Description
Base class for a torsion-bar suspension system using a rotational damper (template definition).
#include <ChRotationalDamperRWAssembly.h>
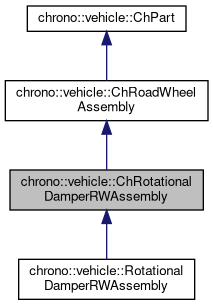
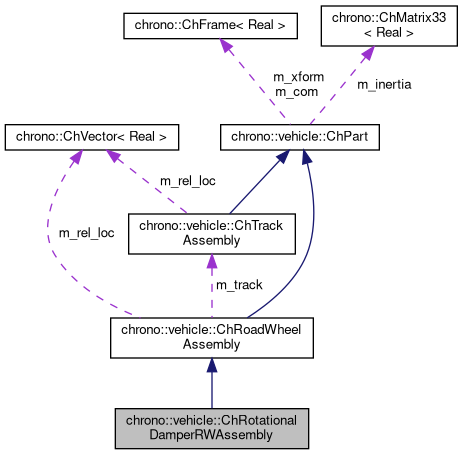
Public Member Functions | |
ChRotationalDamperRWAssembly (const std::string &name, bool has_shock=true) | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual std::shared_ptr< ChBody > | GetCarrierBody () const override |
Get a handle to the carrier body. | |
virtual double | GetCarrierAngle () const override |
Return the current pitch angle of the carrier body. More... | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis, const ChVector<> &location, ChTrackAssembly *track) override |
Initialize this suspension subsystem. More... | |
virtual ForceTorque | ReportSuspensionForce () const override |
Return current suspension forces or torques, as appropriate (spring and shock). More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the suspension subsystem. | |
virtual void | RemoveVisualizationAssets () override final |
Remove visualization assets for the suspension subsystem. | |
void | LogConstraintViolations () override |
Log current constraint violations. | |
![]() | |
GuidePinType | GetType () const |
Return the type of track shoe consistent with this road wheel. | |
std::shared_ptr< ChRoadWheel > | GetRoadWheel () const |
Return a handle to the road wheel subsystem. | |
std::shared_ptr< ChBody > | GetWheelBody () const |
Get a handle to the road wheel body. | |
std::shared_ptr< ChLinkLockRevolute > | GetWheelRevolute () const |
Get a handle to the revolute joint of the road-wheel. | |
double | GetWheelRadius () const |
Get the radius of the road wheel. | |
virtual void | SetOutput (bool state) override |
Enable/disable output for this subsystem. More... | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Protected Types | |
enum | PointId { ARM, ARM_WHEEL, ARM_CHASSIS, NUM_POINTS } |
Identifiers for the various hardpoints. More... | |
Protected Member Functions | |
virtual void | InitializeInertiaProperties () override |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties () override |
Update subsystem inertia properties. More... | |
virtual const ChVector | GetLocation (PointId which)=0 |
Return the location of the specified hardpoint. More... | |
virtual double | GetArmMass () const =0 |
Return the mass of the arm body. | |
virtual const ChVector & | GetArmInertia () const =0 |
Return the moments of inertia of the arm body. | |
virtual double | GetArmVisRadius () const =0 |
Return a visualization radius for the arm body. | |
virtual std::shared_ptr< ChLinkRSDA::TorqueFunctor > | GetSpringTorqueFunctor () const =0 |
Return the functor object for the torsional spring torque. | |
virtual std::shared_ptr< ChLinkRSDA::TorqueFunctor > | GetShockTorqueCallback () const =0 |
Return the functor object for the rotational shock force. | |
virtual std::shared_ptr< ChVehicleBushingData > | getArmBushingData () const |
Return stiffness and damping data for the arm bushing. More... | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const override |
Export this subsystem's component list to the specified JSON object. More... | |
virtual void | Output (ChVehicleOutput &database) const override |
Output data for this subsystem's component list to the specified database. | |
![]() | |
ChRoadWheelAssembly (const std::string &name, bool has_shock) | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector<> &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
Protected Attributes | |
std::shared_ptr< ChBody > | m_arm |
handle to the trailing arm body | |
std::shared_ptr< ChVehicleJoint > | m_revolute |
handle to the revolute joint arm-chassis | |
std::shared_ptr< ChLinkRSDA > | m_spring |
