Description
Definition of a suspension test rig with direct actuation on the spindle bodies.
#include <ChSuspensionTestRig.h>
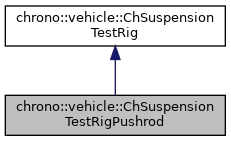
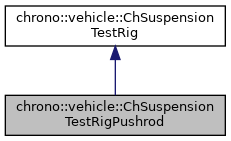
Public Member Functions | |
ChSuspensionTestRigPushrod (std::shared_ptr< ChWheeledVehicle > vehicle, std::vector< int > axle_index, double displ_limit) | |
Construct a test rig for specified sets of axles and sterring mechanisms of a given vehicle. More... | |
ChSuspensionTestRigPushrod (const std::string &spec_filename) | |
Construct a test rig from the given JSON specification file. More... | |
virtual double | GetActuatorDisp (int axle, VehicleSide side) override |
Return the current post displacement for the specified axle and vehicle side. | |
virtual double | GetActuatorForce (int axle, VehicleSide side) override |
Return the current post actuation force for the specified axle and vehicle side. / TODO: not yet implemented. | |
virtual double | GetRideHeight (int axle) const override |
Get current ride height (relative to the chassis reference frame). More... | |
![]() | |
virtual | ~ChSuspensionTestRig () |
Destructor. | |
void | SetDriver (std::shared_ptr< ChSuspensionTestRigDriver > driver) |
Set driver system. | |
void | SetInitialRideHeight (double height) |
Set the initial ride height (relative to the chassis reference frame). More... | |
void | SetDisplacementLimit (double limit) |
Set the limits for post displacement (same for jounce and rebound). | |
void | IncludeSteeringMechanism (int index) |
Include the specified steering mechanism in testing. | |
void | IncludeSubchassis (int index) |
Include the specified subchassis in testing. | |
void | SetSuspensionVisualizationType (VisualizationType vis) |
Set visualization type for the suspension subsystem (default: PRIMITIVES). | |
void | SetSteeringVisualizationType (VisualizationType vis) |
Set visualization type for the steering subsystems (default: PRIMITIVES). | |
void | SetSubchassisVisualizationType (VisualizationType vis) |
Set visualization type for the subchassis subsystems (default: PRIMITIVES). | |
void | SetWheelVisualizationType (VisualizationType vis) |
Set visualization type for the wheel subsystems (default: NONE). | |
void | SetTireVisualizationType (VisualizationType vis) |
Set visualization type for the tire subsystems (default: PRIMITIVES). | |
void | Initialize () |
Initialize the suspension test rig. More... | |
void | Advance (double step) |
Advance the state of the suspension test rig by the specified time step. | |
ChWheeledVehicle & | GetVehicle () const |
Get a handle to the underlying vehicle system. | |
const ChVector3d & | GetSpindlePos (int axle, VehicleSide side) const |
Get the global location of the specified spindle. More... | |
ChQuaternion | GetSpindleRot (int axle, VehicleSide side) const |
Get the global rotation of the specified spindle. More... | |
const ChVector3d & | GetSpindleLinVel (int axle, VehicleSide side) const |
Get the linear velocity of the specified spindle (expressed in the global reference frame). More... | |
ChVector3d | GetSpindleAngVel (int axle, VehicleSide side) const |
Get the angular velocity of the specified spindle (expressed in the global reference frame). More... | |
double | GetSteeringInput () const |
Return current driver steering input. | |
double | GetWheelTravel (int axle, VehicleSide side) const |
Get wheel travel, defined here as the vertical displacement of the spindle center from its position at the initial specified ride height (or initial configuration, if an initial ride height is not specified). More... | |
std::string | GetDriverMessage () const |
Return the info message from the STR driver. | |
bool | DriverEnded () const |
Return true when driver stopped producing inputs. | |
void | LogConstraintViolations () |
Log current constraint violations. | |
void | SetOutput (ChVehicleOutput::Type type, const std::string &out_dir, const std::string &out_name, double output_step) |
Enable output for this vehicle system (default: false). More... | |
void | SetPlotOutput (double output_step) |
Enable data collection for plot generation (default: false). More... | |
void | PlotOutput (const std::string &out_dir, const std::string &out_name) |
Plot collected data. More... | |
Additional Inherited Members | |
![]() | |
ChSuspensionTestRig (std::shared_ptr< ChWheeledVehicle > vehicle, std::vector< int > axle_index, double displ_limit) | |
Construct a test rig for specified sets of axles and sterring mechanisms of a given vehicle. More... | |
ChSuspensionTestRig (const std::string &spec_filename) | |
Construct a test rig from the given JSON specification file. More... | |
void | CollectPlotData (double time) |
Collect data for plot output. | |
![]() | |
std::shared_ptr< ChWheeledVehicle > | m_vehicle |
associated vehicle | |
int | m_naxles |
number of tested axles | |
std::vector< int > | m_axle_index |
list of tested vehicle axles in rig | |
std::vector< int > | m_steering_index |
list of tested vehicle steering mechanisms in rig | |
std::vector< int > | m_subchassis_index |
list of tested vehicle subchassis mechanisms in rig | |
double | m_ride_height |
ride height | |
double | m_displ_limit |
scale factor for post displacement | |
std::vector< double > | m_displ_offset |
post displacement offsets (to set reference positions) | |
Constructor & Destructor Documentation
◆ ChSuspensionTestRigPushrod() [1/2]
chrono::vehicle::ChSuspensionTestRigPushrod::ChSuspensionTestRigPushrod | ( | std::shared_ptr< ChWheeledVehicle > | vehicle, |
std::vector< int > | axle_index, | ||
double | displ_limit | ||
) |
Construct a test rig for specified sets of axles and sterring mechanisms of a given vehicle.
- Parameters
-
vehicle test vehicle axle_index list of tested vehicle axles displ_limit displacement limits
◆ ChSuspensionTestRigPushrod() [2/2]
chrono::vehicle::ChSuspensionTestRigPushrod::ChSuspensionTestRigPushrod | ( | const std::string & | spec_filename | ) |
Construct a test rig from the given JSON specification file.
An STR specification file includes the JSON specification for the underlying vehicle, the sets of tested vehicle subsystems, and the displacement limit for the rig posts.
Member Function Documentation
◆ GetRideHeight()
|
overridevirtual |
Get current ride height (relative to the chassis reference frame).
This estimate uses the average of the left and right posts. Note: 'axle' is the index in the set of tested axles.
Implements chrono::vehicle::ChSuspensionTestRig.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/test_rig/ChSuspensionTestRig.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/test_rig/ChSuspensionTestRig.cpp