Description
Base class for a visualization asset for rendering (run-time or post processing).
Encapsulates basic information about the shape position, materials, and visibility.
#include <ChVisualShape.h>
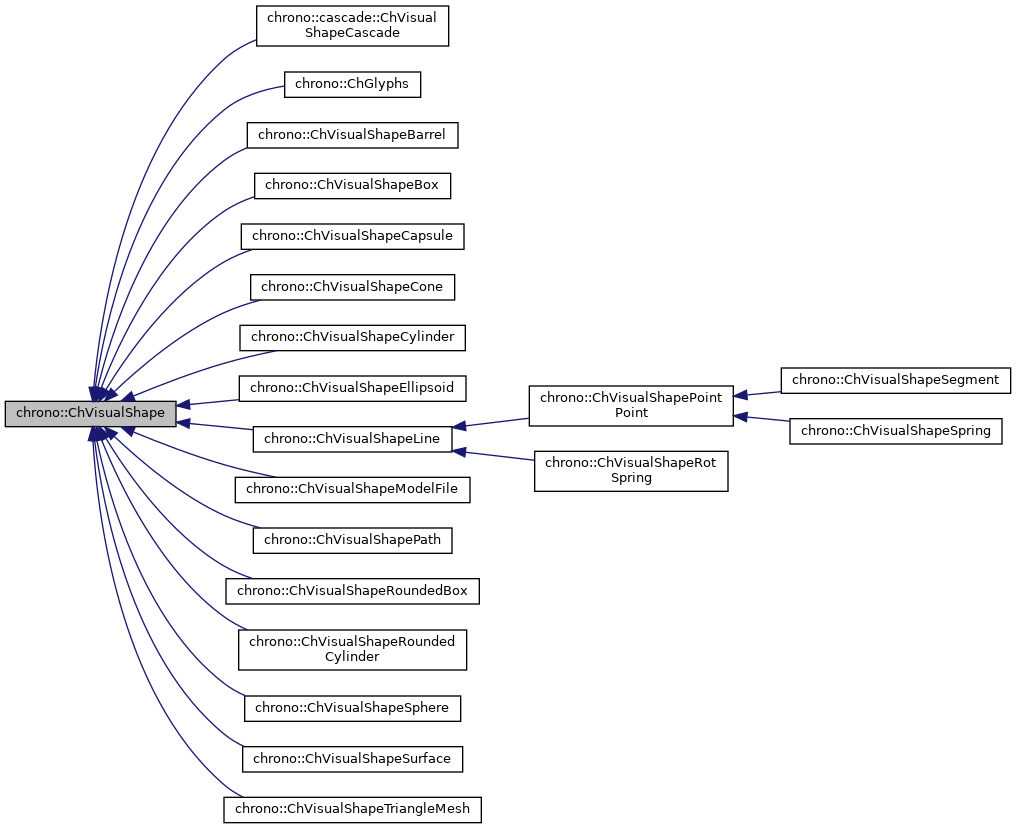
Public Member Functions | |
void | SetVisible (bool mv) |
Set this visualization asset as visible. | |
bool | IsVisible () const |
Return true if the asset is set as visible. | |
void | SetColor (const ChColor &col) |
Set the diffuse color for this shape. More... | |
ChColor | GetColor () const |
Return the diffuse color of the first material in the list of materials for this shape. More... | |
void | SetOpacity (float val) |
Set opacity for this shape (0: fully transparent; 1: fully opaque). More... | |
float | GetOpacity () const |
Get opacity of the first material in the list of materials for this shape. More... | |
void | SetTexture (const std::string &filename, float scale_x=1, float scale_y=1) |
Set the diffuse texture map for this shape. More... | |
std::string | GetTexture () const |
Return the diffuse texture map of the first material in the list of materials for this shape. More... | |
void | SetMutable (bool val) |
Set this visualization shape as modifiable (default: false for primitive shapes, true otherwise). More... | |
bool | IsMutable () const |
Return true if the visualization shape is marked as modifiable. | |
int | AddMaterial (std::shared_ptr< ChVisualMaterial > material) |
Add a visualization material and return its index in the list of materials. | |
void | SetMaterial (int i, std::shared_ptr< ChVisualMaterial > material) |
Replace the material with specified index. More... | |
std::vector< std::shared_ptr< ChVisualMaterial > > & | GetMaterials () |
Get the list of visualization materials. | |
std::shared_ptr< ChVisualMaterial > | GetMaterial (int i) |
Get the specified material in the list. | |
unsigned int | GetNumMaterials () const |
Get the number of visualization materials. | |
virtual ChAABB | GetBoundingBox () const |
Get the shape bounding box. More... | |
virtual void | ArchiveOut (ChArchiveOut &archive_out) |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &archive_in) |
Method to allow de-serialization of transient data from archives. | |
Protected Member Functions | |
virtual void | Update (ChObj *updater, const ChFrame<> &frame) |
Update this visual shape with information for the owning object. More... | |
Protected Attributes | |
bool | visible |
shape visibility flag | |
bool | is_mutable |
flag indicating whether the shape is rigid or deformable | |
std::vector< std::shared_ptr< ChVisualMaterial > > | material_list |
list of visualization materials | |
Friends | |
class | ChVisualModel |
Member Function Documentation
◆ GetBoundingBox()
|
inlinevirtual |
Get the shape bounding box.
