Description
Parallel systems using smooth contact (penalty-based) method.
#include <ChSystemParallel.h>
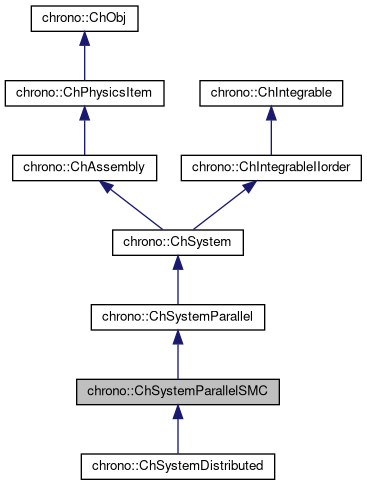
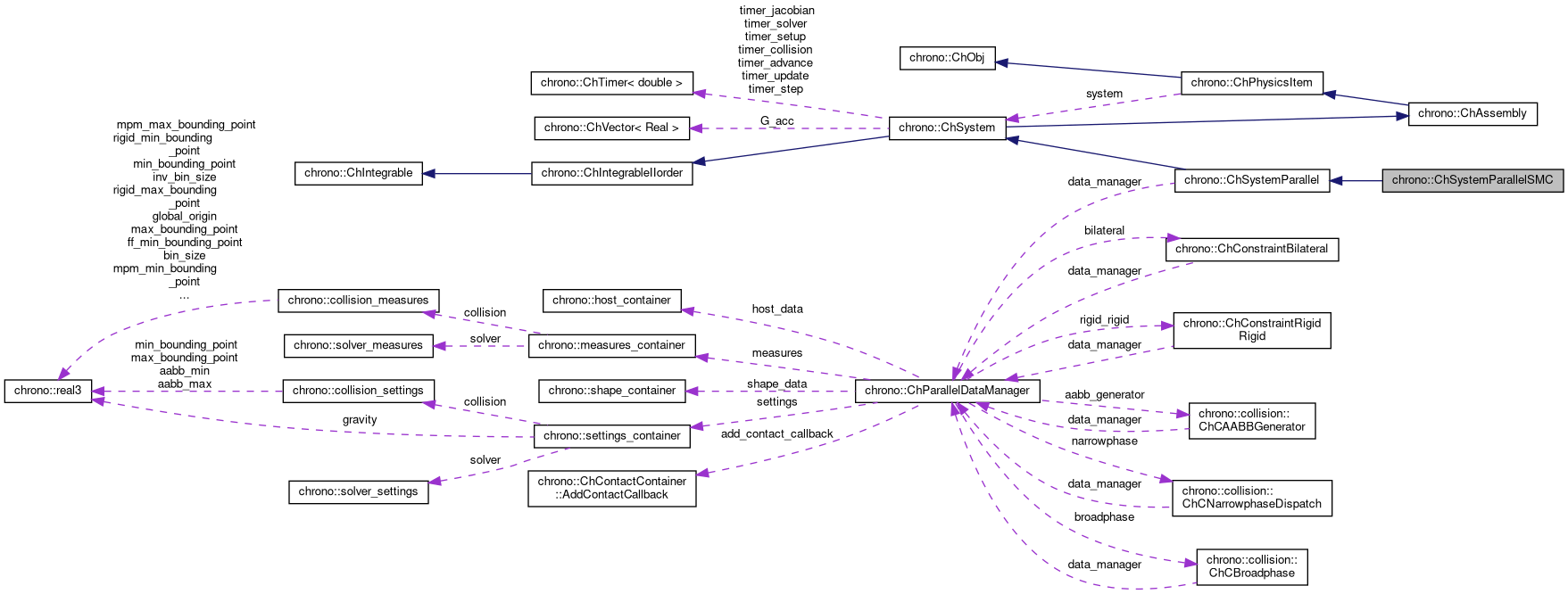
Public Member Functions | |
ChSystemParallelSMC (const ChSystemParallelSMC &other) | |
virtual ChSystemParallelSMC * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
virtual ChMaterialSurfaceNSC::ContactMethod | GetContactMethod () const override |
Return the contact method supported by this system. More... | |
virtual ChBody * | NewBody () override |
Create and return the pointer to a new body. More... | |
virtual ChBodyAuxRef * | NewBodyAuxRef () override |
Create and return the pointer to a new body with auxiliary reference frame. More... | |
virtual void | AddMaterialSurfaceData (std::shared_ptr< ChBody > newbody) override |
virtual void | UpdateMaterialSurfaceData (int index, ChBody *body) override |
virtual void | Setup () override |
Counts the number of bodies and links. More... | |
virtual void | ChangeCollisionSystem (CollisionSystemType type) override |
virtual real3 | GetBodyContactForce (uint body_id) const override |
Get the contact force on the body with specified id. More... | |
virtual real3 | GetBodyContactTorque (uint body_id) const override |
Get the contact torque on the body with specified id. More... | |
virtual void | PrintStepStats () override |
double | GetTimerProcessContact () const |
virtual real3 | GetBodyContactForce (uint body_id) const=0 |
Get the contact force on the body with specified id. More... | |
real3 | GetBodyContactForce (std::shared_ptr< ChBody > body) const |
Get the contact force on the specified body. More... | |
virtual real3 | GetBodyContactTorque (uint body_id) const=0 |
Get the contact torque on the body with specified id. More... | |
real3 | GetBodyContactTorque (std::shared_ptr< ChBody > body) const |
Get the contact torque on the specified body. More... | |
![]() | |
ChSystemParallel (const ChSystemParallel &other) | |
virtual bool | Integrate_Y () override |
Performs a single dynamical simulation step, according to current values of: Y, time, step (and other minor settings) Depending on the integration type, it switches to one of the following: | |
virtual void | AddBody (std::shared_ptr< ChBody > newbody) override |
Attach a body to this assembly. | |
virtual void | AddLink (std::shared_ptr< ChLinkBase > link) override |
Attach a link to this assembly. | |
virtual void | AddMesh (std::shared_ptr< fea::ChMesh > mesh) override |
Attach a mesh to this assembly. | |
virtual void | AddOtherPhysicsItem (std::shared_ptr< ChPhysicsItem > newitem) override |
Attach a ChPhysicsItem object that is not a body, link, or mesh. | |
void | ClearForceVariables () |
virtual void | Update () |
virtual void | UpdateBilaterals () |
virtual void | UpdateLinks () |
virtual void | UpdateOtherPhysics () |
virtual void | UpdateRigidBodies () |
virtual void | UpdateShafts () |
virtual void | UpdateMotorLinks () |
virtual void | Update3DOFBodies () |
void | RecomputeThreads () |
void | SetMaterialCompositionStrategy (std::unique_ptr< ChMaterialCompositionStrategy< real >> &&strategy) |
Change the default composition laws for contact surface materials (coefficient of friction, cohesion, compliance, etc.). | |
unsigned int | GetNumBodies () |
unsigned int | GetNumShafts () |
unsigned int | GetNumContacts () |
unsigned int | GetNumBilaterals () |
virtual double | GetTimerStep () const override |
Return the time (in seconds) spent for computing the time step. | |
virtual double | GetTimerAdvance () const override |
Return the time (in seconds) for time integration, within the time step. | |
virtual double | GetTimerSolver () const override |
Return the time (in seconds) for the solver, within the time step. More... | |
virtual double | GetTimerSetup () const override |
Return the time (in seconds) for the solver Setup phase, within the time step. | |
virtual double | GetTimerJacobian () const override |
Return the time (in seconds) for calculating/loading Jacobian information, within the time step. | |
virtual double | GetTimerCollision () const override |
Return the time (in seconds) for runnning the collision detection step, within the time step. | |
virtual double | GetTimerUpdate () const override |
Return the time (in seconds) for updating auxiliary data, within the time step. | |
void | CalculateBodyAABB () |
Calculate current body AABBs. | |
virtual void | CalculateContactForces () |
Calculate cummulative contact forces for all bodies in the system. More... | |
real3 | GetBodyContactForce (std::shared_ptr< ChBody > body) const |
Get the contact force on the specified body. More... | |
real3 | GetBodyContactTorque (std::shared_ptr< ChBody > body) const |
Get the contact torque on the specified body. More... | |
settings_container * | GetSettings () |
void | SetLoggingLevel (LoggingLevel level, bool state=true) |
double | CalculateConstraintViolation (std::vector< double > &cvec) |
Calculate the (linearized) bilateral constraint violations. More... | |
![]() | |
ChSystem () | |
Create a physical system. | |
ChSystem (const ChSystem &other) | |
Copy constructor. | |
virtual | ~ChSystem () |
Destructor. | |
void | SetStep (double m_step) |
Sets the time step used for integration (dynamical simulation). More... | |
double | GetStep () const |
Gets the current time step used for the integration (dynamical simulation). | |
void | SetEndTime (double m_end_time) |
Sets the end of simulation. | |
double | GetEndTime () const |
Gets the end of the simulation. | |
void | SetStepMin (double m_step_min) |
