Description
Base class for wheeled and tracked SynVehicleAgents. Both know about ChTerrains, ChDrivers and ChVehicleBrains.
#include <SynVehicleAgent.h>
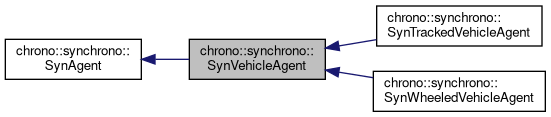
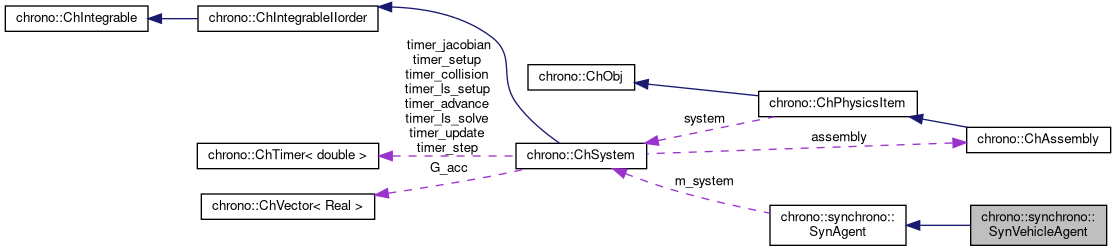
Public Member Functions | |
SynVehicleAgent (unsigned int rank, ChSystem *system=0) | |
Construct a vehicle agent with the specified rank and system Underlying agent is set to a vehicle type automatically. More... | |
virtual | ~SynVehicleAgent () |
Destructor. | |
virtual void | Synchronize (double time, vehicle::ChDriver::Inputs driver_inputs)=0 |
Synchronize the underlying vehicle. More... | |
virtual void | InitializeZombie (ChSystem *system=0) override |
Initialize the zombie representation of the underlying vehicle. More... | |
virtual void | SynchronizeZombie (SynMessage *message) override |
Synchronoize this agents zombie with the rest of the simulation. More... | |
virtual void | Advance (double time_of_next_sync) override |
Advance the state of this vehicle agent until agent time syncs with passed time. More... | |
virtual void | ProcessMessage (SynMessage *msg) override |
Process an incoming message. More... | |
virtual std::shared_ptr< SynVehicle > | GetVehicle ()=0 |
Get this agent's vehicle. | |
std::shared_ptr< SynTerrain > | GetTerrain () |
Get/Set the underlying terrain. | |
void | SetTerrain (std::shared_ptr< SynTerrain > terrain) |
std::shared_ptr< SynVehicleBrain > | GetBrain () |
Get/Set the underlying brain for this agent. | |
void | SetBrain (std::shared_ptr< SynVehicleBrain > brain) |
std::shared_ptr< vehicle::ChDriver > | GetDriver () |
Helper method to get the Chrono driver from the brain. | |
vehicle::ChVehicle & | GetChVehicle () |
Helper method to get the ChVehicle from the SynVehicle. | |
![]() | |
SynAgent (unsigned int rank, SynAgentType type, ChSystem *system=nullptr) | |
Construct a agent with the specified rank and type. More... | |
virtual | ~SynAgent () |
Destructor. | |
virtual std::shared_ptr< SynMessageState > | GetState ()=0 |
Get the state of this agent. More... | |
virtual std::shared_ptr< SynAgentMessage > | GetMessage ()=0 |
Get the this agent's message to be pass to other ranks. More... | |
virtual void | GenerateMessagesToSend (std::vector< SynMessage * > &messages)=0 |
Generates messages to be sent to other ranks Will create or get messages and pass them into the referenced message vector. More... | |
void | SetVisualizationManager (std::shared_ptr< SynVisualizationManager > vis_manager) |
Set the VisualizationManager for this agent. | |
std::shared_ptr< SynBrain > | GetBrain () |
Get/Set this agent's brain. | |
void | SetBrain (std::shared_ptr< SynBrain > brain) |
unsigned int | GetRank () |
Get/Set this agent's rank. | |
void | SetRank (unsigned int rank) |
ChSystem * | GetSystem () |
Get/Set the Chrono system associated with this agent. | |
void | SetSystem (ChSystem *system) |
double | GetStepSize () |
Get/Set this agent's step size. | |
void | SetStepSize (double step_size) |
SynAgentType | GetType () const |
Get the type of this agent. | |
Protected Member Functions | |
rapidjson::Document | ParseVehicleAgentFileJSON (const std::string &filename) |
Parse a vehicle agent json specification file. More... | |
void | VehicleAgentFromJSON (rapidjson::Document &d) |
Construct a VehicleAgent from the document. More... | |
