Description
Base class for tracked vehicle suspension (road-wheel assembly) subsystem.
#include <ChRoadWheelAssembly.h>
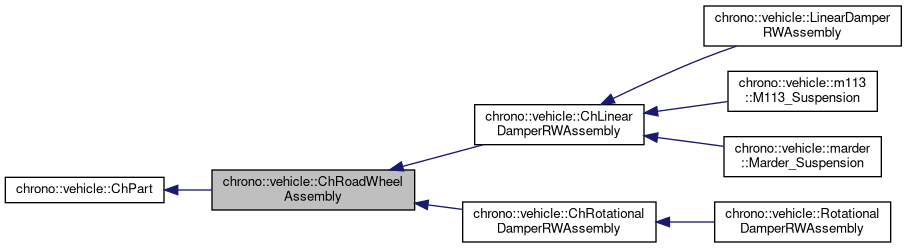
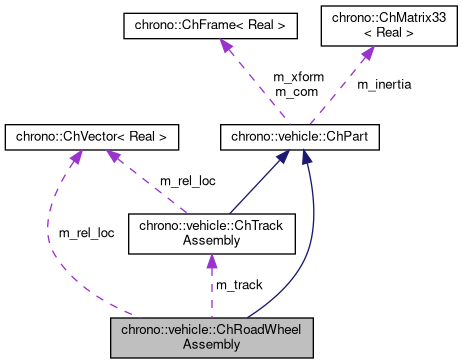
Classes | |
struct | ForceTorque |
Output structure for spring-damper forces or torques. More... | |
Public Member Functions | |
GuidePinType | GetType () const |
Return the type of track shoe consistent with this road wheel. | |
std::shared_ptr< ChRoadWheel > | GetRoadWheel () const |
Return a handle to the road wheel subsystem. | |
virtual std::shared_ptr< ChBody > | GetCarrierBody () const =0 |
Return a handle to the carrier body. | |
virtual double | GetCarrierAngle () const =0 |
Return the current pitch angle of the carrier body. More... | |
std::shared_ptr< ChBody > | GetWheelBody () const |
Get a handle to the road wheel body. | |
std::shared_ptr< ChLinkLockRevolute > | GetWheelRevolute () const |
Get a handle to the revolute joint of the road-wheel. | |
double | GetWheelRadius () const |
Get the radius of the road wheel. | |
virtual void | Initialize (std::shared_ptr< ChChassis > chassis, const ChVector<> &location, ChTrackAssembly *track) |
Initialize this suspension subsystem. More... | |
virtual ForceTorque | ReportSuspensionForce () const =0 |
Return current suspension forces or torques, as appropriate (spring and shock). More... | |
virtual void | SetOutput (bool state) override |
Enable/disable output for this subsystem. More... | |
virtual void | LogConstraintViolations ()=0 |
Log current constraint violations. | |
![]() | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
virtual std::string | GetTemplateName () const =0 |
Get the name of the vehicle subsystem template. | |
double | GetMass () const |
Get the subsystem mass. More... | |
const ChFrame & | GetCOMFrame () const |
Get the current subsystem COM frame (relative to and expressed in the subsystem's reference frame). More... | |
const ChMatrix33 & | GetInertia () const |
Get the current subsystem inertia (relative to the subsystem COM frame). More... | |
const ChFrame & | GetTransform () const |
Get the current subsystem position relative to the global frame. More... | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
virtual void | AddVisualizationAssets (VisualizationType vis) |
Add visualization assets to this subsystem, for the specified visualization mode. | |
virtual void | RemoveVisualizationAssets () |
Remove all visualization assets from this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
virtual void | Output (ChVehicleOutput &database) const |
Output data for this subsystem's component list to the specified database. | |
Protected Member Functions | |
ChRoadWheelAssembly (const std::string &name, bool has_shock) | |
virtual void | ExportComponentList (rapidjson::Document &jsonDocument) const override |
Export this subsystem's component list to the specified JSON object. More... | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. | |
virtual void | InitializeInertiaProperties ()=0 |
Initialize subsystem inertia properties. More... | |
virtual void | UpdateInertiaProperties ()=0 |
Update subsystem inertia properties. More... | |
void | AddMass (double &mass) |
Add this subsystem's mass. More... | |
void | AddInertiaProperties (ChVector<> &com, ChMatrix33<> &inertia) |
Add this subsystem's inertia properties. More... | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
Protected Attributes | |
GuidePinType | m_type |
type of the track shoe matching this road wheel | |
bool | m_has_shock |
specifies whether or not the suspension has a damper | |
