Description
Base class for all "motor" constraints between two frames on two bodies.
Look for children classes for specialized behaviors, for example chrono::ChLinkMotorRotationAngle.
#include <ChLinkMotor.h>
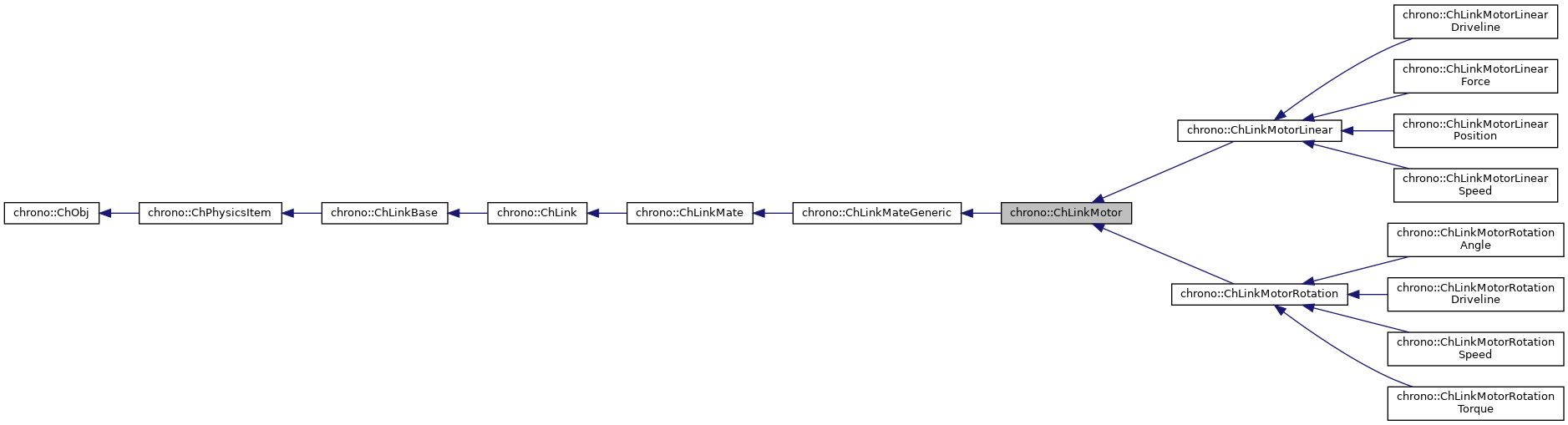
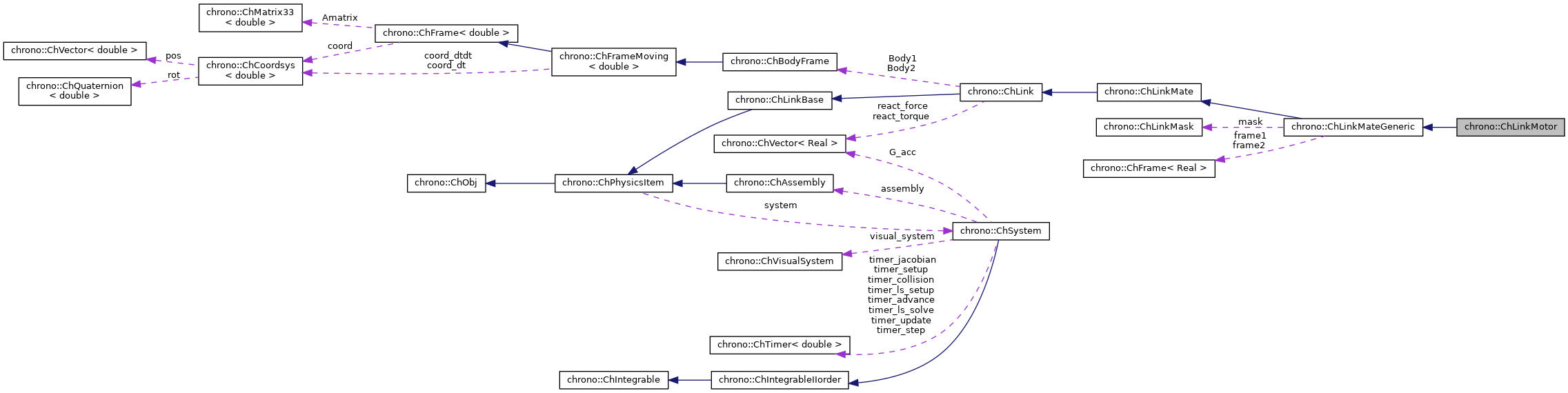
Public Member Functions | |
ChLinkMotor (const ChLinkMotor &other) | |
void | SetMotorFunction (const std::shared_ptr< ChFunction > function) |
Set the actuation function of time F(t). More... | |
std::shared_ptr< ChFunction > | GetMotorFunction () const |
Get the actuation function F(t). | |
virtual ChLinkMotor * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual void | Update (double mytime, bool update_assets) override |
Update state of the LinkMotor. | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow deserialization of transient data from archives. | |
![]() | |
ChLinkMateGeneric (bool mc_x=true, bool mc_y=true, bool mc_z=true, bool mc_rx=true, bool mc_ry=true, bool mc_rz=true) | |
ChLinkMateGeneric (const ChLinkMateGeneric &other) | |
virtual ChCoordsys | GetLinkRelativeCoords () override |
Get the link coordinate system, expressed relative to Body2 (the 'master' body). More... | |
virtual ChFrame | GetVisualModelFrame (unsigned int nclone=0) override |
Get the reference frame (expressed in and relative to the absolute frame) of the visual model. More... | |
ChFrame & | GetFrame1 () |
Access the coordinate system considered attached to body1. More... | |
ChFrame & | GetFrame2 () |
Access the coordinate system considered attached to body2. More... | |
bool | IsConstrainedX () const |
bool | IsConstrainedY () const |
bool | IsConstrainedZ () const |
bool | IsConstrainedRx () const |
bool | IsConstrainedRy () const |
bool | IsConstrainedRz () const |
void | SetConstrainedCoords (bool mc_x, bool mc_y, bool mc_z, bool mc_rx, bool mc_ry, bool mc_rz) |
Sets which movements (of frame 1 respect to frame 2) are constrained. | |
virtual void | Initialize (std::shared_ptr< ChBodyFrame > mbody1, std::shared_ptr< ChBodyFrame > mbody2, ChFrame<> mabsframe) |
Initialize the generic mate, given the two bodies to be connected, and the absolute position of the mate (the two frames to connect on the bodies will be initially coincindent to that frame). More... | |
virtual void | Initialize (std::shared_ptr< ChBodyFrame > mbody1, std::shared_ptr< ChBodyFrame > mbody2, bool pos_are_relative, ChFrame<> mframe1, ChFrame<> mframe2) |
Initialize the generic mate, given the two bodies to be connected, the positions of the two frames to connect on the bodies (each expressed in body or abs. More... | |
virtual void | Initialize (std::shared_ptr< ChBodyFrame > mbody1, std::shared_ptr< ChBodyFrame > mbody2, bool pos_are_relative, ChVector<> mpt1, ChVector<> mpt2, ChVector<> mnorm1, ChVector<> mnorm2) |
Initialization based on passing two vectors (point + dir) on the two bodies, which will represent the X axes of the two frames (Y and Z will be built from the X vector via Gram Schmidt orthonormalization). More... | |
virtual int | RestoreRedundant () override |
If some constraint is redundant, return to normal state. | |
virtual void | SetDisabled (bool mdis) override |
User can use this to enable/disable all the constraint of the link as desired. | |
virtual void | SetBroken (bool mon) override |
Ex:3rd party software can set the 'broken' status via this method. | |
virtual int | GetDOC () override |
Get the number of scalar constraints, if any, in this item. | |
virtual int | GetDOC_c () override |
Get the number of scalar constraints, if any, in this item (only bilateral constr.) Children classes might override this. | |
virtual int | GetDOC_d () override |
