Description
Base class for collecting objects inherited from ChConstraint, ChVariables and optionally ChKblock.
These objects can be used to define a sparse representation of the system. This collector is important because it contains all the required information that is sent to a solver (usually a VI/CCP solver, or as a subcase, a linear solver).
The problem is described by a variational inequality VI(Z*x-d,K):
The matrix \(Z\) that represents the problem has this form:
| H -Cq'|*|q|- | f|= |0| | Cq -E | |l| |-b| |c|
with \(Y \ni \mathbb{l} \perp \mathbb{c} \in N_y\)
where \(N_y\) is the normal cone to \(Y\)
By flipping the sign of \(l_i\), the matrix \(Z\) can be symmetric (but in general non positive definite)
| H Cq'|*| q|-| f|=|0| | Cq E | |-l| |-b| |c|
- Linear Problem: \( \forall i \,Y_i = \mathbb{R}, N_{y_{i}} = 0\) (e.g. all bilateral)
- Linear Complementarity Problem (LCP): \( 0\le c \perp l\ge0 \) (i.e. \(Y_i = \mathbb{R}^+\))
- Cone Complementarity Problem (CCP): \(Y \ni \mathbb{l} \perp \mathbb{c} \in N_y\) ( \(Y_i\) are friction cones)
Notes
- most often you call ConvertToMatrixForm() right after a dynamic simulation step, in order to get the system matrices updated to the last timestep;
- when using Anitescu default stepper, the 'f' vector contains forces*timestep = F*dt
- when using Anitescu default stepper, 'q' represents the 'delta speed',
- when using Anitescu default stepper, 'b' represents the dt/phi stabilization term.
- usually, H = M, the mass matrix, but in some cases, ex. when using implicit integrators, objects inherited from ChKblock can be added too, hence H could be H=a*M+b*K+c*R (but not all solvers handle ChKblock!)
All solvers require that the description of the system is passed by means of a ChSystemDescriptor object that holds a list of all the constraints, variables, masses, known terms (ex.forces) under the form of ChVariables, ChConstraints and ChKblock.
In this default implementation, the ChSystemDescriptor simply holds a vector of pointers to ChVariables or to ChConstraints, but more advanced implementations (ex. for supporting parallel GPU solvers) could store constraints and variables structures with other, more efficient data schemes.
#include <ChSystemDescriptor.h>
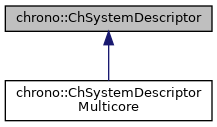
Public Member Functions | |
ChSystemDescriptor () | |
Constructor. | |
virtual | ~ChSystemDescriptor () |
Destructor. | |
std::vector< ChConstraint * > & | GetConstraintsList () |
Access the vector of constraints. | |
std::vector< ChVariables * > & | GetVariablesList () |
Access the vector of variables. | |
std::vector< ChKblock * > & | GetKblocksList () |
Access the vector of stiffness matrix blocks. | |
virtual void | BeginInsertion () |
Begin insertion of items. | |
virtual void | InsertConstraint (ChConstraint *mc) |
Insert reference to a ChConstraint object. | |
virtual void | InsertVariables (ChVariables *mv) |
Insert reference to a ChVariables object. | |
virtual void | InsertKblock (ChKblock *mk) |
Insert reference to a ChKblock object (a piece of matrix) | |
virtual void | EndInsertion () |
End insertion of items. More... | |
virtual int | CountActiveVariables () |
Count & returns the scalar variables in the system (excluding ChVariable objects that have IsActive() as false). More... | |
virtual int | CountActiveConstraints () |
Count & returns the scalar constraints in the system (excluding ChConstraint objects that have IsActive() as false). More... | |
virtual void | UpdateCountsAndOffsets () |
Updates counts of scalar variables and scalar constraints, if you added/removed some item or if you switched some active state, otherwise CountActiveVariables() and CountActiveConstraints() might fail. | |
virtual void | SetMassFactor (const double mc_a) |
Sets the c_a coefficient (default=1) used for scaling the M masses of the vvariables when performing ShurComplementProduct(), SystemProduct(), ConvertToMatrixForm(),. | |
virtual double | GetMassFactor () |
Gets the c_a coefficient (default=1) used for scaling the M masses of the vvariables when performing ShurComplementProduct(), SystemProduct(), ConvertToMatrixForm(),. | |
virtual int | BuildFbVector (ChVectorDynamic<> &Fvector) |
