Description
A triangle mesh with connectivity info: vertices can be shared between faces.
To keep this simple, the class assumes that you will manage the size of vectors m_vertices, m_normals etc., so ve cautious about resizing etc. We assume that
- if no m_face_uv_indices but m_UV.size() == m_vertices.size(), then m_UV represents per-vertex UV, otherwise per-face-corner
- if no m_face_col_indices but m_colors.size() == m_vertices.size(), then m_colors represents per-vertex colors, otherwise per-face-corner
#include <ChTriangleMeshConnected.h>
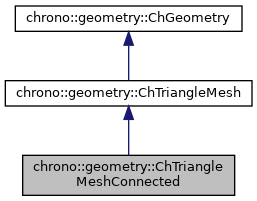
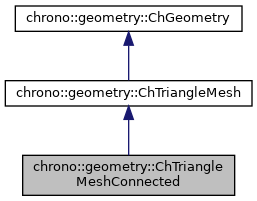
Classes | |
class | ChRefineEdgeCriterion |
Class to be used optionally in RefineMeshEdges(). More... | |
Public Member Functions | |
ChTriangleMeshConnected (const ChTriangleMeshConnected &source) | |
virtual ChTriangleMeshConnected * | Clone () const override |
"Virtual" copy constructor (covariant return type). | |
std::vector< ChVector< double > > & | getCoordsVertices () |
std::vector< ChVector< double > > & | getCoordsNormals () |
std::vector< ChVector2< double > > & | getCoordsUV () |
std::vector< ChColor > & | getCoordsColors () |
std::vector< ChVector< int > > & | getIndicesVertexes () |
std::vector< ChVector< int > > & | getIndicesNormals () |
std::vector< ChVector< int > > & | getIndicesUV () |
std::vector< ChVector< int > > & | getIndicesColors () |
std::vector< int > & | getIndicesMaterials () |
std::vector< ChProperty * > & | getPropertiesPerVertex () |
std::vector< ChProperty * > & | getPropertiesPerFace () |
const std::vector< ChVector< double > > & | getCoordsVertices () const |
const std::vector< ChVector< double > > & | getCoordsNormals () const |
const std::vector< ChVector2< double > > & | getCoordsUV () const |
const std::vector< ChColor > & | getCoordsColors () const |
const std::vector< ChVector< int > > & | getIndicesVertexes () const |
const std::vector< ChVector< int > > & | getIndicesNormals () const |
const std::vector< ChVector< int > > & | getIndicesUV () const |
const std::vector< ChVector< int > > & | getIndicesColors () const |
const std::vector< int > & | getIndicesMaterials () const |
void | AddPropertyPerVertex (ChProperty &mprop) |
Add a property as an array of data per-vertex. More... | |
void | AddPropertyPerFace (ChProperty &mprop) |
Add a property as an array of data per-face. More... | |
bool | LoadWavefrontMesh (const std::string &filename, bool load_normals=true, bool load_uv=false) |
Load a Wavefront OBJ file into this triangle mesh. | |
bool | LoadSTLMesh (const std::string &filename, bool load_normals=true) |
Load an STL file into this triangle mesh. | |
virtual void | addTriangle (const ChVector<> &vertex0, const ChVector<> &vertex1, const ChVector<> &vertex2) override |
Add a triangle to this triangle mesh, by specifying the three coordinates. More... | |
virtual void | addTriangle (const ChTriangle &atriangle) override |
Add a triangle to this triangle mesh, by specifying a ChTriangle. | |
int | getNumVertices () const |
Get the number of vertices in this mesh. | |
int | getNumNormals () const |
Get the number of normals in this mesh. | |
virtual int | getNumTriangles () const override |
Get the number of triangles already added to this mesh. | |
virtual ChTriangle | getTriangle (int index) const override |
Access the n-th triangle in mesh. | |
virtual void | Clear () override |
Clear all data. | |
virtual ChAABB | GetBoundingBox () const override |
Compute bounding box of this triangle mesh. | |
void | ComputeMassProperties (bool bodyCoords, double &mass, ChVector<> ¢er, ChMatrix33<> &inertia) |
Compute barycenter, mass, inertia tensor. | |
const std::string & | GetFileName () const |
Get the filename of the triangle mesh. | |
virtual void | Transform (const ChVector<> displ, const ChMatrix33<> rotscale) override |