Get the number of scalar constraints, if any, in this item (only unilateral constr.) Children classes might override this. | |
virtual ChVectorDynamic | GetConstraintViolation () const override |
Link violation (residuals of the link constraint equations). | |
virtual void | IntStateGatherReactions (const unsigned int off_L, ChVectorDynamic<> &L) override |
From item's reaction forces to global reaction vector. More... | |
virtual void | IntStateScatterReactions (const unsigned int off_L, const ChVectorDynamic<> &L) override |
From global reaction vector to item's reaction forces. More... | |
virtual void | IntLoadResidual_CqL (const unsigned int off_L, ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) override |
Takes the term Cq'*L, scale and adds to R at given offset: R += c*Cq'*L. More... | |
virtual void | IntLoadConstraint_C (const unsigned int off, ChVectorDynamic<> &Qc, const double c, bool do_clamp, double recovery_clamp) override |
Takes the term C, scale and adds to Qc at given offset: Qc += c*C. More... | |
virtual void | IntLoadConstraint_Ct (const unsigned int off, ChVectorDynamic<> &Qc, const double c) override |
Takes the term Ct, scale and adds to Qc at given offset: Qc += c*Ct. More... | |
virtual void | IntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R, const unsigned int off_L, const ChVectorDynamic<> &L, const ChVectorDynamic<> &Qc) override |
Prepare variables and constraints to accommodate a solution: More... | |
virtual void | IntFromDescriptor (const unsigned int off_v, ChStateDelta &v, const unsigned int off_L, ChVectorDynamic<> &L) override |
After a solver solution, fetch values from variables and constraints into vectors: More... | |
virtual void | InjectConstraints (ChSystemDescriptor &mdescriptor) override |
Tell to a system descriptor that there are constraints of type ChConstraint in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | ConstraintsBiReset () override |
Sets to zero the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_C (double factor=1, double recovery_clamp=0.1, bool do_clamp=false) override |
Adds the current C (constraint violation) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Ct (double factor=1) override |
Adds the current Ct (partial t-derivative, as in C_dt=0-> [Cq]*q_dt=-Ct) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsLoadJacobians () override |
Adds the current jacobians in encapsulated ChConstraints. | |
virtual void | ConstraintsFetch_react (double factor=1) override |
Fetches the reactions from the lagrangian multiplier (l_i) of encapsulated ChConstraints. More... | |
![]() | |
ChLinkMate (const ChLinkMate &other) | |
![]() | |
ChLink (const ChLink &other) | |
int | GetLeftDOF () |
Get the number of free degrees of freedom left by this link, between two bodies. | |
virtual int | GetNumCoords () override |
Get the number of scalar variables affected by constraints in this link. | |
ChBodyFrame * | GetBody1 () |
Get the constrained body '1', the 'slave' body. | |
ChBodyFrame * | GetBody2 () |
Get the constrained body '2', the 'master' body. | |
virtual ChCoordsys | GetLinkAbsoluteCoords () override |
Get the link coordinate system in absolute reference. More... | |
virtual ChVector | Get_react_force () override |
Get reaction force, expressed in link coordinate system. | |
virtual ChVector | Get_react_torque () override |
Get reaction torque, expressed in link coordinate system. | |
virtual void | UpdateTime (double mytime) |
Given new time, current body state, update time-dependent quantities in link state, for example motion laws, moving markers, etc. More... | |
virtual void | Update (bool update_assets=true) override |
As above, but with current time. | |
virtual void | UpdatedExternalTime (double prevtime, double time) |
