Description
Interactive driver for a suspension test rig.
This class implements support for keyboard control of a suspension test rig, but is independent of a particular implementation of a keyboard event handler.
#include <ChSuspensionTestRigInteractiveDriver.h>
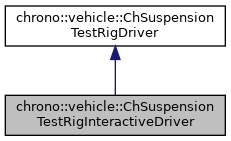
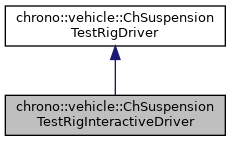
Public Member Functions | |
int | GetActiveAxle () const |
Get the currently actuated axle. | |
double | GetLeft () |
Get the left post displacement for current actuated axle. | |
double | GetRight () |
Get the right post displacement for current actuated axle. | |
void | NextAxle () |
Change current actuated axle to next (cycle as needed). | |
void | PreviousAxle () |
Change current actuated axle to previous (cycle as needed). | |
void | IncrementSteering () |
Increment steering input. | |
void | DecrementSteering () |
Decrement steering input. | |
void | IncrementLeft () |
Increment displacement of left post for current actuated axle. | |
void | DecrementLeft () |
Decrement displacement of left post for current actuated axle. | |
void | IncrementRight () |
Increment displacement of right post for current actuated axle. | |
void | DecrementRight () |
Decrement displacement of right post for current actuated axle. | |
void | SetDisplacementDelta (double delta) |
Set the time response for post displacement control. More... | |
void | SetSteeringDelta (double delta) |
Set the time response for steering control. More... | |
![]() | |
double | GetSteering () const |
Get the driver steering input (in the range [-1,+1]). | |
void | SetSteering (double val, double min_val=-1, double max_val=1) |
Set the value for the driver steering input. | |
const std::vector< double > & | GetDisplacementLeft () const |
Get all left post vertical displacement inputs (each in the range [-1,+1]). | |
void | SetDisplacementLeft (int axle, double val, double min_val=-1, double max_val=1) |
Set the value for the driver left post displacement input. | |
const std::vector< double > & | GetDisplacementRight () const |
Get all right post vertical displacement inputs (each in the range [-1,+1]). | |
void | SetDisplacementRight (int axle, double val, double min_val=-1, double max_val=1) |
Set the value for the driver right post displacement input. | |
const std::vector< double > & | GetDisplacementSpeedLeft () const |
Get the left post displacement rates of change. | |
const std::vector< double > & | GetDisplacementSpeedRight () const |
Get the right post displacement rates of change. | |
bool | Started () const |
Return false while driver inputs are ignored (while the rig is reaching the ride height configuration) and true otherwise. More... | |
virtual bool | Ended () const |
Return true when driver stopped producing inputs. | |
bool | LogInit (const std::string &filename) |
Initialize output file for recording driver inputs. | |
bool | Log (double time) |
Record the current driver inputs to the log file. | |
Additional Inherited Members | |
![]() | |
virtual void | Initialize (int num_axles) |
Initialize this driver system. | |
virtual void | Synchronize (double time) |
Update the state of this driver system at the current time. | |
![]() | |
int | m_naxles |
number of actuated axles | |
std::vector< double > | m_displLeft |
current value of left post displacements | |
std::vector< double > | m_displRight |
current value of right post displacements | |
std::vector< double > | m_displSpeedLeft |
current value of left post displacement rates of change | |
std::vector< double > | m_displSpeedRight |
current value of right post displacement rates of change | |
double | m_steering |
current value of steering input | |
double | m_delay |
time delay before generating inputs | |
Member Function Documentation
◆ SetDisplacementDelta()
|
inline |
Set the time response for post displacement control.
This value represents the time (in seconds) for changing the displacement input from 0 to 1 (or 0 to -1). Default: 1/100.
◆ SetSteeringDelta()
|
inline |
Set the time response for steering control.
This value represents the time (in seconds) for changing the steering input from 0 to 1 (or 0 to -1). Default: 1/250.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/test_rig/ChSuspensionTestRigInteractiveDriver.h
- /builds/uwsbel/chrono/src/chrono_vehicle/wheeled_vehicle/test_rig/ChSuspensionTestRigInteractiveDriver.cpp