ChVector | m_rel_loc |
idler subsystem location relative to chassis | |
std::shared_ptr< ChRoadWheel > | m_road_wheel |
road-wheel subsystem | |
ChTrackAssembly * | m_track |
containing track assembly | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
std::shared_ptr< ChPart > | m_parent |
parent subsystem (empty if parent is vehicle) | |
double | m_mass |
subsystem mass | |
ChMatrix33 | m_inertia |
inertia tensor (relative to subsystem COM) | |
ChFrame | m_com |
COM frame (relative to subsystem reference frame) | |
ChFrame | m_xform |
subsystem frame expressed in the global frame | |
Friends | |
class | ChTrackAssembly |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRSDA >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
static void | RemoveVisualizationAssets (std::shared_ptr< ChPhysicsItem > item) |
Erase all visual shapes from the visual model associated with the specified physics item (if any). | |
static void | RemoveVisualizationAsset (std::shared_ptr< ChPhysicsItem > item, std::shared_ptr< ChVisualShape > shape) |
Erase the given shape from the visual model associated with the specified physics item (if any). | |
Constructor & Destructor Documentation
◆ ChRoadWheelAssembly()
|
protected |
- Parameters
-
[in] name name of the subsystem [in] has_shock specify whether or not the suspension has a damper
Member Function Documentation
◆ ExportComponentList()
|
overrideprotectedvirtual |
Export this subsystem's component list to the specified JSON object.
Derived classes should override this function and first invoke the base class implementation, followed by calls to the various static Export***List functions, as appropriate.
Reimplemented from chrono::vehicle::ChPart.
Reimplemented in chrono::vehicle::ChLinearDamperRWAssembly, and chrono::vehicle::ChRotationalDamperRWAssembly.
◆ GetCarrierAngle()
|
pure virtual |
Return the current pitch angle of the carrier body.
This angle is measured in the x-z transversal plane, from the initial configuration, and follows the right-hand rule.
Implemented in chrono::vehicle::ChLinearDamperRWAssembly, and chrono::vehicle::ChRotationalDamperRWAssembly.
◆ Initialize()
|
virtual |
Initialize this suspension subsystem.
The suspension subsystem is initialized by attaching it to the specified chassis body at the specified location (with respect to and expressed in the reference frame of the chassis). It is assumed that the suspension reference frame is always centered at the location of the road wheel and aligned with the chassis reference frame. Derived classes must call this base class implementation (which only initializes the road wheel).
- Parameters
-
[in] chassis associated chassis subsystem [in] location location relative to the chassis frame [in] track containing track assembly
Reimplemented in chrono::vehicle::ChLinearDamperRWAssembly, and chrono::vehicle::ChRotationalDamperRWAssembly.
◆ ReportSuspensionForce()
|
pure virtual |
Return current suspension forces or torques, as appropriate (spring and shock).
Different derived types (suspension templates) may load different quantities in the output struct.
Implemented in chrono::vehicle::ChLinearDamperRWAssembly, and chrono::vehicle::ChRotationalDamperRWAssembly.
◆ SetOutput()
|
overridevirtual |
Enable/disable output for this subsystem.
This function overrides the output setting for all components of this suspension assembly.
Reimplemented from chrono::vehicle::ChPart.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/ChRoadWheelAssembly.h
- /builds/uwsbel/chrono/src/chrono_vehicle/tracked_vehicle/ChRoadWheelAssembly.cpp