Description
Class for assemblies of items, for example ChBody, ChLink, ChMesh, etc.
Note that an assembly can be added to another assembly, to create a tree-like hierarchy. All positions of rigid bodies, FEA nodes, etc. are assumed with respect to the absolute frame.
#include <ChAssembly.h>
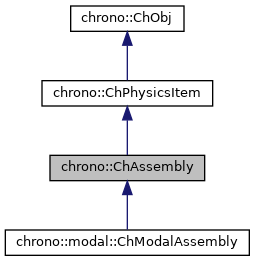
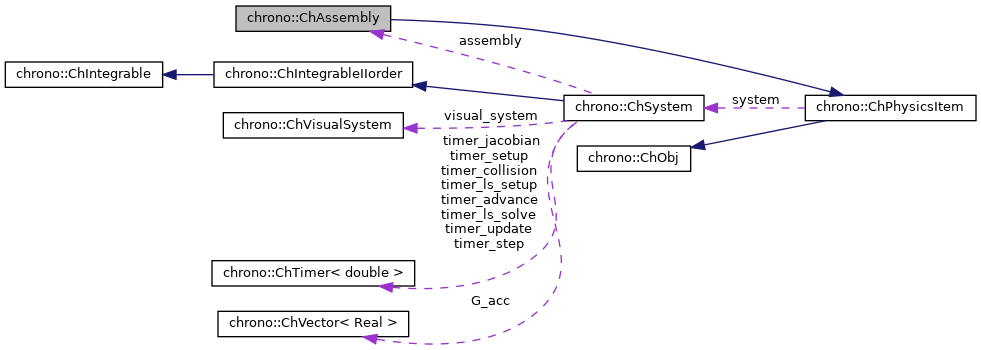
Public Member Functions | |
ChAssembly (const ChAssembly &other) | |
virtual ChAssembly * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
ChAssembly & | operator= (ChAssembly other) |
Assignment operator for ChAssembly. | |
void | Clear () |
Removes all inserted items: bodies, links, etc. | |
void | AddBody (std::shared_ptr< ChBody > body) |
Attach a body to this assembly. | |
void | AddShaft (std::shared_ptr< ChShaft > shaft) |
Attach a shaft to this assembly. | |
void | AddLink (std::shared_ptr< ChLinkBase > link) |
Attach a link to this assembly. | |
void | AddMesh (std::shared_ptr< fea::ChMesh > mesh) |
Attach a mesh to this assembly. | |
void | AddOtherPhysicsItem (std::shared_ptr< ChPhysicsItem > item) |
Attach a ChPhysicsItem object that is not a body, link, or mesh. | |
void | Add (std::shared_ptr< ChPhysicsItem > item) |
Attach an arbitrary ChPhysicsItem (e.g. More... | |
void | AddBatch (std::shared_ptr< ChPhysicsItem > item) |
Items added in this way are added like in the Add() method, but not instantly, they are simply queued in a batch of 'to add' items, that are added automatically at the first Setup() call. More... | |
void | FlushBatch () |
If some items are queued for addition in the assembly, using AddBatch(), this will effectively add them and clean the batch. More... | |
void | RemoveBody (std::shared_ptr< ChBody > body) |
Remove a body from this assembly. | |
void | RemoveShaft (std::shared_ptr< ChShaft > shaft) |
Remove a shaft from this assembly. | |
void | RemoveLink (std::shared_ptr< ChLinkBase > link) |
Remove a link from this assembly. | |
void | RemoveMesh (std::shared_ptr< fea::ChMesh > mesh) |
Remove a mesh from the assembly. | |
void | RemoveOtherPhysicsItem (std::shared_ptr< ChPhysicsItem > item) |
Remove a ChPhysicsItem object that is not a body or a link. | |
void | Remove (std::shared_ptr< ChPhysicsItem > item) |
Remove arbitrary ChPhysicsItem that was added to the assembly. | |
void | RemoveAllBodies () |
Remove all bodies from this assembly. | |
void | RemoveAllShafts () |
Remove all shafts from this assembly. | |
void | RemoveAllLinks () |
Remove all links from this assembly. | |
void | RemoveAllMeshes () |
Remove all meshes from this assembly. | |
void | RemoveAllOtherPhysicsItems () |
Remove all physics items not in the body, link, or mesh lists. | |
const std::vector< std::shared_ptr< ChBody > > & | Get_bodylist () const |
Get the list of bodies. | |
const std::vector< std::shared_ptr< ChShaft > > & | Get_shaftlist () const |
Get the list of shafts. | |
const std::vector< std::shared_ptr< ChLinkBase > > & | Get_linklist () const |
Get the list of links. | |
const std::vector< std::shared_ptr< fea::ChMesh > > & | Get_meshlist () const |
Get the list of meshes. | |
const std::vector< std::shared_ptr< ChPhysicsItem > > & | Get_otherphysicslist () const |
Get the list of physics items that are not in the body or link lists. | |
std::shared_ptr< ChBody > | SearchBody (const char *name) |
Search a body by its name. | |
std::shared_ptr< ChBody > | SearchBodyID (int bodyID) |
Search a body by its ID. | |
std::shared_ptr< ChShaft > | SearchShaft (const char *name) |
Search a shaft by its name. | |
std::shared_ptr< ChLinkBase > | SearchLink (const char *name) |
Search a link by its name. | |
std::shared_ptr< fea::ChMesh > | SearchMesh (const char *name) |
Search a mesh by its name. | |
std::shared_ptr< ChPhysicsItem > | SearchOtherPhysicsItem (const char *name) |
Search from other ChPhysics items (not bodies, links, or meshes) by name. | |
std::shared_ptr< ChMarker > | SearchMarker (const char *name) |
Search a marker by its name. | |
std::shared_ptr< ChMarker > | SearchMarker (int markID) |
Search a marker by its unique ID. | |
std::shared_ptr< ChPhysicsItem > | Search (const char *name) |
Search an item (body, link or other ChPhysics items) by name. | |