Transform all vertexes, by displacing and rotating (rotation via matrix, so also scaling if needed). | |
bool | ComputeNeighbouringTriangleMap (std::vector< std::array< int, 4 >> &tri_map) const |
Create a map of neighboring triangles, vector [Ti TieA TieB TieC] (the free sides have triangle id = -1). More... | |
bool | ComputeWingedEdges (std::map< std::pair< int, int >, std::pair< int, int >> &winged_edges, bool allow_single_wing=true) const |
Create a winged edge structure, map of {key, value} as {{edgevertexA, edgevertexB}, {triangleA, triangleB}}. More... | |
int | RepairDuplicateVertexes (double tolerance=1e-18) |
Connect overlapping vertexes. More... | |
bool | MakeOffset (double offset) |
Offset the mesh, by a specified value, orthogonally to the faces. More... | |
std::pair< int, int > | GetTriangleEdgeIndexes (const ChVector< int > &face_indices, int nedge, bool unique) |
Return the indexes of the two vertexes of the i-th edge of the triangle. More... | |
bool | SplitEdge (int itA, int itB, int neA, int neB, int &itA_1, int &itA_2, int &itB_1, int &itB_2, std::vector< std::array< int, 4 >> &tri_map, std::vector< std::vector< double > * > &aux_data_double, std::vector< std::vector< int > * > &aux_data_int, std::vector< std::vector< bool > * > &aux_data_bool, std::vector< std::vector< ChVector<>> * > &aux_data_vect) |
Split a given edge by inserting a vertex in the middle: from two triangles one gets four triangles. More... | |
void | RefineMeshEdges (std::vector< int > &marked_tris, double edge_maxlen, ChRefineEdgeCriterion *criterion, std::vector< std::array< int, 4 >> *atri_map, std::vector< std::vector< double > * > &aux_data_double, std::vector< std::vector< int > * > &aux_data_int, std::vector< std::vector< bool > * > &aux_data_bool, std::vector< std::vector< ChVector<>> * > &aux_data_vect) |
Performs mesh refinement using Rivara LEPP long-edge bisection algorithm. More... | |
const std::vector< ChVector<> > & | getFaceVertices () |
const std::vector< ChVector<> > & | getFaceNormals () |
const std::vector< ChColor > & | getFaceColors () |
const std::vector< ChVector<> > & | getAverageNormals () |
virtual Type | GetClassType () const override |
Get the class type as an enum. | |
virtual void | ArchiveOut (ChArchiveOut &marchive) override |
Method to allow serialization of transient data to archives. | |
virtual void | ArchiveIn (ChArchiveIn &marchive) override |
Method to allow de-serialization of transient data from archives. | |
![]() | |
virtual void | Transform (const ChVector<> displ, const ChQuaternion<> mquat=ChQuaternion<>(1, 0, 0, 0)) |
Transform all vertexes, by displacing and rotating (rotation via matrix, so also scaling if needed) | |
virtual int | GetManifoldDimension () const override |
This is a surface. | |
![]() | |
ChGeometry (const ChGeometry &source) | |
void | InflateBoundingBox (ChAABB &bbox) const |
Enlarge the given existing bounding box with the bounding box of this object. | |
virtual double | GetBoundingSphereRadius () const |
Returns the radius of a bounding sphere for this geometry. More... | |
virtual ChVector | Baricenter () const |
Compute center of mass. | |
virtual void | Update () |
Generic update of internal data. | |
Static Public Member Functions | |
static std::shared_ptr< ChTriangleMeshConnected > | CreateFromWavefrontFile (const std::string &filename, bool load_normals=true, bool load_uv=false) |
Create and return a ChTriangleMeshConnected from a Wavefront OBJ file. More... | |
static std::shared_ptr< ChTriangleMeshConnected > | CreateFromSTLFile (const std::string &filename, bool load_normals=true) |
Create and return a ChTriangleMeshConnected from an STL file. More... | |
static void | WriteWavefront (const std::string &filename, const std::vector< ChTriangleMeshConnected > &meshes) |