The default implementation returns an inverted AABB.
Reimplemented in chrono::ChVisualShapeSurface, chrono::ChVisualShapeTriangleMesh, chrono::ChVisualShapeLine, chrono::ChVisualShapeBarrel, chrono::ChVisualShapeRoundedBox, chrono::ChVisualShapeCapsule, chrono::ChVisualShapeRoundedCylinder, chrono::ChVisualShapeBox, chrono::ChVisualShapeCylinder, chrono::ChVisualShapePath, chrono::ChVisualShapeEllipsoid, chrono::ChVisualShapeCone, chrono::ChVisualShapeSphere, and chrono::ChVisualShapeModelFile.
◆ GetColor()
ChColor chrono::ChVisualShape::GetColor | ( | ) | const |
Return the diffuse color of the first material in the list of materials for this shape.
If no materials are defined, return the color of the default material.
◆ GetOpacity()
float chrono::ChVisualShape::GetOpacity | ( | ) | const |
Get opacity of the first material in the list of materials for this shape.
If no materials are defined, return the color of the default material.
◆ GetTexture()
std::string chrono::ChVisualShape::GetTexture | ( | ) | const |
Return the diffuse texture map of the first material in the list of materials for this shape.
If no materials are defined, return an empty string (no texture for the default material).
◆ SetColor()
void chrono::ChVisualShape::SetColor | ( | const ChColor & | col | ) |
Set the diffuse color for this shape.
This changes the color of the first material in the list of materials for this shape. If no materials are defined for a shape, one is first created by duplicating the default material.
◆ SetMaterial()
void chrono::ChVisualShape::SetMaterial | ( | int | i, |
std::shared_ptr< ChVisualMaterial > | material | ||
) |
Replace the material with specified index.
No-op if there is no material with given index.
◆ SetMutable()
|
inline |
Set this visualization shape as modifiable (default: false for primitive shapes, true otherwise).
Set to false to indicate that the asset never changes and therefore does not require updates (e.g. for a non-deformable triangular mesh). Note that this also includes changes in materials. A particular visualization system may take advantage of this setting to accelerate rendering.
◆ SetOpacity()
void chrono::ChVisualShape::SetOpacity | ( | float | val | ) |
Set opacity for this shape (0: fully transparent; 1: fully opaque).
This changes the first material in the list of materials for this shape. If no materials are defined for a shape, one is first created by duplicating the default material.
◆ SetTexture()
void chrono::ChVisualShape::SetTexture | ( | const std::string & | filename, |
float | scale_x = 1 , |
||
float | scale_y = 1 |
||
) |
Set the diffuse texture map for this shape.
This changes the texture of the first material in the list of materials for this shape. If no materials are defined for a shape, one is first created by duplicating the default material.
◆ Update()
|
inlineprotectedvirtual |
Update this visual shape with information for the owning object.
Since a visual shape can be shared in multiple instances, this function may be called with different updaters.
Reimplemented in chrono::peridynamics::ChVisualPeriBBimplicitBonds, chrono::peridynamics::ChVisualPeriBBimplicit, chrono::peridynamics::ChVisualPeriLinearElasticBonds, chrono::peridynamics::ChVisualPeriLinearElastic, chrono::peridynamics::ChVisualPeriBBBonds, chrono::peridynamics::ChVisualPeriBB, chrono::peridynamics::ChVisualPeriLiquid, chrono::ChVisualShapeRotSpring, and chrono::ChVisualShapePointPoint.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/assets/ChVisualShape.h
- /builds/uwsbel/chrono/src/chrono/assets/ChVisualShape.cpp