Description
This is a base class for all BINARY INPUT streams, in a way such that the stream is platform independent (see the 'little endian' stuff in 'floating point to persistent data' topics..) Defines some << operators from basic types, converting all them into calls to the Output() function.
At least some inherited class should be used by the user in order to get some practical effect (file saving, etc.)
#include <ChStream.h>

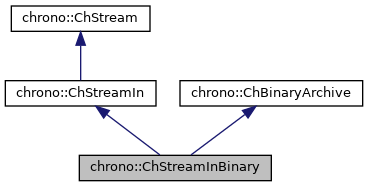
Public Member Functions | |
template<class T > | |
ChStreamInBinary & | operator>> (T &obj) |
Generic >> operator for all classes which implement serialization by means of a method named 'void StreamIN(ChStreamInBinary&)'. | |
ChStreamInBinary & | operator>> (char &Val) |
Specialized operators for basic primitives (numbers like long, double, int. More... | |
ChStreamInBinary & | operator>> (bool &Val) |
ChStreamInBinary & | operator>> (int &Val) |
ChStreamInBinary & | operator>> (unsigned int &Val) |
ChStreamInBinary & | operator>> (double &Val) |
ChStreamInBinary & | operator>> (float &Val) |
ChStreamInBinary & | operator>> (long &Val) |
ChStreamInBinary & | operator>> (unsigned long &Val) |
ChStreamInBinary & | operator>> (unsigned long long &Val) |
ChStreamInBinary & | operator>> (std::string &str) |
ChStreamInBinary & | operator>> (char *str) |
Specialized operator for C strings. | |
template<class T > | |
void | GenericBinaryInput (T &ogg) |
Generic operator for raw binary streaming of generic objects WARNING!!! raw byte streaming! If class 'T' contains double, int, long, etc, these may give problems when loading on another platform which has big-endian ordering, if generated on a little-endian platform... | |
template<class t > | |
t * | AbstractReadCreate (t **mObj) |
Extract an object from the archive, and assignes the pointer to it. More... | |
template<class t > | |
t * | AbstractReadAllCreate (t **mObj) |
Extract an object from the archive, and assigns the pointer to it. More... | |
int | VersionRead () |
Some objects may write class version at the beginning of the streamed data, they can use this function to read class from stream. | |
![]() | |
virtual bool | End_of_stream () const |
Returns true if end of stream reached. More... | |
![]() | |
bool | IsBigEndianMachine () |
Returns true if the machine where the code runs has big endian byte ordering, returns false otherwise. | |
void | Init () |
Reinitialize the vector of pointers to loaded/saved objects. | |
int | PutPointer (void *object) |
Put a pointer in pointer vector, but only if it was not previously inserted. More... | |
Additional Inherited Members | |
![]() | |
enum | eChMode { CHFILE_NORMAL = 0, CHFILE_NOWRITE, CHFILE_SAFEWRITE, CHFILE_OPENLATER } |
Modes for chrono files (the ch-modes) - obsolete -. More... | |
enum | eChStreamError { CHSTREAM_OK = 0, CHSTREAM_EOF, CHSTREAM_FAIL } |
Errors for chrono files (the ch-modes) More... | |
![]() | |
virtual void | Input (char *data, size_t n)=0 |
Inputs a raw chunk of data, from pointer 'data' up to 'n' chars (bytes). More... | |
![]() | |
bool | big_endian_machine |
std::vector< void * > | objects_pointers |
vector of pointers to stored/retrieved objects, to avoid saving duplicates or deadlocks | |
Member Function Documentation
◆ AbstractReadAllCreate()
|
inline |
Extract an object from the archive, and assigns the pointer to it.
This function can be used to load objects whose class is not known in advance (anyway, assuming the class had been registered with Chrono class factory registration). It creates the object of the proper downcasted class, and deserializes it. Note: the object must be saved with ChStreamOutBinary::AbstractWrite() Also, the AbstractWrite()-AbstractReadCreate() mechanism avoids storing/creating multiple times the shared objects. Supports only objects with Chrono RTTI and serializer member StreamIN().
◆ AbstractReadCreate()
|
inline |
Extract an object from the archive, and assignes the pointer to it.
This function can be used to load objects whose class is not known in advance (anyway, assuming the class had been registered with Chrono class factory registration). It creates the object of the proper downcasted class, and deserializes it. Note: the object must be saved with ChStreamOutBinary::AbstractWrite() Also, the AbstractWrite()-AbstractReadCreate() mechanism avoids storing/creating multiple times the shared objects. Supports only objects with Chrono RTTI and serializer member StreamIN().
◆ operator>>()
ChStreamInBinary & chrono::ChStreamInBinary::operator>> | ( | char & | Val | ) |
Specialized operators for basic primitives (numbers like long, double, int.
etc., and booleans) Handle byte ordering issues in little.endian/big.endian representations, for cross platform portability.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/core/ChStream.h
- /builds/uwsbel/chrono/src/chrono/core/ChStream.cpp