Description
Isogeometric formulation (IGA) of a Cosserat rod, with large displacements, based on the Geometrically Exact Beam Theory.
User-defined order n (ex: 1=linear 2=quadratic, 3=cubic), where each element is a span of a b-spline, so each element uses n+1 control points, ie. nodes of chrono::fea::ChNodeFEAxyzrot type. As a thick beam, shear effects are possible, v. Timoshenko theory. Reduced integration to correct shear locking (*note, use order 1 for the moment, this must be improved) Initial curved configuration is supported. The section is defined in a modular way, via a chrono::fea::ChBeamSectionCosserat object that is composed via an elastic model, an inertial model, a damping (optional) model, a plastic (optional) model. Some of the ready-to-use implementation of those models allow a very generic beam where the center of mass, center of shear etc. are arbitrarily offset from the beam centerline, thus allowing the simulation of advanced cases like helicopter blades etc.
#include <ChElementBeamIGA.h>
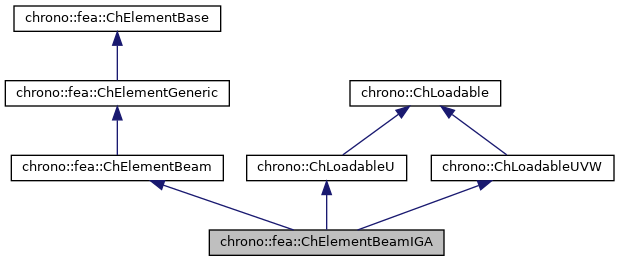
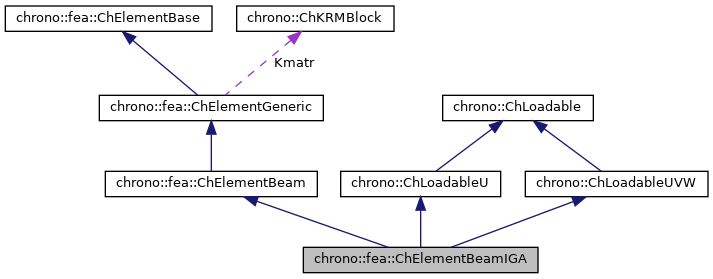
Public Types | |
enum | QuadratureType { FULL_OVER, FULL_EXACT, REDUCED, SELECTIVE, CUSTOM1, URI2 } |
For testing purposes: | |
Public Member Functions | |
ChElementBeamIGA (const ChElementBeamIGA &)=delete | |
ChElementBeamIGA & | operator= (const ChElementBeamIGA &)=delete |
virtual unsigned int | GetNumNodes () override |
Get the number of nodes used by this element. | |
virtual unsigned int | GetNumCoordsPosLevel () override |
Get the number of coordinates in the field used by the referenced nodes. More... | |
virtual unsigned int | GetNodeNumCoordsPosLevel (unsigned int n) override |
Get the number of coordinates from the specified node that are used by this element. More... | |
virtual std::shared_ptr< ChNodeFEAbase > | GetNode (unsigned int n) override |
Access the nth node. | |
virtual std::vector< std::shared_ptr< ChNodeFEAxyzrot > > & | GetNodes () |
virtual void | SetNodesCubic (std::shared_ptr< ChNodeFEAxyzrot > nodeA, std::shared_ptr< ChNodeFEAxyzrot > nodeB, std::shared_ptr< ChNodeFEAxyzrot > nodeC, std::shared_ptr< ChNodeFEAxyzrot > nodeD, double knotA1, double knotA2, double knotB1, double knotB2, double knotB3, double knotB4, double knotB5, double knotB6) |
virtual void | SetNodesGenericOrder (std::vector< std::shared_ptr< ChNodeFEAxyzrot >> mynodes, std::vector< double > myknots, int myorder) |
void | SetIntegrationPoints (int npoints_s, int npoints_b) |
Set the integration points, for shear components and for bending components: | |
void | SetSection (std::shared_ptr< ChBeamSectionCosserat > my_material) |
Set the section & material of beam element . More... | |
std::shared_ptr< ChBeamSectionCosserat > | GetSection () |
Get the section & material of the element. | |
ChVectorDynamic & | GetKnotSequence () |
Access the local knot sequence of this element (ex.for diagnostics) | |
double | GetU1 () |
Get the parametric coordinate at the beginning of the span. | |
double | GetU2 () |
Get the parametric coordinate at the end of the span. | |
virtual void | Update () override |
Update, called at least at each time step. More... | |
std::vector< std::unique_ptr< ChBeamMaterialInternalData > > & | GetPlasticData () |
Get the plastic data, in a vector with as many elements as Gauss points. | |
std::vector< ChVector3d > & | GetStressN () |
Get the stress, as cut-force [N], in a vector with as many elements as Gauss points. | |