Called from a external package (i.e. a plugin, a CAD app.) to report that time has changed. | |
![]() | |
ChLinkBase (const ChLinkBase &other) | |
bool | IsValid () |
Tells if the link data is currently valid. More... | |
void | SetValid (bool mon) |
Set the status of link validity. | |
bool | IsDisabled () |
Tells if all constraints of this link are currently turned on or off by the user. | |
bool | IsBroken () |
Tells if the link is broken, for excess of pulling/pushing. | |
virtual bool | IsActive () const override |
Return true if the link is currently active and thereofre included into the system solver. More... | |
virtual bool | IsRequiringWaking () |
Tells if this link requires that the connected ChBody objects must be waken if they are sleeping. More... | |
![]() | |
ChPhysicsItem (const ChPhysicsItem &other) | |
ChSystem * | GetSystem () const |
Get the pointer to the parent ChSystem(). | |
virtual void | SetSystem (ChSystem *m_system) |
Set the pointer to the parent ChSystem(). More... | |
void | AddVisualModel (std::shared_ptr< ChVisualModel > model) |
Add an (optional) visualization model. More... | |
std::shared_ptr< ChVisualModel > | GetVisualModel () const |
Access the visualization model (if any). More... | |
void | AddVisualShape (std::shared_ptr< ChVisualShape > shape, const ChFrame<> &frame=ChFrame<>()) |
Add the specified visual shape to the visualization model. More... | |
std::shared_ptr< ChVisualShape > | GetVisualShape (unsigned int i) const |
Access the specified visualization shape in the visualization model (if any). More... | |
void | AddVisualShapeFEA (std::shared_ptr< ChVisualShapeFEA > shapeFEA) |
Add the specified FEA visualization object to the visualization model. More... | |
std::shared_ptr< ChVisualShapeFEA > | GetVisualShapeFEA (unsigned int i) const |
Access the specified FEA visualization object in the visualization model (if any). More... | |
virtual unsigned int | GetNumVisualModelClones () const |
Return the number of clones of the visual model associated with this physics item. More... | |
void | AddCamera (std::shared_ptr< ChCamera > camera) |
Attach a ChCamera to this physical item. More... | |
std::vector< std::shared_ptr< ChCamera > > | GetCameras () const |
Get the set of cameras attached to this physics item. | |
virtual bool | GetCollide () const |
Tell if the object is subject to collision. More... | |
virtual void | SyncCollisionModels () |
If this physical item contains one or more collision models, synchronize their coordinates and bounding boxes to the state of the item. | |
virtual void | AddCollisionModelsToSystem () |
If this physical item contains one or more collision models, add them to the system's collision engine. | |
virtual void | RemoveCollisionModelsFromSystem () |
If this physical item contains one or more collision models, remove them from the system's collision engine. | |
virtual void | GetTotalAABB (ChVector<> &bbmin, ChVector<> &bbmax) |
Get the entire AABB axis-aligned bounding box of the object. More... | |
virtual void | GetCenter (ChVector<> &mcenter) |
Get a symbolic 'center' of the object. More... | |
virtual void | StreamINstate (ChStreamInBinary &mstream) |
Method to deserialize only the state (position, speed) Must be implemented by child classes. | |
virtual void | StreamOUTstate (ChStreamOutBinary &mstream) |
Method to serialize only the state (position, speed) Must be implemented by child classes. | |
virtual void | Setup () |
This might recompute the number of coordinates, DOFs, constraints, in case this might change (ex in ChAssembly), as well as state offsets of contained items (ex in ChMesh) | |
virtual void | SetNoSpeedNoAcceleration () |
Set zero speed (and zero accelerations) in state, without changing the position. More... | |