Get a vector with all the 'fb' known terms ('forces'etc.) associated to all variables, ordered into a column vector. More... | |
virtual int | BuildBiVector (ChVectorDynamic<> &Bvector) |
Get a vector with all the 'bi' known terms ('constraint residuals' etc.) associated to all constraints, ordered into a column vector. More... | |
virtual int | BuildDiVector (ChVectorDynamic<> &Dvector) |
Get the d vector = {f; -b} with all the 'fb' and 'bi' known terms, as in Z*y-d (it is the concatenation of BuildFbVector and BuildBiVector) The column vector must be passed as a ChMatrix<> object, which will be automatically reset and resized to the proper length if necessary. More... | |
virtual int | BuildDiagonalVector (ChVectorDynamic<> &Diagonal_vect) |
Get the D diagonal of the Z system matrix, as a single column vector (it includes all the diagonal masses of M, and all the diagonal E (-cfm) terms). More... | |
virtual int | FromVariablesToVector (ChVectorDynamic<> &mvector, bool resize_vector=true) |
Using this function, one may get a vector with all the variables 'q' ordered into a column vector. More... | |
virtual int | FromVectorToVariables (const ChVectorDynamic<> &mvector) |
Using this function, one may go in the opposite direction of the FromVariablesToVector() function, i.e. More... | |
virtual int | FromConstraintsToVector (ChVectorDynamic<> &mvector, bool resize_vector=true) |
Using this function, one may get a vector with all the constraint reactions 'l_i' ordered into a column vector. More... | |
virtual int | FromVectorToConstraints (const ChVectorDynamic<> &mvector) |
Using this function, one may go in the opposite direction of the FromConstraintsToVector() function, i.e. More... | |
virtual int | FromUnknownsToVector (ChVectorDynamic<> &mvector, bool resize_vector=true) |
Using this function, one may get a vector with all the unknowns x={q,l} i.e. More... | |
virtual int | FromVectorToUnknowns (const ChVectorDynamic<> &mvector) |
Using this function, one may go in the opposite direction of the FromUnknownsToVector() function, i.e. More... | |
virtual void | ShurComplementProduct (ChVectorDynamic<> &result, const ChVectorDynamic<> &lvector, std::vector< bool > *enabled=nullptr) |
Performs the product of N, the Shur complement of the KKT matrix, by an 'l' vector. More... | |
virtual void | SystemProduct (ChVectorDynamic<> &result, const ChVectorDynamic<> &x) |
Performs the product of the entire system matrix (KKT matrix), by a vector x ={q,l}. More... | |
virtual void | ConstraintsProject (ChVectorDynamic<> &multipliers) |
Performs projection of constraint multipliers onto allowed set (in case of bilateral constraints it does not affect multipliers, but for frictional constraints, for example, it projects multipliers onto the friction cones) Note! the 'l_i' data in the ChConstraints of the system descriptor are changed by this operation (they get the value of 'multipliers' after the projection), so it may happen that you need to backup them via FromConstraintToVector(). More... | |
virtual void | UnknownsProject (ChVectorDynamic<> &mx) |
As ConstraintsProject(), but instead of passing the l vector, the entire vector of unknowns x={q,-l} is passed. More... | |
virtual void | ComputeFeasabilityViolation (double &resulting_maxviolation, double &resulting_feasability) |
The following (obsolete) function may be called after a solver's 'Solve()' operation has been performed. More... | |
virtual void | ConvertToMatrixForm (ChSparseMatrix *Cq, ChSparseMatrix *H, ChSparseMatrix *E, ChVectorDynamic<> *Fvector, ChVectorDynamic<> *Bvector, ChVectorDynamic<> *Frict, bool only_bilaterals=false, bool skip_contacts_uv=false) |
The following function may be used to create the Jacobian and the mass matrix of the variational problem in matrix form, by assembling all the jacobians of all the constraints/contacts, all the mass matrices, all vectors, as they are currently stored in the sparse data of all ChConstraint and ChVariables contained in this ChSystemDescriptor. More... | |
virtual void | ConvertToMatrixForm (ChSparseMatrix *Z, ChVectorDynamic<> *rhs) |
Create and return the assembled system matrix and RHS vector. More... | |