std::vector< ChVector3d > & | GetStressM () |
Get the stress, as cut-torque [Nm], in a vector with as many elements as Gauss points. | |
std::vector< ChVector3d > & | GetStrainE () |
Get the strain (total=elastic+plastic), as deformation (x is axial strain), in a vector with as many elements as Gauss points. | |
std::vector< ChVector3d > & | GetStrainK () |
Get the strain (total=elastic+plastic), as curvature (x is torsion), in a vector with as many elements as Gauss points. | |
virtual void | GetStateBlock (ChVectorDynamic<> &mD) override |
Fills the D vector with the current field values at the nodes of the element, with proper ordering. More... | |
virtual void | ComputeNodalMass () override |
Add contribution of element inertia to total nodal masses. | |
virtual void | ComputeKRMmatricesGlobal (ChMatrixRef H, double Kfactor, double Rfactor=0, double Mfactor=0) override |
Sets H as the global stiffness matrix K, scaled by Kfactor. More... | |
virtual void | ComputeInternalForces (ChVectorDynamic<> &Fi) override |
Computes the internal forces (ex. More... | |
void | ComputeInternalForces_impl (ChVectorDynamic<> &Fi, ChState &state_x, ChStateDelta &state_w, bool used_for_differentiation=false) |
virtual void | ComputeGravityForces (ChVectorDynamic<> &Fg, const ChVector3d &G_acc) override |
Compute gravity forces, grouped in the Fg vector, one node after the other. | |
virtual void | EvaluateSectionDisplacement (const double eta, ChVector3d &u_displ, ChVector3d &u_rotaz) override |
Gets the xyz displacement of a point on the beam line, and the rotation RxRyRz of section plane, at abscyssa 'eta'. More... | |
virtual void | EvaluateSectionPoint (const double eta, ChVector3d &point) |
Gets the absolute xyz position of a point on the beam line, at abscissa 'eta'. More... | |
virtual void | EvaluateSectionFrame (const double eta, ChVector3d &point, ChQuaternion<> &rot) override |
Gets the absolute xyz position of a point on the beam line, and the absolute rotation of section plane, at abscissa 'eta'. More... | |
virtual void | EvaluateSectionForceTorque (const double eta, ChVector3d &Fforce, ChVector3d &Mtorque) override |
Gets the force (traction x, shear y, shear z) and the torque (torsion on x, bending on y, on bending on z) at a section along the beam line, at abscissa 'eta'. More... | |
virtual void | EvaluateSectionStrain (const double eta, ChVector3d &StrainV) override |
Gets the axial and bending strain of the ANCF "cable" element. | |
virtual void | EleDoIntegration () override |
This is optionally implemented if there is some internal state that requires integration. | |
virtual unsigned int | GetLoadableNumCoordsPosLevel () override |
Gets the number of DOFs affected by this element (position part) | |
virtual unsigned int | GetLoadableNumCoordsVelLevel () override |
Gets the number of DOFs affected by this element (speed part) | |
virtual void | LoadableGetStateBlockPosLevel (int block_offset, ChState &mD) override |
Gets all the DOFs packed in a single vector (position part) | |
virtual void | LoadableGetStateBlockVelLevel (int block_offset, ChStateDelta &mD) override |
Gets all the DOFs packed in a single vector (speed part) | |
virtual void | LoadableStateIncrement (const unsigned int off_x, ChState &x_new, const ChState &x, const unsigned int off_v, const ChStateDelta &Dv) override |
Increment all DOFs using a delta. | |
virtual unsigned int | GetNumFieldCoords () override |