Sets the lower limit for time step (only needed if using integration methods which support time step adaption). | |
double | GetStepMin () const |
Gets the lower limit for time step. | |
void | SetStepMax (double m_step_max) |
Sets the upper limit for time step (only needed if using integration methods which support time step adaption). | |
double | GetStepMax () const |
Gets the upper limit for time step. | |
void | SetTimestepperType (ChTimestepper::Type type) |
Set the method for time integration (time stepper type). More... | |
ChTimestepper::Type | GetTimestepperType () const |
Get the current method for time integration (time stepper type). | |
void | SetTimestepper (std::shared_ptr< ChTimestepper > mstepper) |
Set the timestepper object to be used for time integration. | |
std::shared_ptr< ChTimestepper > | GetTimestepper () const |
Get the timestepper currently used for time integration. | |
void | SetMaxiter (int m_maxiter) |
Sets outer iteration limit for assembly constraints. More... | |
int | GetMaxiter () const |
Gets iteration limit for assembly constraints. | |
void | SetMaterialCompositionStrategy (std::unique_ptr< ChMaterialCompositionStrategy< float >> &&strategy) |
Change the default composition laws for contact surface materials (coefficient of friction, cohesion, compliance, etc.) | |
const ChMaterialCompositionStrategy< float > & | GetMaterialCompositionStrategy () const |
Accessor for the current composition laws for contact surface material. | |
void | SetMinBounceSpeed (double mval) |
For elastic collisions, with objects that have nonzero restitution coefficient: objects will rebounce only if their relative colliding speed is above this threshold. More... | |
double | GetMinBounceSpeed () const |
Objects will rebounce only if their relative colliding speed is above this threshold. | |
void | SetMaxPenetrationRecoverySpeed (double mval) |
For the default stepper, you can limit the speed of exiting from penetration situations. More... | |
double | GetMaxPenetrationRecoverySpeed () const |
Get the limit on the speed for exiting from penetration situations (for Anitescu stepper) | |
virtual void | SetSolver (std::shared_ptr< ChSolver > newsolver) |
Attach a solver (derived from ChSolver) for use by this system. | |
virtual std::shared_ptr< ChSolver > | GetSolver () |
Access the solver currently associated with this system. | |
virtual void | SetSolverType (ChSolver::Type type) |
Choose the solver type, to be used for the simultaneous solution of the constraints in dynamical simulations (as well as in kinematics, statics, etc.) More... | |
ChSolver::Type | GetSolverType () const |
Gets the current solver type. | |
void | SetSolverMaxIterations (int max_iters) |
Set the maximum number of iterations, if using an iterative solver. More... | |
int | GetSolverMaxIterations () const |
Get the current maximum number of iterations, if using an iterative solver. More... | |
void | SetSolverTolerance (double tolerance) |
Set the solver tolerance threshold (used with iterative solvers only). More... | |
double | GetSolverTolerance () const |
Get the current tolerance value (used with iterative solvers only). | |
void | SetSolverForceTolerance (double tolerance) |
Set a solver tolerance threshold at force level (default: not specified). More... | |
double | GetSolverForceTolerance () const |
Get the current value of the force-level tolerance (used with iterative solvers only). | |
void | SetSystemDescriptor (std::shared_ptr< ChSystemDescriptor > newdescriptor) |
Instead of using the default 'system descriptor', you can create your own custom descriptor (inherited from ChSystemDescriptor) and plug it into the system using this function. | |
std::shared_ptr< ChSystemDescriptor > | GetSystemDescriptor () |
Access directly the 'system descriptor'. | |
void | Set_G_acc (const ChVector<> &m_acc) |
Sets the G (gravity) acceleration vector, affecting all the bodies in the system. | |
const ChVector & | Get_G_acc () const |
Gets the G (gravity) acceleration vector affecting all the bodies in the system. | |
void | Clear () |
Removes all bodies/marker/forces/links/contacts, also resets timers and events. | |
virtual void | SetContactContainer (std::shared_ptr< ChContactContainer > container) |
Replace the contact container. | |
std::shared_ptr< ChContactContainer > | GetContactContainer () |
Get the contact container. | |
void | Reference_LM_byID () |
Given inserted markers and links, restores the pointers of links to markers given the information about the marker IDs. More... | |
int | GetNcontacts () |
Gets the number of contacts. | |
double | GetTimerCollisionBroad () const |
Return the time (in seconds) for broadphase collision detection, within the time step. | |
double | GetTimerCollisionNarrow () const |
Return the time (in seconds) for narrowphase collision detection, within the time step. | |
void | ResetTimers () |
Resets the timers. | |
virtual void | Update (bool update_assets=true) override |
Updates all the auxiliary data and children of bodies, forces, links, given their current state. | |
void | ForceUpdate () |
In normal usage, no system update is necessary at the beginning of a new dynamics step (since an update is performed at the end of a step). More... | |
virtual void | IntStateGather (const unsigned int off_x, ChState &x, const unsigned int off_v, ChStateDelta &v, double &T) override |
From item's state to global state vectors y={x,v} pasting the states at the specified offsets. More... | |
