Description
Driver.
#include <ChAIDriver.h>
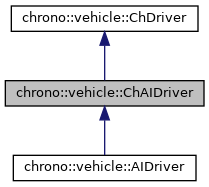
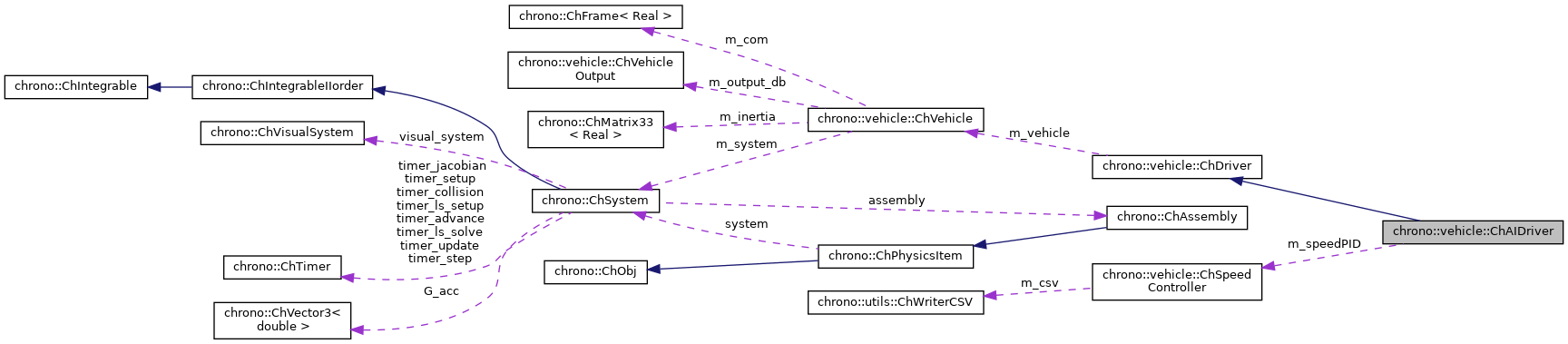
Public Member Functions | |
ChAIDriver (ChVehicle &vehicle) | |
void | SetSpeedControllerGains (double Kp, double Ki, double Kd) |
Set speed controller PID gains. | |
void | SetThresholdThrottle (double val) |
Specify the throttle value below which braking is enabled. More... | |
ChSpeedController & | GetSpeedController () |
Get the underlying speed controller object. | |
virtual double | CalculateSteering (double front_axle_angle, double rear_axle_angle)=0 |
Return the value of the vehicle steering input (in [-1,+1]) given the desired front and rear wheel angles. More... | |
void | Synchronize (double time, double long_acc, double front_axle_angle, double rear_axle_angle) |
Set the controls from the autonomy stack: longitudinal acceleration (m/s2) and the front & rear wheel angles (in radians). More... | |
virtual void | Advance (double step) override |
Advance the state of this driver system by the specified duration. | |
![]() | |
ChDriver (ChVehicle &vehicle) | |
Construct a driver subsystem associated with the given vehicle. | |
double | GetThrottle () const |
Get the driver throttle input (in the range [0,1]). | |
double | GetSteering () const |
Get the driver steering input (in the range [-1,+1]). | |
double | GetBraking () const |
Get the driver braking input (in the range [0,1]). | |
double | GetClutch () const |
Get the driver clutch input (in the range [0,1]). | |
DriverInputs | GetInputs () const |
Get all current inputs at once. | |
virtual void | Initialize () |
Initialize this driver system. | |
bool | LogInit (const std::string &filename) |
Initialize output file for recording driver inputs. | |
bool | Log (double time) |
Record the current driver inputs to the log file. | |
void | SetSteering (double steering) |
Overwrite the value for the driver steering input (input is clamped in [-1,+1]). | |
void | SetThrottle (double throttle) |
Overwrite the value for the driver throttle input (input is clamped in [0,+1]). | |
void | SetBraking (double braking) |
Overwrite the value for the driver braking input (input is clamped in [0,+1]). | |
void | SetClutch (double clutch) |
Overwrite the value for the clutch braking input (input is clamped in [0,+1]). | |
Protected Attributes | |
ChSpeedController | m_speedPID |
speed controller | |
double | m_throttle_threshold |
throttle value below which brakes are applied | |
double | m_target_speed |
current value of target speed | |
double | m_last_time |
last time | |
double | m_last_speed |
last target speed | |
![]() | |
ChVehicle & | m_vehicle |
reference to associated vehicle | |
double | m_throttle |
current value of throttle input | |
double | m_steering |
current value of steering input | |
double | m_braking |
current value of braking input | |
double | m_clutch |
current value of clutch input | |
Member Function Documentation
◆ CalculateSteering()
|
pure virtual |
Return the value of the vehicle steering input (in [-1,+1]) given the desired front and rear wheel angles.
The underlying assumption is of Ackermann steering of a bicycle model. Note that the Chrono::Vehicle ISO reference frame convention implies that a positive front angle corresponds to a turn to the left (i.e., positive value of vehicle steering input).
Implemented in chrono::vehicle::AIDriver.
◆ SetThresholdThrottle()
|
inline |
Specify the throttle value below which braking is enabled.
If the vehicle is moving faster than the set speed, the controller attempts to reduce speed either by reducing the throttle input (if the current throttle input is above the threshold value) or by applying brakes (otherwise).
◆ Synchronize()
void chrono::vehicle::ChAIDriver::Synchronize | ( | double | time, |
double | long_acc, | ||
double | front_axle_angle, | ||
double | rear_axle_angle | ||
) |
Set the controls from the autonomy stack: longitudinal acceleration (m/s2) and the front & rear wheel angles (in radians).
The underlying assumption is of Ackermann steering of a bicycle model.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/driver/ChAIDriver.h
- /builds/uwsbel/chrono/src/chrono_vehicle/driver/ChAIDriver.cpp