handle to the rotational spring link | |
std::shared_ptr< ChLinkRSDA > | m_shock |
handle to the rotational shock link | |
![]() | |
GuidePinType | m_type |
type of the track shoe matching this road wheel | |
bool | m_has_shock |
specifies whether or not the suspension has a damper | |
ChVector | m_rel_loc |
idler subsystem location relative to chassis | |
std::shared_ptr< ChRoadWheel > | m_road_wheel |
road-wheel subsystem | |
ChTrackAssembly * | m_track |
containing track assembly | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Member Enumeration Documentation
◆ PointId
|
protected |
Constructor & Destructor Documentation
◆ ChRotationalDamperRWAssembly()
chrono::vehicle::ChRotationalDamperRWAssembly::ChRotationalDamperRWAssembly | ( | const std::string & | name, |
bool | has_shock = true |
||
) |
- Parameters
-
[in] name name of the subsystem [in] has_shock specify whether or not the suspension has a damper
Member Function Documentation
◆ ExportComponentList()
|
overrideprotectedvirtual |
Export this subsystem's component list to the specified JSON object.
Derived classes should override this function and first invoke the base class implementation, followed by calls to the various static Export***List functions, as appropriate.
Reimplemented from chrono::vehicle::ChRoadWheelAssembly.
◆ getArmBushingData()
|
inlineprotectedvirtual |
Return stiffness and damping data for the arm bushing.
Returning nullptr (default) results in using a kinematic revolute joint.
◆ GetCarrierAngle()
|
overridevirtual |
Return the current pitch angle of the carrier body.
This angle is measured in the x-z transversal plane, from the initial configuration, and follows the right-hand rule.
Implements chrono::vehicle::ChRoadWheelAssembly.
◆ GetLocation()
|
protectedpure virtual |
Return the location of the specified hardpoint.
The returned location must be expressed in the idler subsystem reference frame.
◆ Initialize()
|
overridevirtual |
Initialize this suspension subsystem.
The suspension subsystem is initialized by attaching it to the specified chassis body at the specified location (with respect to and expressed in the reference frame of the chassis). It is assumed that the suspension reference frame is always centered at the location of the road wheel and aligned with the chassis reference frame.
- Parameters
-
[in] chassis associated chassis subsystem [in] location location relative to the chassis frame [in] track containing track assembly
Reimplemented from chrono::vehicle::ChRoadWheelAssembly.
◆ InitializeInertiaProperties()
|
overrideprotectedvirtual |
Initialize subsystem inertia properties.
Derived classes must override this function and set the subsystem mass (m_mass) and, if constant, the subsystem COM frame and its inertia tensor. This function is called during initialization of the vehicle system.
Implements chrono::vehicle::ChPart.
◆ ReportSuspensionForce()
|
overridevirtual |
Return current suspension forces or torques, as appropriate (spring and shock).
A ChRotationalDamperRWAssembly returns torques for both the spring and the damper.
Implements chrono::vehicle::ChRoadWheelAssembly.
◆ UpdateInertiaProperties()
|
overrideprotectedvirtual |
Update subsystem inertia properties.
Derived classes must override this function and set the global subsystem transform (m_xform) and, unless constant, the subsystem COM frame (m_com) and its inertia tensor (m_inertia). Calculate the current inertia properties and global frame of this subsystem. This function is called every time the state of the vehicle system is advanced in time.
Implements chrono::vehicle::ChPart.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/suspension/ChRotationalDamperRWAssembly.h
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/suspension/ChRotationalDamperRWAssembly.cpp