Number of coordinates in the interpolated field, ex=3 for a tetrahedron finite element or a cable, = 1 for a thermal problem, etc. | |
virtual unsigned int | GetNumSubBlocks () override |
Get the number of DOFs sub-blocks. | |
virtual unsigned int | GetSubBlockOffset (unsigned int nblock) override |
Get the offset of the specified sub-block of DOFs in global vector. | |
virtual unsigned int | GetSubBlockSize (unsigned int nblock) override |
Get the size of the specified sub-block of DOFs in global vector. | |
virtual bool | IsSubBlockActive (unsigned int nblock) const override |
Check if the specified sub-block of DOFs is active. | |
virtual void | LoadableGetVariables (std::vector< ChVariables * > &mvars) override |
Get the pointers to the contained ChVariables, appending to the mvars vector. | |
virtual void | ComputeNF (const double U, ChVectorDynamic<> &Qi, double &detJ, const ChVectorDynamic<> &F, ChVectorDynamic<> *state_x, ChVectorDynamic<> *state_w) override |
Evaluate N'*F , where N is some type of shape function evaluated at U coordinates of the line, ranging in -1..+1 F is a load, N'*F is the resulting generalized load Returns also det[J] with J=[dx/du,..], that might be useful in gauss quadrature. More... | |
virtual void | ComputeNF (const double U, const double V, const double W, ChVectorDynamic<> &Qi, double &detJ, const ChVectorDynamic<> &F, ChVectorDynamic<> *state_x, ChVectorDynamic<> *state_w) override |
Evaluate N'*F , where N is some type of shape function evaluated at U,V,W coordinates of the volume, each ranging in -1..+1 F is a load, N'*F is the resulting generalized load Returns also det[J] with J=[dx/du,..], that might be useful in gauss quadrature. More... | |
virtual double | GetDensity () override |
This is needed so that it can be accessed by ChLoaderVolumeGravity. | |
![]() | |
double | GetMass () |
The full mass of the beam, (with const. section, density, etc.) | |
double | GetRestLength () |
The rest length of the bar. | |
void | SetRestLength (double ml) |
Set the rest length of the bar (usually this should be automatically done when SetupInitial is called on beams element, given the current state, but one might need to override this, ex for precompressed beams etc). | |
![]() | |
ChKRMBlock & | Kstiffness () |
Access the proxy to stiffness, for sparse solver. | |
virtual void | EleIntLoadResidual_F (ChVectorDynamic<> &R, const double c) override |
Add the internal forces (pasted at global nodes offsets) into a global vector R, multiplied by a scaling factor c, as R += forces * c This default implementation is SLIGHTLY INEFFICIENT. | |
virtual void | EleIntLoadResidual_Mv (ChVectorDynamic<> &R, const ChVectorDynamic<> &w, const double c) override |
Add the product of element mass M by a vector w (pasted at global nodes offsets) into a global vector R, multiplied by a scaling factor c, as R += M * w * c This default implementation is VERY INEFFICIENT. | |
virtual void | EleIntLoadLumpedMass_Md (ChVectorDynamic<> &Md, double &error, const double c) override |
Adds the lumped mass to a Md vector, representing a mass diagonal matrix. More... | |
virtual void | EleIntLoadResidual_F_gravity (ChVectorDynamic<> &R, const ChVector3d &G_acc, const double c) override |
Add the contribution of gravity loads, multiplied by a scaling factor c, as: R += M * g * c This default implementation is VERY INEFFICIENT. More... | |
virtual void | ComputeMmatrixGlobal (ChMatrixRef M) override |