virtual void | WriteMatrix (const std::string &path, const std::string &prefix) |
Write the current assembled system matrix and right-hand side vector. More... | |
virtual void | WriteMatrixBlocks (const std::string &path, const std::string &prefix) |
Write the current system matrix blocks and right-hand side components. More... | |
virtual void | WriteMatrixSpmv (const std::string &path, const std::string &prefix) |
Write the current assembled system matrix and right-hand side vector. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) |
Method to allow de-serialization of transient data from archives. | |
Protected Attributes | |
std::vector< ChConstraint * > | vconstraints |
list of pointers to all the ChConstraint in the current Chrono system | |
std::vector< ChVariables * > | vvariables |
list of pointers to all the ChVariables in the current Chrono system | |
std::vector< ChKblock * > | vstiffness |
list of pointers to all the ChKblock in the current Chrono system | |
double | c_a |
Member Function Documentation
◆ BuildBiVector()
|
virtual |
Get a vector with all the 'bi' known terms ('constraint residuals' etc.) associated to all constraints, ordered into a column vector.
The column vector must be passed as a ChMatrix<> object, which will be automatically reset and resized to the proper length if necessary.
- Parameters
-
Bvector system-level vector 'b'
◆ BuildDiagonalVector()
|
virtual |
Get the D diagonal of the Z system matrix, as a single column vector (it includes all the diagonal masses of M, and all the diagonal E (-cfm) terms).
The Diagonal_vect must already have the size of n. of unknowns, otherwise it will be resized if necessary).
- Parameters
-
Diagonal_vect system-level vector of terms on M and E diagonal
◆ BuildDiVector()
|
virtual |
Get the d vector = {f; -b} with all the 'fb' and 'bi' known terms, as in Z*y-d (it is the concatenation of BuildFbVector and BuildBiVector) The column vector must be passed as a ChMatrix<> object, which will be automatically reset and resized to the proper length if necessary.
- Parameters
-
Dvector system-level vector {f;-b}
◆ BuildFbVector()
|
virtual |
Get a vector with all the 'fb' known terms ('forces'etc.) associated to all variables, ordered into a column vector.
The column vector must be passed as a ChMatrix<> object, which will be automatically reset and resized to the proper length if necessary.
- Parameters
-
Fvector system-level vector 'f'
◆ ComputeFeasabilityViolation()
|
virtual |
The following (obsolete) function may be called after a solver's 'Solve()' operation has been performed.
This gives an estimate of 'how good' the solver had been in finding the proper solution. Resulting estimates are passed as references in member arguments.
- Parameters
-
resulting_maxviolation gets the max constraint violation (either bi- and unilateral.) resulting_feasability gets the max feasability as max |l*c| , for unilateral only
◆ ConstraintsProject()
|
virtual |
Performs projection of constraint multipliers onto allowed set (in case of bilateral constraints it does not affect multipliers, but for frictional constraints, for example, it projects multipliers onto the friction cones) Note! the 'l_i' data in the ChConstraints of the system descriptor are changed by this operation (they get the value of 'multipliers' after the projection), so it may happen that you need to backup them via FromConstraintToVector().
- Parameters
-
multipliers system-level vector of 'l_i' multipliers to be projected
◆ ConvertToMatrixForm() [1/2]
|
virtual |
The following function may be used to create the Jacobian and the mass matrix of the variational problem in matrix form, by assembling all the jacobians of all the constraints/contacts, all the mass matrices, all vectors, as they are currently stored in the sparse data of all ChConstraint and ChVariables contained in this ChSystemDescriptor.
This can be useful for debugging, data dumping, and similar purposes (most solvers avoid using these matrices, for performance), for example you will load these matrices in Matlab. Optionally, tangential (u,v) contact jacobians may be skipped, or only bilaterals can be considered The matrices and vectors are automatically resized if needed.
