Description
Concrete path-following steering PID controller with multiple path support.
#include <SynMultiPathDriver.h>
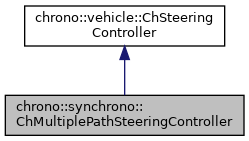
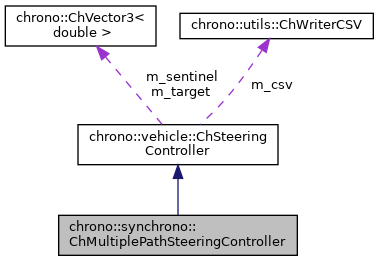
Public Member Functions | |
ChMultiplePathSteeringController (std::vector< std::shared_ptr< ChBezierCurve >> paths, int lane=0) | |
Construct a steering controller to track the specified path. More... | |
~ChMultiplePathSteeringController () | |
Destructor for ChPathSteeringController. | |
void | SetGains (double Kp, double Ki, double Kd) |
Set the gains for the PID controller. | |
std::vector< std::shared_ptr< ChBezierCurve > > | GetPath () const |
Return a pointer to the Bezier curve. | |
void | changePath (int target_lane) |
Change the path to the index of the desire path. | |
unsigned | addPath (std::shared_ptr< ChBezierCurve > path) |
Add another path to the steering Controller, will return the path index in the array. | |
virtual void | Reset (const ChFrameMoving<> &ref_frame) override |
Reset the PID controller. More... | |
virtual void | CalcTargetLocation () override |
Calculate the current target point location. More... | |
virtual double | Advance (const ChFrameMoving<> &ref_frame, double time, double step) override |
Advance the state of the PID controller. More... | |
![]() | |
virtual | ~ChSteeringController () |
Destructor. | |
void | SetLookAheadDistance (double dist) |
Specify the look-ahead distance. More... | |
const ChVector3d & | GetSentinelLocation () const |
Return the current location of the sentinel point. More... | |
const ChVector3d & | GetTargetLocation () const |
Return the current value of the target point. More... | |
std::shared_ptr< ChBezierCurve > | GetPath () const |
Return a pointer to the Bezier curve. | |
void | StartDataCollection () |
Start/restart data collection. | |
void | StopDataCollection () |
Suspend/stop data collection. | |
bool | IsDataCollectionEnabled () const |
Return true if data is being collected. | |
bool | IsDataAvailable () const |
Return true if data is available for output. | |
void | WriteOutputFile (const std::string &filename) |
Output data collected so far to the specified file. | |
Additional Inherited Members | |
![]() | |
ChSteeringController (std::shared_ptr< ChBezierCurve > path) | |
Construct a steering controller with default parameters. | |
![]() | |
std::shared_ptr< ChBezierCurve > | m_path |
tracked path (piecewise cubic Bezier curve) | |
double | m_dist |
look-ahead distance | |
ChVector3d | m_sentinel |
position of sentinel point in global frame | |
ChVector3d | m_target |
position of target point in global frame | |
double | m_err |
current error (signed distance to target point) | |
double | m_errd |
error derivative | |
double | m_erri |
integral of error | |
utils::ChWriterCSV * | m_csv |
ChWriterCSV object for data collection. | |
bool | m_collect |
flag indicating whether or not data is being collected | |
Constructor & Destructor Documentation
◆ ChMultiplePathSteeringController()
chrono::synchrono::ChMultiplePathSteeringController::ChMultiplePathSteeringController | ( | std::vector< std::shared_ptr< ChBezierCurve >> | paths, |
int | lane = 0 |
||
) |
Construct a steering controller to track the specified path.
This version uses default controller parameters (zero gains). The user is responsible for calling SetGains and SetLookAheadDistance.
Member Function Documentation
◆ Advance()
|
overridevirtual |
Advance the state of the PID controller.
This function performs the required integration for the integral component of the PID controller and returns the calculated steering value.
Implements chrono::vehicle::ChSteeringController.
◆ CalcTargetLocation()
|
overridevirtual |
Calculate the current target point location.
The target point is the point on the associated path that is closest to the current location of the sentinel point.
Implements chrono::vehicle::ChSteeringController.
◆ Reset()
|
overridevirtual |
Reset the PID controller.
This function resets the underlying path tracker using the current location of the sentinel point.
Reimplemented from chrono::vehicle::ChSteeringController.
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_synchrono/controller/driver/SynMultiPathDriver.h
- /builds/uwsbel/chrono/src/chrono_synchrono/controller/driver/SynMultiPathDriver.cpp