Description
Class to set up a Chrono::FSI problem using particles and markers on a Cartesian coordinates grid.
#include <ChFsiProblemSPH.h>
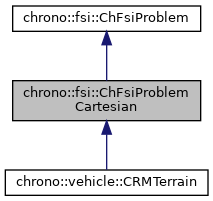
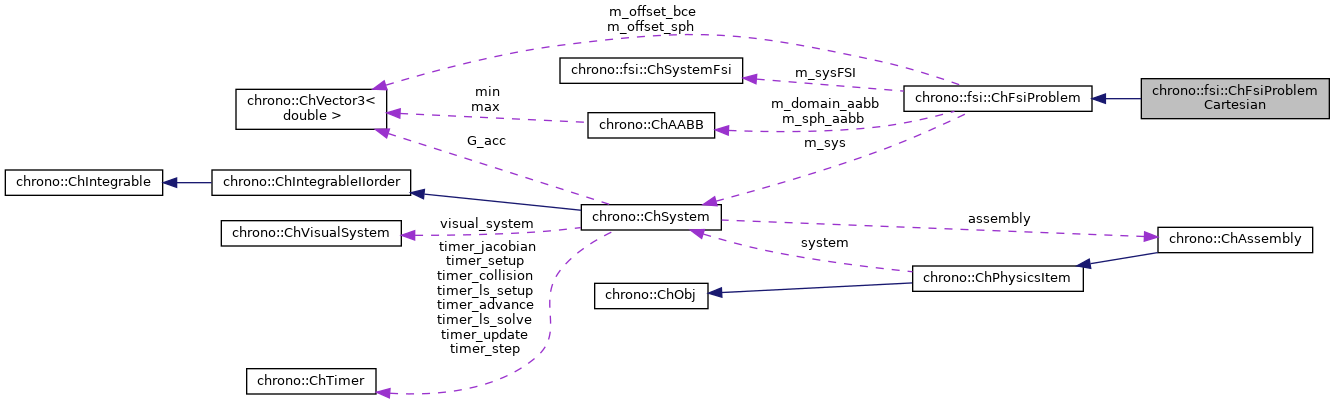
Classes | |
class | WaveTankProfile |
Interface for callback to specify wave tank profile. More... | |
Public Member Functions | |
ChFsiProblemCartesian (ChSystem &sys, double spacing) | |
Create a ChFsiProblemSPH object. More... | |
void | Construct (const std::string &sph_file, const std::string &bce_file, const ChVector3d &pos) |
Construct using information from the specified files. More... | |
void | Construct (const ChVector3d &box_size, const ChVector3d &pos, int side_flags) |
Construct SPH particles and optionally BCE markers in a box of given dimensions. More... | |
void | Construct (const std::string &heightmap_file, double length, double width, const ChVector2d &height_range, double depth, bool uniform_depth, const ChVector3d &pos, int side_flags) |
Construct SPH particles and optionally BCE markers from a given heightmap. More... | |
size_t | AddBoxContainer (const ChVector3d &box_size, const ChVector3d &pos, int side_flags) |
Add fixed BCE markers, representing a container for the computational domain. More... | |
std::shared_ptr< ChBody > | ConstructWaveTank (WavemakerType type, const ChVector3d &pos, const ChVector3d &box_size, double depth, std::shared_ptr< ChFunction > piston_fun, std::shared_ptr< WaveTankProfile > profile=nullptr, bool end_wall=true) |
Add a wave tank with a rigid-body wavemaker (piston-type or flap-type). More... | |
![]() | |
void | SetVerbose (bool verbose) |
Enable verbose output during construction of ChFsiProblemSPH (default: false). | |
ChFsiSystemSPH & | GetSystemFSI () |
Access the underlying FSI system. | |
ChFluidSystemSPH & | GetFluidSystemSPH () |
Access the underlying SPH system. | |
ChSystem & | GetMultibodySystem () |
Access the underlying MBS system. | |
void | SetCfdSPH (const ChFluidSystemSPH::FluidProperties &fluid_props) |
Enable solution of a CFD problem. | |
void | SetElasticSPH (const ChFluidSystemSPH::ElasticMaterialProperties &mat_props) |
Enable solution of elastic SPH (for continuum representation of granular dynamics). More... | |
void | SetSPHParameters (const ChFluidSystemSPH::SPHParameters &sph_params) |
Set SPH method parameters. | |
size_t | AddRigidBody (std::shared_ptr< ChBody > body, const chrono::utils::ChBodyGeometry &geometry, bool check_embedded, bool use_grid_bce=false) |
Add a rigid body to the FSI problem. More... | |
size_t | AddRigidBodySphere (std::shared_ptr< ChBody > body, const ChVector3d &pos, double radius, bool use_grid_bce=false) |
size_t | AddRigidBodyBox (std::shared_ptr< ChBody > body, const ChFramed &pos, const ChVector3d &size) |
size_t | AddRigidBodyCylinderX (std::shared_ptr< ChBody > body, const ChFramed &pos, double radius, double length, bool use_grid_bce=false) |
size_t | AddRigidBodyMesh (std::shared_ptr< ChBody > body, const ChVector3d &pos, const std::string &obj_file, const ChVector3d &interior_point, double scale) |
void | SetBcePattern1D (BcePatternMesh1D pattern, bool remove_center=false) |
Set the BCE marker pattern for 1D flexible solids for subsequent calls to AddFeaMesh. More... | |
void | SetBcePattern2D (BcePatternMesh2D pattern, bool remove_center=false) |
Set the BCE marker pattern for 2D flexible solids for subsequent calls to AddFeaMesh. More... | |
size_t | AddFeaMesh (std::shared_ptr< fea::ChMesh > mesh, bool check_embedded) |
Add an FEA mesh to the FSI problem. More... | |
void | RegisterParticlePropertiesCallback (std::shared_ptr< ParticlePropertiesCallback > callback) |
Register a callback for setting SPH particle initial properties. | |
void | SetGravitationalAcceleration (const ChVector3d &gravity) |
Set gravitational acceleration for both multibody and fluid systems. | |
void | SetStepSizeCFD (double step) |
Set integration step size for fluid dynamics. | |