virtual void | IntStateScatter (const unsigned int off_x, const ChState &x, const unsigned int off_v, const ChStateDelta &v, const double T) override |
From global state vectors y={x,v} to item's state (and update) fetching the states at the specified offsets. More... | |
virtual void | IntStateGatherAcceleration (const unsigned int off_a, ChStateDelta &a) override |
From item's state acceleration to global acceleration vector. More... | |
virtual void | IntStateScatterAcceleration (const unsigned int off_a, const ChStateDelta &a) override |
From global acceleration vector to item's state acceleration. More... | |
virtual void | IntStateGatherReactions (const unsigned int off_L, ChVectorDynamic<> &L) override |
From item's reaction forces to global reaction vector. More... | |
virtual void | IntStateScatterReactions (const unsigned int off_L, const ChVectorDynamic<> &L) override |
From global reaction vector to item's reaction forces. More... | |
virtual void | IntStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) override |
Computes x_new = x + Dt , using vectors at specified offsets. More... | |
virtual void | IntLoadResidual_F (const unsigned int off, ChVectorDynamic<> &R, const double c) override |
Takes the F force term, scale and adds to R at given offset: R += c*F. More... | |
virtual void | IntLoadResidual_Mv (const unsigned int off, ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) override |
Takes the M*v term, multiplying mass by a vector, scale and adds to R at given offset: R += c*M*w. More... | |
virtual void | IntLoadResidual_CqL (const unsigned int off_L, ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) override |
Takes the term Cq'*L, scale and adds to R at given offset: R += c*Cq'*L. More... | |
virtual void | IntLoadConstraint_C (const unsigned int off, ChVectorDynamic<> &Qc, const double c, bool do_clamp, double recovery_clamp) override |
Takes the term C, scale and adds to Qc at given offset: Qc += c*C. More... | |
virtual void | IntLoadConstraint_Ct (const unsigned int off, ChVectorDynamic<> &Qc, const double c) override |
Takes the term Ct, scale and adds to Qc at given offset: Qc += c*Ct. More... | |
virtual void | IntToDescriptor (const unsigned int off_v, const ChStateDelta &v, const ChVectorDynamic<> &R, const unsigned int off_L, const ChVectorDynamic<> &L, const ChVectorDynamic<> &Qc) override |
Prepare variables and constraints to accommodate a solution: More... | |
virtual void | IntFromDescriptor (const unsigned int off_v, ChStateDelta &v, const unsigned int off_L, ChVectorDynamic<> &L) override |
After a solver solution, fetch values from variables and constraints into vectors: More... | |
virtual void | InjectVariables (ChSystemDescriptor &mdescriptor) override |
Tell to a system descriptor that there are variables of type ChVariables in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | InjectConstraints (ChSystemDescriptor &mdescriptor) override |
Tell to a system descriptor that there are constraints of type ChConstraint in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | ConstraintsLoadJacobians () override |
Adds the current jacobians in encapsulated ChConstraints. | |
virtual void | InjectKRMmatrices (ChSystemDescriptor &mdescriptor) override |
Tell to a system descriptor that there are items of type ChKblock in this object (for further passing it to a solver) Basically does nothing, but maybe that inherited classes may specialize this. | |
virtual void | KRMmatricesLoad (double Kfactor, double Rfactor, double Mfactor) override |
Adds the current stiffness K and damping R and mass M matrices in encapsulated ChKblock item(s), if any. More... | |
virtual void | VariablesFbReset () override |
Sets the 'fb' part (the known term) of the encapsulated ChVariables to zero. | |
virtual void | VariablesFbLoadForces (double factor=1) override |
Adds the current forces (applied to item) into the encapsulated ChVariables, in the 'fb' part: qf+=forces*factor. | |
virtual void | VariablesQbLoadSpeed () override |
Initialize the 'qb' part of the ChVariables with the current value of speeds. More... | |
virtual void | VariablesFbIncrementMq () override |
Adds M*q (masses multiplied current 'qb') to Fb, ex. More... | |
virtual void | VariablesQbSetSpeed (double step=0) override |
Fetches the item speed (ex. More... | |
virtual void | VariablesQbIncrementPosition (double step) override |
Increment item positions by the 'qb' part of the ChVariables, multiplied by a 'step' factor. More... | |
virtual void | ConstraintsBiReset () override |
Sets to zero the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_C (double factor=1, double recovery_clamp=0.1, bool do_clamp=false) override |
Adds the current C (constraint violation) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Ct (double factor=1) override |
Adds the current Ct (partial t-derivative, as in C_dt=0-> [Cq]*q_dt=-Ct) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsBiLoad_Qc (double factor=1) override |
Adds the current Qc (the vector of C_dtdt=0 -> [Cq]*q_dtdt=Qc ) to the known term (b_i) of encapsulated ChConstraints. | |
virtual void | ConstraintsFbLoadForces (double factor=1) override |
Adds the current link-forces, if any, (caused by springs, etc.) to the 'fb' vectors of the ChVariables referenced by encapsulated ChConstraints. | |
virtual void | ConstraintsFetch_react (double factor=1) override |
Fetches the reactions from the lagrangian multiplier (l_i) of encapsulated ChConstraints. More... | |
virtual int | GetNcoords_x () override |
Tells the number of position coordinates x in y = {x, v}. | |
virtual int | GetNcoords_v () override |
