Description
VSG-based Chrono run-time visualization system.
#include <ChVisualSystemVSG.h>

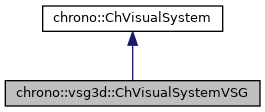
Classes | |
struct | DeformableMesh |
Data related to deformable meshes (FEA and SCM). More... | |
struct | ParticleCloud |
Data for particle clouds. More... | |
Public Member Functions | |
ChVisualSystemVSG (int num_divs=24) | |
Create the Chrono::VSG run-time visualization system. More... | |
virtual void | Initialize () override |
Initialize the visualization system. | |
virtual void | BindAll () override |
Process all visual assets in the associated ChSystem. More... | |
virtual void | BindItem (std::shared_ptr< ChPhysicsItem > item) override |
Process the visual assets for the specified physics item. More... | |
virtual bool | Run () override |
Check if rendering is running. More... | |
virtual void | Quit () override |
Terminate the visualization system. | |
virtual void | BeginScene () override |
Perform any necessary operations at the beginning of each rendering frame. | |
virtual void | Render () override |
Draw all 3D shapes and GUI elements at the current frame. More... | |
virtual void | RenderCOGFrames (double axis_length=1) override |
Render COG frames for all bodies in the system. | |
void | SetCOGFrameScale (double axis_length) |
void | ToggleCOGFrameVisibility () |
void | RenderJointFrames (double axis_length=1) |
Render joint frames for all links in the system. | |
void | SetJointFrameScale (double axis_length) |
void | ToggleJointFrameVisibility () |
virtual void | EndScene () override |
End the scene draw at the end of each animation frame. | |
virtual void | WriteImageToFile (const std::string &filename) override |
Create a snapshot of the frame to be rendered and save it to the provided file. More... | |
void | SetWindowSize (const ChVector2i &size) |
void | SetWindowSize (int width, int height) |
void | SetWindowPosition (const ChVector2i &pos) |
void | SetWindowPosition (int from_left, int from_top) |
void | SetWindowTitle (const std::string &title) |
void | SetClearColor (const ChColor &color) |
void | SetOutputScreen (int screenNum=0) |
void | SetFullscreen (bool yesno=false) |
void | SetUseSkyBox (bool yesno) |
void | SetWireFrameMode (bool mode=true) |
Draw the scene objects as wireframes. | |
void | SetCameraVertical (CameraVerticalDir upDir) |
Set the camera up vector (default: Z). | |
virtual int | AddCamera (const ChVector3d &pos, ChVector3d targ=VNULL) override |
Add a camera to the VSG scene. More... | |
virtual void | SetCameraPosition (int id, const ChVector3d &pos) override |
Set the location of the specified camera. | |
virtual void | SetCameraTarget (int id, const ChVector3d &target) override |
Set the target (look-at) point of the specified camera. | |
virtual void | SetCameraPosition (const ChVector3d &pos) override |
Set the location of the current (active) camera. | |
virtual void | SetCameraTarget (const ChVector3d &target) override |
Set the target (look-at) point of the current (active) camera. | |
virtual ChVector3d | GetCameraPosition () const override |
Get the location of the current (active) camera. | |
virtual ChVector3d | GetCameraTarget () const override |
Get the target (look-at) point of the current (active) camera. | |
double | GetRenderingFPS () const |
Get estimated FPS. | |
void | SetShadows (bool yesno=false) |
Enable/disable rendering of shadows. More... | |
void | SetLightIntensity (float intensity) |
void | SetLightDirection (double azimuth, double elevation) |
void | SetCameraAngleDeg (double angleDeg) |
void | SetGuiFontSize (float theSize) |
virtual void | AddGrid (double x_step, double y_step, int nx, int ny, ChCoordsys<> pos=CSYSNORM, ChColor col=ChColor(0.1f, 0.1f, 0.1f)) override |
Add a grid with specified parameters in the x-y plane of the given frame. More... | |
virtual int | AddVisualModel (std::shared_ptr< ChVisualModel > model, const ChFrame<> &frame) override |
Add a visual model not associated with a physical item. More... | |
virtual int | AddVisualModel (std::shared_ptr< ChVisualShape > model, const ChFrame<> &frame) override |
Add a visual model not associated with a physical item. More... | |
virtual void | UpdateVisualModel (int id, const ChFrame<> &frame) override |
Update the position of the specified visualization-only model. | |
size_t | AddGuiComponent (std::shared_ptr< ChGuiComponentVSG > gc) |
Add a user-defined GUI component. More... | |
size_t | AddGuiColorbar (const std::string &title, double min_val, double max_val) |
Add a colorbar as a GUI component. More... | |
std::shared_ptr< ChGuiComponentVSG > | GetGuiComponent (size_t id) |
Access the specified GUI component. More... | |
void | SetGuiVisibility (bool show_gui) |
Set visibility for all GUI components (default: true). | |
void | ToggleGuiVisibility () |
Toggle GUI visibility for all GUI components. | |
bool | IsGuiVisible () const |
Return boolean indicating whether or not GUI are visible. | |
void | SetBaseGuiVisibility (bool show_gui) |
Set visibility for the default (base) GUI component (default: true). | |
void | ToggleBaseGuiVisibility () |
Toggle GUI visibility for the default (base) GUI component. | |