void | SetStepsizeMBD (double step) |
Set integration step size for multibody dynamics. More... | |
void | DisableMBD () |
Disable automatic integration of the associated multibody system. More... | |
void | SetComputationalDomainSize (ChAABB aabb) |
Explicitly set the computational domain limits. More... | |
void | Initialize () |
Complete construction of the FSI problem and initialize the FSI system. More... | |
void | DoStepDynamics (double step) |
Advance the dynamics of the underlying FSI system by the specified step. | |
std::shared_ptr< ChBody > | GetGroundBody () const |
Get the ground body. | |
size_t | GetNumSPHParticles () const |
Get number of SPH particles. | |
size_t | GetNumBoundaryBCEMarkers () const |
Get number of boundary BCE markers. | |
const ChAABB & | GetComputationalDomainSize () const |
Get limits of computational domain. | |
const ChAABB & | GetSPHBoundingBox () const |
Get limits of SPH volume. | |
const ChVector3d & | GetFsiBodyForce (std::shared_ptr< ChBody > body) const |
Return the FSI applied force on the specified body (as returned by AddRigidBody). More... | |
const ChVector3d & | GetFsiBodyTorque (std::shared_ptr< ChBody > body) const |
Return the FSI applied torque on on the specified body (as returned by AddRigidBody). More... | |
double | GetRtfCFD () const |
Get current estimated RTF (real time factor) for the fluid system. | |
double | GetRtfMBD () const |
Get current estimated RTF (real time factor) for the multibody system. | |
void | SetOutputLevel (OutputLevel output_level) |
Set SPH simulation data output level (default: STATE_PRESSURE). More... | |
void | SaveOutputData (double time, const std::string &sph_dir, const std::string &fsi_dir) |
Save current SPH and solid data to files. More... | |
void | SaveInitialMarkers (const std::string &out_dir) const |
Save the set of initial SPH and BCE grid locations to files in the specified output directory. | |
PhysicsProblem | GetPhysicsProblem () const |
std::string | GetPhysicsProblemString () const |
std::string | GetSphMethodTypeString () const |
Additional Inherited Members | |
![]() | |
enum | WavemakerType { PISTON, FLAP } |
Wave generator types. | |
![]() | |
typedef std::unordered_set< ChVector3i, CoordHash > | GridPoints |
typedef std::vector< ChVector3d > | RealPoints |
![]() | |
ChFsiProblemSPH (ChSystem &sys, double spacing) | |
Create a ChFsiProblemSPH object. More... | |
void | ProcessBody (RigidBody &b) |
Prune SPH markers that are inside the solid body volume. More... | |
int | ProcessBodyMesh (RigidBody &b, ChTriangleMeshConnected trimesh, const ChVector3d &interior_point) |
Prune SPH markers that are inside a body mesh volume. More... | |
![]() | |
ChFluidSystemSPH | m_sysSPH |
underlying Chrono SPH system | |
ChFsiSystemSPH | m_sysFSI |
underlying Chrono FSI system | |
double | m_spacing |
particle and marker spacing | |
std::shared_ptr< ChBody > | m_ground |
ground body | |
GridPoints | m_sph |
SPH particle grid locations. | |
GridPoints | m_bce |
boundary BCE marker grid locations | |
ChVector3d | m_offset_sph |
SPH particles offset. | |
ChVector3d | m_offset_bce |
boundary BCE particles offset | |
ChAABB | m_domain_aabb |
computational domain bounding box | |
ChAABB | m_sph_aabb |
SPH volume bounding box. | |
std::vector< RigidBody > | m_bodies |
list of FSI rigid bodies | |
std::vector< FeaMesh > | m_meshes |
list of FSI FEA meshes | |
std::unordered_map< std::shared_ptr< ChBody >, size_t > | m_fsi_bodies |
std::shared_ptr< ParticlePropertiesCallback > | m_props_cb |
callback for particle properties | |
bool | m_verbose |
if true, write information to standard output | |
bool | m_initialized |
set to 'true' once terrain is initialized | |
Constructor & Destructor Documentation
◆ ChFsiProblemCartesian()
chrono::fsi::ChFsiProblemCartesian::ChFsiProblemCartesian | ( | ChSystem & | sys, |
double | spacing | ||
) |
Create a ChFsiProblemSPH object.