virtual int | GetDOF () |
Get the number of scalar coordinates (variables), if any, in this item. More... | |
virtual int | GetDOF_w () |
Get the number of scalar coordinates of variables derivatives (usually = DOF, but might be different than DOF, ex. More... | |
unsigned int | GetOffset_x () |
Get offset in the state vector (position part) | |
unsigned int | GetOffset_w () |
Get offset in the state vector (speed part) | |
unsigned int | GetOffset_L () |
Get offset in the lagrangian multipliers. | |
void | SetOffset_x (const unsigned int moff) |
Set offset in the state vector (position part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_w (const unsigned int moff) |
Set offset in the state vector (speed part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_L (const unsigned int moff) |
Set offset in the lagrangian multipliers Note: only the ChSystem::Setup function should use this. | |
virtual void | IntStateGather (const unsigned int off_x, ChState &x, const unsigned int off_v, ChStateDelta &v, double &T) |
From item's state to global state vectors y={x,v} pasting the states at the specified offsets. More... | |
virtual void | IntStateScatter (const unsigned int off_x, const ChState &x, const unsigned int off_v, const ChStateDelta &v, const double T, bool full_update) |
From global state vectors y={x,v} to item's state (and update) fetching the states at the specified offsets. More... | |
virtual void | IntStateGatherAcceleration (const unsigned int off_a, ChStateDelta &a) |
From item's state acceleration to global acceleration vector. More... | |
virtual void | IntStateScatterAcceleration (const unsigned int off_a, const ChStateDelta &a) |
From global acceleration vector to item's state acceleration. More... | |
virtual void | IntStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) |
Computes x_new = x + Dt , using vectors at specified offsets. More... | |
virtual void | IntStateGetIncrement (const unsigned int off_x, const ChState &x_new, const ChState &x, const unsigned int off_v, ChStateDelta &Dv) |
Computes Dt = x_new - x, using vectors at specified offsets. More... | |
virtual void | IntLoadResidual_F (const unsigned int off, ChVectorDynamic<> &R, const double c) |
Takes the F force term, scale and adds to R at given offset: R += c*F. More... | |
virtual void | IntLoadResidual_Mv (const unsigned int off, ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) |
Takes the M*v term, multiplying mass by a vector, scale and adds to R at given offset: R += c*M*w. More... | |
virtual void | VariablesFbReset () |
Sets the 'fb' part (the known term) of the encapsulated ChVariables to zero. | |
virtual void | VariablesFbLoadForces (double factor=1) |
Adds the current forces (applied to item) into the encapsulated ChVariables, in the 'fb' part: qf+=forces*factor. | |
virtual void | VariablesQbLoadSpeed () |
Initialize the 'qb' part of the ChVariables with the current value of speeds. More... | |
virtual void | VariablesFbIncrementMq () |
Adds M*q (masses multiplied current 'qb') to Fb, ex. More... | |
virtual void | VariablesQbSetSpeed (double step=0) |
Fetches the item speed (ex. More... | |
virtual void | VariablesQbIncrementPosition (double step) |
Increment item positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor. More... | |
virtual void | InjectVariables (ChSystemDescriptor &mdescriptor) |
Tell to a system descriptor that there are variables of type ChVariables in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | ConstraintsBiLoad_Qc (double factor=1) |
Adds the current Qc (the vector of C_dtdt=0 -> [Cq]*q_dtdt=Qc ) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsFbLoadForces (double factor=1) |
