chrono::vehicle::sedan::Sedan_DoubleWishbone Class Reference
Description
Double wishbone suspension model for a sedan vehicle (can be used in front or rear).
#include <Sedan_DoubleWishbone.h>
Inheritance diagram for chrono::vehicle::sedan::Sedan_DoubleWishbone:
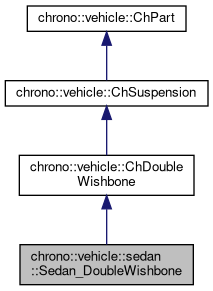
Collaboration diagram for chrono::vehicle::sedan::Sedan_DoubleWishbone:
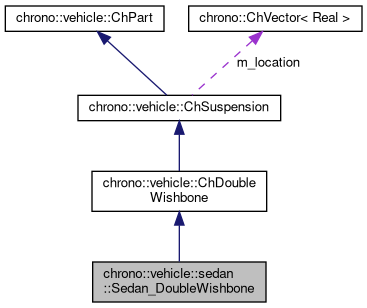
Public Member Functions | |
Sedan_DoubleWishbone (const std::string &name) | |
virtual const ChVector | getLocation (PointId which) override |
Return the location of the specified hardpoint. More... | |
virtual double | getSpindleMass () const override |
Return the mass of the spindle body. | |
virtual double | getUCAMass () const override |
Return the mass of the upper control arm body. | |
virtual double | getLCAMass () const override |
Return the mass of the lower control body. | |
virtual double | getUprightMass () const override |
Return the mass of the upright body. | |
virtual double | getSpindleRadius () const override |
Return the radius of the spindle body (visualization only). | |
virtual double | getSpindleWidth () const override |
Return the width of the spindle body (visualization only). | |
virtual double | getUCARadius () const override |
Return the radius of the upper control arm body (visualization only). | |
virtual double | getLCARadius () const override |
Return the radius of the lower control arm body (visualization only). | |
virtual double | getUprightRadius () const override |
Return the radius of the upright body (visualization only). | |
virtual const ChVector & | getSpindleInertia () const override |
Return the moments of inertia of the spindle body. | |
virtual const ChVector & | getUCAInertiaMoments () const override |
Return the moments of inertia of the upper control arm body. | |
virtual const ChVector & | getUCAInertiaProducts () const override |
Return the products of inertia of the upper control arm body. | |
virtual const ChVector & | getLCAInertiaMoments () const override |
Return the moments of inertia of the lower control arm body. | |
virtual const ChVector & | getLCAInertiaProducts () const override |
Return the products of inertia of the lower control arm body. | |
virtual const ChVector & | getUprightInertiaMoments () const override |
Return the moments of inertia of the upright body. | |
virtual const ChVector & | getUprightInertiaProducts () const override |
Return the products of inertia of the upright body. | |
virtual double | getAxleInertia () const override |
Return the inertia of the axle shaft. | |
virtual double | getSpringRestLength () const override |
Return the free (rest) length of the spring element. | |
virtual ChLinkTSDA::ForceFunctor * | getSpringForceFunctor () const override |
Return the functor object for spring force. | |
virtual ChLinkTSDA::ForceFunctor * | getShockForceFunctor () const override |
Return the functor object for shock force. | |
![]() | |
virtual std::string | GetTemplateName () const override |
Get the name of the vehicle subsystem template. | |
virtual bool | IsSteerable () const final override |
Specify whether or not this suspension can be steered. | |
virtual bool | IsIndependent () const final override |
Specify whether or not this is an independent suspension. | |
virtual void | Initialize (std::shared_ptr< ChBodyAuxRef > chassis, const ChVector<> &location, std::shared_ptr< ChBody > tierod_body, int steering_index, double left_ang_vel=0, double right_ang_vel=0) override |
Initialize this suspension subsystem. More... | |
virtual void | AddVisualizationAssets (VisualizationType vis) override |
Add visualization assets for the suspension subsystem. More... | |
virtual void | RemoveVisualizationAssets () override |
Remove visualization assets for the suspension subsystem. | |
virtual double | GetMass () const override |
Get the total mass of the suspension subsystem. | |
virtual ChVector | GetCOMPos () const override |
Get the current global COM location of the suspension subsystem. | |
virtual double | GetTrack () override |
Get the wheel track for the suspension subsystem. | |
std::shared_ptr< ChLinkTSDA > | GetSpring (VehicleSide side) const |
Get a handle to the specified spring element. | |
std::shared_ptr< ChLinkTSDA > | GetShock (VehicleSide side) const |
Get a handle to the specified shock (damper) element. | |
virtual ChSuspension::Force | ReportSuspensionForce (VehicleSide side) const override |
Return current suspension forces (spring and shock) on the specified side. | |
double | GetSpringForce (VehicleSide side) const |
Get the force in the spring element. | |
double | GetSpringLength (VehicleSide side) const |
