Description
Base class for all vehicle wrappers. Forces them to provide for initialization and synchronization of their zombie.
#include <SynVehicle.h>
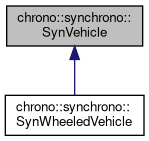
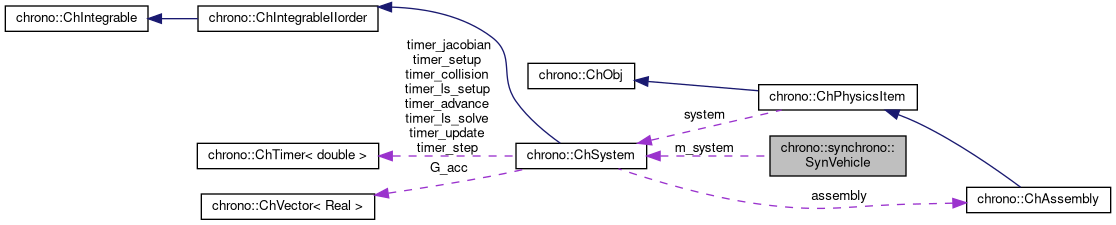
Public Member Functions | |
SynVehicle (bool is_zombie=true) | |
Default constructor for a SynVehicle. More... | |
SynVehicle (const std::string &filename, ChContactMethod contact_method) | |
Constructor for vehicles specified through json and a contact method This constructor will create it's own system. More... | |
SynVehicle (const std::string &filename, ChSystem *system) | |
Constructor for vehicles specified through json and a contact method This vehicle will use the passed system and not create its own. More... | |
SynVehicle (const std::string &filename) | |
Constructor for zombie vehicles specified through json. More... | |
virtual | ~SynVehicle () |
Destroy the SynVehicle object. | |
virtual void | InitializeZombie (ChSystem *system)=0 |
Initialize the zombie representation of the vehicle. More... | |
virtual void | SynchronizeZombie (SynMessage *message)=0 |
Synchronize the position and orientation of this vehicle with other ranks. More... | |
virtual void | Update ()=0 |
Update the current state of this vehicle. | |
bool | IsZombie () |
Get whether this vehicle is a zombie. More... | |
virtual void | Advance (double step) |
Advance the state of this vehicle by the time amount specified If the system is not owned by this vehicle, this method should be overriden. More... | |
virtual vehicle::ChVehicle & | GetVehicle ()=0 |
Get the ChVehicle being wrapped by this class. More... | |
ChSystem * | GetSystem () |
Get the ChSystem. More... | |
Protected Member Functions | |
virtual rapidjson::Document | ParseVehicleFileJSON (const std::string &filename) |
Parse a JSON specification file that describes a vehicle. More... | |
virtual void | CreateVehicle (const ChCoordsys<> &coord_sys, const std::string &filename, ChSystem *system)=0 |
Helper method for creating a vehicle from a json specification file. More... | |
virtual void | CreateZombie (const std::string &filename) |
Construct a zombie from a json specification file. More... | |
std::shared_ptr< ChTriangleMeshShape > | CreateMeshZombieComponent (const std::string &filename) |
Helper method used to create a ChTriangleMeshShape to be used on as a zombie body. More... | |
void | CreateChassisZombieBody (const std::string &filename, ChSystem *system) |
Create a zombie chassis body. More... | |
Protected Attributes | |
bool | m_is_zombie |
is this vehicle a zombie | |
bool | m_owns_vehicle |
has the vehicle been created by this object or passed by user | |
ChSystem * | m_system |
pointer to the chrono system | |
std::shared_ptr< ChBodyAuxRef > | m_zombie_body |
agent's zombie body reference | |
Constructor & Destructor Documentation
◆ SynVehicle() [1/4]
chrono::synchrono::SynVehicle::SynVehicle | ( | bool | is_zombie = true | ) |
Default constructor for a SynVehicle.
- Parameters
-
is_zombie whether this agent is a zombie
◆ SynVehicle() [2/4]
chrono::synchrono::SynVehicle::SynVehicle | ( | const std::string & | filename, |
ChContactMethod | contact_method | ||
) |
Constructor for vehicles specified through json and a contact method This constructor will create it's own system.
- Parameters
-
filename the json specification file to build the vehicle from contact_method the contact method used for the Chrono dynamics
◆ SynVehicle() [3/4]
chrono::synchrono::SynVehicle::SynVehicle | ( | const std::string & | filename, |
ChSystem * | system | ||
) |
Constructor for vehicles specified through json and a contact method This vehicle will use the passed system and not create its own.
- Parameters
-
filename the json specification file to build the vehicle from system the system to be used for Chrono dynamics and to add bodies to
◆ SynVehicle() [4/4]
chrono::synchrono::SynVehicle::SynVehicle | ( | const std::string & | filename | ) |
Constructor for zombie vehicles specified through json.
In order to visualize objects in the correct environment, no ChSystem is initialized
- Parameters
-
filename the json specification file to build the zombie from
Member Function Documentation
◆ Advance()
|
virtual |
Advance the state of this vehicle by the time amount specified If the system is not owned by this vehicle, this method should be overriden.
- Parameters
-
step the amount of time to advance by
◆ CreateChassisZombieBody()
|
protected |
Create a zombie chassis body.
All ChVehicles have a chassis, so this can be defined here
- Parameters
-
filename the filename that describes the ChTriangleMeshShape that should represent the chassis system the system to add the body to
◆ CreateMeshZombieComponent()
|
protected |
Helper method used to create a ChTriangleMeshShape to be used on as a zombie body.
- Parameters
-
filename the file to generate a ChTriangleMeshShape from
- Returns
- std::shared_ptr<ChTriangleMeshShape>
◆ CreateVehicle()
|
protectedpure virtual |
Helper method for creating a vehicle from a json specification file.
- Parameters
-
coord_sys the initial position and orientation of the vehicle filename the json specification file system a ChSystem to be used when constructing a vehicle
Implemented in chrono::synchrono::SynWheeledVehicle.
◆ CreateZombie()
|
inlineprotectedvirtual |
Construct a zombie from a json specification file.
- Parameters
-
filename the json specification file
◆ GetSystem()
◆ GetVehicle()
|
pure virtual |
Get the ChVehicle being wrapped by this class.
- Returns
- ChVehicle& the reference to the underlying ChVehicle
Implemented in chrono::synchrono::SynWheeledVehicle.
◆ InitializeZombie()
|
pure virtual |
Initialize the zombie representation of the vehicle.
Will create and add bodies that are visual representation of the vehicle. The position and orientation is updated by SynChrono and the passed messages
- Parameters
-
system the system used to add bodies to for consistent visualization
Implemented in chrono::synchrono::SynWheeledVehicle.
◆ IsZombie()
|
inline |
Get whether this vehicle is a zombie.
- Returns
- true it is a zombie
- false it is not a zombie
◆ ParseVehicleFileJSON()
|
protectedvirtual |
Parse a JSON specification file that describes a vehicle.
- Parameters
-
filename the json specification file
Reimplemented in chrono::synchrono::SynWheeledVehicle.
◆ SynchronizeZombie()
|
pure virtual |
Synchronize the position and orientation of this vehicle with other ranks.
Any message can be passed, so a check should be done to ensure this message was intended for this agent
- Parameters
-
message the received message that describes the position and orientation
Implemented in chrono::synchrono::SynWheeledVehicle.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_synchrono/vehicle/SynVehicle.h
- /builds/uwsbel/chrono/src/chrono_synchrono/vehicle/SynVehicle.cpp