Description
Class for the basic properties of materials in an elastoplastic continuum, with strain yield limit based on Von Mises yield.
#include <ChContinuumMaterial.h>
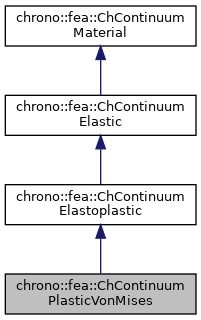
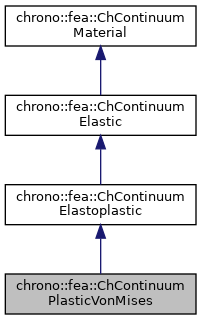
Public Member Functions | |
ChContinuumPlasticVonMises (double young=10000000, double poisson=0.4, double density=1000, double elastic_yield=0.1, double plastic_yield=0.2) | |
Create a continuum isotropic elastoplastic material, where you can define also plastic and elastic max. More... | |
ChContinuumPlasticVonMises (const ChContinuumPlasticVonMises &other) | |
void | SetElasticYield (double elastic_yield) |
Set the elastic yield modulus as the maximum VonMises equivalent strain that can be withstood by material before starting plastic flow. More... | |
double | GetElasticYield () const |
Get the elastic yield modulus. | |
void | SetPlasticYield (double plastic_yield) |
Set the plastic yield modulus as the maximum VonMises equivalent strain that can be withstood by material before fracture. More... | |
double | GetPlasticYield () const |
Get the plastic yield modulus. | |
virtual void | SetPlasticFlowRate (double flow_rate) override |
Set the plastic flow rate. More... | |
virtual double | GetPlasticFlowRate () const override |
Set the plastic flow rate. | |
virtual double | ComputeYieldFunction (const ChStressTensor<> &stress) const override |
Return a scalar value that is 0 on the yield surface, <0 inside (elastic), >0 outside (incompatible->plastic flow) | |
virtual void | ComputeReturnMapping (ChStrainTensor<> &plasticstrainflow, const ChStrainTensor<> &incrementstrain, const ChStrainTensor<> &lastelasticstrain, const ChStrainTensor<> &lastplasticstrain) const override |
Correct the strain-stress by enforcing that elastic stress must remain on the yield surface, computing a plastic flow to be added to plastic strain while integrating. | |
virtual void | ComputePlasticStrainFlow (ChStrainTensor<> &plasticstrainflow, const ChStrainTensor<> &totstrain) const override |
Compute plastic strain flow (flow derivative dE_plast/dt) from strain, according to VonMises strain yield theory. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
![]() | |
ChContinuumElastoplastic (double young=10000000, double poisson=0.4, double density=1000) | |
![]() | |
ChContinuumElastic (double young=10000000, double poisson=0.4, double density=1000) | |
ChContinuumElastic (const ChContinuumElastic &other) | |
void | SetYoungModulus (double E) |
Set the Young elastic modulus, in Pa (N/m^2), as the ratio of the uniaxial stress over the uniaxial strain, for hookean materials. | |
double | GetYoungModulus () const |
Get the Young elastic modulus, in Pa (N/m^2). | |
void | SetPoissonRatio (double v) |
Set the Poisson ratio, as v=-transverse_strain/axial_strain, so takes into account the 'squeezing' effect of materials that are pulled. More... | |
double | GetPoissonRatio () const |
Get the Poisson ratio, as v=-transverse_strain/axial_strain. | |
void | SetShearModulus (double G) |
Set the shear modulus G, in Pa (N/m^2). More... | |
double | GetShearModulus () const |
Get the shear modulus G, in Pa (N/m^2) | |
double | GetLameFirstParam () const |
Get Lamé first parameter (the second is shear modulus, so GetShearModulus() ) | |
double | GetBulkModulus () const |
Get bulk modulus (increase of pressure for decrease of volume), in Pa. | |
double | GetPWaveModulus () const |
Get P-wave modulus (if V=speed of propagation of a P-wave, then (M/density)=V^2 ) | |
void | ComputeStressStrainMatrix () |
Computes Elasticity matrix and stores the value in this->StressStrainMatrix Note: is performed every time you change a material parameter. | |
ChMatrixDynamic & | GetStressStrainMatrix () |
Get the Elasticity matrix. | |
void | ComputeElasticStress (ChStressTensor<> &stress, const ChStrainTensor<> &strain) const |
Compute elastic stress from elastic strain (using column tensors, in Voight notation) | |
void | ComputeElasticStrain (ChStrainTensor<> &strain, const ChStressTensor<> &stress) const |
Compute elastic strain from elastic stress (using column tensors, in Voight notation) | |
void | SetRayleighDampingAlpha (double alpha) |
Set the Rayleigh mass-proportional damping factor alpha, to build damping R as R=alpha*M + beta*K. | |
double | GetRayleighDampingAlpha () const |
Set the Rayleigh mass-proportional damping factor alpha, in R=alpha*M + beta*K. | |
void | SetRayleighDampingBeta (double beta) |
Set the Rayleigh stiffness-proportional damping factor beta, to build damping R as R=alpha*M + beta*K. | |
double | GetRayleighDampingBeta () const |
Set the Rayleigh stiffness-proportional damping factor beta, in R=alpha*M + beta*K. | |
![]() | |
ChContinuumMaterial (double density=1000) | |
ChContinuumMaterial (const ChContinuumMaterial &other) | |
void | SetDensity (double density) |
Set the density of the material, in kg/m^2. | |
double | GetDensity () const |
Get the density of the material, in kg/m^2. | |
Additional Inherited Members | |
![]() | |
double | m_density |
Constructor & Destructor Documentation
◆ ChContinuumPlasticVonMises()
chrono::fea::ChContinuumPlasticVonMises::ChContinuumPlasticVonMises | ( | double | young = 10000000 , |
double | poisson = 0.4 , |
||
double | density = 1000 , |
||
double | elastic_yield = 0.1 , |
||
double | plastic_yield = 0.2 |
||
) |
Create a continuum isotropic elastoplastic material, where you can define also plastic and elastic max.
stress (yield limits for transition elastic->plastic and plastic->fracture).
Member Function Documentation
◆ SetElasticYield()
|
inline |
Set the elastic yield modulus as the maximum VonMises equivalent strain that can be withstood by material before starting plastic flow.
It defines the transition elastic->plastic.
◆ SetPlasticFlowRate()
|
inlineoverridevirtual |
Set the plastic flow rate.
The lower the value, the slower the plastic flow during dynamic simulations.
Implements chrono::fea::ChContinuumElastoplastic.
◆ SetPlasticYield()
|
inline |
Set the plastic yield modulus as the maximum VonMises equivalent strain that can be withstood by material before fracture.
It defines the transition plastic->fracture.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChContinuumMaterial.h
- /builds/uwsbel/chrono/src/chrono/fea/ChContinuumMaterial.cpp