Description
Load representing a concentrated force acting on a rigid body.
The force can rotate together with the body (if in body local coordinates) or not. The application point can follow the body (if in body local coordinates) or not. The magnitude of the applied force can be optionally modulated by a function of time. The default modulation is the constant function with value 1.
#include <ChLoadsBody.h>
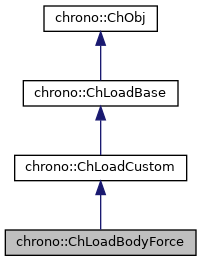
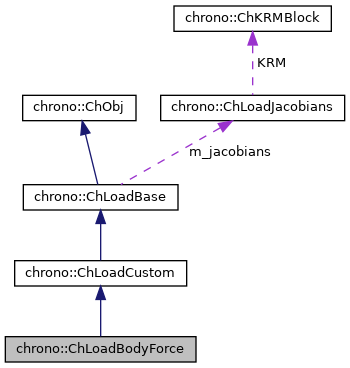
Public Member Functions | |
ChLoadBodyForce (std::shared_ptr< ChBody > body, const ChVector3d &force, bool local_force, const ChVector3d &point, bool local_point=true) | |
virtual ChLoadBodyForce * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
void | SetForce (const ChVector3d &force, bool is_local) |
Set the constant force vector. More... | |
ChVector3d | GetForce () const |
Return the current force vector (scaled by the current modulation value). | |
void | SetApplicationPoint (const ChVector3d &point, const bool is_local) |
Set the application point of force, assumed to be constant. More... | |
ChVector3d | GetApplicationPoint () const |
Return the location of the application point. | |
void | SetModulationFunction (std::shared_ptr< ChFunction > modulation) |
Set modulation function. More... | |
virtual void | ComputeQ (ChState *state_x, ChStateDelta *state_w) override |
Compute the generalized load(s). More... | |
![]() | |
ChLoadCustom (std::shared_ptr< ChLoadable > loadable_object) | |
virtual int | LoadGetNumCoordsPosLevel () override |
Gets the number of DOFs affected by this load (position part). | |
virtual int | LoadGetNumCoordsVelLevel () override |
Gets the number of DOFs affected by this load (speed part). | |
virtual void | LoadGetStateBlock_x (ChState &mD) override |
Gets all the current DOFs packed in a single vector (position part). | |
virtual void | LoadGetStateBlock_w (ChStateDelta &mD) override |
Gets all the current DOFs packed in a single vector (speed part). | |
virtual void | LoadStateIncrement (const ChState &x, const ChStateDelta &dw, ChState &x_new) override |
Increment a packed state (e.g., as obtained by LoadGetStateBlock_x()) by a given packed state-delta. More... | |
virtual int | LoadGetNumFieldCoords () override |
Number of coordinates in the interpolated field. More... | |
virtual void | ComputeJacobian (ChState *state_x, ChStateDelta *state_w) override |
Compute Jacobian matrices K=-dQ/dx, R=-dQ/dv, and M=-dQ/da. More... | |
virtual void | LoadIntLoadResidual_F (ChVectorDynamic<> &R, double c) override |
Add the internal loads Q (pasted at global offsets) into a global vector R, multiplied by a scaling factor c. More... | |
virtual void | LoadIntLoadResidual_Mv (ChVectorDynamic<> &R, const ChVectorDynamic<> &w, double c) override |
Increment a vector R with the matrix-vector product M*w, scaled by the factor c. More... | |
virtual void | LoadIntLoadLumpedMass_Md (ChVectorDynamic<> &Md, double &err, const double c) override |
Add the lumped mass to an Md vector, representing a mass diagonal matrix. More... | |
virtual void | CreateJacobianMatrices () override |
Create the Jacobian loads if needed and set the ChVariables referenced by the sparse KRM block. | |
virtual ChVectorDynamic & | GetQ () |
Access the generalized load vector Q. | |
![]() | |
ChLoadJacobians * | GetJacobians () |
Access the Jacobians (if any, i.e. if this is a stiff load). | |
virtual void | InjectKRMMatrices (ChSystemDescriptor &descriptor) |
Register with the given system descriptor any ChKRMBlock objects associated with this item. | |
virtual void | LoadKRMMatrices (double Kfactor, double Rfactor, double Mfactor) |
Compute and load current stiffnes (K), damping (R), and mass (M) matrices in encapsulated ChKRMBlock objects. More... | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Get the unique integer identifier of this object. More... | |
void | SetTag (int tag) |
Set an object integer tag (default: -1). More... | |
int | GetTag () const |
Get the tag of this object. | |
void | SetName (const std::string &myname) |
Set the name of this object. | |
const std::string & | GetName () const |
Get the name of this object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) |
Method to allow de-serialization of transient data from archives. | |
virtual std::string & | ArchiveContainerName () |
Additional Inherited Members | |
![]() | |
std::shared_ptr< ChLoadable > | loadable |
ChVectorDynamic | load_Q |
![]() | |
int | GenerateUniqueIdentifier () |
![]() | |
ChLoadJacobians * | m_jacobians |
![]() | |
double | ChTime |
object simulation time | |
std::string | m_name |
object name | |
int | m_identifier |
object unique identifier | |
int | m_tag |
user-supplied tag | |
Constructor & Destructor Documentation
◆ ChLoadBodyForce()
chrono::ChLoadBodyForce::ChLoadBodyForce | ( | std::shared_ptr< ChBody > | body, |
const ChVector3d & | force, | ||
bool | local_force, | ||
const ChVector3d & | point, | ||
bool | local_point = true |
||
) |
- Parameters
-
body object to apply load to force force to apply local_force force is in body local coords point application point for the force local_point application point is in body local coords
Member Function Documentation
◆ ComputeQ()
|
overridevirtual |
Compute the generalized load(s).
- Parameters
-
state_x state position to evaluate Q state_w state speed to evaluate Q
Implements chrono::ChLoadBase.
◆ SetApplicationPoint()
void chrono::ChLoadBodyForce::SetApplicationPoint | ( | const ChVector3d & | point, |
const bool | is_local | ||
) |
Set the application point of force, assumed to be constant.
It can be expressed in absolute coordinates or body local coordinates
◆ SetForce()
void chrono::ChLoadBodyForce::SetForce | ( | const ChVector3d & | force, |
bool | is_local | ||
) |
Set the constant force vector.
It can be expressed in absolute coordinates or body local coordinates. This value is optionally modulated by a function of time.
◆ SetModulationFunction()
|
inline |
Set modulation function.
This is a function of time which (optionally) modulates the specified applied force. By default the modulation is a constant function, always returning a value of 1.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChLoadsBody.h
- /builds/uwsbel/chrono/src/chrono/physics/ChLoadsBody.cpp