- Parameters
-
Cq fill this system jacobian matrix, if not null H fill this system H (mass+stiffness+damp) matrix, if not null E fill this system 'compliance' matrix , if not null Fvector fill this vector as the known term 'f', if not null Bvector fill this vector as the known term 'b', if not null Frict fill as a vector with friction coefficients (=-1 for tangent comp.; =-2 for bilaterals), if not null only_bilaterals skip unilateral constraints skip_contacts_uv skip the tangential reaction constraints
◆ ConvertToMatrixForm() [2/2]
|
virtual |
Create and return the assembled system matrix and RHS vector.
- Parameters
-
[out] Z assembled system matrix [out] rhs assembled RHS vector
◆ CountActiveConstraints()
|
virtual |
Count & returns the scalar constraints in the system (excluding ChConstraint objects that have IsActive() as false).
Note: this function also updates the offsets of all constraints in 'l' global vector (see GetOffset() in ChConstraint).
◆ CountActiveVariables()
|
virtual |
Count & returns the scalar variables in the system (excluding ChVariable objects that have IsActive() as false).
Note: the number of scalar variables is not necessarily the number of inserted ChVariable objects, some could be inactive. Note: this function also updates the offsets of all variables in 'q' global vector (see GetOffset() in ChVariables).
◆ EndInsertion()
|
inlinevirtual |
End insertion of items.
A derived class should always call UpdateCountsAndOffsets.
◆ FromConstraintsToVector()
|
virtual |
Using this function, one may get a vector with all the constraint reactions 'l_i' ordered into a column vector.
The column vector must be passed as a ChMatrix<> object, which will be automatically reset and resized to the proper length if necessary (but uf you are sure that the vector has already the proper size, you can optimize the performance a bit by setting resize_vector as false). Optionally, you can pass an 'enabled' vector of bools, that must have the same length of the l_i reactions vector; constraints with enabled=false are not handled.
- Returns
- the number of scalar constr.multipliers (i.e. the rows of the column vector).
- Parameters
-
mvector system-level vector 'l_i' resize_vector if true, resize vector as necessary
◆ FromUnknownsToVector()
|
virtual |
Using this function, one may get a vector with all the unknowns x={q,l} i.e.
q variables & l_i constr. ordered into a column vector. The column vector must be passed as a ChMatrix<> object, which will be automatically reset and resized to the proper length if necessary (but if you are sure that the vector has already the proper size, you can optimize the performance a bit by setting resize_vector as false).
- Returns
- the number of scalar unknowns
- Parameters
-
mvector system-level vector x={q,l} resize_vector if true, resize vector as necessary
◆ FromVariablesToVector()
|
virtual |
Using this function, one may get a vector with all the variables 'q' ordered into a column vector.
The column vector must be passed as a ChMatrix<> object, which will be automatically reset and resized to the proper length if necessary (but if you are sure that the vector has already the proper size, you can optimize the performance a bit by setting resize_vector as false).
- Returns
- the number of scalar variables (i.e. the rows of the column vector).
- Parameters
-
mvector system-level vector 'q' resize_vector if true, resize vector as necessary
◆ FromVectorToConstraints()
|
virtual |
Using this function, one may go in the opposite direction of the FromConstraintsToVector() function, i.e.
one gives a vector with all the constr.reactions 'l_i' ordered into a column vector, and the constraint objects are updated according to these values. Optionally, you can pass an 'enabled' vector of bools, that must have the same length of the l_i reactions vector; constraints with enabled=false are not handled. NOTE!!! differently from FromConstraintsToVector(), which always works, this function will fail if mvector does not match the amount and ordering of the variable objects!!! (it is up to the user to check this!) btw: most often, this is called after FromConstraintsToVector() to do a kind of 'undo', for example.
- Returns
- the number of scalar constraint multipliers (i.e. the rows of the column vector).
- Parameters
-
mvector system-level vector 'l_i'
◆ FromVectorToUnknowns()
|
virtual |
Using this function, one may go in the opposite direction of the FromUnknownsToVector() function, i.e.
one gives a vector with all the unknowns x={q,l} ordered into a column vector, and the variables q and constr.multipliers l objects are updated according to these values. NOTE!!! differently from FromUnknownsToVector(), which always works, this function will fail if mvector does not match the amount and ordering of the variable and constraint objects!!! (it is up to the user to check this!)