int | GetNbodies () const |
Get the number of active bodies (so, excluding those that are sleeping or are fixed to ground). | |
int | GetNbodiesSleeping () const |
Get the number of bodies that are in sleeping mode (excluding fixed bodies). | |
int | GetNbodiesFixed () const |
Get the number of bodies that are fixed to ground. | |
int | GetNbodiesTotal () const |
Get the total number of bodies added to the assembly, including the grounded and sleeping bodies. | |
int | GetNshafts () const |
Get the number of shafts. | |
int | GetNshaftsSleeping () const |
Get the number of shafts that are in sleeping mode (excluding fixed shafts). | |
int | GetNshaftsFixed () const |
Get the number of shafts that are fixed to ground. | |
int | GetNshaftsTotal () const |
Get the total number of shafts added to the assembly, including the grounded and sleeping shafts. | |
int | GetNlinks () const |
Get the number of links. | |
int | GetNmeshes () const |
Get the number of meshes. | |
int | GetNphysicsItems () const |
Get the number of other physics items (other than bodies, links, or meshes). | |
int | GetNcoords () const |
Get the number of coordinates (considering 7 coords for rigid bodies because of the 4 dof of quaternions). | |
int | GetNdof () const |
Get the number of degrees of freedom of the assembly. | |
int | GetNdoc () const |
Get the number of scalar constraints added to the assembly, including constraints on quaternion norms. | |
int | GetNsysvars () const |
Get the number of system variables (coordinates plus the constraint multipliers, in case of quaternions). | |
int | GetNcoords_w () const |
Get the number of coordinates (considering 6 coords for rigid bodies, 3 transl.+3rot.) | |
int | GetNdoc_w () const |
Get the number of scalar constraints added to the assembly. | |
int | GetNdoc_w_C () const |
Get the number of scalar constraints added to the assembly (only bilaterals). | |
int | GetNdoc_w_D () const |
Get the number of scalar constraints added to the assembly (only unilaterals). | |
int | GetNsysvars_w () const |
Get the number of system variables (coordinates plus the constraint multipliers). | |
virtual void | SetSystem (ChSystem *m_system) override |
Set the pointer to the parent ChSystem() and also add to new collision system / remove from old coll.system. | |
virtual void | SyncCollisionModels () override |
If this physical item contains one or more collision models, synchronize their coordinates and bounding boxes to the state of the item. | |
virtual void | Setup () override |
Counts the number of bodies, links, and meshes. More... | |
virtual void | Update (double mytime, bool update_assets=true) override |
Updates all the auxiliary data and children of bodies, forces, links, given their current state. | |
virtual void | Update (bool update_assets=true) override |
Updates all the auxiliary data and children of bodies, forces, links, given their current state. | |
virtual void | SetNoSpeedNoAcceleration () override |
Set zero speed (and zero accelerations) in state, without changing the position. | |
virtual int | GetDOF () override |
Get the number of scalar coordinates (ex. dim of position vector) | |
virtual int | GetDOF_w () override |
Get the number of scalar coordinates of variables derivatives (ex. dim of speed vector) | |
virtual int | GetDOC () override |
Get the number of scalar constraints, if any, in this item. | |
virtual int | GetDOC_c () override |
Get the number of scalar constraints, if any, in this item (only bilateral constr.) | |
virtual int | GetDOC_d () override |
Get the number of scalar constraints, if any, in this item (only unilateral constr.) | |
virtual void | IntStateGather (const unsigned int off_x, ChState &x, const unsigned int off_v, ChStateDelta &v, double &T) override |
From item's state to global state vectors y={x,v} pasting the states at the specified offsets. More... | |
virtual void | IntStateScatter (const unsigned int off_x, const ChState &x, const unsigned int off_v, const ChStateDelta &v, const double T, bool full_update) override |
From global state vectors y={x,v} to item's state (and update) fetching the states at the specified offsets. More... | |
