Description
Definition of a suspension test rig.
#include <ChTrackTestRig.h>
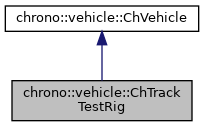
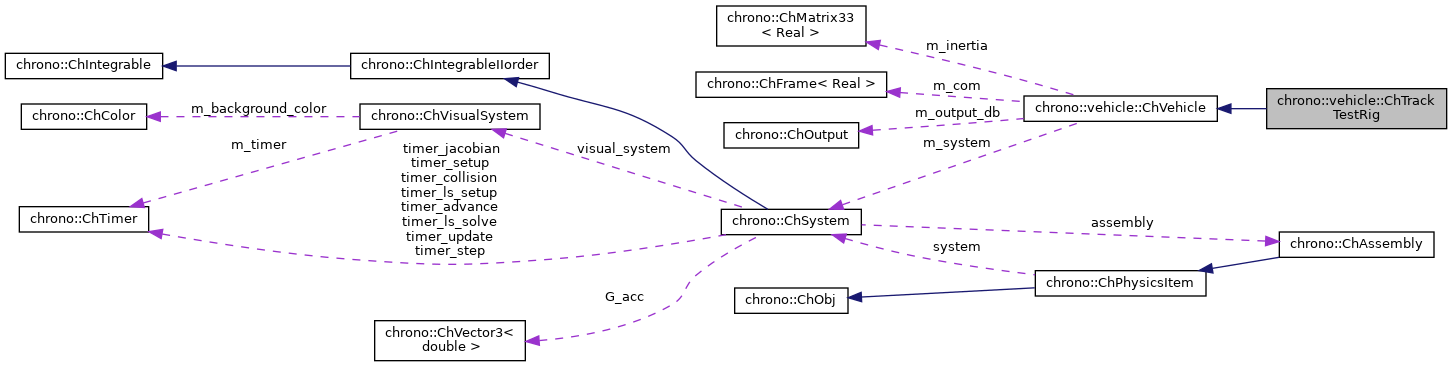
Public Member Functions | |
ChTrackTestRig () | |
Default constructor. | |
ChTrackTestRig (const std::string &filename, bool create_track=true, ChContactMethod contact_method=ChContactMethod::NSC, bool detracking_control=false) | |
Construct a test rig from specified track assembly JSON file. More... | |
ChTrackTestRig (std::shared_ptr< ChTrackAssembly > assembly, bool create_track=true, ChContactMethod contact_method=ChContactMethod::NSC, bool detracking_control=false) | |
Construct a test rig using the specified track assembly and subsystem locations. More... | |
~ChTrackTestRig () | |
Destructor. | |
void | SetDriver (std::shared_ptr< ChTrackTestRigDriver > driver) |
Set driver system. | |
void | SetInitialRideHeight (double height) |
Set the initial ride height (relative to the sprocket reference frame). More... | |
void | SetDisplacementLimit (double limit) |
Set the limits for post displacement (same for jounce and rebound). More... | |
void | SetDisplacementDelay (double delay) |
Set time delay before applying post displacement. More... | |
void | SetMaxTorque (double val) |
Set maximum sprocket torque. | |
void | SetSprocketVisualizationType (VisualizationType vis) |
Set visualization type for the sprocket subsystem (default: PRIMITIVES). | |
void | SetIdlerVisualizationType (VisualizationType vis) |
Set visualization type for the idler subsystem (default: PRIMITIVES). | |
void | SetSuspensionVisualizationType (VisualizationType vis) |
Set visualization type for the track suspension subsystem (default: PRIMITIVES). | |
void | SetIdlerWheelVisualizationType (VisualizationType vis) |
Set visualization type for the idler-wheel subsystem (default: PRIMITIVES). | |
void | SetRoadWheelVisualizationType (VisualizationType vis) |
Set visualization type for the road-wheel subsystem (default: PRIMITIVES). | |
void | SetTrackShoeVisualizationType (VisualizationType vis) |
Set visualization type for the track shoe subsystems (default: PRIMITIVES). | |
void | SetDriverLogFilename (const std::string &filename) |
Set filename for optional driver log. | |
void | SetTrackAssemblyOutput (bool state) |
Enable/disable output for the track assembly. More... | |
void | Initialize () |
Initialize this track test rig. | |
virtual void | Advance (double step) override |
Advance the state of the track test rig by the specified time step. | |
void | EnableCollision (int flags) |
Set collision flags for the various subsystems. More... | |
void | SetPostCollide (bool flag) |
Enable/disable collision for the rig posts (default: true). | |
void | MonitorContacts (int flags) |
Set contacts to be monitored. More... | |
void | SetRenderContactNormals (bool val) |
Render normals of all monitored contacts. | |
void | SetRenderContactForces (bool val, double scale) |
Render forces of all monitored contacts. | |
void | SetContactCollection (bool val) |
Turn on/off contact data collection. More... | |
bool | IsPartInContact (TrackedCollisionFlag::Enum part) const |
Return true if the specified vehicle part is currently experiencing a collision. | |
ChVector3d | GetSprocketResistiveTorque (VehicleSide side) const |
Return estimated resistive torque on the specified sprocket. More... | |
void | WriteContacts (const std::string &filename) |
Write contact information to file. More... | |
double | GetThrottleInput () const |
double | GetDisplacementInput (int index) |
double | GetActuatorDisp (int index) |
double | GetActuatorForce (int index) |
double | GetActuatorMarkerDist (int index) |
double | GetMass () const |
Get the rig total mass. More... | |
std::shared_ptr< ChTrackAssembly > | GetTrackAssembly () const |
