Description
Class for the basic properties of elastoplastic materials of Drucker-Prager type, that are useful for simulating soils.
#include <ChContinuumMaterial.h>
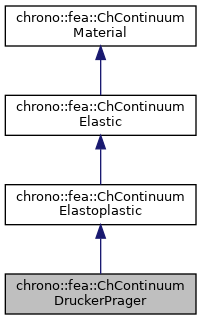
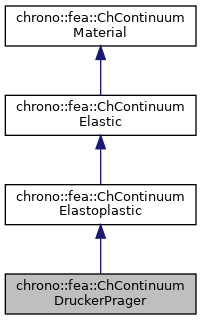
Public Member Functions | |
ChContinuumDruckerPrager (double young=10000000, double poisson=0.4, double density=1000, double elastic_yield=0.1, double alpha=0.5, double dilatancy=0) | |
Create a continuum isotropic Drucker-Prager material. | |
ChContinuumDruckerPrager (const ChContinuumDruckerPrager &other) | |
void | SetElasticYield (double elastic_yield) |
Set the D-P yield modulus C, for Drucker-Prager yield. More... | |
double | GetElasticYield () const |
Get the elastic yield modulus C. | |
void | SetInternalFriction (double alpha) |
Set the internal friction coefficient A. | |
double | GetInternalFriction () const |
Get the internal friction coefficient A. | |
void | SetFromMohrCoulomb (double phi, double cohesion, bool inner_approx=true) |
Sets the C and A parameters of the Drucker-Prager model starting from more 'practical' values of inner friction angle phi and cohesion, as used in the faceted Mohr-Coulomb model. More... | |
virtual void | SetPlasticFlowRate (double flow_rate) override |
Set the plastic flow rate multiplier. More... | |
virtual double | GetPlasticFlowRate () const override |
Get the flow rate multiplier. | |
void | SetDilatancy (double dilatancy) |
Set the internal dilatation coefficient (usually 0.. < int.friction) | |
double | GetDilatancy () const |
Get the internal dilatation coefficient. | |
void | SetHardeningLimit (double hl) |
Set the hardening limit (usually a bit larger than yield), or softening. | |
double | GetHardeningLimit () const |
Get the hardening limit. | |
void | SetHardeningSpeed (double hl) |
Set the hardening inverse speed coeff. More... | |
double | GetHardeningSpeed () const |
Get the hardening speed. | |
virtual double | ComputeYieldFunction (const ChStressTensor<> &stress) const override |
Return a scalar value that is 0 on the yield surface, <0 inside (elastic), >0 outside (incompatible->plastic flow) | |
virtual void | ComputeReturnMapping (ChStrainTensor<> &plasticstrainflow, const ChStrainTensor<> &incrementstrain, const ChStrainTensor<> &lastelasticstrain, const ChStrainTensor<> &lastplasticstrain) const override |
Correct the strain-stress by enforcing that elastic stress must remain on the yield surface, computing a plastic flow to be added to plastic strain while integrating. | |
virtual void | ComputePlasticStrainFlow (ChStrainTensor<> &plasticstrainflow, const ChStrainTensor<> &totstrain) const override |
Compute plastic strain flow direction from strain according to Drucker-Prager. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) override |
virtual void | ArchiveIn (ChArchiveIn &archive_in) override |
![]() | |
ChContinuumElastoplastic (double young=10000000, double poisson=0.4, double density=1000) | |
![]() | |
ChContinuumElastic (double young=10000000, double poisson=0.4, double density=1000) | |
ChContinuumElastic (const ChContinuumElastic &other) | |
void | SetYoungModulus (double E) |
Set the Young elastic modulus, in Pa (N/m^2), as the ratio of the uniaxial stress over the uniaxial strain, for hookean materials. | |
double | GetYoungModulus () const |
Get the Young elastic modulus, in Pa (N/m^2). | |
void | SetPoissonRatio (double v) |
Set the Poisson ratio, as v=-transverse_strain/axial_strain, so takes into account the 'squeezing' effect of materials that are pulled. More... | |
double | GetPoissonRatio () const |
Get the Poisson ratio, as v=-transverse_strain/axial_strain. | |
void | SetShearModulus (double G) |
Set the shear modulus G, in Pa (N/m^2). More... | |
double | GetShearModulus () const |
Get the shear modulus G, in Pa (N/m^2) | |
double | GetLameFirstParam () const |
Get Lamé first parameter (the second is shear modulus, so GetShearModulus() ) | |
double | GetBulkModulus () const |
Get bulk modulus (increase of pressure for decrease of volume), in Pa. | |
double | GetPWaveModulus () const |
Get P-wave modulus (if V=speed of propagation of a P-wave, then (M/density)=V^2 ) | |
void | ComputeStressStrainMatrix () |
Computes Elasticity matrix and stores the value in this->StressStrainMatrix Note: is performed every time you change a material parameter. | |
ChMatrixDynamic & | GetStressStrainMatrix () |
Get the Elasticity matrix. | |
void | ComputeElasticStress (ChStressTensor<> &stress, const ChStrainTensor<> &strain) const |
Compute elastic stress from elastic strain (using column tensors, in Voight notation) | |
void | ComputeElasticStrain (ChStrainTensor<> &strain, const ChStressTensor<> &stress) const |
Compute elastic strain from elastic stress (using column tensors, in Voight notation) | |
void | SetRayleighDampingAlpha (double alpha) |
Set the Rayleigh mass-proportional damping factor alpha, to build damping R as R=alpha*M + beta*K. | |
double | GetRayleighDampingAlpha () const |
Set the Rayleigh mass-proportional damping factor alpha, in R=alpha*M + beta*K. | |
void | SetRayleighDampingBeta (double beta) |
Set the Rayleigh stiffness-proportional damping factor beta, to build damping R as R=alpha*M + beta*K. | |
double | GetRayleighDampingBeta () const |
Set the Rayleigh stiffness-proportional damping factor beta, in R=alpha*M + beta*K. | |
![]() | |
ChContinuumMaterial (double density=1000) | |
ChContinuumMaterial (const ChContinuumMaterial &other) | |
void | SetDensity (double density) |
Set the density of the material, in kg/m^2. | |
double | GetDensity () const |
Get the density of the material, in kg/m^2. | |
Additional Inherited Members | |
![]() | |
double | m_density |
Member Function Documentation
◆ SetElasticYield()
|
inline |
Set the D-P yield modulus C, for Drucker-Prager yield.
It defines the transition elastic->plastic.
◆ SetFromMohrCoulomb()
void chrono::fea::ChContinuumDruckerPrager::SetFromMohrCoulomb | ( | double | phi, |
double | cohesion, | ||
bool | inner_approx = true |
||
) |
Sets the C and A parameters of the Drucker-Prager model starting from more 'practical' values of inner friction angle phi and cohesion, as used in the faceted Mohr-Coulomb model.
Use the optional parameter inner_approx to set if the faceted Mohr-Coulomb must be approximated with D-P inscribed (default) or circumscribed.
◆ SetHardeningSpeed()
|
inline |
Set the hardening inverse speed coeff.
for exponential hardening (the larger, the slower the hardening or softening process that will asymptotically make yield = hardening_limit )
◆ SetPlasticFlowRate()
|
inlineoverridevirtual |
Set the plastic flow rate multiplier.
The lower the value, the slower the plastic flow during dynamic simulations.
Implements chrono::fea::ChContinuumElastoplastic.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChContinuumMaterial.h
- /builds/uwsbel/chrono/src/chrono/fea/ChContinuumMaterial.cpp