virtual void | IntStateGatherAcceleration (const unsigned int off_a, ChStateDelta &a) override |
From item's state acceleration to global acceleration vector. More... | |
virtual void | IntStateScatterAcceleration (const unsigned int off_a, const ChStateDelta &a) override |
From global acceleration vector to item's state acceleration. More... | |
virtual void | IntStateGatherReactions (const unsigned int off_L, ChVectorDynamic<> &L) override |
From item's reaction forces to global reaction vector. More... | |
virtual void | IntStateScatterReactions (const unsigned int off_L, const ChVectorDynamic<> &L) override |
From global reaction vector to item's reaction forces. More... | |
virtual void | IntStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) override |
Computes x_new = x + Dt , using vectors at specified offsets. More... | |
virtual void | IntStateGetIncrement (const unsigned int off_x, const ChState &x_new, const ChState &x, const unsigned int off_v, ChStateDelta &Dv) override |
Computes Dt = x_new - x, using vectors at specified offsets. More... | |
virtual void | IntLoadResidual_F (const unsigned int off, ChVectorDynamic<> &R, const double c) override |
Takes the F force term, scale and adds to R at given offset: R += c*F. More... | |
virtual void | IntLoadResidual_Mv (const unsigned int off, ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) override |
Takes the M*v term, multiplying mass by a vector, scale and adds to R at given offset: R += c*M*w. More... | |
virtual void | IntLoadResidual_CqL (const unsigned int off_L, ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) override |
Takes the term Cq'*L, scale and adds to R at given offset: R += c*Cq'*L. More... | |
virtual void | IntLoadConstraint_C (const unsigned int off, ChVectorDynamic<> &Qc, const double c, bool do_clamp, double recovery_clamp) override |
Takes the term C, scale and adds to Qc at given offset: Qc += c*C. More... | |
virtual void | IntLoadConstraint_Ct (const unsigned int off, ChVectorDynamic<> &Qc, const double c) override |
Takes the term Ct, scale and adds to Qc at given offset: Qc += c*Ct. More... | |
virtual void | IntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R, const unsigned int off_L, const ChVectorDynamic<> &L, const ChVectorDynamic<> &Qc) override |
Prepare variables and constraints to accommodate a solution: More... | |
virtual void | IntFromDescriptor (const unsigned int off_v, ChStateDelta &v, const unsigned int off_L, ChVectorDynamic<> &L) override |
After a solver solution, fetch values from variables and constraints into vectors: More... | |
virtual void | InjectVariables (ChSystemDescriptor &mdescriptor) override |
Tell to a system descriptor that there are variables of type ChVariables in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | InjectConstraints (ChSystemDescriptor &mdescriptor) override |
Tell to a system descriptor that there are constraints of type ChConstraint in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | ConstraintsLoadJacobians () override |
Adds the current jacobians in encapsulated ChConstraints. | |
virtual void | InjectKRMmatrices (ChSystemDescriptor &mdescriptor) override |
Tell to a system descriptor that there are items of type ChKblock in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | KRMmatricesLoad (double Kfactor, double Rfactor, double Mfactor) override |
Adds the current stiffness K and damping R and mass M matrices in encapsulated ChKblock item(s), if any. More... | |
virtual void | VariablesFbReset () override |
Sets the 'fb' part (the known term) of the encapsulated ChVariables to zero. | |
virtual void | VariablesFbLoadForces (double factor=1) override |
Adds the current forces (applied to item) into the encapsulated ChVariables, in the 'fb' part: qf+=forces*factor. | |
virtual void | VariablesQbLoadSpeed () override |
Initialize the 'qb' part of the ChVariables with the current value of speeds. More... | |
virtual void | VariablesFbIncrementMq () override |
Adds M*q (masses multiplied current 'qb') to Fb, ex. More... | |
virtual void | VariablesQbSetSpeed (double step=0) override |
Fetches the item speed (ex. More... | |