Get the current length of the spring element. | |
double | GetSpringDeformation (VehicleSide side) const |
Get the current deformation of the spring element. | |
double | GetShockForce (VehicleSide side) const |
Get the force in the shock (damper) element. | |
double | GetShockLength (VehicleSide side) const |
Get the current length of the shock (damper) element. | |
double | GetShockVelocity (VehicleSide side) const |
Get the current deformation velocity of the shock (damper) element. | |
ChVector | Get_LCA_sph_pos (VehicleSide side) |
Global coordinates, LCA ball joint position. | |
ChVector | Get_UCA_sph_pos (VehicleSide side) |
Global coordinates, UCA ball joint position. | |
virtual void | LogConstraintViolations (VehicleSide side) override |
Log current constraint violations. | |
virtual std::shared_ptr< ChBody > | GetLeftBody () const override |
Specify the left body for a possible antirollbar subsystem. More... | |
virtual std::shared_ptr< ChBody > | GetRightBody () const override |
Specify the right body for a possible antirollbar subsystem. More... | |
void | LogHardpointLocations (const ChVector<> &ref, bool inches=false) |
Log the locations of all hardpoints. More... | |
![]() | |
ChSuspension (const std::string &name) | |
const ChVector & | GetLocation () const |
Get the location of the suspension subsystem relative to the chassis reference frame. More... | |
std::shared_ptr< ChBody > | GetSpindle (VehicleSide side) const |
Get a handle to the spindle body on the specified side. | |
std::shared_ptr< ChShaft > | GetAxle (VehicleSide side) const |
Get a handle to the axle shaft on the specified side. | |
std::shared_ptr< ChLinkLockRevolute > | GetRevolute (VehicleSide side) const |
Get a handle to the revolute joint on the specified side. | |
const ChVector & | GetSpindlePos (VehicleSide side) const |
Get the global location of the spindle on the specified side. | |
const ChQuaternion & | GetSpindleRot (VehicleSide side) const |
Get the orientation of the spindle body on the specified side. More... | |
const ChVector & | GetSpindleLinVel (VehicleSide side) const |
Get the linear velocity of the spindle body on the specified side. More... | |
ChVector | GetSpindleAngVel (VehicleSide side) const |
Get the angular velocity of the spindle body on the specified side. More... | |
double | GetAxleSpeed (VehicleSide side) const |
Get the angular speed of the axle on the specified side. | |
int | GetSteeringIndex () const |
Get the index of the associated steering index (-1 if non-steered). | |
void | Synchronize () |
Synchronize this suspension subsystem. More... | |
void | ApplyAxleTorque (VehicleSide side, double torque) |
Apply the provided motor torque. More... | |
![]() | |
ChPart (const std::string &name) | |
Construct a vehicle subsystem with the specified name. More... | |
const std::string & | GetName () const |
Get the name identifier for this subsystem. | |
void | SetName (const std::string &name) |
Set the name identifier for this subsystem. | |
void | SetVisualizationType (VisualizationType vis) |
Set the visualization mode for this subsystem. | |
void | SetContactFrictionCoefficient (float friction_coefficient) |
Set coefficient of friction. More... | |
void | SetContactRestitutionCoefficient (float restitution_coefficient) |
Set coefficient of restitution. More... | |
void | SetContactMaterialProperties (float young_modulus, float poisson_ratio) |
Set contact material properties. More... | |
void | SetContactMaterialCoefficients (float kn, float gn, float kt, float gt) |
Set contact material coefficients. More... | |
float | GetCoefficientFriction () const |
Get coefficient of friction for contact material. | |
float | GetCoefficientRestitution () const |
Get coefficient of restitution for contact material. | |
float | GetYoungModulus () const |
Get Young's modulus of elasticity for contact material. | |
float | GetPoissonRatio () const |
Get Poisson ratio for contact material. | |
float | GetKn () const |
Get normal stiffness coefficient for contact material. | |
float | GetKt () const |
Get tangential stiffness coefficient for contact material. | |
float | GetGn () const |
Get normal viscous damping coefficient for contact material. | |
float | GetGt () const |
Get tangential viscous damping coefficient for contact material. | |
virtual void | SetOutput (bool state) |
Enable/disable output for this subsystem. | |
bool | OutputEnabled () const |
Return the output state for this subsystem. | |
Additional Inherited Members | |
![]() | |
static ChMatrix33 | TransformInertiaMatrix (const ChVector<> &moments, const ChVector<> &products, const ChMatrix33<> &vehicle_rot, const ChMatrix33<> &body_rot) |