Calculate the mass matrix, expressed in global reference. More... | |
virtual void | InjectKRMMatrices (ChSystemDescriptor &descriptor) override |
Register with the given system descriptor any ChKRMBlock objects associated with this item. | |
virtual void | LoadKRMMatrices (double Kfactor, double Rfactor, double Mfactor) override |
Compute and load current stiffnes (K), damping (R), and mass (M) matrices in encapsulated ChKRMBlock objects. More... | |
virtual void | VariablesFbLoadInternalForces (double factor=1.) override |
Add the internal forces, expressed as nodal forces, into the encapsulated ChVariables. | |
virtual void | VariablesFbIncrementMq () override |
Add M*q (internal masses multiplied current 'qb'). | |
![]() | |
virtual unsigned int | GetNumCoordsPosLevelActive () |
Get the actual number of active degrees of freedom. More... | |
virtual unsigned int | GetNodeNumCoordsPosLevelActive (unsigned int n) |
Get the actual number of active coordinates from the specified node that are used by this element. More... | |
![]() | |
virtual bool | IsTetrahedronIntegrationNeeded () |
If true, use quadrature over u,v,w in [0..1] range as tetrahedron volumetric coords (with z=1-u-v-w) otherwise use default quadrature over u,v,w in [-1..+1] as box isoparametric coords. | |
virtual bool | IsTrianglePrismIntegrationNeeded () |
If true, use quadrature over u,v in [0..1] range as triangle natural coords (with z=1-u-v), and use linear quadrature over w in [-1..+1], otherwise use default quadrature over u,v,w in [-1..+1] as box isoparametric coords. | |
Static Public Attributes | |
static QuadratureType | quadrature_type = ChElementBeamIGA::QuadratureType::FULL_EXACT |
For testing purposes: | |
static double | Delta = 1e-10 |
For testing purposes: | |
static bool | lumped_mass = true |
Set if the element mass matrix is computed in lumped or consistent way. | |
static bool | add_gyroscopic_terms = true |
Set if the element forces will include the gyroscopic and centrifugal terms (slower performance, but might be needed esp. More... | |
Friends | |
class | ChExtruderBeamIGA |
Additional Inherited Members | |
![]() | |
double | mass |
double | length |
![]() | |
ChKRMBlock | Kmatr |
Member Function Documentation
◆ ComputeInternalForces()
|
overridevirtual |
Computes the internal forces (ex.
the actual position of nodes is not in relaxed reference position) and set values in the Fi vector.
Implements chrono::fea::ChElementBase.
◆ ComputeInternalForces_impl()
void chrono::fea::ChElementBeamIGA::ComputeInternalForces_impl | ( | ChVectorDynamic<> & | Fi, |
ChState & | state_x, | ||
ChStateDelta & | state_w, | ||
bool | used_for_differentiation = false |
||
) |
< here return N and dN/du
< here return N and dN/du
- Parameters
-
Fi output vector of internal forces state_x state position to evaluate Fi state_w state speed to evaluate Fi used_for_differentiation used during FD Jacobian evaluation?
◆ ComputeKRMmatricesGlobal()
|
overridevirtual |
Sets H as the global stiffness matrix K, scaled by Kfactor.
Optionally, also superimposes global damping matrix R, scaled by Rfactor, and global mass matrix M multiplied by Mfactor.
Implements chrono::fea::ChElementBase.
◆ ComputeNF() [1/2]
|
overridevirtual |
Evaluate N'*F , where N is some type of shape function evaluated at U coordinates of the line, ranging in -1..+1 F is a load, N'*F is the resulting generalized load Returns also det[J] with J=[dx/du,..], that might be useful in gauss quadrature.