virtual void | VariablesQbIncrementPosition (double step) override |
Increment item positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor. More... | |
virtual void | ConstraintsBiReset () override |
Sets to zero the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_C (double factor=1, double recovery_clamp=0.1, bool do_clamp=false) override |
Adds the current C (constraint violation) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Ct (double factor=1) override |
Adds the current Ct (partial t-derivative, as in C_dt=0-> [Cq]*q_dt=-Ct) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Qc (double factor=1) override |
Adds the current Qc (the vector of C_dtdt=0 -> [Cq]*q_dtdt=Qc ) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsFbLoadForces (double factor=1) override |
Adds the current link-forces, if any, (caused by springs, etc.) to the 'fb' vectors of the ChVariables referenced by encapsulated ChConstraints. | |
virtual void | ConstraintsFetch_react (double factor=1) override |
Fetches the reactions from the lagrangian multiplier (l_i) of encapsulated ChConstraints. More... | |
void | ShowHierarchy (ChStreamOutAscii &m_file, int level=0) const |
Writes the hierarchy of contained bodies, markers, etc. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow deserialization of transient data from archives. | |
![]() | |
ChPhysicsItem (const ChPhysicsItem &other) | |
ChSystem * | GetSystem () const |
Get the pointer to the parent ChSystem(). | |
void | AddVisualModel (std::shared_ptr< ChVisualModel > model) |
Add an (optional) visualization model. More... | |
std::shared_ptr< ChVisualModel > | GetVisualModel () const |
Access the visualization model (if any). More... | |
void | AddVisualShape (std::shared_ptr< ChVisualShape > shape, const ChFrame<> &frame=ChFrame<>()) |
Add the specified visual shape to the visualization model. More... | |
std::shared_ptr< ChVisualShape > | GetVisualShape (unsigned int i) const |
Access the specified visualization shape in the visualization model (if any). More... | |
void | AddVisualShapeFEA (std::shared_ptr< ChVisualShapeFEA > shapeFEA) |
Add the specified FEA visualization object to the visualization model. More... | |
std::shared_ptr< ChVisualShapeFEA > | GetVisualShapeFEA (unsigned int i) const |
Access the specified FEA visualization object in the visualization model (if any). More... | |
virtual ChFrame | GetVisualModelFrame (unsigned int nclone=0) |
Get the reference frame (expressed in and relative to the absolute frame) of the visual model. More... | |
virtual unsigned int | GetNumVisualModelClones () const |
Return the number of clones of the visual model associated with this physics item. More... | |
void | AddCamera (std::shared_ptr< ChCamera > camera) |
Attach a ChCamera to this physical item. More... | |
std::vector< std::shared_ptr< ChCamera > > | GetCameras () const |
Get the set of cameras attached to this physics item. | |
virtual bool | IsActive () const |
Return true if the object is active and included in dynamics. | |
virtual bool | GetCollide () const |
Tell if the object is subject to collision. More... | |
virtual void | AddCollisionModelsToSystem () |
If this physical item contains one or more collision models, add them to the system's collision engine. | |
virtual void | RemoveCollisionModelsFromSystem () |
If this physical item contains one or more collision models, remove them from the system's collision engine. | |
virtual void | GetTotalAABB (ChVector<> &bbmin, ChVector<> &bbmax) |
Get the entire AABB axis-aligned bounding box of the object. More... | |
virtual void | GetCenter (ChVector<> &mcenter) |
Get a symbolic 'center' of the object. More... | |
virtual void | StreamINstate (ChStreamInBinary &mstream) |
Method to deserialize only the state (position, speed) Must be implemented by child classes. | |
virtual void | StreamOUTstate (ChStreamOutBinary &mstream) |
Method to serialize only the state (position, speed) Must be implemented by child classes. | |
unsigned int | GetOffset_x () |
Get offset in the state vector (position part) | |
unsigned int | GetOffset_w () |
Get offset in the state vector (speed part) | |
unsigned int | GetOffset_L () |
Get offset in the lagrangian multipliers. | |