Tells the number of speed coordinates of v in y = {x, v} and dy/dt={v, a}. | |
virtual int | GetNconstr () override |
Tells the number of lagrangian multipliers (constraints) | |
virtual void | StateGather (ChState &x, ChStateDelta &v, double &T) override |
From system to state y={x,v}. | |
virtual void | StateScatter (const ChState &x, const ChStateDelta &v, const double T) override |
From state Y={x,v} to system. | |
virtual void | StateGatherAcceleration (ChStateDelta &a) override |
From system to state derivative (acceleration), some timesteppers might need last computed accel. | |
virtual void | StateScatterAcceleration (const ChStateDelta &a) override |
From state derivative (acceleration) to system, sometimes might be needed. | |
virtual void | StateGatherReactions (ChVectorDynamic<> &L) override |
From system to reaction forces (last computed) - some timestepper might need this. | |
virtual void | StateScatterReactions (const ChVectorDynamic<> &L) override |
From reaction forces to system, ex. store last computed reactions in ChLink objects for plotting etc. | |
virtual void | StateIncrementX (ChState &x_new, const ChState &x, const ChStateDelta &Dx) override |
Perform x_new = x + dx, for x in Y = {x, dx/dt}. More... | |
virtual bool | StateSolveCorrection (ChStateDelta &Dv, ChVectorDynamic<> &L, const ChVectorDynamic<> &R, const ChVectorDynamic<> &Qc, const double c_a, const double c_v, const double c_x, const ChState &x, const ChStateDelta &v, const double T, bool force_state_scatter=true, bool force_setup=true) override |
Assuming a DAE of the form. More... | |
virtual void | LoadResidual_F (ChVectorDynamic<> &R, const double c) override |
Increment a vector R with the term c*F: R += c*F. More... | |
virtual void | LoadResidual_Mv (ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) override |
Increment a vector R with a term that has M multiplied a given vector w: R += c*M*w. More... | |
virtual void | LoadResidual_CqL (ChVectorDynamic<> &R, const ChVectorDynamic<> &L, const double c) override |
Increment a vectorR with the term Cq'*L: R += c*Cq'*L. More... | |
virtual void | LoadConstraint_C (ChVectorDynamic<> &Qc, const double c, const bool do_clamp=false, const double mclam=1e30) override |
Increment a vector Qc with the term C: Qc += c*C. More... | |
virtual void | LoadConstraint_Ct (ChVectorDynamic<> &Qc, const double c) override |
Increment a vector Qc with the term Ct = partial derivative dC/dt: Qc += c*Ct. More... | |
virtual void | CustomEndOfStep () |
Executes custom processing at the end of step. More... | |
void | SynchronizeLastCollPositions () |
All bodies with collision detection data are requested to store the current position as "last position collision-checked". | |
double | ComputeCollisions () |
Perform the collision detection. More... | |
void | RegisterCustomCollisionCallback (CustomCollisionCallback *mcallb) |
Specify a callback object to be invoked at each collision detection step. More... | |
void | SetCollisionSystem (std::shared_ptr< collision::ChCollisionSystem > newcollsystem) |
For higher performance (ex. More... | |
std::shared_ptr< collision::ChCollisionSystem > | GetCollisionSystem () const |
Access the collision system, the engine which computes the contact points (usually you don't need to access it, since it is automatically handled by the client ChSystem object). | |
void | SetUseSleeping (bool ms) |
Turn on this feature to let the system put to sleep the bodies whose motion has almost come to a rest. More... | |
bool | GetUseSleeping () const |
Tell if the system will put to sleep the bodies whose motion has almost come to a rest. | |
int | DoStepDynamics (double m_step) |
Advances the dynamical simulation for a single step, of length m_step. More... | |
bool | DoFrameDynamics (double m_endtime) |
Performs integration until the m_endtime is exactly reached, but current time step may be automatically "retouched" to meet exactly the m_endtime after n steps. More... | |
bool | DoEntireDynamics () |
Given the current state, the sw simulates the dynamical behavior of the system, until the end time is reached, repeating many steps (maybe the step size will be automatically changed if the integrator method supports step size adaption). | |
bool | DoEntireUniformDynamics (double frame_step) |
Like "DoEntireDynamics", but results are provided at uniform steps "frame_step", using the DoFrameDynamics() many times. | |
size_t | GetStepcount () const |
Return the total number of time steps taken so far. | |
void | ResetStepcount () |
Reset to 0 the total number of time steps. | |
int | GetSolverCallsCount () const |
Return the number of calls to the solver's Solve() function. More... | |
int | GetSolverSetupCount () const |
Return the number of calls to the solver's Setup() function. More... | |
void | SetDumpSolverMatrices (bool md) |
Set this to "true" to enable automatic saving of solver matrices at each time step, for debugging purposes. More... | |
bool | GetDumpSolverMatrices () const |
void | DumpSystemMatrices (bool save_M, bool save_K, bool save_R, bool save_Cq, const char *path) |
Dump the current M mass matrix, K damping matrix, R damping matrix, Cq constraint jacobian matrix (at the current configuration). More... | |
void | GetMassMatrix (ChSparseMatrix *M) |
Compute the system-level mass matrix. More... | |
void | GetStiffnessMatrix (ChSparseMatrix *K) |
Compute the system-level stiffness matrix, i.e. More... | |
void | GetDampingMatrix (ChSparseMatrix *R) |
Compute the system-level damping matrix, i.e. More... | |