< h
- Parameters
-
U eta parametric coordinate in line -1..+1 Qi Return result of Q = N'*F here detJ Return det[J] here F Input F vector, size is =n. field coords. state_x if != 0, update state (pos. part) to this, then evaluate Q state_w if != 0, update state (speed part) to this, then evaluate Q
Implements chrono::ChLoadableU.
◆ ComputeNF() [2/2]
|
overridevirtual |
Evaluate N'*F , where N is some type of shape function evaluated at U,V,W coordinates of the volume, each ranging in -1..+1 F is a load, N'*F is the resulting generalized load Returns also det[J] with J=[dx/du,..], that might be useful in gauss quadrature.
- Parameters
-
U parametric coordinate in volume V parametric coordinate in volume W parametric coordinate in volume Qi Return result of N'*F here, maybe with offset block_offset detJ Return det[J] here F Input F vector, size is = n.field coords. state_x if != 0, update state (pos. part) to this, then evaluate Q state_w if != 0, update state (speed part) to this, then evaluate Q
Implements chrono::ChLoadableUVW.
◆ EvaluateSectionDisplacement()
|
inlineoverridevirtual |
Gets the xyz displacement of a point on the beam line, and the rotation RxRyRz of section plane, at abscyssa 'eta'.
Note, eta=-1 at node1, eta=+1 at node2. Note, 'displ' is the displ.state of 2 nodes, ex. get it as GetStateBlock() Results are not corotated.
Implements chrono::fea::ChElementBeam.
◆ EvaluateSectionForceTorque()
|
inlineoverridevirtual |
Gets the force (traction x, shear y, shear z) and the torque (torsion on x, bending on y, on bending on z) at a section along the beam line, at abscissa 'eta'.
Note, eta=-1 at node1, eta=+1 at node2.
Implements chrono::fea::ChElementBeam.
◆ EvaluateSectionFrame()
|
inlineoverridevirtual |
Gets the absolute xyz position of a point on the beam line, and the absolute rotation of section plane, at abscissa 'eta'.
Note, eta=-1 at node1, eta=+1 at node2.
Implements chrono::fea::ChElementBeam.
◆ EvaluateSectionPoint()
|
inlinevirtual |
Gets the absolute xyz position of a point on the beam line, at abscissa 'eta'.
Note, eta=-1 at node1, eta=+1 at node2.
< here return in N
◆ GetNodeNumCoordsPosLevel()
|
inlineoverridevirtual |
Get the number of coordinates from the specified node that are used by this element.
Note that this may be different from the value returned by GetNode(n)->GetNumCoordsVelLevel().
Implements chrono::fea::ChElementBase.
◆ GetNumCoordsPosLevel()
|
inlineoverridevirtual |
Get the number of coordinates in the field used by the referenced nodes.
This is for example the size (number of rows/columns) of the local stiffness matrix.
Implements chrono::fea::ChElementBase.
◆ GetStateBlock()
|
inlineoverridevirtual |
Fills the D vector with the current field values at the nodes of the element, with proper ordering.
If the D vector has not the size of this->GetNumCoordsPosLevel(), it will be resized.
Implements chrono::fea::ChElementBase.
◆ SetSection()
|
inline |
Set the section & material of beam element .
It is a shared property, so it can be shared between other beams.
◆ Update()
|
inlineoverridevirtual |
Update, called at least at each time step.
If the element has to keep updated some auxiliary data, such as the rotation matrices for corotational approach, this should be implemented in this function.
Reimplemented from chrono::fea::ChElementBase.
Member Data Documentation
◆ add_gyroscopic_terms
|
static |
Set if the element forces will include the gyroscopic and centrifugal terms (slower performance, but might be needed esp.
when center of mass is offset)
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono/fea/ChElementBeamIGA.h
- /builds/uwsbel/chrono/src/chrono/fea/ChElementBeamIGA.cpp