, int xpos, int ypos, int length=120, int height=15) |
void | renderTextBox (const std::string &msg, int xpos, int ypos, int length=120, int height=15, irr::video::SColor color=irr::video::SColor(255, 20, 20, 20)) |
void | renderStats () |
![]() | |
void | CreateIrrNodes (const ChAssembly *assembly, std::unordered_set< const ChAssembly * > &trace) |
Create the ChIrrNodes for all visual model instances in the specified assembly. | |
void | CreateIrrNode (std::shared_ptr< ChPhysicsItem > item) |
Create the ChIrrNodeModel for the visual model instance of the specified physics item. | |
void | PopulateIrrNode (irr::scene::ISceneNode *node, std::shared_ptr< ChVisualModel > model, const ChFrame<> &parent_frame) |
Populate the ChIrrNodeModel for the visual model instance of the specified physics item. | |
void | PurgeIrrNodes () |
Purge Irrlicht nodes associated with a deleted physics item or with a deleted visual model. | |
void | AddShadowToIrrNode (irr::scene::ISceneNode *node) |
Add shadow to an Irrlicht node. | |
virtual void | OnSetup (ChSystem *sys) override |
Perform necessary setup operations at the beginning of a time step. | |
virtual void | OnUpdate (ChSystem *sys) override |
Perform necessary update operations at the end of a time step. | |
virtual void | OnClear (ChSystem *sys) override |
Remove all visualization objects from this visualization system. | |
Protected Attributes | |
ChChaseCameraEventReceiver * | m_camera_control |
event receiver for chase-cam control | |
ChVehicleEventReceiver * | m_vehicle_control |
event receiver for vehicle control | |
ChJoystickIRR * | m_joystick |
joystick setup | |
bool | m_renderStats |
turn on/off rendering of stats | |
int | m_HUD_x |
x-coordinate of upper-left corner of HUD elements | |
int | m_HUD_y |
y-coordinate of upper-left corner of HUD elements | |
![]() | |
std::vector< std::shared_ptr< RTSCamera > > | m_cameras |
list of cameras defined for the scene | |
std::vector< GridData > | m_grids |
list of visualization grids | |
std::unordered_map< ChPhysicsItem *, std::shared_ptr< ChIrrNodeModel > > | m_nodes |
scene nodes for physics items | |
std::vector< std::shared_ptr< ChIrrNodeVisual > > | m_vis_nodes |
scene nodes for vis-only models | |
bool | m_yup |
use Y-up if true, Z-up if false | |
std::string | m_win_title |
window title | |
irr::SIrrlichtCreationParameters | m_device_params |
Irrlicht device parameters. | |
irr::IrrlichtDevice * | m_device |
Irrlicht visualization device. | |
irr::gui::IGUIFont * | m_monospace_font |
Fixed-size font. | |
irr::scene::ISceneNode * | m_container |
Irrlicht scene container. | |
std::unique_ptr< ChIrrGUI > | m_gui |
associated Irrlicht GUI and event receiver | |
std::unique_ptr< EffectHandler > | m_effect_handler |
effect handler for shadow maps | |
bool | m_use_effects |
flag to enable/disable effects | |
bool | m_utility_flag = false |
utility flag that may be accessed from outside | |
irr::u32 | m_quality |
JPEG quality level (for saved snapshots) | |
irr::scene::IAnimatedMesh * | sphereMesh |
irr::scene::IMesh * | cubeMesh |
irr::scene::IMesh * | cylinderMesh |
irr::scene::IMesh * | capsuleMesh |
irr::scene::IMesh * | coneMesh |
bool | m_draw_colorbar |
std::string | m_colorbar_title |
ChVector2d | m_colorbar_range |
ChColormap::Type | m_colormap_type |
std::unique_ptr< ChColormap > | m_colormap |
ChVector2i | m_colorbar_pos |
ChVector2i | m_colorbar_size |
bool | m_colormap_bimodal |
![]() | |
bool | m_verbose |
terminal output | |
bool | m_initialized |
visual system initialized | |
ChColor | m_background_color |
window background color | |
ChTimer | m_timer |
timer for evaluating RTF | |
double | m_rtf |
overall real time factor | |
std::vector< ChSystem * > | m_systems |
associated Chrono system(s) | |
bool | m_write_images |
if true, save snapshots | |
std::string | m_image_dir |
directory for image files | |
Friends | |
class | ChChaseCameraEventReceiver |
class | ChVehicleEventReceiver |
Additional Inherited Members | |
![]() | |
enum | Type { Type::IRRLICHT, Type::VSG, Type::OptiX, NONE } |
Supported run-time visualization systems. More... | |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/visualization/ChVehicleVisualSystemIrrlicht.h
- /builds/uwsbel/chrono/src/chrono_vehicle/visualization/ChVehicleVisualSystemIrrlicht.cpp