Protected Attributes | |
std::shared_ptr< SynTerrain > | m_terrain |
handle to this agent's terrain | |
std::shared_ptr< SynVehicleBrain > | m_brain |
handle to this agent's brain | |
![]() | |
unsigned int | m_rank |
agent rank | |
SynAgentType | m_type |
agent type | |
double | m_step_size |
Step size of the underlying agent. | |
std::shared_ptr< SynBrain > | m_brain |
handle to this agent's brain | |
std::shared_ptr< SynVisualizationManager > | m_vis_manager |
handle to this agent's visualization manager | |
ChSystem * | m_system |
pointer to the Chrono system | |
Additional Inherited Members | |
![]() | |
static rapidjson::Document | ParseAgentFileJSON (const std::string &filename) |
Parse an agent json specification file. More... | |
Constructor & Destructor Documentation
◆ SynVehicleAgent()
chrono::synchrono::SynVehicleAgent::SynVehicleAgent | ( | unsigned int | rank, |
ChSystem * | system = 0 |
||
) |
Construct a vehicle agent with the specified rank and system Underlying agent is set to a vehicle type automatically.
Construct a vehicle agent with the specified rank and system Underlying agent is set to a vehicle type automatically
- Parameters
-
rank the rank of this agent system an optional argument of a ChSystem to build zombies and get the Time
Member Function Documentation
◆ Advance()
|
overridevirtual |
Advance the state of this vehicle agent until agent time syncs with passed time.
Advance the state of this agent until agent time syncs with passed time.
- Parameters
-
time_of_next_sync time at which this agent should stop advancing
Implements chrono::synchrono::SynAgent.
◆ InitializeZombie()
|
inlineoverridevirtual |
Initialize the zombie representation of the underlying vehicle.
Simply calls the same method on the underlying vehicle.
- Parameters
-
system the system used to add bodies to for consistent visualization
Implements chrono::synchrono::SynAgent.
◆ ParseVehicleAgentFileJSON()
|
protected |
Parse a vehicle agent json specification file.
- Parameters
-
filename the json specification file
- Returns
- rapidjson::Document the parsed rapidjson document
◆ ProcessMessage()
|
overridevirtual |
Process an incoming message.
Will pass the message directly to this agent's brain.
- Parameters
-
msg the received message to be processed
Reimplemented from chrono::synchrono::SynAgent.
◆ Synchronize()
|
pure virtual |
Synchronize the underlying vehicle.
- Parameters
-
time the time to synchronize to driver_inputs the driver inputs (i.e. throttle, braking, steering)
Implemented in chrono::synchrono::SynTrackedVehicleAgent, and chrono::synchrono::SynWheeledVehicleAgent.
◆ SynchronizeZombie()
|
inlineoverridevirtual |
Synchronoize this agents zombie with the rest of the simulation.
Updates agent based on specified message.
Synchronize the position and orientation of this vehicle with other ranks. Simply calls the same method on the underlying vehicle.
- Parameters
-
message the received message that describes the position and orientation.
Implements chrono::synchrono::SynAgent.
◆ VehicleAgentFromJSON()
|
protected |
Construct a VehicleAgent from the document.
- Parameters
-
d a rapidjson document that contains the required elements
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_synchrono/agent/SynVehicleAgent.h
- /builds/uwsbel/chrono/src/chrono_synchrono/agent/SynVehicleAgent.cpp