void | SetOffset_x (const unsigned int moff) |
Set offset in the state vector (position part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_w (const unsigned int moff) |
Set offset in the state vector (speed part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_L (const unsigned int moff) |
Set offset in the lagrangian multipliers Note: only the ChSystem::Setup function should use this. | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Gets the numerical identifier of the object. | |
void | SetIdentifier (int id) |
Sets the numerical identifier of the object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
const char * | GetName () const |
Gets the name of the object as C Ascii null-terminated string -for reading only! | |
void | SetName (const char myname[]) |
Sets the name of this object, as ascii string. | |
std::string | GetNameString () const |
Gets the name of the object as C Ascii null-terminated string. | |
void | SetNameString (const std::string &myname) |
Sets the name of this object, as std::string. | |
void | MFlagsSetAllOFF (int &mflag) |
void | MFlagsSetAllON (int &mflag) |
void | MFlagSetON (int &mflag, int mask) |
void | MFlagSetOFF (int &mflag, int mask) |
int | MFlagGet (int &mflag, int mask) |
virtual std::string & | ArchiveContainerName () |
Protected Member Functions | |
virtual void | SetupInitial () override |
Protected Attributes | |
std::vector< std::shared_ptr< ChBody > > | bodylist |
list of rigid bodies | |
std::vector< std::shared_ptr< ChShaft > > | shaftlist |
list of 1-D shafts | |
std::vector< std::shared_ptr< ChLinkBase > > | linklist |
list of joints (links) | |
std::vector< std::shared_ptr< fea::ChMesh > > | meshlist |
list of meshes | |
std::vector< std::shared_ptr< ChPhysicsItem > > | otherphysicslist |
list of other physics objects | |
std::vector< std::shared_ptr< ChPhysicsItem > > | batch_to_insert |
list of items to insert at once | |
int | nbodies |
number of bodies (currently active) | |
int | nbodies_sleep |
number of bodies that are sleeping | |
int | nbodies_fixed |
number of bodies that are fixed | |
int | nshafts |
number of shafts (currently active) | |
int | nshafts_sleep |
number of shafts that are sleeping | |
int | nshafts_fixed |
number of shafts that are fixed | |
int | nlinks |
number of links | |
int | nmeshes |
number of meshes | |
int | nphysicsitems |
number of other physics items | |
int | ncoords |
number of scalar coordinates (including 4th dimension of quaternions) for all active bodies | |
int | ndoc |
number of scalar constraints (including constr. on quaternions) | |
int | nsysvars |
number of variables (coords+lagrangian mult.), i.e. = ncoords+ndoc for all active bodies | |
int | ncoords_w |
number of scalar coordinates when using 3 rot. dof. per body; for all active bodies | |
int | ndoc_w |
number of scalar constraints when using 3 rot. dof. per body; for all active bodies | |
int | nsysvars_w |
number of variables when using 3 rot. dof. per body; i.e. = ncoords_w+ndoc_w | |
int | ndof |
number of degrees of freedom, = ncoords-ndoc = ncoords_w-ndoc_w , | |
int | ndoc_w_C |
number of scalar constraints C, when using 3 rot. dof. per body (excluding unilaterals) | |
int | ndoc_w_D |
number of scalar constraints D, when using 3 rot. dof. per body (only unilaterals) | |
![]() | |
ChSystem * | system |
parent system | |
std::shared_ptr< ChVisualModelInstance > | vis_model_instance |
instantiated visualization model | |
std::vector< std::shared_ptr< ChCamera > > | cameras |
set of cameras | |
unsigned int | offset_x |
offset in vector of state (position part) | |
unsigned int | offset_w |
offset in vector of state (speed part) | |
unsigned int | offset_L |
offset in vector of lagrangian multipliers | |
![]() | |
double | ChTime |
the time of simulation for the object | |
Friends | |
class | ChSystem |
class | ChSystemMulticore |
class | ChSystemDistributed |
void | swap (ChAssembly &first, ChAssembly &second) |
Swap the contents of the two provided ChAssembly objects. More... | |
Member Function Documentation
◆ Add()
void chrono::ChAssembly::Add | ( | std::shared_ptr< ChPhysicsItem > | item | ) |
Attach an arbitrary ChPhysicsItem (e.g.
