chrono::vehicle::ChHumanDriver Class Reference
Description
Closed-loop path-follower and speed maintaining driver model.
A driver model that combines a path steering controller and a speed controller. The controller adjusts the steering input to follow the prescribed path. The output also adjusts throttle and braking inputs in order to maintain a varying speed that depends on the curvature of the road.
#include <ChHumanDriver.h>
Inheritance diagram for chrono::vehicle::ChHumanDriver:
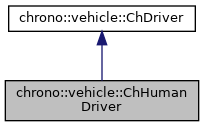
Collaboration diagram for chrono::vehicle::ChHumanDriver:
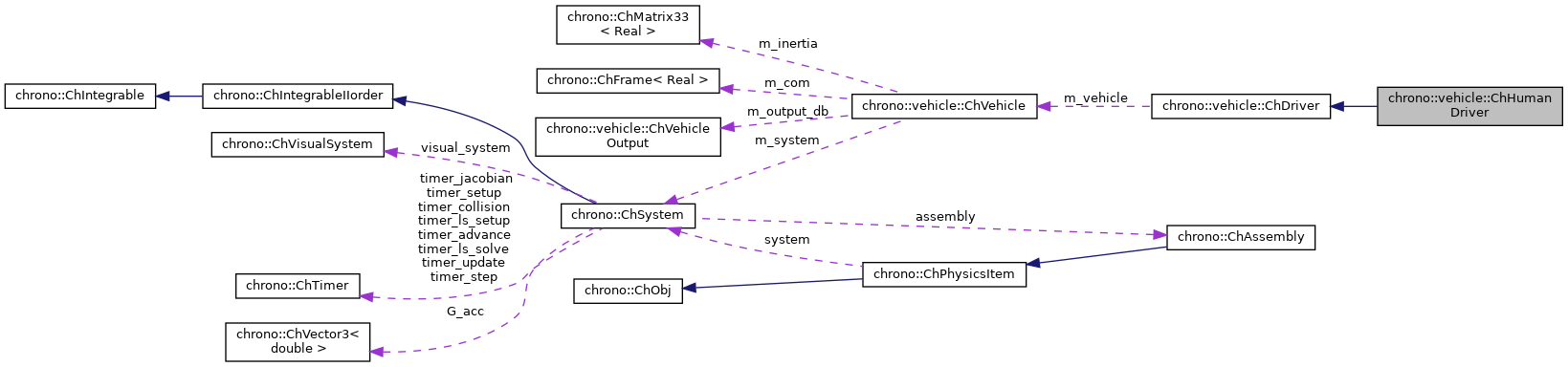
Public Member Functions | |
ChHumanDriver (ChVehicle &vehicle, std::shared_ptr< ChBezierCurve > path, const std::string &path_name, double road_width=5.0, double max_wheel_turn_angle=0, double axle_space=2.5) | |
Construct using the specified Bezier curve. More... | |
ChHumanDriver (const std::string &filename, ChVehicle &vehicle, std::shared_ptr< ChBezierCurve > path, const std::string &path_name, double road_width=5.0, double max_wheel_turn_angle=0, double axle_space=2.5) | |
Construct using a JSON parameter file. More... | |
virtual void | Advance (double step) override |
Advance the state of this driver system by the specified duration. | |
virtual void | Initialize () override |
Initialize the driver system. | |
void | ExportPathPovray (const std::string &out_dir) |
Export the Bezier curve for POV-Ray postprocessing. | |
void | SetPreviewTime (double Tp=0.5) |
void | SetLateralGains (double Klat=0.1, double Kug=0) |
void | SetLongitudinalGains (double Klong=0.1, double Kplus=0.1, double Kminus=0.1) |
void | SetSpeedRange (double u0=10.0, double umax=30.0) |
ChVector3d | GetTargetLocation () |
ChVector3d | GetSentinelLocation () |
double | GetTraveledDistance () |
double | GetAverageSpeed () |
double | GetMaxSpeed () |
double | GetMinSpeed () |
double | GetMaxLatAcc () |
double | GetMinLatAcc () |
![]() | |
ChDriver (ChVehicle &vehicle) | |
Construct a driver subsystem associated with the given vehicle. | |
double | GetThrottle () const |
Get the driver throttle input (in the range [0,1]). | |
double | GetSteering () const |
Get the driver steering input (in the range [-1,+1]). | |
double | GetBraking () const |
Get the driver braking input (in the range [0,1]). | |
double | GetClutch () const |
Get the driver clutch input (in the range [0,1]). | |
DriverInputs | GetInputs () const |
Get all current inputs at once. | |
virtual void | Synchronize (double time) |
Update the state of this driver system at the current time. | |
bool | LogInit (const std::string &filename) |
Initialize output file for recording driver inputs. | |
bool | Log (double time) |
Record the current driver inputs to the log file. | |
void | SetSteering (double steering) |
Overwrite the value for the driver steering input (input is clamped in [-1,+1]). | |
void | SetThrottle (double throttle) |
Overwrite the value for the driver throttle input (input is clamped in [0,+1]). | |
void | SetBraking (double braking) |
Overwrite the value for the driver braking input (input is clamped in [0,+1]). | |
void | SetClutch (double clutch) |
Overwrite the value for the clutch braking input (input is clamped in [0,+1]). | |
Additional Inherited Members | |
![]() | |
ChVehicle & | m_vehicle |
reference to associated vehicle | |
double | m_throttle |
current value of throttle input | |
double | m_steering |
current value of steering input | |
double | m_braking |
current value of braking input | |
double | m_clutch |
current value of clutch input | |
Constructor & Destructor Documentation
◆ ChHumanDriver() [1/2]
chrono::vehicle::ChHumanDriver::ChHumanDriver | ( | ChVehicle & | vehicle, |
std::shared_ptr< ChBezierCurve > | path, | ||
const std::string & | path_name, | ||
double | road_width = 5.0 , |
||
double | max_wheel_turn_angle = 0 , |
||
double | axle_space = 2.5 |
||
) |
Construct using the specified Bezier curve.
- Parameters
-
vehicle associated vehicle path Bezier curve with target path path_name name of the path curve road_width road width max_wheel_turn_angle maximum wheel turning angle axle_space wheel track
◆ ChHumanDriver() [2/2]
chrono::vehicle::ChHumanDriver::ChHumanDriver | ( | const std::string & | filename, |
ChVehicle & | vehicle, | ||
std::shared_ptr< ChBezierCurve > | path, | ||
const std::string & | path_name, | ||
double | road_width = 5.0 , |
||
double | max_wheel_turn_angle = 0 , |
||
double | axle_space = 2.5 |
||
) |
Construct using a JSON parameter file.
- Parameters
-
filename path of the JSON file vehicle associated vehicle path Bezier curve with target path path_name name of the path curve road_width road width max_wheel_turn_angle maximum wheel turning angle axle_space wheel track
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/driver/ChHumanDriver.h
- /builds/uwsbel/chrono/src/chrono_vehicle/driver/ChHumanDriver.cpp