Write the specified meshes in a Wavefront .obj file. | |
static ChTriangleMeshConnected | Merge (std::vector< ChTriangleMeshConnected > &meshes) |
Utility function for merging multiple meshes. | |
static ChAABB | GetBoundingBox (std::vector< ChVector<>> vertices) |
Return the bounding box of a triangle mesh with given vertices. | |
Public Attributes | |
std::vector< ChVector< double > > | m_vertices |
std::vector< ChVector< double > > | m_normals |
std::vector< ChVector2< double > > | m_UV |
std::vector< ChColor > | m_colors |
std::vector< ChVector< int > > | m_face_v_indices |
std::vector< ChVector< int > > | m_face_n_indices |
std::vector< ChVector< int > > | m_face_uv_indices |
std::vector< ChVector< int > > | m_face_col_indices |
std::vector< int > | m_face_mat_indices |
std::string | m_filename |
file string if loading an obj file | |
std::vector< ChProperty * > | m_properties_per_vertex |
std::vector< ChProperty * > | m_properties_per_face |
std::vector< ChVector<> > | m_tmp_vectors |
std::vector< ChColor > | m_tmp_colors |
Additional Inherited Members | |
![]() | |
enum | Type { NONE, SPHERE, ELLIPSOID, BOX, CYLINDER, TRIANGLE, CAPSULE, CONE, LINE, LINE_ARC, LINE_BEZIER, LINE_CAM, LINE_PATH, LINE_POLY, LINE_SEGMENT, ROUNDED_BOX, ROUNDED_CYLINDER, TRIANGLEMESH, TRIANGLEMESH_CONNECTED, TRIANGLEMESH_SOUP } |
Enumeration of geometric object types. | |
Member Function Documentation
◆ AddPropertyPerFace()
|
inline |
Add a property as an array of data per-face.
Deletion will be automatic at the end of mesh life. Warning: mprop.data.size() must be equal to m_vertices.size(). Cost: allocation a data copy.
◆ AddPropertyPerVertex()
|
inline |
Add a property as an array of data per-vertex.
Deletion will be automatic at the end of mesh life. Warning: mprop.data.size() must be equal to m_vertices.size(). Cost: allocation and a data copy.
◆ addTriangle()
|
overridevirtual |
Add a triangle to this triangle mesh, by specifying the three coordinates.
This is disconnected - no vertex sharing is used even if it could be.
Implements chrono::geometry::ChTriangleMesh.
◆ ComputeNeighbouringTriangleMap()
bool chrono::geometry::ChTriangleMeshConnected::ComputeNeighbouringTriangleMap | ( | std::vector< std::array< int, 4 >> & | tri_map | ) | const |
Create a map of neighboring triangles, vector [Ti TieA TieB TieC] (the free sides have triangle id = -1).
Return false if some edge has more than 2 neighboring triangles
◆ ComputeWingedEdges()
bool chrono::geometry::ChTriangleMeshConnected::ComputeWingedEdges | ( | std::map< std::pair< int, int >, std::pair< int, int >> & | winged_edges, |
bool | allow_single_wing = true |
||
) | const |
Create a winged edge structure, map of {key, value} as {{edgevertexA, edgevertexB}, {triangleA, triangleB}}.
If allow_single_wing = false, only edges with at least 2 triangles are returned. Else, also boundary edges with 1 triangle (the free side has triangle id = -1). Return false if some edge has more than 2 neighboring triangles.
◆ CreateFromSTLFile()
|
static |
Create and return a ChTriangleMeshConnected from an STL file.
If an error occurrs during loading, an empty shared pointer is returned.
◆ CreateFromWavefrontFile()
|
static |
Create and return a ChTriangleMeshConnected from a Wavefront OBJ file.
If an error occurrs during loading, an empty shared pointer is returned.
◆ GetTriangleEdgeIndexes()
std::pair< int, int > chrono::geometry::ChTriangleMeshConnected::GetTriangleEdgeIndexes | ( | const ChVector< int > & | face_indices, |
int | nedge, | ||
bool | unique | ||
) |
Return the indexes of the two vertexes of the i-th edge of the triangle.
If unique=true, swap the pair so that 1st < 2nd, to permit test sharing with other triangle.
- Parameters
-
face_indices indices of a triangular face nedge number of edge: 0, 1, 2 unique swap?