void | GetConstraintJacobianMatrix (ChSparseMatrix *Cq) |
Compute the system-level constraint jacobian matrix, i.e. More... | |
bool | DoStepKinematics (double m_step) |
Advances the kinematic simulation for a single step, of length m_step. More... | |
bool | DoFrameKinematics (double m_endtime) |
Performs kinematics until the m_endtime is exactly reached, but current time step may be automatically "retouched" to meet exactly the m_endtime after n steps. | |
bool | DoEntireKinematics () |
Given the current state, this kinematic simulation satisfies all the constraints with the "DoStepKinematics" procedure for each time step, from the current time to the end time. | |
bool | DoAssembly (int action) |
Given the current time and state, attempt to satisfy all constraints, using a Newton-Raphson iteration loop. More... | |
bool | DoFullAssembly () |
Shortcut for full position/velocity/acceleration assembly. | |
bool | DoStaticLinear () |
Solve the position of static equilibrium (and the reactions). More... | |
bool | DoStaticNonlinear (int nsteps=10, bool verbose=false) |
Solve the position of static equilibrium (and the reactions). More... | |
bool | DoStaticNonlinear (std::shared_ptr< ChStaticNonLinearAnalysis > analysis) |
Solve the position of static equilibrium (and the reactions). More... | |
bool | DoStaticRelaxing (int nsteps=10) |
Finds the position of static equilibrium (and the reactions) starting from the current position. More... | |
virtual void | ArchiveOUT (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIN (ChArchiveIn &marchive) override |
Method to allow deserialization of transient data from archives. | |
int | FileProcessChR (ChStreamInBinary &m_file) |
Process a ".chr" binary file containing the full system object hierarchy as exported -for example- by the R3D modeler, with chrono plug-in version, or by using the FileWriteChR() function. | |
int | FileWriteChR (ChStreamOutBinary &m_file) |
Write a ".chr" binary file containing the full system object hierarchy (bodies, forces, links, etc.) (deprecated function - obsolete) | |
![]() | |
ChAssembly (const ChAssembly &other) | |
void | Clear () |
Removes all inserted items: bodies, links, etc. | |
void | Add (std::shared_ptr< ChPhysicsItem > item) |
Attach an arbitrary ChPhysicsItem (e.g. More... | |
void | AddBatch (std::shared_ptr< ChPhysicsItem > item) |
Items added in this way are added like in the Add() method, but not instantly, they are simply queued in a batch of 'to add' items, that are added automatically at the first Setup() call. More... | |
void | FlushBatch () |
If some items are queued for addition in the assembly, using AddBatch(), this will effectively add them and clean the batch. More... | |
virtual void | RemoveBody (std::shared_ptr< ChBody > body) |
Remove a body from this assembly. | |
virtual void | RemoveLink (std::shared_ptr< ChLinkBase > link) |
Remove a link from this assembly. | |
virtual void | RemoveMesh (std::shared_ptr< fea::ChMesh > mesh) |
Remove a mesh from the assembly. | |
virtual void | RemoveOtherPhysicsItem (std::shared_ptr< ChPhysicsItem > item) |
Remove a ChPhysicsItem object that is not a body or a link. | |
void | Remove (std::shared_ptr< ChPhysicsItem > item) |
Remove arbitrary ChPhysicsItem that was added to the assembly. | |
void | RemoveAllBodies () |
Remove all bodies from this assembly. | |
void | RemoveAllLinks () |
Remove all links from this assembly. | |
void | RemoveAllMeshes () |
Remove all meshes from this assembly. | |
void | RemoveAllOtherPhysicsItems () |
Remove all physics items not in the body, link, or mesh lists. | |
const std::vector< std::shared_ptr< ChBody > > & | Get_bodylist () const |
Get the list of bodies. | |
const std::vector< std::shared_ptr< ChLinkBase > > & | Get_linklist () const |
Get the list of links. | |
const std::vector< std::shared_ptr< fea::ChMesh > > & | Get_meshlist () const |
Get the list of meshes. | |
const std::vector< std::shared_ptr< ChPhysicsItem > > & | Get_otherphysicslist () const |
Get the list of physics items that are not in the body or link lists. | |
std::shared_ptr< ChBody > | SearchBody (const char *name) |
Search a body by its name. | |
std::shared_ptr< ChLinkBase > | SearchLink (const char *name) |
Search a link by its name. | |
std::shared_ptr< fea::ChMesh > | SearchMesh (const char *name) |
Search a mesh by its name. | |
std::shared_ptr< ChPhysicsItem > | SearchOtherPhysicsItem (const char *name) |
Search from other ChPhysics items (not bodies, links, or meshes) by name. | |
std::shared_ptr< ChPhysicsItem > | Search (const char *name) |
Search an item (body, link or other ChPhysics items) by name. | |
std::shared_ptr< ChBody > | SearchBodyID (int markID) |
Search a body by its ID. | |
std::shared_ptr< ChMarker > | SearchMarker (const char *name) |
Search a marker by its name. | |
std::shared_ptr< ChMarker > | SearchMarker (int markID) |
Search a marker by its unique ID. | |
int | GetNbodies () const |
Get the number of active bodies (so, excluding those that are sleeping or are fixed to ground). | |
int | GetNbodiesSleeping () const |
Get the number of bodies that are in sleeping mode (excluding fixed bodies). | |
int | GetNbodiesFixed () const |
Get the number of bodies that are fixed to ground. | |
int | GetNbodiesTotal () const |
Get the total number of bodies added to the assembly, including the grounded and sleeping bodies. | |
int | GetNlinks () const |
Get the number of links. | |
int | GetNmeshes () const |
Get the number of meshes. | |
int | GetNphysicsItems () const |
Get the number of other physics items (other than bodies, links, or meshes). | |