Adds the current link-forces, if any, (caused by springs, etc.) to the 'fb' vectors of the ChVariables referenced by encapsulated ChConstraints. | |
virtual void | InjectKRMmatrices (ChSystemDescriptor &mdescriptor) |
Tell to a system descriptor that there are items of type ChKblock in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | KRMmatricesLoad (double Kfactor, double Rfactor, double Mfactor) |
Adds the current stiffness K and damping R and mass M matrices in encapsulated ChKblock item(s), if any. More... | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Gets the numerical identifier of the object. | |
void | SetIdentifier (int id) |
Sets the numerical identifier of the object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
const char * | GetName () const |
Gets the name of the object as C Ascii null-terminated string -for reading only! | |
void | SetName (const char myname[]) |
Sets the name of this object, as ascii string. | |
std::string | GetNameString () const |
Gets the name of the object as C Ascii null-terminated string. | |
void | SetNameString (const std::string &myname) |
Sets the name of this object, as std::string. | |
void | MFlagsSetAllOFF (int &mflag) |
void | MFlagsSetAllON (int &mflag) |
void | MFlagSetON (int &mflag, int mask) |
void | MFlagSetOFF (int &mflag, int mask) |
int | MFlagGet (int &mflag, int mask) |
virtual std::string & | ArchiveContainerName () |
Protected Attributes | |
std::shared_ptr< ChFunction > | m_func |
![]() | |
ChFrame | frame1 |
ChFrame | frame2 |
bool | c_x |
bool | c_y |
bool | c_z |
bool | c_rx |
bool | c_ry |
bool | c_rz |
int | ndoc |
number of DOC, degrees of constraint | |
int | ndoc_c |
number of DOC, degrees of constraint (only bilaterals) | |
int | ndoc_d |
number of DOC, degrees of constraint (only unilaterals) | |
ChLinkMask | mask |
ChConstraintVectorX | C |
residuals | |
![]() | |
ChBodyFrame * | Body1 |
first connected body | |
ChBodyFrame * | Body2 |
second connected body | |
ChVector | react_force |
store the xyz reactions, expressed in local coordinate system of link; | |
ChVector | react_torque |
store the torque reactions, expressed in local coordinate system of link; | |
![]() | |
bool | disabled |
all constraints of link disabled because of user needs | |
bool | valid |
link data is valid | |
bool | broken |
link is broken because of excessive pulling/pushing. | |
![]() | |
ChSystem * | system |
parent system | |
std::shared_ptr< ChVisualModelInstance > | vis_model_instance |
instantiated visualization model | |
std::vector< std::shared_ptr< ChCamera > > | cameras |
set of cameras | |
unsigned int | offset_x |
offset in vector of state (position part) | |
unsigned int | offset_w |
offset in vector of state (speed part) | |
unsigned int | offset_L |
offset in vector of lagrangian multipliers | |
![]() | |
double | ChTime |
the time of simulation for the object | |
Additional Inherited Members | |
![]() | |
using | ChConstraintVectorX = Eigen::Matrix< double, Eigen::Dynamic, 1, Eigen::ColMajor, 6, 1 > |
![]() | |
void | SetupLinkMask () |
void | ChangedLinkMask () |
Member Function Documentation
◆ SetMotorFunction()
|
inline |
Set the actuation function of time F(t).
The return value of this function has different meanings in various derived classes and can represent a position, angle, linear speed, angular speed, force, or torque. If controlling a position-level quantity (position or angle), this function must be C0 continuous (ideally C1 continuous to prevent spikes in accelerations). If controlling a velocity-level quantity (linear on angular speed), this function should ideally be C0 continuous to prevent acceleration spikes.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChLinkMotor.h
- /builds/uwsbel/chrono/src/chrono/physics/ChLinkMotor.cpp