bool | IsBaseGuiVisible () const |
Return boolean indicating whether or not the default (base) GUI is visible. | |
void | SetLogoVisible (bool yesno) |
Set logo visible (default: true). | |
void | SetLogoHeight (float height) |
Set logo display height (in pixels, default: 64). | |
void | SetLogoPosition (const ChVector2f &position) |
Set logo position (default: [10,10]). More... | |
bool | IsLogoVisible () const |
Return boolean indicating whether or not logo is visible. | |
void | AddEventHandler (std::shared_ptr< ChEventHandlerVSG > eh) |
Add a user-defined VSG event handler. | |
![]() | |
void | SetVerbose (bool verbose) |
Enable/disable information terminal output during initialization (default: false). | |
virtual void | AttachSystem (ChSystem *sys) |
Attach a Chrono system to this visualization system. | |
virtual void | UnbindItem (std::shared_ptr< ChPhysicsItem > item) |
Remove the visual assets for the specified physics item from this visualization system. | |
void | UpdateCamera (int id, const ChVector3d &pos, ChVector3d target) |
Update the location and/or target points of the specified camera. | |
void | UpdateCamera (const ChVector3d &pos, ChVector3d target) |
virtual void | RenderFrame (const ChFrame<> &frame, double axis_length=1) |
Render the specified reference frame. | |
virtual double | GetSimulationRTF () const |
Return the simulation real-time factor (simulation time / simulated time). More... | |
virtual double | GetSimulationTime () const |
Return the current simulated time. More... | |
void | SetImageOutputDirectory (const std::string &dir) |
Set output directory for saving frame snapshots (default: "."). | |
void | SetImageOutput (bool val) |
Enable/disable writing of frame snapshots to file. | |
std::vector< ChSystem * > | GetSystems () const |
Get the list of associated Chrono systems. | |
ChSystem & | GetSystem (int i) const |
Get the specified associated Chrono system. | |
Protected Member Functions | |
virtual void | OnSetup (ChSystem *sys) override |
Perform necessary setup operations at the beginning of a time step. | |
void | UpdateFromMBS () |
void | exportScreenImage () |
export screen image as file (png, bmp, tga, jpg) | |
![]() | |
virtual void | OnUpdate (ChSystem *sys) |
Perform any necessary update operations at the end of a time step. More... | |
virtual void | OnClear (ChSystem *sys) |
Remove all visualization objects from this visualization system. More... | |
Protected Attributes | |
int | m_screen_num = -1 |
bool | m_use_fullscreen = false |
bool | m_use_shadows = false |
vsg::ref_ptr< vsg::Window > | m_window |
vsg::ref_ptr< vsg::Viewer > | m_viewer |
high-level VSG rendering manager | |
vsg::ref_ptr< vsg::RenderGraph > | m_renderGraph |
bool | m_show_logo |
float | m_logo_height |
ChVector2f | m_logo_pos |
std::string | m_logo_filename |
bool | m_show_gui |
flag to toggle global GUI visibility | |
bool | m_show_base_gui |
flag to toggle base GUI visibility | |
size_t | m_camera_gui |
identifier for the camera info GUI component | |
std::shared_ptr< ChGuiComponentVSG > | m_base_gui |
default (base) GUI component | |
std::vector< std::shared_ptr< ChGuiComponentVSG > > | m_gui |
list of all additional GUI components | |
std::vector< std::shared_ptr< ChEventHandlerVSG > > | m_evhandler |
list of all additional event handlers | |
vsg::dvec3 | m_vsg_cameraEye = vsg::dvec3(-10.0, 0.0, 0.0) |
vsg::dvec3 | m_vsg_cameraTarget = vsg::dvec3(0.0, 0.0, 0.0) |
vsg::ref_ptr< vsg::LookAt > | m_lookAt |
vsg::ref_ptr< vsg::Camera > | m_vsg_camera |
bool | m_camera_trackball |
create a camera trackball control? | |
vsg::ref_ptr< vsg::Group > | m_scene |
vsg::ref_ptr< vsg::Group > | m_bodyScene |
vsg::ref_ptr< vsg::Group > | m_pointpointScene |
vsg::ref_ptr< vsg::Group > | m_particleScene |
vsg::ref_ptr< vsg::Group > | m_deformableScene |
vsg::ref_ptr< vsg::Group > | m_decoScene |
vsg::ref_ptr< vsg::Switch > | m_cogFrameScene |
vsg::ref_ptr< vsg::Switch > | m_jointFrameScene |
vsg::ref_ptr< vsg::Options > | m_options |
I/O related options for vsg::read/write calls. | |
vsg::ref_ptr< vsg::Builder > | m_vsgBuilder |
vsg::ref_ptr< ShapeBuilder > | m_shapeBuilder |
bool | m_wireframe |
draw as wireframes | |
bool | m_capture_image |
export current frame to image file | |
std::string | m_imageFilename |
name of file to export current frame | |
std::vector< DeformableMesh > | m_def_meshes |
std::vector< ParticleCloud > | m_clouds |
![]() | |
bool | m_verbose |
terminal output | |
bool | m_initialized |
std::vector< ChSystem * > | m_systems |
associated Chrono system(s) | |
bool | m_write_images |
if true, save snapshots | |
std::string | m_image_dir |
directory for image files | |
Friends | |
class | ChMainGuiVSG |
class | ChBaseGuiComponentVSG |
class | ChBaseEventHandlerVSG |
Additional Inherited Members | |
![]() | |
enum | Type { Type::IRRLICHT, Type::VSG, Type::OpenGL, Type::OptiX, NONE } |
Supported run-time visualization systems. More... | |
Constructor & Destructor Documentation
◆ ChVisualSystemVSG()
chrono::vsg3d::ChVisualSystemVSG::ChVisualSystemVSG | ( | int | num_divs = 24 | ) |
Create the Chrono::VSG run-time visualization system.