int | GetNcoords () const |
Get the number of coordinates (considering 7 coords for rigid bodies because of the 4 dof of quaternions). | |
int | GetNdof () const |
Get the number of degrees of freedom of the assembly. | |
int | GetNdoc () const |
Get the number of scalar constraints added to the assembly, including constraints on quaternion norms. | |
int | GetNsysvars () const |
Get the number of system variables (coordinates plus the constraint multipliers, in case of quaternions). | |
int | GetNcoords_w () const |
Get the number of coordinates (considering 6 coords for rigid bodies, 3 transl.+3rot.) | |
int | GetNdoc_w () const |
Get the number of scalar constraints added to the assembly. | |
int | GetNdoc_w_C () const |
Get the number of scalar constraints added to the assembly (only bilaterals). | |
int | GetNdoc_w_D () const |
Get the number of scalar constraints added to the assembly (only unilaterals). | |
int | GetNsysvars_w () const |
Get the number of system variables (coordinates plus the constraint multipliers). | |
virtual void | SetSystem (ChSystem *m_system) override |
Set the pointer to the parent ChSystem() and also add to new collision system / remove from old coll.system. | |
virtual void | SyncCollisionModels () override |
If this physical item contains one or more collision models, synchronize their coordinates and bounding boxes to the state of the item. | |
virtual void | Update (double mytime, bool update_assets=true) override |
Updates all the auxiliary data and children of bodies, forces, links, given their current state. | |
virtual void | SetNoSpeedNoAcceleration () override |
Set zero speed (and zero accelerations) in state, without changing the position. | |
virtual int | GetDOF () override |
Get the number of scalar coordinates (ex. dim of position vector) | |
virtual int | GetDOF_w () override |
Get the number of scalar coordinates of variables derivatives (ex. dim of speed vector) | |
virtual int | GetDOC () override |
Get the number of scalar constraints, if any, in this item. | |
virtual int | GetDOC_c () override |
Get the number of scalar constraints, if any, in this item (only bilateral constr.) | |
virtual int | GetDOC_d () override |
Get the number of scalar constraints, if any, in this item (only unilateral constr.) | |
void | ShowHierarchy (ChStreamOutAscii &m_file, int level=0) |
Writes the hierarchy of contained bodies, markers, etc. More... | |
![]() | |
ChPhysicsItem (const ChPhysicsItem &other) | |
ChSystem * | GetSystem () const |
Get the pointer to the parent ChSystem() | |
void | AddAsset (std::shared_ptr< ChAsset > masset) |
Add an optional asset (it can be used to define visualization shapes, es ChSphereShape, or textures, or custom attached properties that the user can define by creating his class inherited from ChAsset) | |
std::vector< std::shared_ptr< ChAsset > > & | GetAssets () |
Access to the list of optional assets. | |
std::shared_ptr< ChAsset > | GetAssetN (unsigned int num) |
Access the Nth asset in the list of optional assets. | |
virtual ChFrame | GetAssetsFrame (unsigned int nclone=0) |
Get the master coordinate system for assets that have some geometric meaning. More... | |
virtual unsigned int | GetAssetsFrameNclones () |
Optionally, a ChPhysicsItem can return multiple asset coordinate systems; this can be helpful if, for example, when a ChPhysicsItem contains 'clones' with the same assets (ex. More... | |
virtual bool | GetCollide () const |
Tell if the object is subject to collision. More... | |
virtual void | AddCollisionModelsToSystem () |
If this physical item contains one or more collision models, add them to the system's collision engine. | |
virtual void | RemoveCollisionModelsFromSystem () |
If this physical item contains one or more collision models, remove them from the system's collision engine. | |
virtual void | GetTotalAABB (ChVector<> &bbmin, ChVector<> &bbmax) |
Get the entire AABB axis-aligned bounding box of the object. More... | |
virtual void | GetCenter (ChVector<> &mcenter) |
Get a symbolic 'center' of the object. More... | |
virtual void | StreamINstate (ChStreamInBinary &mstream) |
Method to deserialize only the state (position, speed) Must be implemented by child classes. | |
virtual void | StreamOUTstate (ChStreamOutBinary &mstream) |
Method to serialize only the state (position, speed) Must be implemented by child classes. | |
unsigned int | GetOffset_x () |
Get offset in the state vector (position part) | |
unsigned int | GetOffset_w () |
Get offset in the state vector (speed part) | |
unsigned int | GetOffset_L () |
Get offset in the lagrangian multipliers. | |
void | SetOffset_x (const unsigned int moff) |
Set offset in the state vector (position part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_w (const unsigned int moff) |
Set offset in the state vector (speed part) Note: only the ChSystem::Setup function should use this. | |
void | SetOffset_L (const unsigned int moff) |
Set offset in the lagrangian multipliers Note: only the ChSystem::Setup function should use this. | |
![]() | |
ChObj (const ChObj &other) | |
int | GetIdentifier () const |
Gets the numerical identifier of the object. | |
void | SetIdentifier (int id) |
Sets the numerical identifier of the object. | |
double | GetChTime () const |
Gets the simulation time of this object. | |
void | SetChTime (double m_time) |
Sets the simulation time of this object. | |
const char * | GetName () const |
Gets the name of the object as C Ascii null-terminated string -for reading only! | |
void | SetName (const char myname[]) |
