Description
Continuum representation SPH deformable terrain model.
#include <SPHTerrain.h>
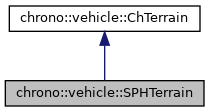
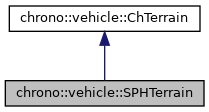
Public Member Functions | |
SPHTerrain (ChSystem &sys, double spacing) | |
Create a granular SPH terrain object. More... | |
void | SetVerbose (bool verbose) |
Enable verbose output during construction of SPHTerrain (default: false). | |
fsi::ChSystemFsi & | GetSystemFSI () |
Access the underlying FSI system. | |
void | AddRigidObstacle (const std::string &obj_file, double scale, double density, const ChContactMaterialData &cmat, const ChFrame<> &pos, const ChVector<> &interior_point=ChVector<>(0)) |
Add a rigid obstacle. More... | |
void | Construct (const std::string &sph_file, const std::string &bce_file, const ChVector<> &pos=ChVector<>(0), double yaw_angle=0) |
Construct a granular SPH terrain object using information from the specified files. More... | |
void | Construct (double length, double width, double depth, int bce_layers=3, const ChVector<> &pos=ChVector<>(0), double yaw_angle=0, bool side_walls=true) |
Construct a rectangular patch granular SPH terrain object of given dimensions. More... | |
void | Construct (const std::string &heightmap_file, double length, double width, const ChVector2<> &height_range, double depth, int bce_layers=3, const ChVector<> &pos=ChVector<>(0), double yaw_angle=0, bool side_walls=true) |
Construct a granular SPH terrain object from a given heightmap. More... | |
void | Initialize () |
Initialize the terrain. More... | |
std::shared_ptr< ChBody > | GetGroundBody () const |
Get the ground body. | |
void | GetAABB (ChVector<> &aabb_min, ChVector<> &aabb_max) const |
Get the AABB of terrain SPH particles. | |
void | SaveMarkers (const std::string &out_dir) const |
Save the set of SPH and BCE grid locations to the files in the specified output directory. | |
virtual void | Synchronize (double time) override |
Update the state of the terrain system at the specified time. | |
virtual double | GetHeight (const ChVector<> &loc) const override |
Get the terrain height below the specified location. | |
virtual chrono::ChVector | GetNormal (const ChVector<> &loc) const override |
Get the terrain normal at the point below the specified location. | |
virtual float | GetCoefficientFriction (const ChVector<> &loc) const override |
Get the terrain coefficient of friction at the point below the specified location. More... | |
![]() | |
virtual void | Advance (double step) |
Advance the state of the terrain system by the specified duration. | |
virtual void | GetProperties (const ChVector<> &loc, double &height, ChVector<> &normal, float &friction) const |
Get all terrain characteristics at the point below the specified location. | |
void | RegisterHeightFunctor (std::shared_ptr< HeightFunctor > functor) |
Specify the functor object to provide the terrain height at given locations. More... | |
void | RegisterNormalFunctor (std::shared_ptr< NormalFunctor > functor) |
Specify the functor object to provide the terrain normal at given locations. More... | |
void | RegisterFrictionFunctor (std::shared_ptr< FrictionFunctor > functor) |
Specify the functor object to provide the coefficient of friction at given locations. | |
Additional Inherited Members | |
![]() | |
std::shared_ptr< HeightFunctor > | m_height_fun |
functor for location-dependent terrain height | |
std::shared_ptr< NormalFunctor > | m_normal_fun |
functor for location-dependent terrain normal | |
std::shared_ptr< FrictionFunctor > | m_friction_fun |
functor for location-dependent coefficient of friction | |
Constructor & Destructor Documentation
◆ SPHTerrain()
chrono::vehicle::SPHTerrain::SPHTerrain | ( | ChSystem & | sys, |
double | spacing | ||
) |
Create a granular SPH terrain object.
No SPH parameters are set.