Get the track assembly subsystem. | |
double | GetRideHeight () const |
Get current ride height (relative to the chassis reference frame). More... | |
void | LogDriverInputs () |
Log current driver inputs. | |
virtual void | LogConstraintViolations () override |
Log current constraint violations. | |
void | SetPlotOutput (double output_step) |
Enable/disable data collection for plot generation (default: false). More... | |
virtual void | PlotOutput (const std::string &out_dir, const std::string &out_name) |
Plot collected data. More... | |
![]() | |
virtual | ~ChVehicle () |
Destructor. | |
const std::string & | GetName () const |
Get the name identifier for this vehicle. | |
void | SetName (const std::string &name) |
Set the name identifier for this vehicle. | |
ChSystem * | GetSystem () |
Get a pointer to the Chrono ChSystem. | |
double | GetChTime () const |
Get the current simulation time of the underlying ChSystem. | |
std::shared_ptr< ChChassis > | GetChassis () const |
Get a handle to the vehicle's main chassis subsystem. | |
std::shared_ptr< ChChassisRear > | GetChassisRear (int id) const |
Get the specified specified rear chassis subsystem. | |
std::shared_ptr< ChChassisConnector > | GetChassisConnector (int id) const |
Get a handle to the specified chassis connector. | |
std::shared_ptr< ChBodyAuxRef > | GetChassisBody () const |
Get a handle to the vehicle's chassis body. | |
std::shared_ptr< ChBodyAuxRef > | GetChassisRearBody (int id) const |
Get a handle to the specified rear chassis body. | |
std::shared_ptr< ChPowertrainAssembly > | GetPowertrainAssembly () const |
Get the powertrain attached to this vehicle. | |
std::shared_ptr< ChEngine > | GetEngine () const |
Get the engine in the powertrain assembly (if a powertrain is attached). | |
std::shared_ptr< ChTransmission > | GetTransmission () const |
Get the transmission in the powertrain assembly (if a powertrain is attached). | |
double | GetMass () const |
Get the vehicle total mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current vehicle COM frame (relative to and expressed in the vehicle reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current vehicle inertia (relative to the vehicle COM frame). | |
const ChFrameMoving & | GetRefFrame () const |
Get the current vehicle reference frame. More... | |
const ChFrame & | GetTransform () const |
Get the current vehicle transform relative to the global frame. More... | |
const ChVector3d & | GetPos () const |
Get the vehicle global location. More... | |
ChQuaternion | GetRot () const |
Get the vehicle orientation. More... | |
double | GetRoll () const |
Get vehicle roll angle. More... | |
double | GetPitch () const |
Get vehicle pitch angle. More... | |
double | GetRoll (const ChTerrain &terrain) const |
Get vehicle roll angle (relative to local terrain). More... | |
double | GetPitch (const ChTerrain &terrain) const |
Get vehicle pitch angle (relative to local terrain). More... | |
double | GetSpeed () const |
Get the vehicle speed (velocity component in the vehicle forward direction). More... | |
double | GetSlipAngle () const |
Get the vehicle slip angle. More... | |
double | GetRollRate () const |
Get the vehicle roll rate. More... | |
double | GetPitchRate () const |
Get the vehicle pitch rate. More... | |
double | GetYawRate () const |
Get the vehicle yaw rate. More... | |
double | GetTurnRate () const |
Get the vehicle turn rate. More... | |
ChVector3d | GetPointLocation (const ChVector3d &locpos) const |
Get the global position of the specified point. More... | |
ChVector3d | GetPointVelocity (const ChVector3d &locpos) const |
Get the global velocity of the specified point. More... | |
ChVector3d | GetPointAcceleration (const ChVector3d &locpos) const |