Sets the name of this object, as ascii string. | |
std::string | GetNameString () const |
Gets the name of the object as C Ascii null-terminated string. | |
void | SetNameString (const std::string &myname) |
Sets the name of this object, as std::string. | |
void | MFlagsSetAllOFF (int &mflag) |
void | MFlagsSetAllON (int &mflag) |
void | MFlagSetON (int &mflag, int mask) |
void | MFlagSetOFF (int &mflag, int mask) |
int | MFlagGet (int &mflag, int mask) |
virtual std::string & | ArchiveContainerName () |
![]() | |
virtual int | GetNcoords_a () |
Return the number of acceleration coordinates of a in dy/dt={v, a} This is a default implementation that works in almost all cases, as dim(a) = dim(v),. | |
virtual void | StateSetup (ChState &x, ChStateDelta &v, ChStateDelta &a) |
Set up the system state with separate II order components x, v, a for y = {x, v} and dy/dt={v, a}. | |
virtual bool | StateSolveA (ChStateDelta &Dvdt, ChVectorDynamic<> &L, const ChState &x, const ChStateDelta &v, const double T, const double dt, bool force_state_scatter=true) |
Solve for accelerations: a = f(x,v,t) Given current state y={x,v} , computes acceleration a in the state derivative dy/dt={v,a} and lagrangian multipliers L (if any). More... | |
virtual int | GetNcoords_y () override |
Return the number of coordinates in the state Y. More... | |
virtual int | GetNcoords_dy () override |
Return the number of coordinates in the state increment. More... | |
virtual void | StateGather (ChState &y, double &T) override |
Gather system state in specified array. More... | |
virtual void | StateScatter (const ChState &y, const double T) override |
Scatter the states from the provided array to the system. More... | |
virtual void | StateGatherDerivative (ChStateDelta &Dydt) override |
Gather from the system the state derivatives in specified array. More... | |
virtual void | StateScatterDerivative (const ChStateDelta &Dydt) override |
Scatter the state derivatives from the provided array to the system. More... | |
virtual void | StateIncrement (ChState &y_new, const ChState &y, const ChStateDelta &Dy) override |
Increment state array: y_new = y + Dy. More... | |
virtual bool | StateSolve (ChStateDelta &dydt, ChVectorDynamic<> &L, const ChState &y, const double T, const double dt, bool force_state_scatter=true) override |
Solve for state derivatives: dy/dt = f(y,t). More... | |
virtual bool | StateSolveCorrection (ChStateDelta &Dy, ChVectorDynamic<> &L, const ChVectorDynamic<> &R, const ChVectorDynamic<> &Qc, const double a, const double b, const ChState &y, const double T, const double dt, bool force_state_scatter=true, bool force_setup=true) override |
This was for Ist order implicit integrators, but here we disable it. | |
![]() | |
virtual void | StateSetup (ChState &y, ChStateDelta &dy) |
Set up the system state. | |
virtual void | LoadResidual_Hv (ChVectorDynamic<> &R, const ChVectorDynamic<> &v, const double c) |
Increment a vector R (usually the residual in a Newton Raphson iteration for solving an implicit integration step) with a term that has H multiplied a given vector w: R += c*H*w. More... | |
Additional Inherited Members | |
![]() | |
ChParallelDataManager * | data_manager |
int | current_threads |
![]() | |
virtual void | DescriptorPrepareInject (ChSystemDescriptor &mdescriptor) |
Pushes all ChConstraints and ChVariables contained in links, bodies, etc. into the system descriptor. | |
![]() | |
double | old_timer |
double | old_timer_cd |
bool | detect_optimal_threads |
int | detect_optimal_bins |
std::vector< double > | timer_accumulator |
std::vector< double > | cd_accumulator |
uint | frame_threads |
uint | frame_bins |
uint | counter |
std::vector< ChLink * >::iterator | it |
CollisionSystemType | collision_system_type |
![]() | |
std::shared_ptr< ChContactContainer > | contact_container |
the container of contacts | |
ChVector | G_acc |
gravitational acceleration | |
bool | is_initialized |
if false, an initial setup is required (i.e. a call to SetupInitial) | |
bool | is_updated |
if false, a new update is required (i.e. a call to Update) | |
double | end_time |
end of simulation | |
double | step |
time step | |
double | step_min |
min time step | |
double | step_max |
max time step | |
double | tol_force |
tolerance for forces (used to obtain a tolerance for impulses) | |
int | maxiter |
max iterations for nonlinear convergence in DoAssembly() | |
bool | use_sleeping |
if true, put to sleep objects that come to rest | |
std::shared_ptr< ChSystemDescriptor > | descriptor |
system descriptor | |
std::shared_ptr< ChSolver > | solver |
solver for DVI or DAE problem | |
double | min_bounce_speed |
minimum speed for rebounce after impacts. Lower speeds are clamped to 0 | |
double | max_penetration_recovery_speed |
limit for the speed of penetration recovery (positive, speed of exiting) | |
size_t | stepcount |
internal counter for steps | |
int | setupcount |
number of calls to the solver's Setup() | |
int | solvecount |
number of StateSolveCorrection (reset to 0 at each timestep of static analysis) | |
bool | dump_matrices |
for debugging | |
int | ncontacts |
total number of contacts | |
std::shared_ptr< collision::ChCollisionSystem > | collision_system |
collision engine | |
std::vector< CustomCollisionCallback * > | collision_callbacks |
std::unique_ptr< ChMaterialCompositionStrategy< float > > | composition_strategy |
ChTimer< double > | timer_step |
material composition strategy More... | |
ChTimer< double > | timer_advance |
timer for time integration | |
ChTimer< double > | timer_solver |