- Parameters
-
mvector system-level vector x={q,l}
◆ FromVectorToVariables()
|
virtual |
Using this function, one may go in the opposite direction of the FromVariablesToVector() function, i.e.
one gives a vector with all the variables 'q' ordered into a column vector, and the variables objects are updated according to these values. NOTE!!! differently from FromVariablesToVector(), which always works, this function will fail if mvector does not match the amount and ordering of the variable objects!!! (it is up to the user to check this!) btw: most often, this is called after FromVariablesToVector() to do a kind of 'undo', for example.
- Returns
- the number of scalar variables (i.e. the rows of the column vector).
- Parameters
-
mvector system-level vector 'q'
◆ ShurComplementProduct()
|
virtual |
Performs the product of N, the Shur complement of the KKT matrix, by an 'l' vector.
result = [N]*l = [ [Cq][M^(-1)][Cq'] - [E] ] * l
where [Cq] are the jacobians, [M] is the mass matrix, [E] is the matrix of the optional cfm 'constraint force mixing' terms for compliant constraints. The N matrix is not built explicitly, to exploit sparsity, it is described by the inserted constraints and inserted variables. Optionally, you can pass an 'enabled' vector of bools, that must have the same length of the l_i reactions vector; constraints with enabled=false are not handled. NOTE! the 'q' data in the ChVariables of the system descriptor is changed by this operation, so it may happen that you need to backup them via FromVariablesToVector() NOTE! currently this function does NOT support the cases that use also ChKblock objects, because it would need to invert the global M+K, that is not diagonal, for doing = [N]*l = [ [Cq][(M+K)^(-1)][Cq'] - [E] ] * l
- Parameters
-
result result of N * l_i lvector vector to be multiplied enabled optional: vector of "enabled" flags, one per scalar constraint.
◆ SystemProduct()
|
virtual |
Performs the product of the entire system matrix (KKT matrix), by a vector x ={q,l}.
Note that the 'q' data in the ChVariables of the system descriptor is changed by this operation, so thay may need to be backed up via FromVariablesToVector()
- Parameters
-
result result vector (multiplication of system matrix by x) x vector to be multiplied
◆ UnknownsProject()
|
virtual |
As ConstraintsProject(), but instead of passing the l vector, the entire vector of unknowns x={q,-l} is passed.
Note! the 'l_i' data in the ChConstraints of the system descriptor are changed by this operation (they get the value of 'multipliers' after the projection), so it may happen that you need to backup them via FromConstraintToVector().
- Parameters
-
mx system-level vector of unknowns x={q,-l} (only the l part is projected)
◆ WriteMatrix()
|
virtual |
Write the current assembled system matrix and right-hand side vector.
The system matrix is formed by calling ConvertToMatrixForm() as used with direct linear solvers. The following files are written in the directory specified by [path]:
- [prefix]_Z.dat the assembled optimization matrix (Matlab sparse format)
- [prefix]_rhs.dat the assmbled RHS
◆ WriteMatrixBlocks()
|
virtual |
Write the current system matrix blocks and right-hand side components.
The system matrix is formed by calling ConvertToMatrixForm() as used with direct linear solvers. The following files are written in the directory specified by [path]:
- [prefix]_H.dat masses and/or stiffness (Matlab sparse format)
- [prefix]_Cq.dat Jacobians (Matlab sparse format)
- [prefix]_E.dat constraint compliance (Matlab sparse format)
- [prefix]_f.dat applied loads
- [prefix]_b.dat constraint rhs
◆ WriteMatrixSpmv()
|
virtual |
Write the current assembled system matrix and right-hand side vector.
The system matrix is formed by multiple calls to SystemProduct() as used with iterative linear solvers. The following files are written in the directory specified by [path]:
- [prefix]_Z.dat the assembled optimization matrix (Matlab sparse format)
- [prefix]_rhs.dat the assmbled RHS
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/solver/ChSystemDescriptor.h
- /builds/uwsbel/chrono/src/chrono/solver/ChSystemDescriptor.cpp