◆ MakeOffset()
bool chrono::geometry::ChTriangleMeshConnected::MakeOffset | ( | double | offset | ) |
Offset the mesh, by a specified value, orthogonally to the faces.
The offset can be inward or outward. Note: self-collisions and inverted faces resulting from excessive offsets are NOT trimmed; so this is mostly meant to be a fast tool for making small offsets.
◆ RefineMeshEdges()
void chrono::geometry::ChTriangleMeshConnected::RefineMeshEdges | ( | std::vector< int > & | marked_tris, |
double | edge_maxlen, | ||
ChRefineEdgeCriterion * | criterion, | ||
std::vector< std::array< int, 4 >> * | atri_map, | ||
std::vector< std::vector< double > * > & | aux_data_double, | ||
std::vector< std::vector< int > * > & | aux_data_int, | ||
std::vector< std::vector< bool > * > & | aux_data_bool, | ||
std::vector< std::vector< ChVector<>> * > & | aux_data_vect | ||
) |
Performs mesh refinement using Rivara LEPP long-edge bisection algorithm.
Given a conforming, non-degenerate triangulation, it construct a locally refined triangulation with a prescribed resolution. This algorithm, for increasing resolution, tends to produce triangles with bounded angles even if starting from skewed/skinny triangles in the coarse mesh. Based on "Multithread parallelization of Lepp-bisection algorithms" M.-C. Rivara et al., Applied Numerical Mathematics 62 (2012) 473�488 The auxiliary buffers are used for refinement and assumed to be indexed like the vertex buffer.
- Parameters
-
marked_tris indexes of triangles to refine (also surrounding triangles might be affected by refinements) edge_maxlen maximum length of edge (small values give higher resolution) criterion criterion for computing lenght (or other merit function) of edge, if null uses default (euclidean length) atri_map optional triangle connectivity map aux_data_double auxiliary buffer aux_data_int auxiliary buffer aux_data_bool auxiliary buffer aux_data_vect auxiliary buffer
◆ RepairDuplicateVertexes()
int chrono::geometry::ChTriangleMeshConnected::RepairDuplicateVertexes | ( | double | tolerance = 1e-18 | ) |
Connect overlapping vertexes.
This can beused to attempt to repair a mesh with 'open edges' to transform it into a watertight mesh. Say, if a cube is modeled with 6 faces with 4 distinct vertexes each, it might display properly, but for some algorithms, ex. collision detection, topological information might be needed, hence adjacent faces must be connected. Return the number of merged vertexes.
- Parameters
-
tolerance ignore vertexes closer than this value
◆ SplitEdge()
bool chrono::geometry::ChTriangleMeshConnected::SplitEdge | ( | int | itA, |
int | itB, | ||
int | neA, | ||
int | neB, | ||
int & | itA_1, | ||
int & | itA_2, | ||
int & | itB_1, | ||
int & | itB_2, | ||
std::vector< std::array< int, 4 >> & | tri_map, | ||
std::vector< std::vector< double > * > & | aux_data_double, | ||
std::vector< std::vector< int > * > & | aux_data_int, | ||
std::vector< std::vector< bool > * > & | aux_data_bool, | ||
std::vector< std::vector< ChVector<>> * > & | aux_data_vect | ||
) |
Split a given edge by inserting a vertex in the middle: from two triangles one gets four triangles.
It also interpolate normals, colors, uv. It also used and modifies the triangle neighboring map. If the two triangles do not share an edge, returns false. The auxiliary buffers are used for interpolation and assumed to be indexed like the vertex buffer.
- Parameters
-
itA triangle A index, itB triangle B index, -1 if not present (free edge on A) neA n.edge on tri A: 0,1,2 neB n.edge on tri B: 0,1,2 itA_1 returns the index of split triangle A, part1 itA_2 returns the index of split triangle A, part2 itB_1 returns the index of split triangle B, part1 itB_2 returns the index of split triangle B, part2 tri_map triangle neighbouring map aux_data_double auxiliary buffers aux_data_int auxiliary buffers aux_data_bool auxiliary buffers aux_data_vect auxiliary buffers
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/geometry/ChTriangleMeshConnected.h
- /builds/uwsbel/chrono/src/chrono/geometry/ChTriangleMeshConnected.cpp