Get the acceleration at the specified point. More... | |
ChVector3d | GetDriverPos () const |
Get the global location of the driver. | |
void | EnableRealtime (bool val) |
Enable/disable soft real-time (default: false). More... | |
double | GetRTF () const |
Get current estimated RTF (real time factor). More... | |
double | GetStepRTF () const |
Get current estimated step RTF (real time factor). More... | |
void | SetCollisionSystemType (ChCollisionSystem::Type collsys_type) |
Change the default collision detection system. More... | |
void | SetOutput (ChVehicleOutput::Type type, const std::string &out_dir, const std::string &out_name, double output_step) |
Enable output for this vehicle system. More... | |
void | SetOutput (ChVehicleOutput::Type type, std::ostream &out_stream, double output_step) |
Enable output for this vehicle system using an existing output stream. More... | |
void | InitializePowertrain (std::shared_ptr< ChPowertrainAssembly > powertrain) |
Initialize the given powertrain assembly and associate it to this vehicle. More... | |
void | SetChassisVisualizationType (VisualizationType vis) |
Set visualization mode for the chassis subsystem. | |
void | SetChassisRearVisualizationType (VisualizationType vis) |
Set visualization mode for the rear chassis subsystems. | |
void | SetChassisCollide (bool state) |
Enable/disable collision for the chassis subsystem. More... | |
virtual void | SetChassisVehicleCollide (bool state) |
Enable/disable collision between the chassis and all other vehicle subsystems. More... | |
void | SetChassisOutput (bool state) |
Enable/disable output from the chassis subsystem. | |
bool | HasBushings () const |
Return true if the vehicle model contains bushings. | |
Friends | |
class | ChTrackTestRigVisualSystemIrrlicht |
Additional Inherited Members | |
![]() | |
ChVehicle (const std::string &name, ChContactMethod contact_method=ChContactMethod::NSC) | |
Construct a vehicle system with an underlying ChSystem. More... | |
ChVehicle (const std::string &name, ChSystem *system) | |
Construct a vehicle system using the specified ChSystem. More... | |
void | SetSystem (ChSystem *sys) |
Set the associated Chrono system. | |
![]() | |
template<typename T > | |
static bool | AnyOutput (const std::vector< std::shared_ptr< T >> &list) |
Utility function for testing if any subsystem in a list generates output. | |
![]() | |
std::string | m_name |
vehicle name | |
ChSystem * | m_system |
pointer to the Chrono system | |
bool | m_ownsSystem |
true if system created at construction | |
double | m_mass |
total vehicle mass | |
ChFrame | m_com |
current vehicle COM (relative to the vehicle reference frame) | |
ChMatrix33 | m_inertia |
current total vehicle inertia (Relative to the vehicle COM frame) | |
bool | m_output |
generate ouput for this vehicle system | |
ChVehicleOutput * | m_output_db |
vehicle output database | |
double | m_output_step |
output time step | |
double | m_next_output_time |
time for next output | |
int | m_output_frame |
current output frame | |
std::shared_ptr< ChChassis > | m_chassis |
handle to the main chassis subsystem | |
ChChassisRearList | m_chassis_rear |
list of rear chassis subsystems (can be empty) | |
ChChassisConnectorList | m_chassis_connectors |
list of chassis connector (must match m_chassis_rear) | |
std::shared_ptr< ChPowertrainAssembly > | m_powertrain_assembly |
associated powertrain system | |
Constructor & Destructor Documentation
◆ ChTrackTestRig() [1/2]
chrono::vehicle::ChTrackTestRig::ChTrackTestRig | ( | const std::string & | filename, |
bool | create_track = true , |
||
ChContactMethod | contact_method = ChContactMethod::NSC , |
||
bool | detracking_control = false |
||
) |
Construct a test rig from specified track assembly JSON file.