Utility function for transforming inertia tensors between centroidal frames. More... | |
![]() | |
enum | PointId { SPINDLE, UPRIGHT, UCA_F, UCA_B, UCA_U, UCA_CM, LCA_F, LCA_B, LCA_U, LCA_CM, SHOCK_C, SHOCK_A, SPRING_C, SPRING_A, TIEROD_C, TIEROD_U, NUM_POINTS } |
Identifiers for the various hardpoints. More... | |
![]() | |
ChDoubleWishbone (const std::string &name, bool vehicle_frame_inertia=false) | |
Protected constructor. More... | |
void | SetVehicleFrameInertiaFlag (bool val) |
Indicate whether or not inertia matrices are specified with respect to a vehicle-aligned centroidal frame (flag=true) or with respect to the body centroidal frame (flag=false). More... | |
![]() | |
virtual void | Create (const rapidjson::Document &d) |
Create a vehicle subsystem from JSON data. More... | |
![]() | |
static void | ExportBodyList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChBody >> bodies) |
Export the list of bodies to the specified JSON document. | |
static void | ExportShaftList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaft >> shafts) |
Export the list of shafts to the specified JSON document. | |
static void | ExportJointList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLink >> joints) |
Export the list of joints to the specified JSON document. | |
static void | ExportCouplesList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChShaftsCouple >> couples) |
Export the list of shaft couples to the specified JSON document. | |
static void | ExportMarkerList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChMarker >> markers) |
Export the list of markers to the specified JSON document. | |
static void | ExportLinSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkTSDA >> springs) |
Export the list of translational springs to the specified JSON document. | |
static void | ExportRotSpringList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLinkRotSpringCB >> springs) |
Export the list of rotational springs to the specified JSON document. | |
static void | ExportBodyLoadList (rapidjson::Document &jsonDocument, std::vector< std::shared_ptr< ChLoadBodyBody >> loads) |
Export the list of body-body loads to the specified JSON document. | |
![]() | |
std::shared_ptr< ChBody > | m_upright [2] |
handles to the upright bodies (left/right) | |
std::shared_ptr< ChBody > | m_UCA [2] |
handles to the upper control arm bodies (left/right) | |
std::shared_ptr< ChBody > | m_LCA [2] |
handles to the lower control arm bodies (left/right) | |
std::shared_ptr< ChLinkLockRevolute > | m_revoluteUCA [2] |
handles to the chassis-UCA revolute joints (left/right) | |
std::shared_ptr< ChLinkLockSpherical > | m_sphericalUCA [2] |
handles to the upright-UCA spherical joints (left/right) | |
std::shared_ptr< ChLinkLockRevolute > | m_revoluteLCA [2] |
handles to the chassis-LCA revolute joints (left/right) | |
std::shared_ptr< ChLinkLockSpherical > | m_sphericalLCA [2] |
handles to the upright-LCA spherical joints (left/right) | |
std::shared_ptr< ChLinkDistance > | m_distTierod [2] |
handles to the tierod distance constraints (left/right) | |
std::shared_ptr< ChLinkTSDA > | m_shock [2] |
handles to the spring links (left/right) | |
std::shared_ptr< ChLinkTSDA > | m_spring [2] |
handles to the shock links (left/right) | |
![]() | |
ChVector | m_location |
location relative to chassis | |
int | m_steering_index |
index of associated steering mechanism | |
std::shared_ptr< ChBody > | m_spindle [2] |
handles to spindle bodies | |
std::shared_ptr< ChShaft > | m_axle [2] |
handles to axle shafts | |
std::shared_ptr< ChShaftsBody > | m_axle_to_spindle [2] |
handles to spindle-shaft connectors | |
std::shared_ptr< ChLinkLockRevolute > | m_revolute [2] |
handles to spindle revolute joints | |
![]() | |
std::string | m_name |
subsystem name | |
bool | m_output |
specifies whether or not output is generated for this subsystem | |
float | m_friction |
contact coefficient of friction | |
float | m_restitution |
contact coefficient of restitution | |
float | m_young_modulus |
contact material Young modulus | |
float | m_poisson_ratio |
contact material Poisson ratio | |
float | m_kn |
normal contact stiffness | |
float | m_gn |
normal contact damping | |
float | m_kt |
tangential contact stiffness | |
float | m_gt |
tangential contact damping | |
Member Function Documentation
◆ getLocation()
|
overridevirtual |
Return the location of the specified hardpoint.
The returned location must be expressed in the suspension reference frame.
Implements chrono::vehicle::ChDoubleWishbone.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_models/vehicle/sedan/Sedan_DoubleWishbone.h
- /builds/uwsbel/chrono/src/chrono_models/vehicle/sedan/Sedan_DoubleWishbone.cpp