Member Function Documentation
◆ AddRigidObstacle()
void chrono::vehicle::SPHTerrain::AddRigidObstacle | ( | const std::string & | obj_file, |
double | scale, | ||
double | density, | ||
const ChContactMaterialData & | cmat, | ||
const ChFrame<> & | pos, | ||
const ChVector<> & | interior_point = ChVector<>(0) |
||
) |
Add a rigid obstacle.
A rigid body with visualization and collision geometry read from the Wavefront OBJ file is created at the specified position and with the specified orientation. BCE markers are created with the separation value specified at construction. Must be called before Initialize().
- Parameters
-
obj_file Wavefront OBJ file name scale scale factor density material density cmat contact material properties pos obstacle position in absolute frame interior_point point inside obstacle volume
◆ Construct() [1/3]
void chrono::vehicle::SPHTerrain::Construct | ( | const std::string & | heightmap_file, |
double | length, | ||
double | width, | ||
const ChVector2<> & | height_range, | ||
double | depth, | ||
int | bce_layers = 3 , |
||
const ChVector<> & | pos = ChVector<>(0) , |
||
double | yaw_angle = 0 , |
||
bool | side_walls = true |
||
) |
Construct a granular SPH terrain object from a given heightmap.
The image file is read with STB, using the number of channels defined in the input file and reading the image as 16-bit (8-bit images are automatically converted). Supported image formats: JPEG, PNG, BMP, GIF, PSD, PIC, PNM. Create the SPH particle grid locations for a terrain patch of specified X and Y dimensions with optional translation and rotation. The height at each grid point is obtained through bilinear interpolation from the gray values in the provided heightmap image (with pure black corresponding to the lower height range and pure white to the upper height range). SPH particle grid locations are generated to cover the specified depth under each grid point. BCE marker layers are created below the bottom-most layer of SPH particles and (optionally) on the sides of the terrain patch.
- Parameters
-
heightmap_file filename for the heightmap image length patch length (X direction) width patch width (Y direction) height_range height range (black to white level) depth soil depth bce_layers number of BCE layers pos patch center yaw_angle patch yaw rotation side_walls create side boundaries
◆ Construct() [2/3]
void chrono::vehicle::SPHTerrain::Construct | ( | const std::string & | sph_file, |
const std::string & | bce_file, | ||
const ChVector<> & | pos = ChVector<>(0) , |
||
double | yaw_angle = 0 |
||
) |
Construct a granular SPH terrain object using information from the specified files.
The SPH particle and BCE marker locations are assumed to be provided on an integer grid. Locations in real space are generated using the specified grid separation value and the terrain patch translated and rotated aboutn the vertical.
- Parameters
-
sph_file filename with SPH grid particle positions bce_file filename with BCE grid marker positions pos patch center yaw_angle patch yaw rotation
◆ Construct() [3/3]
void chrono::vehicle::SPHTerrain::Construct | ( | double | length, |
double | width, | ||
double | depth, | ||
int | bce_layers = 3 , |
||
const ChVector<> & | pos = ChVector<>(0) , |
||
double | yaw_angle = 0 , |
||
bool | side_walls = true |
||
) |
Construct a rectangular patch granular SPH terrain object of given dimensions.
- Parameters
-
length patch length (X direction) width patch width (Y direction) depth patch depth (Z direction) bce_layers number of BCE layers pos patch center yaw_angle patch yaw rotation side_walls create side boundaries
◆ GetCoefficientFriction()
|
inlineoverridevirtual |
Get the terrain coefficient of friction at the point below the specified location.
This coefficient of friction value may be used by certain tire models to modify the tire characteristics, but it will have no effect on the interaction of the terrain with other objects (including tire models that do not explicitly use it).
Reimplemented from chrono::vehicle::ChTerrain.
◆ Initialize()
void chrono::vehicle::SPHTerrain::Initialize | ( | ) |
Initialize the terrain.
After this call, no additional solid bodies should be added to the FSI problem.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/SPHTerrain.h
- /builds/uwsbel/chrono/src/chrono_vehicle/terrain/SPHTerrain.cpp