timer for solver (excluding setup phase) | |
ChTimer< double > | timer_setup |
timer for solver setup | |
ChTimer< double > | timer_jacobian |
timer for computing/loading Jacobian information | |
ChTimer< double > | timer_collision |
timer for collision detection | |
ChTimer< double > | timer_update |
timer for system update | |
std::shared_ptr< ChTimestepper > | timestepper |
time-stepper object | |
bool | last_err |
indicates error over the last kinematic/dynamics/statics | |
![]() | |
std::vector< std::shared_ptr< ChBody > > | bodylist |
list of rigid bodies | |
std::vector< std::shared_ptr< ChLinkBase > > | linklist |
list of joints (links) | |
std::vector< std::shared_ptr< fea::ChMesh > > | meshlist |
list of meshes | |
std::vector< std::shared_ptr< ChPhysicsItem > > | otherphysicslist |
list of other physics objects | |
std::vector< std::shared_ptr< ChPhysicsItem > > | batch_to_insert |
list of items to insert at once | |
int | nbodies |
number of bodies (currently active) | |
int | nlinks |
number of links | |
int | nmeshes |
number of meshes | |
int | nphysicsitems |
number of other physics items | |
int | ncoords |
number of scalar coordinates (including 4th dimension of quaternions) for all active bodies | |
int | ndoc |
number of scalar constraints (including constr. on quaternions) | |
int | nsysvars |
number of variables (coords+lagrangian mult.), i.e. = ncoords+ndoc for all active bodies | |
int | ncoords_w |
number of scalar coordinates when using 3 rot. dof. per body; for all active bodies | |
int | ndoc_w |
number of scalar constraints when using 3 rot. dof. per body; for all active bodies | |
int | nsysvars_w |
number of variables when using 3 rot. dof. per body; i.e. = ncoords_w+ndoc_w | |
int | ndof |
number of degrees of freedom, = ncoords-ndoc = ncoords_w-ndoc_w , | |
int | ndoc_w_C |
number of scalar constraints C, when using 3 rot. dof. per body (excluding unilaterals) | |
int | ndoc_w_D |
number of scalar constraints D, when using 3 rot. dof. per body (only unilaterals) | |
int | nbodies_sleep |
number of bodies that are sleeping | |
int | nbodies_fixed |
number of bodies that are fixed | |
![]() | |
ChSystem * | system |
parent system | |
std::vector< std::shared_ptr< ChAsset > > | assets |
set of assets | |
unsigned int | offset_x |
offset in vector of state (position part) | |
unsigned int | offset_w |
offset in vector of state (speed part) | |
unsigned int | offset_L |
offset in vector of lagrangian multipliers | |
![]() | |
double | ChTime |
the time of simulation for the object | |
Member Function Documentation
◆ GetBodyContactForce() [1/3]
|
inline |
Get the contact force on the specified body.
Note that ComputeContactForces must be called prior to calling this function at any time where reporting of contact forces is desired.
◆ GetBodyContactForce() [2/3]
|
overridevirtual |
Get the contact force on the body with specified id.
Note that ComputeContactForces must be called prior to calling this function at any time where reporting of contact forces is desired.
Implements chrono::ChSystemParallel.
Reimplemented in chrono::ChSystemDistributed.
◆ GetBodyContactForce() [3/3]
virtual real3 chrono::ChSystemParallel::GetBodyContactForce |
Get the contact force on the body with specified id.
Note that ComputeContactForces must be called prior to calling this function at any time where reporting of contact forces is desired.
◆ GetBodyContactTorque() [1/3]
|
inline |
Get the contact torque on the specified body.
Note that ComputeContactForces must be called prior to calling this function at any time where reporting of contact torques is desired.
◆ GetBodyContactTorque() [2/3]
|
overridevirtual |
Get the contact torque on the body with specified id.
Note that ComputeContactForces must be called prior to calling this function at any time where reporting of contact torques is desired.
Implements chrono::ChSystemParallel.
◆ GetBodyContactTorque() [3/3]
virtual real3 chrono::ChSystemParallel::GetBodyContactTorque |
Get the contact torque on the body with specified id.
Note that ComputeContactForces must be called prior to calling this function at any time where reporting of contact torques is desired.
◆ GetContactMethod()
|
inlineoverridevirtual |
Return the contact method supported by this system.
Bodies added to this system must be compatible.
Implements chrono::ChSystem.
◆ NewBody()
|
overridevirtual |
Create and return the pointer to a new body.
The returned body is created with a contact model consistent with the type of this ChSystem and with the collision system currently associated with this ChSystem. Note that the body is not attached to this system.
Implements chrono::ChSystem.
Reimplemented in chrono::ChSystemDistributed.
◆ NewBodyAuxRef()
|
overridevirtual |
Create and return the pointer to a new body with auxiliary reference frame.
The returned body is created with a contact model consistent with the type of this ChSystem and with the collision system currently associated with this ChSystem. Note that the body is not attached to this system.
Implements chrono::ChSystem.
Reimplemented in chrono::ChSystemDistributed.
◆ Setup()
|
overridevirtual |
Counts the number of bodies and links.
Computes the offsets of object states in the global state. Assumes that offset_x, offset_w, and offset_L are already set as starting point for offsetting all the contained sub objects.
Reimplemented from chrono::ChSystemParallel.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_parallel/physics/ChSystemParallel.h
- /builds/uwsbel/chrono/src/chrono_parallel/physics/ChSystemParallelSMC.cpp