No SPH parameters are set.
Member Function Documentation
◆ AddBoxContainer()
size_t chrono::fsi::ChFsiProblemCartesian::AddBoxContainer | ( | const ChVector3d & | box_size, |
const ChVector3d & | pos, | ||
int | side_flags | ||
) |
Add fixed BCE markers, representing a container for the computational domain.
The specified 'box_size' represents the dimensions of the interior of the box. The reference position is the center of the bottom face of the box. Boundary BCE markers are created outside this volume, in layers, starting at a distance equal to the spacing. 'side_flags' are boolean combinations of BoxSide enums. It is the caller responsibility to ensure that the container BCE markers do not overlap with any SPH particles.
- Parameters
-
box_size box dimensions pos reference positions side_flags sides for which BCE markers are created
◆ Construct() [1/3]
void chrono::fsi::ChFsiProblemCartesian::Construct | ( | const ChVector3d & | box_size, |
const ChVector3d & | pos, | ||
int | side_flags | ||
) |
Construct SPH particles and optionally BCE markers in a box of given dimensions.
The reference position is the center of the bottom face of the box; in other words, SPH particles are generated above this location and BCE markers for the bottom boundary are generated below this location. If created, the BCE markers for the top, bottom, and side walls are adjacent to the SPH domain; 'side_flags' are boolean combinations of BoxSide enums.
- Parameters
-
box_size box dimensions pos reference position side_flags sides for which BCE markers are created
◆ Construct() [2/3]
void chrono::fsi::ChFsiProblemCartesian::Construct | ( | const std::string & | heightmap_file, |
double | length, | ||
double | width, | ||
const ChVector2d & | height_range, | ||
double | depth, | ||
bool | uniform_depth, | ||
const ChVector3d & | pos, | ||
int | side_flags | ||
) |
Construct SPH particles and optionally BCE markers from a given heightmap.
The image file is read with STB, using the number of channels defined in the input file and reading the image as 16-bit (8-bit images are automatically converted). Supported image formats: JPEG, PNG, BMP, GIF, PSD, PIC, PNM. Create the SPH particle grid locations for a patch of specified X and Y dimensions with optional translation. The height at each grid point is obtained through bilinear interpolation from the gray values in the provided heightmap image (with pure black corresponding to the lower height range and pure white to the upper height range). SPH particle grid locations are generated to cover the specified depth under each grid point. If created, BCE marker layers are generated below the bottom-most layer of SPH particles and on the sides of the patch. 'side_flags' are boolean combinations of BoxSide enums.
- Parameters
-
heightmap_file filename for the heightmap image length patch length (X direction) width patch width (Y direction) height_range height range (black to white level) depth fluid phase depth uniform_depth if true, bottom follows surface pos reference position side_flags sides for which BCE markers are created
◆ Construct() [3/3]
void chrono::fsi::ChFsiProblemCartesian::Construct | ( | const std::string & | sph_file, |
const std::string & | bce_file, | ||
const ChVector3d & | pos | ||
) |
Construct using information from the specified files.
The SPH particle and BCE marker locations are assumed to be provided on an integer grid. Locations in real space are generated using the specified grid separation value and the patch translated to the specified position.
- Parameters
-
sph_file filename with SPH grid particle positions bce_file filename with BCE grid marker positions pos reference position
◆ ConstructWaveTank()
std::shared_ptr< ChBody > chrono::fsi::ChFsiProblemCartesian::ConstructWaveTank | ( | WavemakerType | type, |
const ChVector3d & | pos, | ||
const ChVector3d & | box_size, | ||
double | depth, | ||
std::shared_ptr< ChFunction > | piston_fun, | ||
std::shared_ptr< WaveTankProfile > | profile = nullptr , |
||
bool | end_wall = true |
||
) |
Add a wave tank with a rigid-body wavemaker (piston-type or flap-type).
The wave tank can include a beach for wave dissipation, by specifying a profile for the bottom. By default (no profile functor provided), the tank is created with a flat horizontal bottom.
- Parameters
-
type wave generator type pos reference position box_size box dimensions depth fluid depth piston_fun piston actuation function profile profile for tank bottom end_wall include end_wall
The documentation for this class was generated from the following files:
- /builds/uwsbel/chrono/src/chrono_fsi/sph/ChFsiProblemSPH.h
- /builds/uwsbel/chrono/src/chrono_fsi/sph/ChFsiProblemSPH.cpp