Optionally, specify the resolution used for tesselation of primitive shapes, by providing the number of divisions used to discretize a full circle. The default value of 24 corresponds to 15-degree divisions.
Member Function Documentation
◆ AddCamera()
|
overridevirtual |
Add a camera to the VSG scene.
Note that currently only one camera is supported.
Reimplemented from chrono::ChVisualSystem.
◆ AddGrid()
|
overridevirtual |
Add a grid with specified parameters in the x-y plane of the given frame.
- Parameters
-
x_step grid cell size in X direction y_step grid cell size in Y direction nx number of cells in X direction ny number of cells in Y direction pos grid reference frame col grid line color
Reimplemented from chrono::ChVisualSystem.
◆ AddGuiColorbar()
size_t chrono::vsg3d::ChVisualSystemVSG::AddGuiColorbar | ( | const std::string & | title, |
double | min_val, | ||
double | max_val | ||
) |
Add a colorbar as a GUI component.
Returns the index of the new component. This function must be called before Initialize().
◆ AddGuiComponent()
size_t chrono::vsg3d::ChVisualSystemVSG::AddGuiComponent | ( | std::shared_ptr< ChGuiComponentVSG > | gc | ) |
Add a user-defined GUI component.
Returns the index of the new component. This function must be called before Initialize().
◆ AddVisualModel() [1/2]
|
overridevirtual |
Add a visual model not associated with a physical item.
Return an ID which can be used later to modify the position of this visual model.
Reimplemented from chrono::ChVisualSystem.
◆ AddVisualModel() [2/2]
|
overridevirtual |
Add a visual model not associated with a physical item.
This version constructs a visual model consisting of the single specified shape Return an ID which can be used later to modify the position of this visual model.
Reimplemented from chrono::ChVisualSystem.
◆ BindAll()
|
overridevirtual |
Process all visual assets in the associated ChSystem.
This function is called by default by Initialize(), but can also be called later if further modifications to visualization assets occur.
Reimplemented from chrono::ChVisualSystem.
◆ BindItem()
|
overridevirtual |
Process the visual assets for the specified physics item.
This function must be called if a new physics item is added to the system or if changes to its visual model occur after the call to Initialize().
Reimplemented from chrono::ChVisualSystem.
◆ GetGuiComponent()
std::shared_ptr< ChGuiComponentVSG > chrono::vsg3d::ChVisualSystemVSG::GetGuiComponent | ( | size_t | id | ) |
Access the specified GUI component.
Identify the GUI component with the index returned by AddGuiComponent.
◆ Render()
|
overridevirtual |
Draw all 3D shapes and GUI elements at the current frame.
This function is typically called inside a loop such as
while(vis->Run()) {...}
Implements chrono::ChVisualSystem.
◆ Run()
|
overridevirtual |
Check if rendering is running.
Returns false
if the viewer was closed.
Implements chrono::ChVisualSystem.
◆ SetLogoPosition()
|
inline |
Set logo position (default: [10,10]).
This is the position of the right-top corner of the logo image (in pixels) relative to the right-top corner of the rendering window.
◆ SetShadows()
|
inline |
Enable/disable rendering of shadows.
This function must be called before Initialize().
◆ WriteImageToFile()
|
overridevirtual |
Create a snapshot of the frame to be rendered and save it to the provided file.
The file extension determines the image format.
Reimplemented from chrono::ChVisualSystem.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vsg/ChVisualSystemVSG.h
- /builds/uwsbel/chrono/src/chrono_vsg/ChVisualSystemVSG.cpp