ChBody, ChParticles, ChLink, etc.) to the assembly. It will take care of adding it to the proper list of bodies, links, meshes, or generic physic item. (i.e. it calls AddBody(), AddShaft(), AddLink(), AddMesh(), or AddOtherPhysicsItem()). Note, you cannot call Add() during an Update (i.e. items like particle generators that are already inserted in the assembly cannot call this) because not thread safe; instead, use AddBatch().
◆ AddBatch()
void chrono::ChAssembly::AddBatch | ( | std::shared_ptr< ChPhysicsItem > | item | ) |
◆ ConstraintsFetch_react()
|
overridevirtual |
Fetches the reactions from the lagrangian multiplier (l_i) of encapsulated ChConstraints.
Mostly used after the solver provided the solution in ChConstraints. Also, should convert the reactions obtained from dynamical simulation, from link space to intuitive react_force and react_torque.
Reimplemented from chrono::ChPhysicsItem.
◆ FlushBatch()
void chrono::ChAssembly::FlushBatch | ( | ) |
If some items are queued for addition in the assembly, using AddBatch(), this will effectively add them and clean the batch.
Called automatically at each Setup().
◆ IntFromDescriptor()
|
overridevirtual |
After a solver solution, fetch values from variables and constraints into vectors:
- Parameters
-
off_v offset for v v vector to where the q 'unknowns' term of the variables will be copied off_L offset for L L vector to where L 'lagrangian ' term of the constraints will be copied
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntLoadConstraint_C()
|
overridevirtual |
Takes the term C, scale and adds to Qc at given offset: Qc += c*C.
- Parameters
-
off offset in Qc residual Qc result: the Qc residual, Qc += c*C c a scaling factor do_clamp apply clamping to c*C? recovery_clamp value for min/max clamping of c*C
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntLoadConstraint_Ct()
|
overridevirtual |
Takes the term Ct, scale and adds to Qc at given offset: Qc += c*Ct.
- Parameters
-
off offset in Qc residual Qc result: the Qc residual, Qc += c*Ct c a scaling factor
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntLoadResidual_CqL()
|
overridevirtual |
Takes the term Cq'*L, scale and adds to R at given offset: R += c*Cq'*L.
- Parameters
-
off_L offset in L multipliers R result: the R residual, R += c*Cq'*L L the L vector c a scaling factor
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntLoadResidual_F()
|
overridevirtual |
Takes the F force term, scale and adds to R at given offset: R += c*F.
- Parameters
-
off offset in R residual R result: the R residual, R += c*F c a scaling factor
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntLoadResidual_Mv()
|
overridevirtual |
Takes the M*v term, multiplying mass by a vector, scale and adds to R at given offset: R += c*M*w.
- Parameters
-
off offset in R residual R result: the R residual, R += c*M*v w the w vector c a scaling factor
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntStateGather()
|
overridevirtual |
From item's state to global state vectors y={x,v} pasting the states at the specified offsets.
- Parameters
-
off_x offset in x state vector x state vector, position part off_v offset in v state vector v state vector, speed part T time
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntStateGatherAcceleration()
|
overridevirtual |
From item's state acceleration to global acceleration vector.
- Parameters
-
off_a offset in a accel. vector a acceleration part of state vector derivative
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntStateGatherReactions()
|
overridevirtual |
From item's reaction forces to global reaction vector.
- Parameters
-
off_L offset in L vector L L vector of reaction forces
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntStateGetIncrement()
|
overridevirtual |
Computes Dt = x_new - x, using vectors at specified offsets.
By default, when DOF = DOF_w, it does just the difference of two state vectors, but in some cases (ex when using quaternions for rotations) it could do more complex stuff, and children classes might overload it.