- Parameters
-
[in] filename JSON file with rig specification [in] create_track include track shoes? [in] contact_method contact method [in] detracking_control detracking control
◆ ChTrackTestRig() [2/2]
chrono::vehicle::ChTrackTestRig::ChTrackTestRig | ( | std::shared_ptr< ChTrackAssembly > | assembly, |
bool | create_track = true , |
||
ChContactMethod | contact_method = ChContactMethod::NSC , |
||
bool | detracking_control = false |
||
) |
Construct a test rig using the specified track assembly and subsystem locations.
- Parameters
-
[in] assembly handle to the track assembly [in] create_track include track shoes? [in] contact_method contact method [in] detracking_control detracking control
Member Function Documentation
◆ EnableCollision()
|
inline |
Set collision flags for the various subsystems.
By default, collision is enabled for sprocket, idler, road wheels, and track shoes.
◆ GetMass()
double chrono::vehicle::ChTrackTestRig::GetMass | ( | ) | const |
Get the rig total mass.
This is simply the mass of the track subsystem.
◆ GetRideHeight()
double chrono::vehicle::ChTrackTestRig::GetRideHeight | ( | ) | const |
Get current ride height (relative to the chassis reference frame).
This estimate uses the average of the left and right posts.
◆ GetSprocketResistiveTorque()
|
inline |
Return estimated resistive torque on the specified sprocket.
This torque is available only if monitoring of contacts for that sprocket is enabled.
◆ MonitorContacts()
|
inline |
Set contacts to be monitored.
Contact information will be tracked for the specified subsystems.
◆ PlotOutput()
|
virtual |
Plot collected data.
When called (typically at the end of the simulation loop), the collected data is saved in ASCII format in a file with the specified name (and 'txt' extension) in the specified output directory. If the Chrono::Postprocess module is available (with gnuplot support), selected plots are generated.
Collected data includes:
- [col 1] time (s)
- [col 2-4] sprocket location (m)
- [col 5-7] idler location (m)
- [col 8-10] road wheel 1 location (m)
- [col 11-13] road wheel 2 location (m)
- ... ...
◆ SetContactCollection()
|
inline |
Turn on/off contact data collection.
If enabled, contact information will be collected for all monitored subsystems.
◆ SetDisplacementDelay()
|
inline |
Set time delay before applying post displacement.
Default value: 0
◆ SetDisplacementLimit()
|
inline |
Set the limits for post displacement (same for jounce and rebound).
Default value: 0
◆ SetInitialRideHeight()
|
inline |
Set the initial ride height (relative to the sprocket reference frame).
If not specified, the reference height is the track assembly design configuration.
◆ SetPlotOutput()
void chrono::vehicle::ChTrackTestRig::SetPlotOutput | ( | double | output_step | ) |
Enable/disable data collection for plot generation (default: false).
See PlotOutput details on data collected.
◆ SetTrackAssemblyOutput()
void chrono::vehicle::ChTrackTestRig::SetTrackAssemblyOutput | ( | bool | state | ) |
Enable/disable output for the track assembly.
See also ChVehicle::SetOuput.
◆ WriteContacts()
|
inline |
Write contact information to file.
If data collection was enabled and at least one subsystem is monitored, contact information is written (in CSV format) to the specified file.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/test_rig/ChTrackTestRig.h
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/test_rig/ChTrackTestRig.cpp