Public Types |
Public Member Functions |
Protected Member Functions |
Protected Attributes |
List of all members
chrono::vehicle::mrole::mrole Class Referenceabstract
Description
Definition of the mrole assembly.
This class encapsulates a concrete wheeled vehicle model with parameters corresponding to a mrole, the powertrain model, and the 8 tires. It provides wrappers to access the different systems and subsystems, functions for specifying the driveline, powertrain, and tire types, as well as functions for controlling the visualization mode of each component. Note that this is an abstract class which cannot be instantiated. Instead, use one of the concrete classes mrole_Full or mrole_Reduced.
#include <mrole.h>
Inheritance diagram for chrono::vehicle::mrole::mrole:
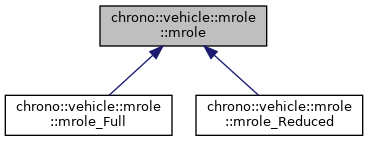
Collaboration diagram for chrono::vehicle::mrole::mrole:
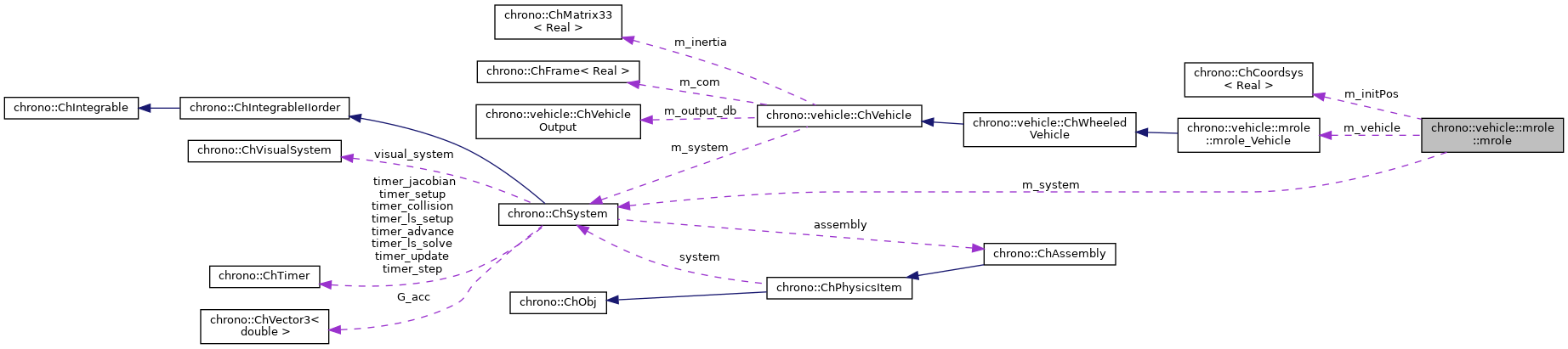
Public Types | |
enum | CTIS { ROAD, OFFROAD_SOIL, OFFROAD_SAND } |
Public Member Functions | |
void | SetContactMethod (ChContactMethod val) |
void | SetCollisionSystemType (ChCollisionSystem::Type collsys_type) |
void | SetChassisFixed (bool val) |
void | SetChassisCollisionType (CollisionType val) |
void | SetDriveType (DrivelineTypeWV val) |
void | SetBrakeType (BrakeType brake_type) |
void | SetEngineType (EngineModelType val) |
void | SetTransmissionType (TransmissionModelType val) |
void | SetTireType (TireModelType val) |
void | SetTireCollisionType (ChTire::CollisionType collision_type) |
void | SetInitPosition (const ChCoordsys<> &pos) |
void | SetInitFwdVel (double fwdVel) |
void | SetInitWheelAngVel (const std::vector< double > &omega) |
void | SetTireStepSize (double step_size) |
void | EnableBrakeLocking (bool lock) |
ChSystem * | GetSystem () const |
ChWheeledVehicle & | GetVehicle () const |
std::shared_ptr< ChChassis > | GetChassis () const |
std::shared_ptr< ChBodyAuxRef > | GetChassisBody () const |
void | Initialize () |
void | LockAxleDifferential (int axle, bool lock) |
void | LockCentralDifferential (int which, bool lock) |
void | SetAerodynamicDrag (double Cd, double area, double air_density) |
void | SetChassisVisualizationType (VisualizationType vis) |
void | SetSuspensionVisualizationType (VisualizationType vis) |
void | SetSteeringVisualizationType (VisualizationType vis) |
void | SetWheelVisualizationType (VisualizationType vis) |
void | SetTireVisualizationType (VisualizationType vis) |
void | Synchronize (double time, const DriverInputs &driver_inputs, const ChTerrain &terrain) |
void | Advance (double step) |
void | SelectRoadOperation () |
void | SelectOffroadSoilOperation () |
void | SelectOffroadSandOperation () |
double | GetMaxTireSpeed () |
Protected Member Functions | |
mrole (ChSystem *system) | |
virtual mrole_Vehicle * | CreateVehicle ()=0 |
Protected Attributes | |
CTIS | m_ctis |
ChContactMethod | m_contactMethod |
ChCollisionSystem::Type | m_collsysType |
CollisionType | m_chassisCollisionType |
bool | m_fixed |
bool | m_brake_locking |
DrivelineTypeWV | m_driveType |
EngineModelType | m_engineType |
TransmissionModelType | m_transmissionType |
BrakeType | m_brake_type |
TireModelType | m_tireType |
ChTire::CollisionType | m_tire_collision_type |
double | m_tire_step_size |
ChCoordsys | m_initPos |
double | m_initFwdVel |
std::vector< double > | m_initOmega |
bool | m_apply_drag |
double | m_Cd |
double | m_area |
double | m_air_density |
ChSystem * | m_system |
mrole_Vehicle * | m_vehicle |
double | m_tire_mass |
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_models/vehicle/mrole/mrole.h
- /builds/uwsbel/chrono/src/chrono_models/vehicle/mrole/mrole.cpp