- Parameters
-
off_x offset in x state vector x_new state vector, final position part x state vector, initial position part off_v offset in v state vector Dv state vector, increment. Here gets the result
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntStateIncrement()
|
overridevirtual |
Computes x_new = x + Dt , using vectors at specified offsets.
By default, when DOF = DOF_w, it does just the sum, but in some cases (ex when using quaternions for rotations) it could do more complex stuff, and children classes might overload it.
- Parameters
-
off_x offset in x state vector x_new state vector, position part, incremented result x state vector, initial position part off_v offset in v state vector Dv state vector, increment
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntStateScatter()
|
overridevirtual |
From global state vectors y={x,v} to item's state (and update) fetching the states at the specified offsets.
- Parameters
-
off_x offset in x state vector x state vector, position part off_v offset in v state vector v state vector, speed part T time full_update perform complete update
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntStateScatterAcceleration()
|
overridevirtual |
From global acceleration vector to item's state acceleration.
- Parameters
-
off_a offset in a accel. vector a acceleration part of state vector derivative
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntStateScatterReactions()
|
overridevirtual |
From global reaction vector to item's reaction forces.
- Parameters
-
off_L offset in L vector L L vector of reaction forces
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ IntToDescriptor()
|
overridevirtual |
Prepare variables and constraints to accommodate a solution:
- Parameters
-
off_v offset for v and R v vector copied into the q 'unknowns' term of the variables R vector copied into the F 'force' term of the variables off_L offset for L and Qc L vector copied into the L 'lagrangian ' term of the constraints Qc vector copied into the Qb 'constraint' term of the constraints
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ KRMmatricesLoad()
|
overridevirtual |
Adds the current stiffness K and damping R and mass M matrices in encapsulated ChKblock item(s), if any.
The K, R, M matrices are added with scaling values Kfactor, Rfactor, Mfactor. NOTE: signs are flipped respect to the ChTimestepper dF/dx terms: K = -dF/dq, R = -dF/dv
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ Setup()
|
overridevirtual |
Counts the number of bodies, links, and meshes.
Computes the offsets of object states in the global state. Assumes that this->offset_x this->offset_w this->offset_L are already set as starting point for offsetting all the contained sub objects.
Reimplemented from chrono::ChPhysicsItem.
Reimplemented in chrono::modal::ChModalAssembly.
◆ ShowHierarchy()
void chrono::ChAssembly::ShowHierarchy | ( | ChStreamOutAscii & | m_file, |
int | level = 0 |
||
) | const |
Writes the hierarchy of contained bodies, markers, etc.
in ASCII readable form, mostly for debugging purposes. Level is the tab spacing at the left.
◆ VariablesFbIncrementMq()
|
overridevirtual |
Adds M*q (masses multiplied current 'qb') to Fb, ex.
if qb is initialized with v_old using VariablesQbLoadSpeed, this method can be used in timestepping schemes that do: M*v_new = M*v_old + forces*dt
Reimplemented from chrono::ChPhysicsItem.
◆ VariablesQbIncrementPosition()
|
overridevirtual |
Increment item positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor.
pos+=qb*step If qb is a speed, this behaves like a single step of 1-st order numerical integration (Eulero integration).
Reimplemented from chrono::ChPhysicsItem.
◆ VariablesQbLoadSpeed()
|
overridevirtual |
Initialize the 'qb' part of the ChVariables with the current value of speeds.
Note: since 'qb' is the unknown, this function seems unnecessary, unless used before VariablesFbIncrementMq()
Reimplemented from chrono::ChPhysicsItem.
◆ VariablesQbSetSpeed()
|
overridevirtual |
Fetches the item speed (ex.
linear and angular vel.in rigid bodies) from the 'qb' part of the ChVariables and sets it as the current item speed. If 'step' is not 0, also should compute the approximate acceleration of the item using backward differences, that is accel=(new_speed-old_speed)/step. Mostly used after the solver provided the solution in ChVariables.
Reimplemented from chrono::ChPhysicsItem.
Friends And Related Function Documentation
◆ swap
|
friend |
Swap the contents of the two provided ChAssembly objects.
Implemented as a friend (as opposed to a member function) so classes with a ChAssembly member can use ADL when implementing their own swap.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/physics/ChAssembly.h
- /builds/uwsbel/chrono/src/chrono/physics/ChAssembly.cpp